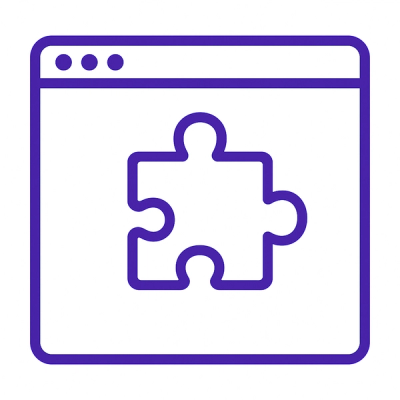
Research
Security News
The Growing Risk of Malicious Browser Extensions
Socket researchers uncover how browser extensions in trusted stores are used to hijack sessions, redirect traffic, and manipulate user behavior.
react-outside-click-handler
Advanced tools
A React component for dealing with clicks outside its subtree
The react-outside-click-handler package is a React component that helps you detect and handle clicks outside of a specified element. This is particularly useful for implementing features like closing dropdowns, modals, or tooltips when a user clicks outside of them.
Basic Outside Click Handling
This feature allows you to detect clicks outside of a specified element. The `onOutsideClick` prop is used to define the function that will be called when an outside click is detected.
import React from 'react';
import OutsideClickHandler from 'react-outside-click-handler';
function MyComponent() {
const handleOutsideClick = () => {
console.log('Clicked outside!');
};
return (
<OutsideClickHandler onOutsideClick={handleOutsideClick}>
<div>
Click outside of this div to trigger the handler.
</div>
</OutsideClickHandler>
);
}
export default MyComponent;
Nested Outside Click Handling
This feature demonstrates handling nested outside click events. You can have multiple `OutsideClickHandler` components nested within each other, each with its own `onOutsideClick` handler.
import React from 'react';
import OutsideClickHandler from 'react-outside-click-handler';
function MyComponent() {
const handleOutsideClick = () => {
console.log('Clicked outside the outer div!');
};
const handleInnerOutsideClick = () => {
console.log('Clicked outside the inner div!');
};
return (
<OutsideClickHandler onOutsideClick={handleOutsideClick}>
<div>
Outer Div
<OutsideClickHandler onOutsideClick={handleInnerOutsideClick}>
<div>
Inner Div
</div>
</OutsideClickHandler>
</div>
</OutsideClickHandler>
);
}
export default MyComponent;
The react-onclickoutside package provides a higher-order component (HOC) that can be used to wrap any component and detect clicks outside of it. It is similar to react-outside-click-handler but uses a different approach by wrapping the component with an HOC.
The react-click-outside package is another alternative that provides a higher-order component for handling outside clicks. It is similar in functionality to react-outside-click-handler but offers a different API and implementation.
The use-onclickoutside package is a React hook that allows you to detect clicks outside of a specified element. It is a more modern approach compared to react-outside-click-handler, leveraging React hooks for a more functional programming style.
A React component for handling outside clicks
import OutsideClickHandler from 'react-outside-click-handler';
function MyComponent() {
return (
<OutsideClickHandler
onOutsideClick={() => {
alert('You clicked outside of this component!!!');
}}
>
Hello World
</OutsideClickHandler>
);
}
PropTypes.node.isRequired
Since the OutsideClickHandler
specifically handles clicks outside a specific subtree, children
is expected to be defined. A consumer should also not render the OutsideClickHandler
in the case that children
are not defined.
Note that if you use a Portal
(native or react-portal
) of any sort in the children
, the OutsideClickHandler
will not behave as expected.
PropTypes.func.isRequired
The onOutsideClick
prop is also required as without it, the OutsideClickHandler
is basically a heavy-weight <div />
. It takes the relevant clickevent as an arg and gets triggered when the user clicks anywhere outside of the subtree generated by the DOM node.
PropTypes.bool
If the disabled
prop is true, outside clicks will not be registered. This can be utilized to temporarily disable interaction without unmounting/remounting the entire tree.
PropTypes.bool
See https://developer.mozilla.org/en-US/docs/Learn/JavaScript/Building_blocks/Events#Event_bubbling_and_capture for more information on event bubbling vs. capture.
If useCapture
is true, the event will be registered in the capturing phase and thus, propagated top-down instead of bottom-up as is the default.
PropTypes.oneOf(['block', 'flex', 'inline-block', 'inline', 'contents'])
By default, the OutsideClickHandler
renders a display: block
<div />
to wrap the subtree defined by children
. If desired, the display
can be set to inline-block
, inline
, flex
, or contents
instead. There is no way not to render a wrapping <div />
.
v1.3.0
display
: add contents
(#34)display
: add inline
(#28)componentWillReceiveProps
with componentDidUpdate
for a side effect (#36)airbnb-prop-types
enzyme-adapter-react-helper
, eslint
, eslint-config-airbnb
, eslint-plugin-import
, eslint-plugin-react
, eslint-plugin-react-with-styles
, rimraf
, safe-publish-latest
, sinon-sandbox
; add eslint-plugin-react-hooks
FAQs
A React component for dealing with clicks outside its subtree
The npm package react-outside-click-handler receives a total of 0 weekly downloads. As such, react-outside-click-handler popularity was classified as not popular.
We found that react-outside-click-handler demonstrated a not healthy version release cadence and project activity because the last version was released a year ago. It has 6 open source maintainers collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Research
Security News
Socket researchers uncover how browser extensions in trusted stores are used to hijack sessions, redirect traffic, and manipulate user behavior.
Research
Security News
An in-depth analysis of credential stealers, crypto drainers, cryptojackers, and clipboard hijackers abusing open source package registries to compromise Web3 development environments.
Security News
pnpm 10.12.1 introduces a global virtual store for faster installs and new options for managing dependencies with version catalogs.