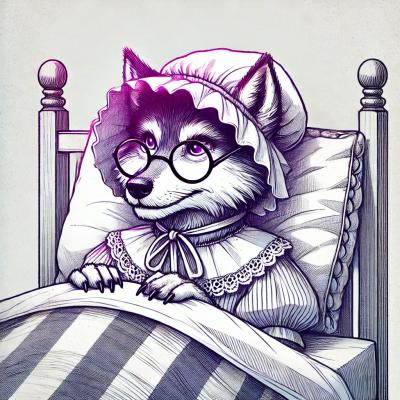
Security News
GitHub Removes Malicious Pull Requests Targeting Open Source Repositories
GitHub removed 27 malicious pull requests attempting to inject harmful code across multiple open source repositories, in another round of low-effort attacks.
react-native-tapjoy
Advanced tools
A React Native module that allows you to use the native Tapjoy advertiser SDK.
This module provides react-native bindings for the Tapjoy SDK.
Tapjoy is a monetization and distribution services provider for mobile applications. Tapjoy’s goal is to maximize the value of every user for freemium mobile app publishers. Its Marketing Automation and Monetization Platform for mobile apps uses market leading data science, user segmentation and predictive analytics to drive deeper engagement and optimize revenue from every user.
React Native lets you build mobile apps using only JavaScript. It uses the same design as React, letting you compose a rich mobile UI from declarative components. You don't build a “mobile web app”, an “HTML5 app”, or a “hybrid app”. You build a real mobile app that's indistinguishable from an app built using Objective-C or Java.
npm install react-native-tapjoy --save
or:
yarn add react-native-tapjoy
react-native link
Add Files to <...>
node_modules
➜ react-native-tapjoy
➜ ios
➜ select RNTapjoy.xcodeproj
libRNTapjoy.a
to Build Phases -> Link Binary With Libraries
Alertnatively, you may use the podspec file:
...
pod 'RNTapjoy', :path => '../node_modules/react-native-tapjoy'
...
NOTE: You have to manually integrate the Push notifications feature as described on the Tapjoy website, because it requires access to the didFinishLaunchingWithOptions:` method in your application’s app delegate file.
Add the following lines to android/settings.gradle
:
include ':react-native-tapjoy'
project(':react-native-tapjoy').projectDir = new File(rootProject.projectDir, '../node_modules/react-native-tapjoy/android')
Update the android build tools version to 3.4.1
in android/build.gradle
:
buildscript {
...
dependencies {
classpath 'com.android.tools.build:gradle:3.4.1'
}
...
}
...
Update the gradle version to 5.4.1
in android/gradle/wrapper/gradle-wrapper.properties
:
...
distributionUrl=https\://services.gradle.org/distributions/gradle-5.4.1-all.zip
Add the compile line to the dependencies in android/app/build.gradle
:
dependencies {
compile project(':react-native-tapjoy')
}
Add the import and link the package in MainApplication.java
:
import com.mariusreimer.tapjoy.TapjoyPackage; // <-- add this import
public class MainApplication extends Application implements ReactApplication {
@Override
protected List<ReactPackage> getPackages() {
return Arrays.<ReactPackage>asList(
new MainReactPackage(),
new TapjoyPackage() // <-- add this line
);
}
}
Add the Tapjoy SDK to your Android project as described on the Tapjoy website.
TypeScript definitions are included.
const options = {
sdkKeyIos: "12345",
sdkKeyAndroid: "12345",
gcmSenderIdAndroid: "12345",
debug: true
};
import { Tapjoy } from 'react-native-tapjoy';
const tapjoy = new Tapjoy(options);
try {
const initialized = await tapjoy.initialise();
// tapjoy is initialized
} catch (e) {
// error on initialization
}
import { useEffect } from 'react';
import { useTapjoy } from 'react-native-tapjoy';
const [
{
tapjoyEvents
},
{
initialiseTapjoy,
listenToEvent,
addTapjoyPlacement,
showTapjoyPlacement,
requestTapjoyPlacementContent,
isTapjoyConnected,
tapjoyListenForEarnedCurrency,
getTapjoyCurrencyBalance,
setTapjoyUserId,
spendTapjoyCurrency,
},
] = useTapjoy(tapjoyOptions);
useEffect(() => {
try {
const initialized = await initialiseTapjoy();
// tapjoy is initialized
} catch (e) {
// error on initialization
}
}, []);
This is a list of events you may subscribe to:
Event Name | Description |
---|---|
earnedCurrency | Fired when currency was earned. The response contains the fields amount currencyName and currencyID (iOS only). |
onPlacementDismiss | Fired when a shown placement was dismissed. The response contains the field placementName . |
onPlacementContentReady | Fired when a placement's content is ready to be shown. The response contains the field placementName . |
onPurchaseRequest | Fired when a purchase is requested. The response contains the fields placementName , requestId , token and productId . |
onRewardRequest | Fired when a reward is requested. The response contains the fields placementName , requestId , token , itemId and quantity . |
tapjoy._on(eventName, () => {
// event has fired
})
You may get a list of events from the constants: tapjoy.constants
. It contains a events
field.
listenToEvent(eventName, () => {
// event has fired
})
You may get a list of events from useTapjoy
hook, named tapjoyEvents
.
try {
await tapjoy.addPlacement();
// tapjoy placement was added
} catch (e) {
// error
}
useEffect(() => {
try {
await addTapjoyPlacement('TestPlacement');
// tapjoy placement was added
} catch (e) {
// error
}
}, []);
try {
await tapjoy.requestContent('TestPlacement');
// tapjoy placement content request was successful
} catch (e) {
// request failed
}
useEffect(() => {
try {
await requestTapjoyPlacementContent('TestPlacement');
// tapjoy placement content request was successful
} catch (e) {
// request failed
}
}, []);
try {
await tapjoy.showPlacement('TestPlacement');
// tapjoy placement content is showing
} catch (e) {
// placement not added, or content not ready
}
useEffect(() => {
try {
await showTapjoyPlacement('TestPlacement');
// tapjoy placement content is showing
} catch (e) {
// placement not added, or content not ready
}
}, []);
try {
await tapjoy.listenForEarnedCurrency(({ amount, currencyName, currencyID }) => {
// user earned currency
});
} catch (e) {
// error
}
useEffect(() => {
try {
await tapjoyListenForEarnedCurrency(({ amount, currencyName, currencyID }) => {
// user earned currency
});
} catch (e) {
// error
}
}, []);
try {
const { amount, currencyName } = await tapjoy.getCurrencyBalance();
} catch (e) {
// error
}
useEffect(() => {
try {
const { amount, currencyName } = await getTapjoyCurrencyBalance();
} catch (e) {
// error
}
}, []);
try {
await tapjoy.spendCurrencyAction(42);
} catch (e) {
// error
}
useEffect(() => {
try {
await spendTapjoyCurrency(42);
} catch (e) {
// error
}
}, []);
FAQs
A React Native module that allows you to use the native Tapjoy advertiser SDK.
The npm package react-native-tapjoy receives a total of 17 weekly downloads. As such, react-native-tapjoy popularity was classified as not popular.
We found that react-native-tapjoy demonstrated a not healthy version release cadence and project activity because the last version was released a year ago. It has 1 open source maintainer collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
GitHub removed 27 malicious pull requests attempting to inject harmful code across multiple open source repositories, in another round of low-effort attacks.
Security News
RubyGems.org has added a new "maintainer" role that allows for publishing new versions of gems. This new permission type is aimed at improving security for gem owners and the service overall.
Security News
Node.js will be enforcing stricter semver-major PR policies a month before major releases to enhance stability and ensure reliable release candidates.