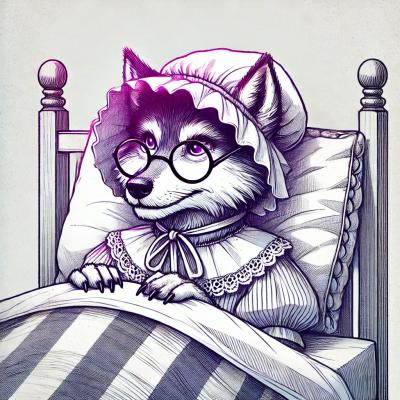
Security News
GitHub Removes Malicious Pull Requests Targeting Open Source Repositories
GitHub removed 27 malicious pull requests attempting to inject harmful code across multiple open source repositories, in another round of low-effort attacks.
react-oidc-context
Advanced tools
OpenID Connect & OAuth2 authentication using react context api as state management
The react-oidc-context package is a React library that provides context and hooks for handling OpenID Connect (OIDC) authentication. It simplifies the process of integrating OIDC authentication into React applications by managing the authentication state, tokens, and user information.
Authentication Provider
The AuthProvider component is used to wrap your application and provide the OIDC context. It takes configuration options such as authority, client_id, redirect_uri, response_type, and scope.
import { AuthProvider } from 'react-oidc-context';
const oidcConfig = {
authority: 'https://example.com',
client_id: 'your-client-id',
redirect_uri: 'http://localhost:3000/callback',
response_type: 'code',
scope: 'openid profile email'
};
function App() {
return (
<AuthProvider {...oidcConfig}>
<YourAppComponents />
</AuthProvider>
);
}
Using Authentication Hooks
The useAuth hook provides access to the authentication state and methods such as login and logout. It also provides the authenticated user's information.
import { useAuth } from 'react-oidc-context';
function UserProfile() {
const { user, isAuthenticated, login, logout } = useAuth();
if (!isAuthenticated) {
return <button onClick={login}>Login</button>;
}
return (
<div>
<h1>Welcome, {user.profile.name}</h1>
<button onClick={logout}>Logout</button>
</div>
);
}
Handling Authentication Callback
The AuthCallback component handles the redirect URI after the user has authenticated. It processes the authentication response and updates the authentication state.
import { AuthProvider, AuthCallback } from 'react-oidc-context';
function App() {
return (
<AuthProvider {...oidcConfig}>
<Route path='/callback' component={AuthCallback} />
<YourAppComponents />
</AuthProvider>
);
}
The oidc-client package is a JavaScript library for managing OpenID Connect (OIDC) authentication. It provides a more low-level API compared to react-oidc-context, which means you have to handle more of the integration details yourself. It is suitable for applications that need more control over the authentication process.
The react-aad-msal package is a React wrapper for the Microsoft Authentication Library (MSAL). It is specifically designed for integrating Azure Active Directory (AAD) authentication into React applications. It provides similar functionality to react-oidc-context but is tailored for use with Microsoft identity platforms.
The react-oauth2-hook package provides hooks for OAuth2 authentication in React applications. It is more generic compared to react-oidc-context and can be used with various OAuth2 providers. It does not provide as much built-in support for OIDC-specific features.
Lightweight auth library using the oidc-client-ts library for React single page applications (SPA). Support for hooks and higher-order components (HOC).
This library implements an auth context provider by making use of the
oidc-client-ts
library. Its configuration is tight coupled to that library.
The
User
and
UserManager
is hold in this context, which is accessible from the
React application. Additionally it intercepts the auth redirects by looking at
the query/fragment parameters and acts accordingly. You still need to setup a
redirect uri, which must point to your application, but you do not need to
create that route.
To renew the access token, the
automatic silent renew
feature of oidc-client-ts
can be used.
Using npm
npm install oidc-client-ts react-oidc-context --save
Using yarn
yarn add oidc-client-ts react-oidc-context
Configure the library by wrapping your application in AuthProvider
:
// src/index.jsx
import React from "react";
import ReactDOM from "react-dom";
import { AuthProvider } from "react-oidc-context";
import App from "./App";
const oidcConfig = {
authority: "<your authority>",
client_id: "<your client id>",
redirect_uri: "<your redirect uri>",
// ...
};
ReactDOM.render(
<AuthProvider {...oidcConfig}>
<App />
</AuthProvider>,
document.getElementById("app")
);
Use the useAuth
hook in your components to access authentication state
(isLoading
, isAuthenticated
and user
) and authentication methods
(signinRedirect
, removeUser
and signOutRedirect
):
// src/App.jsx
import React from "react";
import { useAuth } from "react-oidc-context";
function App() {
const auth = useAuth();
switch (auth.activeNavigator) {
case "signinSilent":
return <div>Signing you in...</div>;
case "signoutRedirect":
return <div>Signing you out...</div>;
}
if (auth.isLoading) {
return <div>Loading...</div>;
}
if (auth.error) {
return <div>Oops... {auth.error.message}</div>;
}
if (auth.isAuthenticated) {
return (
<div>
Hello {auth.user?.profile.sub}{" "}
<button onClick={() => void auth.removeUser()}>Log out</button>
</div>
);
}
return <button onClick={() => void auth.signinRedirect()}>Log in</button>;
}
export default App;
You must provide an implementation of onSigninCallback
to oidcConfig
to remove the payload from the URL upon successful login. Otherwise if you refresh the page and the payload is still there, signinSilent
- which handles renewing your token - won't work.
A working implementation is already in the code here.
Use the withAuth
higher-order component to add the auth
property to class
components:
// src/Profile.jsx
import React from "react";
import { withAuth } from "react-oidc-context";
class Profile extends React.Component {
render() {
// `this.props.auth` has all the same properties as the `useAuth` hook
const auth = this.props.auth;
return <div>Hello {auth.user?.profile.sub}</div>;
}
}
export default withAuth(Profile);
As a child of AuthProvider
with a user containing an access token:
// src/Posts.jsx
import React from "react";
import { useAuth } from "react-oidc-context";
const Posts = () => {
const auth = useAuth();
const [posts, setPosts] = React.useState(Array);
React.useEffect(() => {
(async () => {
try {
const token = auth.user?.access_token;
const response = await fetch("https://api.example.com/posts", {
headers: {
Authorization: `Bearer ${token}`,
},
});
setPosts(await response.json());
} catch (e) {
console.error(e);
}
})();
}, [auth]);
if (!posts.length) {
return <div>Loading...</div>;
}
return (
<ul>
{posts.map((post, index) => {
return <li key={index}>{post}</li>;
})}
</ul>
);
};
export default Posts;
As not a child of AuthProvider
(e.g. redux slice) when using local storage
(WebStorageStateStore
) for the user containing an access token:
// src/slice.js
import { User } from "oidc-client-ts"
function getUser() {
const oidcStorage = localStorage.getItem(`oidc.user:<your authority>:<your client id>`)
if (!oidcStorage) {
return null;
}
return User.fromStorageString(oidcStorage);
}
export const getPosts = createAsyncThunk(
"store/getPosts",
async () => {
const user = getUser();
const token = user?.access_token;
return fetch("https://api.example.com/posts", {
headers: {
Authorization: `Bearer ${token}`,
},
});
},
// ...
)
Secure a route component by using the withAuthenticationRequired
higher-order component. If a user attempts
to access this route without authentication, they will be redirected to the login page.
import React from 'react';
import { withAuthenticationRequired } from "react-oidc-context";
const PrivateRoute = () => (<div>Private</div>);
export default withAuthenticationRequired(PrivateRoute, {
onRedirecting: () => (<div>Redirecting to the login page...</div>)
});
The underlying UserManagerEvents
instance can be imperatively managed with the useAuth
hook.
// src/App.jsx
import React from "react";
import { useAuth } from "react-oidc-context";
function App() {
const auth = useAuth();
React.useEffect(() => {
// the `return` is important - addAccessTokenExpiring() returns a cleanup function
return auth.events.addAccessTokenExpiring(() => {
if (alert("You're about to be signed out due to inactivity. Press continue to stay signed in.")) {
auth.signinSilent();
}
})
}, [auth.events, auth.signinSilent]);
return <button onClick={() => void auth.signinRedirect()}>Log in</button>;
}
export default App;
Automatically sign-in and silently reestablish your previous session, if you close the tab and reopen the application.
// index.jsx
const oidcConfig: AuthProviderProps = {
...
userStore: new WebStorageStateStore({ store: window.localStorage }),
};
// src/App.jsx
import React from "react";
import { useAuth, hasAuthParams } from "react-oidc-context";
function App() {
const auth = useAuth();
const [hasTriedSignin, setHasTriedSignin] = React.useState(false);
// automatically sign-in
React.useEffect(() => {
if (!hasAuthParams() &&
!auth.isAuthenticated && !auth.activeNavigator && !auth.isLoading &&
!hasTriedSignin
) {
auth.signinRedirect();
setHasTriedSignin(true);
}
}, [auth, hasTriedSignin]);
if (auth.isLoading) {
return <div>Signing you in/out...</div>;
}
if (!auth.isAuthenticated) {
return <div>Unable to log in</div>;
}
return <button onClick={() => void auth.removeUser()}>Log out</button>;
}
export default App;
We appreciate feedback and contribution to this repo!
This library is inspired by oidc-react, which lacks error handling and auth0-react, which is focused on auth0.
This project is licensed under the MIT license. See the LICENSE file for more info.
FAQs
OpenID Connect & OAuth2 authentication using react context api as state management
The npm package react-oidc-context receives a total of 23,550 weekly downloads. As such, react-oidc-context popularity was classified as popular.
We found that react-oidc-context demonstrated a healthy version release cadence and project activity because the last version was released less than a year ago. It has 2 open source maintainers collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
GitHub removed 27 malicious pull requests attempting to inject harmful code across multiple open source repositories, in another round of low-effort attacks.
Security News
RubyGems.org has added a new "maintainer" role that allows for publishing new versions of gems. This new permission type is aimed at improving security for gem owners and the service overall.
Security News
Node.js will be enforcing stricter semver-major PR policies a month before major releases to enhance stability and ensure reliable release candidates.