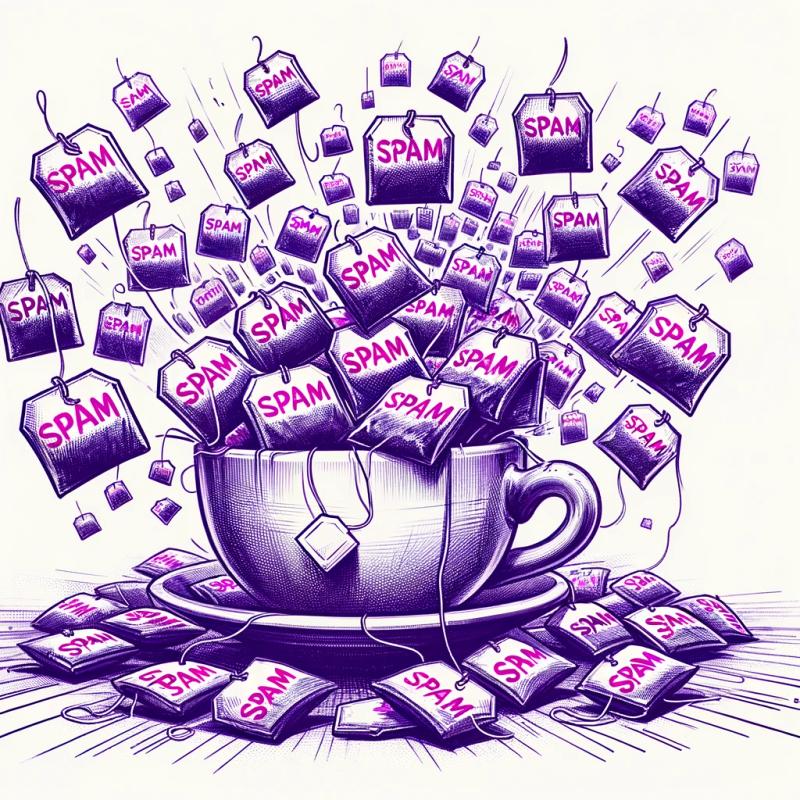
Security News
tea.xyz Spam Plagues npm and RubyGems Package Registries
Tea.xyz, a crypto project aimed at rewarding open source contributions, is once again facing backlash due to an influx of spam packages flooding public package registries.
react-native-vpn-testing-only
Advanced tools
Readme
$ npm install react-native-wireguard --save
$ react-native link react-native-wireguard
Doesn't work on iOS yet. SOON...
AndroidManifest.xml
:
<application>
....
<service
android:name="com.wirevpn.rnwireguard.WGVpnService"
android:permission="android.permission.BIND_VPN_SERVICE">
<intent-filter>
<action android:name="android.net.VpnService" />
</intent-filter>
</service>
....
</application>
res/drawable
or to its multiple hidpi counterparts.applicationId
to the top level build.gradle
to have it accessible.
buildscript {
....
ext {
minSdkVersion = 21
targetSdkVersion = 26
compileSdkVersion = 28
....
applicationId = "com.wirevpn.android"
}
....
}
import WireGuard from 'react-native-wireguard';
// Gets the version of the underying wireguard-go
WireGuard.Version().then((v) => this.setState{version: v});
// Config is of type wg-quick
// MTU is optional and defaults to 1420
// If endpoint resolves to multiple IP addresses,
// IPv4 is preferred over IPv6
var config = `
[Interface]
PrivateKey = mBEJJwnMh6Ht9xLp88nTtHqmOY9pnN7YdriotquvgVI=
Address = 192.168.7.237/32, fdaa::7f3/128
DNS = 192.168.0.0, fdaa::
[Peer]
PublicKey = Cf0rdfToO5gxg7ObB6dLbTwfElO3Xx7Fh8jJobmqCnE=
AllowedIPs = 0.0.0.0/0, ::/0
Endpoint = 209.97.177.222:51820`;
// A name for your session
var session = 'MyVPNSession';
// After a successfull connection, application is brought to
// foreground and needs a notification
var notif = {
icon: 'ic_notif_icon', // Name of the icon in /res directory
title: 'My VPN',
text: 'Connected to ' + country;
}
// Starts the VPN connection
// After successfull connection you will receive an event
WireGuard.Connect(config, session, notif).catch(
(e) => console.warn(e.message));
// Check if VPN service is online
WireGuard.Status().then((b) => {
if(b){
// Update state
}
});
// Terminates the connection
// After successfull termination of the connection you will
// receive an event
WireGuard.Disconnect()
componentDidMount() {
DeviceEventEmitter.addListener(WireGuard.EV_TYPE_SYSTEM, () => {
if(e === WireGuard.EV_STARTED_BY_SYSTEM) {
// This event is emitted when VPN service is started
// by the system. For example if a user enables Always-On
// in settings, system will try to bring the VPN online but
// since it doesn't have any config it will fail and send
// this event instead so that you can start it correctly
// here...
}
});
// If any exceptions occur after calling the Connect()
// method you can catch them here. e is of type string
DeviceEventEmitter.addListener(WireGuard.EV_TYPE_EXCEPTION, () => {
console.log(e);
});
DeviceEventEmitter.addListener(WireGuard.EV_TYPE_REGULAR, () => {
if(e === WireGuard.EV_STOPPED) {
// Update state
} else if(e === WireGuard.EV_STARTED) {
// Update state
}
});
}
componentWillUnmount() {
// You will receive the same event multiple times
// if this not set
DeviceEventEmitter.removeAllListeners();
}
FAQs
WireGuard bridge for React Native
The npm package react-native-vpn-testing-only receives a total of 1 weekly downloads. As such, react-native-vpn-testing-only popularity was classified as not popular.
We found that react-native-vpn-testing-only demonstrated a not healthy version release cadence and project activity because the last version was released a year ago. It has 1 open source maintainer collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
Tea.xyz, a crypto project aimed at rewarding open source contributions, is once again facing backlash due to an influx of spam packages flooding public package registries.
Security News
As cyber threats become more autonomous, AI-powered defenses are crucial for businesses to stay ahead of attackers who can exploit software vulnerabilities at scale.
Security News
UnitedHealth Group disclosed that the ransomware attack on Change Healthcare compromised protected health information for millions in the U.S., with estimated costs to the company expected to reach $1 billion.