React Object Input

A React component which facilitates user-friendly editing of plain javascript objects. The result acts as a controlled component, suitable for use in a React form - no internal state to get out of sync. The UI maintains consistent order of items, despite object
giving no order guarantees.
Check out a demo
In Typescript terms, given a Record<string, T>
, where T is some arbitrary type, and a React component which can edit a single instance of T, ObjectInput
provides the scaffolding for a property-list-style editor.
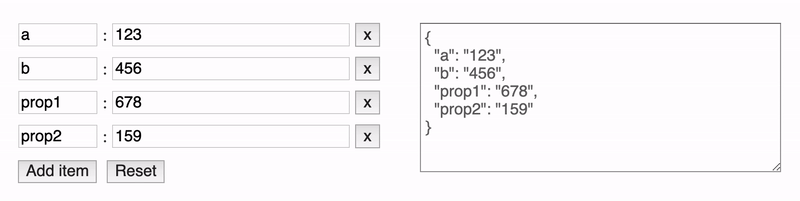
Example implementation
export const MyComponent = () => {
const [value, setValue] = useState<Record<string, string>>({
a: '123',
b: '456'
})
return (
<ObjectInput
obj={value}
onChange={setValue}
renderItem={(key, value, updateKey, updateValue, deleteProperty) => (
// render an editor row for an item, using the provided callbacks
// to propagate changes
<div>
<input
type="text"
value={key}
onChange={e => updateKey(e.target.value)}
/>
:
<input
type="text"
value={value || ''} // value will be undefined for new rows
onChange={e => updateValue(e.target.value)}
/>
<button onClick={deleteProperty}>x</button>
</div>
)}
/>
)
}
Development
To build the library and watch for changes
npm i # or yarn
npm start # or yarn start
With start
running as above, run the example:
cd example
npm i # or yarn to install dependencies
npm start # or yarn start
Tests
npm test # or yarn test