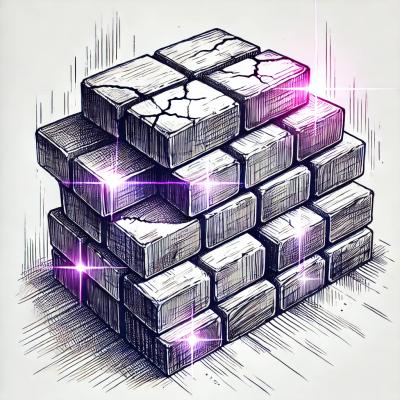
Security News
Input Validation Vulnerabilities Dominate MITRE's 2024 CWE Top 25 List
MITRE's 2024 CWE Top 25 highlights critical software vulnerabilities like XSS, SQL Injection, and CSRF, reflecting shifts due to a refined ranking methodology.
react-native-quick-sqlite
Advanced tools
Copy typeORM patch-package from example dir npm i react-native-quick-sqlite typeorm npx pod-install Enable decorators and configure babel
This library provides a low-level API to execute SQL queries, fast bindings via JSI.
Inspired/compatible with react-native-sqlite-storage and react-native-sqlite2.
interface QueryResult {
status: 0 | 1; // 0 for correct execution
message: string; // if status === 1, here you will find error description
rows: any[];
insertId?: number;
}
interface BatchQueryResult {
status?: 0 | 1;
rowsAffected?: number;
message?: string;
}
interface ISQLite {
open: (dbName: string, location?: string) => { status: 0 | 1 };
close: (dbName: string) => { status: 0 | 1 };
executeSql: (
dbName: string,
query: string,
params: any[] | undefined
) => QueryResult;
asyncExecuteSql: (
dbName: string,
query: string,
params: any[] | undefined,
cb: (res: QueryResult) => void
) => void;
executeSqlBatch: (
dbName: string,
commands: SQLBatchParams[]
) => BatchQueryResult;
asyncExecuteSqlBatch: (
dbName: string,
commands: SQLBatchParams[],
cb: (res: BatchQueryResult) => void
) => void;
loadSqlFile: (dbName: string, location: string) => FileLoadResult;
asyncLoadSqlFile: (
dbName: string,
location: string,
cb: (res: FileLoadResult) => void
) => void;
}
// Import as early as possible, auto-installs bindings
import 'react-native-quick-sqlite';
// `sqlite` is a globally registered object, so you can directly call it from anywhere in your javascript
// the import on the top of the file only registers typescript types but it is not mandatory
const dbOpenResult = sqlite.open('myDatabase', 'databases');
// status === 1, operation failed
if (dbOpenResult.status) {
console.error('Database could not be opened');
}
let result = sqlite.executeSql('myDatabase', 'SELECT somevalue FROM sometable');
if (!result.status) {
// result.status undefined or 0 === sucess
for (let i = 0; i < result.rows.length; i++) {
const row = result.rows.item(i);
console.log(row.somevalue);
}
}
result = sqlite.executeSql(
'myDatabase',
'UPDATE sometable set somecolumn = ? where somekey = ?',
[0, 1]
);
if (!result.status) {
// result.status undefined or 0 === sucess
console.log(`Update affected ${result.rowsAffected} rows`);
}
Batch execution allows transactional execution of a set of commands
const commands = [
['CREATE TABLE TEST (id integer)'],
['INSERT INTO TABLE TEST (id) VALUES (?)', [1]][
('INSERT INTO TABLE TEST (id) VALUES (?)', [2])
][('INSERT INTO TABLE TEST (id) VALUES (?)', [[3], [4], [5], [6]])],
];
const result = sqlite.executeSqlBatch('myDatabase', commands);
if (!result.status) {
// result.status undefined or 0 === sucess
console.log(`Batch affected ${result.rowsAffected} rows`);
}
Async versions are also available if you have too much SQL to execute
sqlite.asyncExecuteSql('myDatabase', 'SELECT * FROM "User";', [], (result) => {
if (result.status === 0) {
console.log('users', result.rows);
}
});
This package offers a low-level API to raw execute SQL queries. I strongly recommend to use TypeORM (with patch-package). TypeORM already has a sqlite-storage driver. In the example
project on the patch
folder you can a find a patch for TypeORM.
Follow the instructions to make TypeORM work with React Native (enable decorators, configure babel, etc), then apply the example patch via patch-package.
If you want to learn how to make your own JSI module buy my JSI/C++ Cheatsheet, I'm also available for freelance work!
react-native-quick-sqlite is licensed under MIT.
FAQs
Fast SQLite for react-native
The npm package react-native-quick-sqlite receives a total of 5,527 weekly downloads. As such, react-native-quick-sqlite popularity was classified as popular.
We found that react-native-quick-sqlite demonstrated a healthy version release cadence and project activity because the last version was released less than a year ago. It has 0 open source maintainers collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
MITRE's 2024 CWE Top 25 highlights critical software vulnerabilities like XSS, SQL Injection, and CSRF, reflecting shifts due to a refined ranking methodology.
Security News
In this segment of the Risky Business podcast, Feross Aboukhadijeh and Patrick Gray discuss the challenges of tracking malware discovered in open source softare.
Research
Security News
A threat actor's playbook for exploiting the npm ecosystem was exposed on the dark web, detailing how to build a blockchain-powered botnet.