react-native-code-push
Advanced tools
Comparing version 5.3.2 to 5.3.3
@@ -388,17 +388,13 @@ import { AcquisitionManager as Sdk } from "code-push/script/acquisition-sdk"; | ||
let message = null; | ||
const dialogButtons = [{ | ||
text: null, | ||
onPress:() => { | ||
doDownloadAndInstall() | ||
.then(resolve, reject); | ||
} | ||
}]; | ||
let installButtonText = null; | ||
const dialogButtons = []; | ||
if (remotePackage.isMandatory) { | ||
message = syncOptions.updateDialog.mandatoryUpdateMessage; | ||
dialogButtons[0].text = syncOptions.updateDialog.mandatoryContinueButtonLabel; | ||
installButtonText = syncOptions.updateDialog.mandatoryContinueButtonLabel; | ||
} else { | ||
message = syncOptions.updateDialog.optionalUpdateMessage; | ||
dialogButtons[0].text = syncOptions.updateDialog.optionalInstallButtonLabel; | ||
// Since this is an optional update, add another button | ||
installButtonText = syncOptions.updateDialog.optionalInstallButtonLabel; | ||
// Since this is an optional update, add a button | ||
// to allow the end-user to ignore it | ||
@@ -413,2 +409,12 @@ dialogButtons.push({ | ||
} | ||
// Since the install button should be placed to the | ||
// right of any other button, add it last | ||
dialogButtons.push({ | ||
text: installButtonText, | ||
onPress:() => { | ||
doDownloadAndInstall() | ||
.then(resolve, reject); | ||
} | ||
}) | ||
@@ -415,0 +421,0 @@ // If the update has a description, and the developer |
@@ -314,3 +314,52 @@ ## Android Setup | ||
``` | ||
If you are using [WIX React Native Navigation **version 2.x**](https://github.com/wix/react-native-navigation/tree/v2) based application, please do the following steps to integrate CodePush: | ||
1. As per React Native Navigation's documentation, `MainActivity.java` should extend `NavigationActivity`, no changes required to incorporate CodePush: | ||
```java | ||
import com.reactnativenavigation.NavigationActivity; | ||
public class MainActivity extends NavigationActivity { | ||
} | ||
``` | ||
2. Update the `MainApplication.java` file to use CodePush via the following changes: | ||
```java | ||
// ... | ||
import com.facebook.react.ReactInstanceManager; | ||
// Add CodePush imports | ||
import com.microsoft.codepush.react.CodePush; | ||
public class MainApplication extends NavigationApplication { | ||
@Override | ||
public boolean isDebug() { | ||
return BuildConfig.DEBUG; | ||
} | ||
@Override | ||
protected ReactGateway createReactGateway() { | ||
ReactNativeHost host = new NavigationReactNativeHost(this, isDebug(), createAdditionalReactPackages()) { | ||
@javax.annotation.Nullable | ||
@Override | ||
protected String getJSBundleFile() { | ||
return CodePush.getJSBundleFile(); | ||
} | ||
}; | ||
return new ReactGateway(this, isDebug(), host); | ||
} | ||
@Override | ||
public List<ReactPackage> createAdditionalReactPackages() { | ||
return Arrays.<ReactPackage>asList( | ||
new CodePush("deployment-key-here", getApplicationContext(), isDebug()) | ||
//,MainReactPackage , etc... | ||
} | ||
} | ||
``` | ||
#### Code Signing setup | ||
@@ -317,0 +366,0 @@ |
@@ -41,18 +41,6 @@ ## iOS Setup | ||
CodePush depends on an internal copy of the `SSZipArchive`, `JWT` and `Base64` libraries, so if your project already includes some of them (either directly or via a transitive dependency), then you can install a version of CodePush which excludes it by depending specifically on the needed subspecs: | ||
*NOTE: The above path needs to be relative to your app's `Podfile`, so adjust it as necessary.* | ||
```ruby | ||
# `SSZipArchive`, `JWT` and `Base64` already in use | ||
pod 'CodePush', :path => '../node_modules/react-native-code-push', :subspecs => ['Core'] | ||
*NOTE: `JWT` library should be >= version 3.0.x* | ||
# or for example | ||
# `SSZipArchive` and `Base64` already in use | ||
pod 'CodePush', :path => '../node_modules/react-native-code-push', :subspecs => ['Core', 'JWT'] | ||
``` | ||
*NOTE: The above paths needs to be relative to your app's `Podfile`, so adjust it as neccessary.* | ||
*NOTE: `JWT` library should be of version 3.0.x* | ||
2. Run `pod install` | ||
@@ -59,0 +47,0 @@ |
import { AcquisitionManager as Sdk } from "code-push/script/acquisition-sdk"; | ||
import { NativeEventEmitter } from "react-native"; | ||
import RestartManager from "./RestartManager"; | ||
import log from "./logging"; | ||
@@ -33,3 +34,10 @@ // This function is used to augment remote and local | ||
const downloadedPackage = await NativeCodePush.downloadUpdate(updatePackageCopy, !!downloadProgressCallback); | ||
reportStatusDownload && reportStatusDownload(this); | ||
if (reportStatusDownload) { | ||
reportStatusDownload(this) | ||
.catch((err) => { | ||
log(`Report download status failed: ${err}`); | ||
}); | ||
} | ||
return { ...downloadedPackage, ...local }; | ||
@@ -36,0 +44,0 @@ } finally { |
{ | ||
"name": "react-native-code-push", | ||
"version": "5.3.2", | ||
"version": "5.3.3", | ||
"description": "React Native plugin for the CodePush service", | ||
@@ -5,0 +5,0 @@ "main": "CodePush.js", |
@@ -71,3 +71,3 @@ [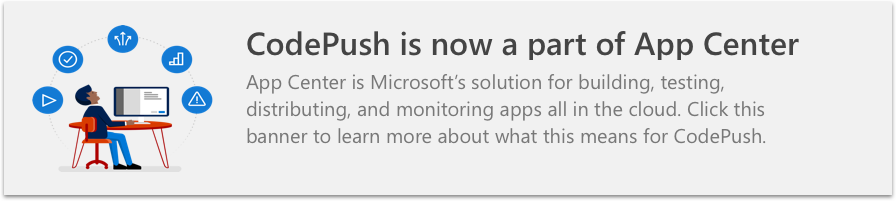](http://microsoft.github.io/code-push/) | ||
| v0.46-v0.53 | v5.1+ *(RN removed unused registration of JS modules)*| | ||
| v0.54 | v5.3+ *(Android Gradle Plugin 3.x integration)* | | ||
| v0.54-v0.55 | v5.3+ *(Android Gradle Plugin 3.x integration)* | | ||
| v0.55+ | TBD :) We work hard to respond to new RN releases, but they do occasionally break us. We will update this chart with each RN release, so that users can check to see what our "official" support is. | ||
@@ -362,3 +362,2 @@ | ||
| I've released new update but changes are not reflected | Be sure that you are running app in modes other than Debug. In Debug mode, React Native app always downloads JS bundle generated by packager, so JS bundle downloaded by CodePush does not apply. | ||
| Images dissappear after installing CodePush update | If your app is using the React Native assets system to load images (i.e. the `require(./foo.png)` syntax), then you **MUST** release your assets along with your JS bundle to CodePush. Follow [these instructions](#releasing-updates-javascript--images) to see how to do this. | | ||
| No JS bundle is being found when running your app against the iOS simulator | By default, React Native doesn't generate your JS bundle when running against the simulator. Therefore, if you're using `[CodePush bundleURL]`, and targetting the iOS simulator, you may be getting a `nil` result. This issue will be fixed in RN 0.22.0, but only for release builds. You can unblock this scenario right now by making [this change](https://github.com/facebook/react-native/commit/9ae3714f4bebdd2bcab4d7fdbf23acebdc5ed2ba) locally. | ||
@@ -365,0 +364,0 @@ |
@@ -15,2 +15,3 @@ var fs = require("fs"); | ||
var ignoreNodeModules = { ignore: "node_modules/**" }; | ||
var ignoreNodeModulesAndPods = { ignore: ["node_modules/**", "ios/Pods/**"] }; | ||
var appDelegatePaths = glob.sync("**/AppDelegate.+(mm|m)", ignoreNodeModules); | ||
@@ -154,3 +155,3 @@ | ||
function getPlistPath(){ | ||
var xcodeProjectPaths = glob.sync(`**/*.xcodeproj/project.pbxproj`, ignoreNodeModules); | ||
var xcodeProjectPaths = glob.sync(`**/*.xcodeproj/project.pbxproj`, ignoreNodeModulesAndPods); | ||
if (!xcodeProjectPaths){ | ||
@@ -198,2 +199,2 @@ return getDefaultPlistPath(); | ||
} | ||
} | ||
} |
Sorry, the diff of this file is not supported yet
Sorry, the diff of this file is not supported yet
New author
Supply chain riskA new npm collaborator published a version of the package for the first time. New collaborators are usually benign additions to a project, but do indicate a change to the security surface area of a package.
Found 1 instance in 1 package
1284918
1729
379
3