react-native-smart-scroll-view
Advanced tools
Comparing version 1.3.0 to 1.3.1
{ | ||
"name": "react-native-smart-scroll-view", | ||
"version": "1.3.0", | ||
"version": "1.3.1", | ||
"description": "Handles keyboard events and auto adjusts content to be visible above keyboard on focus. Further scrolling features available.", | ||
@@ -5,0 +5,0 @@ "main": "SmartScrollView.js", |
@@ -5,2 +5,4 @@ # react-native-smart-scroll-view | ||
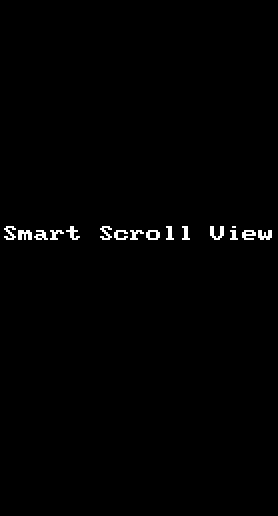 | ||
A pure JS React Native Component for IOS. | ||
@@ -18,3 +20,2 @@ | ||
- [Example](#example) | ||
- [Installation](#installation) | ||
@@ -25,13 +26,2 @@ - [Properties](#properties) | ||
### Example | ||
Here is the smart-scroll-view in action. To run the code yourself open and run the Xcode project. | ||
```bash | ||
open SuperScrollingFormExample/ios/SuperScrollingFormExample.xcodeproj | ||
``` | ||
 | ||
### Installation | ||
@@ -51,3 +41,3 @@ | ||
| :------------ |:---------------:| :---------------:| :-----| | ||
| forceFocusFieldIndex | `undefined` |`number` | Force scroll the view to the TextInput field at the specified index (Chosen TextInputs components indexed in order from 0) | | ||
| forceFocusField | `undefined` |`number` or `string` | Force scroll the view to the TextInput field at the specified index (smart children indexed in order from 0) or scrollRef you have given to your smart child (see smartScrollOptions below) | | ||
| scrollContainerStyle | `{flex: 1}` | `number` | Style options for the View that wraps the ScrollView, the ScrollView will take up all available space. | | ||
@@ -60,2 +50,3 @@ | scrollPadding | `5` | `number` | Padding between the top of the keyboard/ScrollView and the focused TextInput field | | ||
| onScroll | `() => {}` | `func` | Set to the ScrollView onScroll function. It will be called alongside our own | | ||
| onRefFocus | `()=>{}` | `func` | Gives back the scrollRef of the node whenever a smart component is focused | | ||
@@ -73,2 +64,3 @@ #### TextInput Props | ||
| onSubmitEditing(next) | `func` | Optional function that takes a callback. When invoked, the callback will focus the next TextInput field. If no function is specified the next TextInput field is focused. Example: `(next) => { if (condition) { next() } }` | | ||
| scrollRef | `string` | Way to reference a particular component to use the forceFocusField to have control of where the focus is | | ||
@@ -86,41 +78,12 @@ | ||
This a simple example of the SmartScrollView in use for an input form. To see a more exciting example visit this [example](https://github.com/jrans/react-native-smart-scroll-view/blob/master/SuperScrollingFormExample/Example.js). | ||
Code for the above gif is found [here](https://github.com/jrans/react-native-smart-scroll-view/blob/master/complexFormExample.js) | ||
```js | ||
Here is another [example](https://github.com/jrans/react-native-smart-scroll-view/blob/master/SuperScrollingFormExample/Example.js) of the smart-scroll-view in action. | ||
import SmartScrollView from 'react-native-smart-scroll-view'; | ||
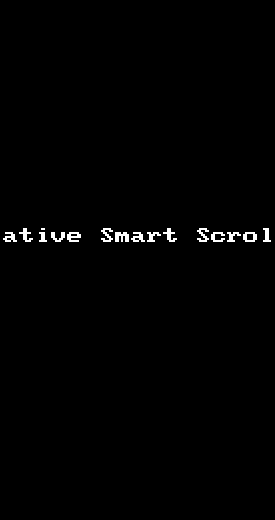 | ||
class Example extends Component { | ||
To run the code yourself and play around open and run the Xcode project. | ||
render () { | ||
return ( | ||
<View style={styles.container}> | ||
<View style={styles.header}/> | ||
<SmartScrollView | ||
contentContainerStyle = { styles.contentContainerStyle } | ||
> | ||
<TextInput | ||
superScrollOptions = {{ | ||
moveToNext: true, | ||
type: 'text' | ||
}} | ||
style = {styles.textInput} | ||
autoCorrect = {false} | ||
/> | ||
<View> | ||
<TextInput | ||
superScrollOptions = {{ | ||
type: 'text' | ||
}} | ||
style = {styles.textInput} | ||
autoCorrect = {false} | ||
/> | ||
</View> | ||
</SmartScrollView> | ||
</View> | ||
) | ||
} | ||
} | ||
```bash | ||
open SuperScrollingFormExample/ios/SuperScrollingFormExample.xcodeproj | ||
``` | ||
@@ -127,0 +90,0 @@ |
@@ -44,4 +44,4 @@ import React, { | ||
componentDidMount() { | ||
if (this.props.forceFocusFieldIndex !== undefined){ | ||
this._focusField('input_' + this.props.forceFocusFieldIndex) | ||
if (this.props.forceFocusField !== this.state.focusedField){ | ||
this._focusField('input_' + this.props.forceFocusField) | ||
} | ||
@@ -61,4 +61,4 @@ this._listeners = [ | ||
componentWillReceiveProps(props) { | ||
if (props.forceFocusFieldIndex !== undefined){ | ||
this._focusField('input_' + props.forceFocusFieldIndex) | ||
if (props.forceFocusField !== this.state.focusedField){ | ||
this._focusField('input_' + props.forceFocusField) | ||
} | ||
@@ -87,4 +87,6 @@ } | ||
_refCreator (ref) { | ||
return component => this[ref] = component | ||
_refCreator () { | ||
const refs = arguments; | ||
return component => Object.keys(refs).forEach(i => this[refs[i]] = component); | ||
} | ||
@@ -107,10 +109,16 @@ | ||
_focusNode (ref) { | ||
const { | ||
scrollPosition, | ||
scrollWindowHeight, | ||
} = this.state; | ||
const { | ||
scrollPadding, | ||
onRefFocus | ||
} = this.props; | ||
const num = React.findNodeHandle(this._smartScroll); | ||
const strippedBackRef = ref.slice('input_'.length); | ||
setTimeout(() => { | ||
const { | ||
scrollPosition, | ||
scrollWindowHeight, | ||
} = this.state; | ||
const { scrollPadding } = this.props; | ||
const num = React.findNodeHandle(this._smartScroll); | ||
onRefFocus(strippedBackRef); | ||
this.setState({focusedField: strippedBackRef}) | ||
this[ref].measureLayout(num, (X,Y,W,H) => { | ||
@@ -140,3 +148,2 @@ const py = Y - scrollPosition; | ||
setTimeout(()=> this._container.measureLayout(1, (x,y,width,height) => { | ||
console.log(x,y,width,height) | ||
this._findScrollWindowHeight = (keyboardHeight) => { | ||
@@ -163,24 +170,24 @@ const spaceBelow = screenHeight - y - height; | ||
if (smartScrollOptions.type === 'text') { | ||
if (smartScrollOptions.type !== undefined) { | ||
const ref = 'input_' + inputIndex; | ||
smartProps.onFocus = () => { | ||
smartProps.onFocus = element.props.onFocus && element.props.onFocus(); | ||
this._focusNode(ref) | ||
}; | ||
smartProps.ref = this._refCreator(ref); | ||
smartProps.ref = this._refCreator(ref, smartScrollOptions.scrollRef && 'input_' + smartScrollOptions.scrollRef); | ||
if (smartScrollOptions.moveToNext === true) { | ||
const nextRef = 'input_' + (inputIndex+1); | ||
const focusNextField = () => this._focusField(nextRef) | ||
smartProps.blurOnSubmit = false; | ||
smartProps.onSubmitEditing = smartScrollOptions.onSubmitEditing ? | ||
() => smartScrollOptions.onSubmitEditing(focusNextField) : | ||
focusNextField | ||
if (smartScrollOptions.type === 'text') { | ||
smartProps.onFocus = () => { | ||
smartProps.onFocus = element.props.onFocus && element.props.onFocus(); | ||
this._focusNode(ref) | ||
}; | ||
if (smartScrollOptions.moveToNext === true) { | ||
const nextRef = 'input_' + (inputIndex+1); | ||
const focusNextField = () => this._focusField(nextRef) | ||
smartProps.blurOnSubmit = false; | ||
smartProps.onSubmitEditing = smartScrollOptions.onSubmitEditing ? | ||
() => smartScrollOptions.onSubmitEditing(focusNextField) : | ||
focusNextField | ||
} | ||
} | ||
inputIndex += 1 | ||
} else if (smartScrollOptions.type === 'custom') { | ||
const ref = 'input_' + inputIndex; | ||
smartProps.ref = this._refCreator(ref); | ||
inputIndex += 1 | ||
} | ||
@@ -249,3 +256,3 @@ | ||
SmartScrollView.propTypes = { | ||
forceFocusFieldIndex: PropTypes.number, | ||
forceFocusField: PropTypes.oneOf(PropTypes.number, PropTypes.string), | ||
scrollContainerStyle: PropTypes.number, | ||
@@ -256,3 +263,4 @@ contentContainerStyle: PropTypes.number, | ||
contentInset: PropTypes.object, | ||
onScroll: PropTypes.func | ||
onScroll: PropTypes.func, | ||
onRefFocus: PropTypes.func, | ||
}; | ||
@@ -266,3 +274,4 @@ | ||
contentInset: {top: 0, left: 0, bottom: 0, right: 0}, | ||
onScroll: () => {} | ||
onScroll: () => {}, | ||
onRefFocus: () => {} | ||
}; | ||
@@ -269,0 +278,0 @@ |
@@ -31,3 +31,3 @@ import React, { | ||
contentContainerStyle = { styles.contentContainerStyle } | ||
forceFocusFieldIndex = { this.state.focusFieldIndex } | ||
forceFocusField = { this.state.focusField } | ||
scrollPadding = { 10 } | ||
@@ -45,3 +45,3 @@ > | ||
style = {styles.button} | ||
onPress = {() => {this.setState({focusFieldIndex: 10})}} | ||
onPress = {() => {this.setState({focusField: 10})}} | ||
> | ||
@@ -48,0 +48,0 @@ <Text style = {styles.buttonText}> |
@@ -10,4 +10,4 @@ { | ||
"react-native": "^0.17.0", | ||
"react-native-smart-scroll-view": "^1.3.0" | ||
"react-native-smart-scroll-view": "^1.3.1" | ||
} | ||
} |
2123462
43
788
94