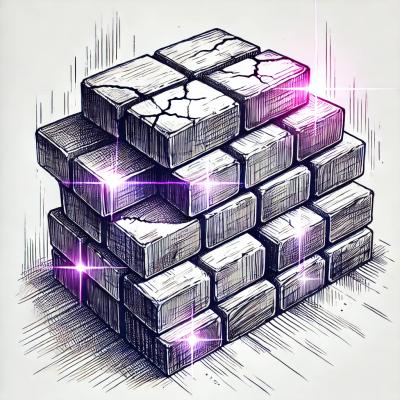
Security News
Input Validation Vulnerabilities Dominate MITRE's 2024 CWE Top 25 List
MITRE's 2024 CWE Top 25 highlights critical software vulnerabilities like XSS, SQL Injection, and CSRF, reflecting shifts due to a refined ranking methodology.
react-native-mmkv
Advanced tools
The fastest key/value storage for React Native. ~30x faster than AsyncStorage! Works on Android, iOS and Web.
react-native-mmkv is a fast, small, and easy-to-use key-value storage library for React Native. It leverages Facebook's MMKV storage library to provide efficient and secure storage solutions for mobile applications.
Basic Key-Value Storage
This feature allows you to store and retrieve basic key-value pairs. The code sample demonstrates how to set and get a string value using MMKV.
import MMKVStorage from 'react-native-mmkv-storage';
const MMKV = new MMKVStorage.Loader().initialize();
// Set a value
MMKV.setString('username', 'john_doe');
// Get a value
const username = MMKV.getString('username');
console.log(username); // Output: john_doe
Object Storage
This feature allows you to store and retrieve objects. The code sample demonstrates how to set and get an object using MMKV.
import MMKVStorage from 'react-native-mmkv-storage';
const MMKV = new MMKVStorage.Loader().initialize();
const user = { name: 'John Doe', age: 30 };
// Set an object
MMKV.setMap('user', user);
// Get an object
const storedUser = MMKV.getMap('user');
console.log(storedUser); // Output: { name: 'John Doe', age: 30 }
Encryption
This feature allows you to store data securely using encryption. The code sample demonstrates how to set and get an encrypted string value using MMKV.
import MMKVStorage from 'react-native-mmkv-storage';
const MMKV = new MMKVStorage.Loader().withEncryption().initialize();
// Set a value
MMKV.setString('secret', 'my_secret_value');
// Get a value
const secret = MMKV.getString('secret');
console.log(secret); // Output: my_secret_value
Multi-Instance Support
This feature allows you to create multiple instances of storage, which can be useful for separating different types of data. The code sample demonstrates how to set and get values from different instances using MMKV.
import MMKVStorage from 'react-native-mmkv-storage';
const userStorage = new MMKVStorage.Loader().withInstanceID('user').initialize();
const settingsStorage = new MMKVStorage.Loader().withInstanceID('settings').initialize();
// Set values in different instances
userStorage.setString('username', 'john_doe');
settingsStorage.setBool('darkMode', true);
// Get values from different instances
const username = userStorage.getString('username');
const darkMode = settingsStorage.getBool('darkMode');
console.log(username); // Output: john_doe
console.log(darkMode); // Output: true
react-native-async-storage is a simple, unencrypted, asynchronous, persistent, key-value storage system that is global to the app. It is more widely used and has a larger community but lacks the performance and encryption features of react-native-mmkv.
react-native-sensitive-info provides secure storage for sensitive data, such as login credentials. It uses the device's secure storage mechanisms (Keychain on iOS and Keystore on Android). While it offers strong security, it may not be as fast as react-native-mmkv for general key-value storage.
redux-persist is a library used to persist and rehydrate a Redux store. It supports various storage backends, including AsyncStorage. While it is not a direct competitor, it can be used for state persistence in applications using Redux, offering more flexibility in terms of storage backends.
react-native-mmkv is sponsored by getstream.io.
Try the React Native Chat tutorial 💬
StorageBenchmark compares popular storage libraries against each other by reading a value from storage for 1000 times:
MMKV vs other storage libraries: Reading a value from Storage 1000 times.
Measured in milliseconds on an iPhone 11 Pro, lower is better.
yarn add react-native-mmkv
cd ios && pod install
expo install react-native-mmkv
expo prebuild
To create a new instance of the MMKV storage, use the MMKV
constructor. It is recommended that you re-use this instance throughout your entire app instead of creating a new instance each time, so export
the storage
object.
import { MMKV } from 'react-native-mmkv'
export const storage = new MMKV()
This creates a new storage instance using the default MMKV storage ID (mmkv.default
).
import { MMKV } from 'react-native-mmkv'
export const storage = new MMKV({
id: `user-${userId}-storage`,
path: `${USER_DIRECTORY}/storage`,
encryptionKey: 'hunter2'
})
This creates a new storage instance using a custom MMKV storage ID. By using a custom storage ID, your storage is separated from the default MMKV storage of your app.
The following values can be configured:
id
: The MMKV instance's ID. If you want to use multiple instances, use different IDs. For example, you can separte the global app's storage and a logged-in user's storage. (required if path
or encryptionKey
fields are specified, otherwise defaults to: 'mmkv.default'
)path
: The MMKV instance's root path. By default, MMKV stores file inside $(Documents)/mmkv/
. You can customize MMKV's root directory on MMKV initialization (documentation: iOS / Android)encryptionKey
: The MMKV instance's encryption/decryption key. By default, MMKV stores all key-values in plain text on file, relying on iOS's/Android's sandbox to make sure the file is encrypted. Should you worry about information leaking, you can choose to encrypt MMKV. (documentation: iOS / Android)storage.set('user.name', 'Marc')
storage.set('user.age', 21)
storage.set('is-mmkv-fast-asf', true)
const username = storage.getString('user.name') // 'Marc'
const age = storage.getNumber('user.age') // 21
const isMmkvFastAsf = storage.getBoolean('is-mmkv-fast-asf') // true
// checking if a specific key exists
const hasUsername = storage.contains('user.name')
// getting all keys
const keys = storage.getAllKeys() // ['user.name', 'user.age', 'is-mmkv-fast-asf']
// delete a specific key + value
storage.delete('user.name')
// delete all keys
storage.clearAll()
const user = {
username: 'Marc',
age: 21
}
// Serialize the object into a JSON string
storage.set('user', JSON.stringify(user))
// Deserialize the JSON string into an object
const jsonUser = storage.getString('user') // { 'username': 'Marc', 'age': 21 }
const userObject = JSON.parse(jsonUser)
// encrypt all data with a private key
storage.recrypt('hunter2')
// remove encryption
storage.recrypt(undefined)
A mocked MMKV instance is automatically used when testing with Jest, so you will be able to use new MMKV()
as per normal in your tests. Refer to example/test/MMKV.test.ts for an example.
As the library uses JSI for synchronous native methods access, remote debugging (e.g. with Chrome) is no longer possible. Instead, you should use Flipper.
Use flipper-plugin-react-native-mmkv to debug your MMKV storage using Flipper. You can also simply console.log
an MMKV instance.
react-native-mmkv is provided as is, I work on it in my free time.
If you're integrating react-native-mmkv in a production app, consider funding this project and contact me to receive premium enterprise support, help with issues, prioritize bugfixes, request features, help at integrating react-native-mmkv, and more.
See the contributing guide to learn how to contribute to the repository and the development workflow.
MIT
FAQs
The fastest key/value storage for React Native. ~30x faster than AsyncStorage! Works on Android, iOS and Web.
We found that react-native-mmkv demonstrated a healthy version release cadence and project activity because the last version was released less than a year ago. It has 1 open source maintainer collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
MITRE's 2024 CWE Top 25 highlights critical software vulnerabilities like XSS, SQL Injection, and CSRF, reflecting shifts due to a refined ranking methodology.
Security News
In this segment of the Risky Business podcast, Feross Aboukhadijeh and Patrick Gray discuss the challenges of tracking malware discovered in open source softare.
Research
Security News
A threat actor's playbook for exploiting the npm ecosystem was exposed on the dark web, detailing how to build a blockchain-powered botnet.