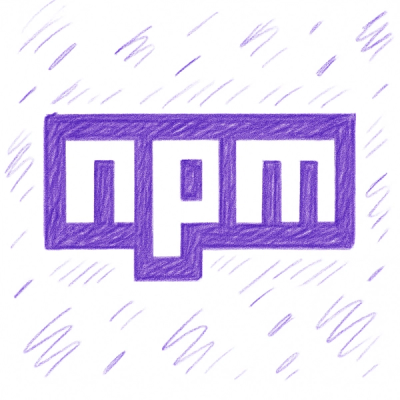
Security News
npm Adopts OIDC for Trusted Publishing in CI/CD Workflows
npm now supports Trusted Publishing with OIDC, enabling secure package publishing directly from CI/CD workflows without relying on long-lived tokens.
react-native-localize
Advanced tools
The react-native-localize package provides localization utilities for React Native applications. It helps in handling various localization tasks such as determining the user's locale, timezone, and country, as well as formatting numbers, dates, and times according to the user's locale.
Get User's Locale
This feature allows you to get the list of locales preferred by the user. The code sample retrieves the user's preferred locales and logs them to the console.
const { getLocales } = require('react-native-localize');
const locales = getLocales();
console.log(locales);
Get User's Timezone
This feature allows you to get the user's current timezone. The code sample retrieves the user's timezone and logs it to the console.
const { getTimeZone } = require('react-native-localize');
const timeZone = getTimeZone();
console.log(timeZone);
Get User's Country
This feature allows you to get the user's current country. The code sample retrieves the user's country and logs it to the console.
const { getCountry } = require('react-native-localize');
const country = getCountry();
console.log(country);
Format Number
This feature allows you to get the number formatting settings for the user's locale. The code sample retrieves the decimal and grouping separators and logs them to the console.
const { getNumberFormatSettings } = require('react-native-localize');
const { decimalSeparator, groupingSeparator } = getNumberFormatSettings();
console.log(`Decimal Separator: ${decimalSeparator}, Grouping Separator: ${groupingSeparator}`);
Handle Localization Changes
This feature allows you to handle changes in localization settings. The code sample adds an event listener that logs a message when localization settings change.
const { addEventListener, removeEventListener } = require('react-native-localize');
const handleLocalizationChange = () => {
console.log('Localization settings changed');
};
addEventListener('change', handleLocalizationChange);
// To remove the listener
// removeEventListener('change', handleLocalizationChange);
i18next is a popular internationalization framework for JavaScript that provides a complete solution for localizing your app. It supports multiple languages, pluralization, and interpolation. Compared to react-native-localize, i18next offers more comprehensive internationalization features but requires more setup.
react-intl is a library for internationalizing React applications. It provides components and an API to format dates, numbers, and strings, and to handle pluralization. While react-intl focuses on formatting and message handling, react-native-localize provides more utilities for determining the user's locale and timezone.
expo-localization is a package from the Expo ecosystem that provides localization utilities similar to react-native-localize. It can determine the user's locale, timezone, and country. However, expo-localization is designed to work seamlessly with Expo projects, whereas react-native-localize can be used in any React Native project.
A toolbox for your React Native app localization.
This library follows the React Native releases support policy.
It is supporting the latest version, and the two previous minor series.
$ npm install --save react-native-localize
# --- or ---
$ yarn add react-native-localize
Don't forget to run pod install
after that !
If you're using Expo, you can specify the supported locales in your app.json
or app.config.js
using the config plugin.
This enables Android 13+ and iOS to display the available locales in the system settings, allowing users to select their preferred language for your app.
{
"expo": {
"plugins": [["react-native-localize", { "locales": ["en", "fr"] }]]
}
}
Alternatively, if you want to define different locales for iOS and Android, you can use:
{
"expo": {
"plugins": [
[
"react-native-localize",
{
"locales": {
"android": ["en"],
"ios": ["en", "fr"]
}
}
]
]
}
}
This package supports react-native-web
. Follow their official guide to configure webpack
.
import { getCurrencies, getLocales } from "react-native-localize";
console.log(getLocales());
console.log(getCurrencies());
Prismy (a proud sponsor of this library ♥️) helps teams ship localized apps faster with an AI-driven, GitHub-native workflow. Want to supercharge your react-native-localize
setup? Here's how Prismy makes localization seamless:
No setup headaches. Just clean, context-aware translations, out of the box.
👉 Learn more at prismy.io
Returns the user preferred locales, in order.
type getLocales = () => Array<{
languageCode: string;
scriptCode?: string;
countryCode: string;
languageTag: string;
isRTL: boolean;
}>;
import { getLocales } from "react-native-localize";
console.log(getLocales());
/* -> [
{ countryCode: "GB", languageTag: "en-GB", languageCode: "en", isRTL: false },
{ countryCode: "US", languageTag: "en-US", languageCode: "en", isRTL: false },
{ countryCode: "FR", languageTag: "fr-FR", languageCode: "fr", isRTL: false },
] */
Returns number formatting settings.
type getNumberFormatSettings = () => {
decimalSeparator: string;
groupingSeparator: string;
};
import { getNumberFormatSettings } from "react-native-localize";
console.log(getNumberFormatSettings());
/* -> {
decimalSeparator: ".",
groupingSeparator: ",",
} */
Returns the user preferred currency codes, in order.
type getCurrencies = () => string[];
import { getCurrencies } from "react-native-localize";
console.log(getCurrencies());
// -> ["EUR", "GBP", "USD"]
Returns the user current country code (based on its device locale, not on its position).
type getCountry = () => string;
import { getCountry } from "react-native-localize";
console.log(getCountry());
// -> "FR"
Devices using Latin American regional settings will return "UN" instead of "419", as the latter is not a standard country code.
Returns the user preferred calendar format.
type getCalendar = () =>
| "gregorian"
| "buddhist"
| "coptic"
| "ethiopic"
| "ethiopic-amete-alem"
| "hebrew"
| "indian"
| "islamic"
| "islamic-umm-al-qura"
| "islamic-civil"
| "islamic-tabular"
| "iso8601"
| "japanese"
| "persian";
import { getCalendar } from "react-native-localize";
console.log(getCalendar());
// -> "gregorian"
Returns the user preferred temperature unit.
type getTemperatureUnit = () => "celsius" | "fahrenheit";
import { getTemperatureUnit } from "react-native-localize";
console.log(getTemperatureUnit());
// -> "celsius"
Returns the user preferred timezone (based on its device settings, not on its position).
type getTimeZone = () => string;
import { getTimeZone } from "react-native-localize";
console.log(getTimeZone());
// -> "Europe/Paris"
Returns true
if the user prefers 24h clock format, false
if they prefer 12h clock format.
type uses24HourClock = () => boolean;
import { uses24HourClock } from "react-native-localize";
console.log(uses24HourClock());
// -> true
Returns true
if the user prefers metric measure system, false
if they prefer imperial.
type usesMetricSystem = () => boolean;
import { usesMetricSystem } from "react-native-localize";
console.log(usesMetricSystem());
// -> true
Tells if the automatic date & time setting is enabled on the phone. Android only
type usesAutoDateAndTime = () => boolean | undefined;
import { usesAutoDateAndTime } from "react-native-localize";
console.log(usesAutoDateAndTime()); // true or false
Tells if the automatic time zone setting is enabled on the phone. Android only
type usesAutoTimeZone = () => boolean | undefined;
import { usesAutoTimeZone } from "react-native-localize";
console.log(usesAutoTimeZone());
Returns the best language tag possible and its reading direction. Useful to pick the best translation available.
[!NOTE]
It respects the user preferred languages list order (see explanations).
type findBestLanguageTag = (
languageTags: string[],
) => { languageTag: string; isRTL: boolean } | void;
import { findBestLanguageTag } from "react-native-localize";
console.log(findBestLanguageTag(["en-US", "en", "fr"]));
// -> { languageTag: "en-US", isRTL: false }
Opens the app language settings.
[!WARNING]
This feature is available only on Android 13+ and require configuring your app's supported locales.
type openAppLanguageSettings = () => Promise<void>;
import { openAppLanguageSettings } from "react-native-localize";
openAppLanguageSettings("application").catch((error) => {
console.warn("Cannot open app language settings", error);
});
Browse the files in the /example directory.
You can add / remove supported localizations in your Xcode project infos:
Because it's a native module, you need to mock this package.
The package provides a default mock you may import in your __mocks__
directory:
// __mocks__/react-native-localize.ts
export * from "react-native-localize/mock"; // or "react-native-localize/mock/jest"
This module is provided as is, I work on it in my free time.
If you or your company uses it in a production app, consider sponsoring this project 💰. You also can contact me for premium enterprise support: help with issues, prioritize bugfixes, feature requests, etc.
FAQs
A toolbox for your React Native app localization.
The npm package react-native-localize receives a total of 268,547 weekly downloads. As such, react-native-localize popularity was classified as popular.
We found that react-native-localize demonstrated a healthy version release cadence and project activity because the last version was released less than a year ago. It has 1 open source maintainer collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
npm now supports Trusted Publishing with OIDC, enabling secure package publishing directly from CI/CD workflows without relying on long-lived tokens.
Research
/Security News
A RubyGems malware campaign used 60 malicious packages posing as automation tools to steal credentials from social media and marketing tool users.
Security News
The CNA Scorecard ranks CVE issuers by data completeness, revealing major gaps in patch info and software identifiers across thousands of vulnerabilities.