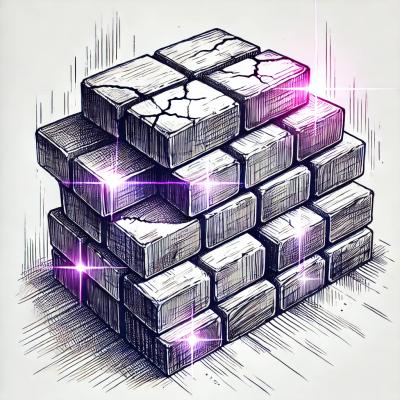
Security News
Input Validation Vulnerabilities Dominate MITRE's 2024 CWE Top 25 List
MITRE's 2024 CWE Top 25 highlights critical software vulnerabilities like XSS, SQL Injection, and CSRF, reflecting shifts due to a refined ranking methodology.
react-native-quick-sqlite
Advanced tools
Copy typeORM patch-package from example dir yarn add react-native-quick-sqlite typeorm npx pod-install Enable decorators and configure babel
yarn add react-native-quick-sqlite npx pod-install
Quick SQLite embeds the latest version of SQLite and provides a low-level API to execute SQL queries, uses fast bindings via JSI. By using an embedded SQLite you get access the latest security patches and latest features.
Inspired/compatible with react-native-sqlite-storage and react-native-sqlite2.
interface QueryResult {
status?: 0 | 1; // 0 for correct execution
insertId?: number;
rowsAffected: number;
message?: string;
rows?: {
/** Raw array with all dataset */
_array: any[];
/** The length of the dataset */
length: number;
};
/**
* Query metadata, available only for select query results
*/
metadata?: ColumnMetadata[];
}
/**
* Column metadata
* Describes some information about columns fetched by the query
* columnDeclaredType - declared column type for this column, when fetched directly from a table or a View resulting from a table column. "UNKNOWN" for dynamic values, like function returned ones.
*/
interface ColumnMetadata = {
columnName: string;
columnDeclaredType: string;
columnIndex: number;
};
interface BatchQueryResult {
status?: 0 | 1;
rowsAffected?: number;
message?: string;
}
interface ISQLite {
open: (dbName: string, location?: string) => { status: 0 | 1 };
close: (dbName: string) => { status: 0 | 1 };
executeSql: (
dbName: string,
query: string,
params: any[] | undefined
) => QueryResult;
asyncExecuteSql: (
dbName: string,
query: string,
params: any[] | undefined,
cb: (res: QueryResult) => void
) => void;
executeSqlBatch: (
dbName: string,
commands: SQLBatchParams[]
) => BatchQueryResult;
asyncExecuteSqlBatch: (
dbName: string,
commands: SQLBatchParams[],
cb: (res: BatchQueryResult) => void
) => void;
loadSqlFile: (dbName: string, location: string) => FileLoadResult;
asyncLoadSqlFile: (
dbName: string,
location: string,
cb: (res: FileLoadResult) => void
) => void;
}
Import as early as possible, auto-installs bindings in a thread-safe manner.
// Thanks to @mrousavy for this installation method, see one example: https://github.com/mrousavy/react-native-mmkv/blob/75b425db530e26cf10c7054308583d03ff01851f/src/createMMKV.ts#L56
import 'react-native-quick-sqlite';
// Afterwards `sqlite` is a globally registered object, so you can directly call it from anywhere in your javascript
const dbOpenResult = sqlite.open('myDatabase', 'databases');
// status === 1, operation failed
if (dbOpenResult.status) {
console.error('Database could not be opened');
}
The basic query is synchronous, it will block rendering on large operations, below there are async versions.
let { status, rows } = sqlite.executeSql(
'myDatabase',
'SELECT somevalue FROM sometable'
);
if (!status) {
rows.forEach((row) => {
console.log(row);
});
}
let { status, rowsAffected } = sqlite.executeSql(
'myDatabase',
'UPDATE sometable SET somecolumn = ? where somekey = ?',
[0, 1]
);
if (!status) {
console.log(`Update affected ${rowsAffected} rows`);
}
Transactions are supported. However, due to the library being opinionated and mostly not throwing errors you need to return a boolean (true for correct execution, false for incorrect execution) to either commit or rollback the transaction.
JSI bindings are fast but there is still some overhead calling executeSql
for single queries, if you want to execute a large set of commands as fast as possible you should use the executeSqlBatch
method below, it still uses transactions, but only transmits data between JS and native once.
sqlite.transaction('myDatabase', (tx) => {
const {
status,
} = tx.executeSql('UPDATE sometable SET somecolumn = ? where somekey = ?', [
0,
1,
]);
if (status) {
return false;
}
return true;
});
Batch execution allows transactional execution of a set of commands
const commands = [
['CREATE TABLE TEST (id integer)'],
['INSERT INTO TABLE TEST (id) VALUES (?)', [1]][
('INSERT INTO TABLE TEST (id) VALUES (?)', [2])
][('INSERT INTO TABLE TEST (id) VALUES (?)', [[3], [4], [5], [6]])],
];
const result = sqlite.executeSqlBatch('myDatabase', commands);
if (!result.status) {
// result.status undefined or 0 === success
console.log(`Batch affected ${result.rowsAffected} rows`);
}
In some scenarios, dynamic applications may need to get some metadata information about the returned result set.
This can be done testing the returned data directly, but in some cases may not be enough, for example when data is stored outside sqlite datatypes. When fetching data directly from tables or views linked to table columns, SQLite is able to identify the table declared types:
let { status, metadata } = sqlite.executeSql(
'myDatabase',
'SELECT int_column_1, bol_column_2 FROM sometable'
);
if (!status) {
metadata.forEach((column) => {
// Output:
// int_column_1 - INTEGER
// bol_column_2 - BOOLEAN
console.log(`${column.columnName} - ${column.columnDeclaredType}`);
});
}
You might have too much SQL to process and it will cause your application to freeze. There are async versions for some of the operations. This will offload the SQLite processing to a different thread.
sqlite.asyncExecuteSql(
'myDatabase',
'SELECT * FROM "User";',
[],
({ status, rows }) => {
if (status === 0) {
console.log('users', rows);
}
}
);
This package offers a low-level API to raw execute SQL queries. I strongly recommend to use TypeORM (with patch-package). TypeORM already has a sqlite-storage driver. In the example
project on the patch
folder you can a find a patch for TypeORM.
Follow the instructions to make TypeORM work with React Native (enable decorators, configure babel, etc), then apply the example patch via patch-package.
If you want to learn how to make your own JSI module buy my JSI/C++ Cheatsheet, I'm also available for freelance work!
react-native-quick-sqlite is licensed under MIT.
FAQs
Fast SQLite for react-native
The npm package react-native-quick-sqlite receives a total of 5,527 weekly downloads. As such, react-native-quick-sqlite popularity was classified as popular.
We found that react-native-quick-sqlite demonstrated a healthy version release cadence and project activity because the last version was released less than a year ago. It has 0 open source maintainers collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
MITRE's 2024 CWE Top 25 highlights critical software vulnerabilities like XSS, SQL Injection, and CSRF, reflecting shifts due to a refined ranking methodology.
Security News
In this segment of the Risky Business podcast, Feross Aboukhadijeh and Patrick Gray discuss the challenges of tracking malware discovered in open source softare.
Research
Security News
A threat actor's playbook for exploiting the npm ecosystem was exposed on the dark web, detailing how to build a blockchain-powered botnet.