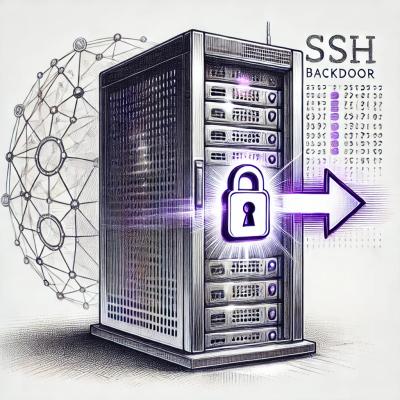
Research
Security News
Malicious npm Packages Inject SSH Backdoors via Typosquatted Libraries
Socket’s threat research team has detected six malicious npm packages typosquatting popular libraries to insert SSH backdoors.
react-native-kiwi
Advanced tools
A React Native wrapper for Kiwi subscription SDK
$ npm install react-native-kiwi --save
$ react-native link react-native-kiwi
You will be prompted for the configUrl
.
Add the following in your android/app/build.gradle
file, so the react-native-kiwi
dependencies are properly included.
repositories {
flatDir {
dirs project(':react-native-kiwi').file('libs')
}
}
Add to your AndroidManifest.xml
the following line into the <application>
tag.
<application ...>
...
<meta-data android:name="com.movile.kiwi.sdk.applicationKey" android:value="@string/kiwi_app_key"/>
...
</application>
And in your strings.xml
file add your kiwi_app_key
<string name="kiwi_app_key">YOUR_APP_KEY</string>
Enable Swift in your project
If you're not using Swift yet you'll need to create a new empty .swift
file (can be empty.swift
) doing right click in your project + New File.
It should offer you to create a Bridging-Header
file: accept it.
As a result, you will have 2 new files (a .swift
and a .h
) and your project should build correctly now.
Include required frameworks in your project
Create a New Group named Frameworks
if it's not already created.
Then do a right click on that folder, select Add files to ..., navigate to <PROJECT_ROOT>/node_modules/react-native-kiwi/ios/Frameworks
and finally select both, Falcon.framework
and openssl.framework
.
Finally go to Build Settings / Framework Search Paths and add this path $(SRCROOT)/../node_modules/react-native-kiwi/ios/Frameworks
, so the project will include these when building.
Add required configuration by Falcon
In Build Phases
add a Copy Files
phase, select Frameworks
as destination and add Falcon.framework
Add a Run Script
phase with the following script
APP_PATH="${TARGET_BUILD_DIR}/${WRAPPER_NAME}"
# This script loops through the frameworks embedded in the application and
# removes unused architectures.
find "$APP_PATH" -name '*.framework' -type d | while read -r FRAMEWORK
do
FRAMEWORK_EXECUTABLE_NAME=$(defaults read "$FRAMEWORK/Info.plist" CFBundleExecutable)
FRAMEWORK_EXECUTABLE_PATH="$FRAMEWORK/$FRAMEWORK_EXECUTABLE_NAME"
echo "Executable is $FRAMEWORK_EXECUTABLE_PATH"
EXTRACTED_ARCHS=()
for ARCH in $ARCHS
do
echo "Extracting $ARCH from $FRAMEWORK_EXECUTABLE_NAME"
lipo -extract "$ARCH" "$FRAMEWORK_EXECUTABLE_PATH" -o "$FRAMEWORK_EXECUTABLE_PATH-$ARCH"
EXTRACTED_ARCHS+=("$FRAMEWORK_EXECUTABLE_PATH-$ARCH")
done
echo "Merging extracted architectures: ${ARCHS}"
lipo -o "$FRAMEWORK_EXECUTABLE_PATH-merged" -create "${EXTRACTED_ARCHS[@]}"
rm "${EXTRACTED_ARCHS[@]}"
echo "Replacing original executable with thinned version"
rm "$FRAMEWORK_EXECUTABLE_PATH"
mv "$FRAMEWORK_EXECUTABLE_PATH-merged" "$FRAMEWORK_EXECUTABLE_PATH"
done
Init Falcon in your app boot
Add the following in your AppDelegate.m
file
NOTE: this guide was written assuming you've multiple environments. Could be simpler if you can hardcode the
kiwiAppKey
andconfigJsonUrl
values.
#import <Falcon/Falcon.h> // <- add this line
// ...
- (BOOL)application:(UIApplication *)application didFinishLaunchingWithOptions:(NSDictionary *)launchOptions
{
// ...
NSString* kiwiAppKey = [[[NSBundle mainBundle] infoDictionary] valueForKey:@"KiwiAppKey"];
NSString* configJsonUrl = [[[NSBundle mainBundle] infoDictionary] valueForKey:@"KiwiConfigUrl"];
[Falcon startWithKiwiAppKey:kiwiAppKey jsonUrl:configJsonUrl completion:^(NSError* error) {
if (error != nil) {
NSLog(@"Error starting Falcon", error);
}
}];
// ...
}
Setup credentials and configuration values
In the Info.plist
file
<key>KiwiAppKey</key>
<string>$(KIWI_APP_KEY)</string>
<key>KiwiConfigUrl</key>
<string>$(KIWI_CONFIG_URL)</string>
Finally, for each environment add in the .xcconfig
KIWI_APP_KEY=<THE_KIWI_APP_KEY>
KIWI_CONFIG_URL=<THE_CONFIG_URL>
IMPORTANT! The double slash (
//
) in the config url needs to be escaped using$()
so your file will end up like the following
KIWI_APP_KEY=abcd1234efgh5678
KIWI_CONFIG_URL=https:/$()/s3.amazonaws.com/project-name/config.json
In order to support Swift you will need to change the target to 9.3 or newer
Falcon.h
not found
Check you've added
$(SRCROOT)/../node_modules/react-native-kiwi/ios/Frameworks
in Build Settings / Framework Search Paths.
You need to enable the Always Embed Swift Standard Libraries in Build Settings (see this)
Chances are your project is not using Swift yet. Please check you've followed the Enable Swift in your project step
import Kiwi from 'react-native-kiwi'
Kiwi.getCountries().then(countries => console.log(countries))
// Retrieves the list of countries with the following format
// iOS:
// [{
// id: 'AR',
// code: 'AR',
// name: 'Argentina',
// ddi: 54
// }]
//
// Android:
// [{
// current: false,
// code: 'BR',
// name: 'Brasil'
// }]
Kiwi.getCarriers().then(carriers => console.log(carriers))
// Retrieves the list of carriers with the following format
// iOS:
// [{
// id: 1
// countryCode: 'BR',
// name: 'Vivo',
// active: true,
// shortCode: ...
// }]
//
// Android:
// [{
// id: 1
// sku: 'com.movile.marvel.br.vivo.1',
// countryCode: 'BR',
// countryName: 'Brasil',
// pricing: 'R$3,99 por semana',
// termsUrl: 'http://...someURL',
// allowUnsubscription: true,
// }]
Kiwi.sendPincode(carrierId, phoneNumber)
// Send pincode with restoration purpose to that carrierId and phoneNumber
//
// @param carrierId (number) the carrier id
// @param phoneNumber (string) the phone number used when the user subscribed
Kiwi.loginWithPincode(carrierId, phoneNumber, pincode)
// Send pincode with restoration purpose to that carrierId and phoneNumber
//
// @param carrierId (number) the carrier id
// @param phoneNumber (string) the phone number used when the user subscribed
// @param pincode (string) the pin code received by SMS
Kiwi.subscribe(carrierId, phoneNumber).then(response => console.log(response))
// [Android only]
// Starts the _subscription flow_
//
// @param carrierId (number)
// @param phoneNumber (string) with just numbers
//
// Resolves with an object with the following format
// {
// subscribed: true|false // indicates if the user is subscribed or not
// }
Kiwi.restoreSubscription(carrierId, phoneNumber).then(response => console.log(response))
// [Android only]
// Starts the _restore subscription flow_
//
// @param carrierId (number)
// @param phoneNumber (string) with just numbers
//
// Resolves with an object with the following format
// {
// subscribed: true|false // indicates if the user is subscribed or not
// }
Kiwi.redeemCode().then(response => console.log(response))
// [Android only]
// Starts the _code redeem flow_, the result should be stored because
// there is no way to check the subscription.
//
// Resolves with an object with the following format
// {
// subscribed: true|false // indicates if the user is subscribed or not
// }
Kiwi.unsubscribe(carrierId, phoneNumber).then(response => console.log(response))
// [Android only]
// Starts the _unsubscription flow_
//
// @param carrierId (number)
// @param phoneNumber (string) with just numbers
//
// Resolves with an object with the following format
// {
// subscribed: true|false // indicates if the user is subscribed or not
// }
Kiwi.isSubscribed(carrierId).then(subscribed => console.log(subscribed))
// Resolves with `true` if the user is subscribed for that carrierId, `false` if not
// @param carrierId (number)
Kiwi.submitFeedback(email, like, recommend, comment)
// [Android only]
// Submit feedback and resolves with `null`
//
// @param email (string) an email address
// @param like (number) from 1 to 5
// @param recommend (number) from 1 to 5
// @param comment (string) a free comment
Kiwi.detectMsisdn(countryCode)
// [Android only]
// Detects the country, carrier and phone number if possible
//
// @param countryCode (string) the two-letter country code
//
// Resolves with an object with the following format
// {
// countryCode: 'AR',
// carrierId: 12,
// phoneNumber: '1140654321',
// }
FAQs
A React Native wrapper for Kiwi subscription SDK
The npm package react-native-kiwi receives a total of 0 weekly downloads. As such, react-native-kiwi popularity was classified as not popular.
We found that react-native-kiwi demonstrated a not healthy version release cadence and project activity because the last version was released a year ago. It has 4 open source maintainers collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Research
Security News
Socket’s threat research team has detected six malicious npm packages typosquatting popular libraries to insert SSH backdoors.
Security News
MITRE's 2024 CWE Top 25 highlights critical software vulnerabilities like XSS, SQL Injection, and CSRF, reflecting shifts due to a refined ranking methodology.
Security News
In this segment of the Risky Business podcast, Feross Aboukhadijeh and Patrick Gray discuss the challenges of tracking malware discovered in open source softare.