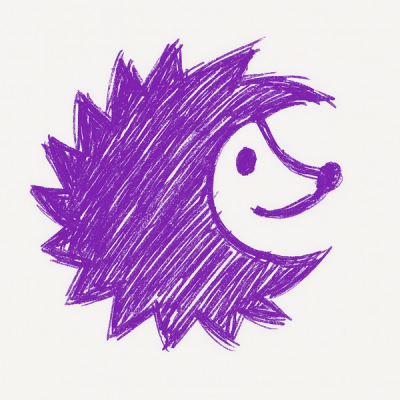
Security News
Browserslist-rs Gets Major Refactor, Cutting Binary Size by Over 1MB
Browserslist-rs now uses static data to reduce binary size by over 1MB, improving memory use and performance for Rust-based frontend tools.
react-particles
Advanced tools
Official tsParticles React Component - Easily create highly customizable particle, confetti and fireworks animations and use them as animated backgrounds for your website. Ready to use components available also for Web Components, Vue.js (2.x and 3.x), An
Official tsParticles ReactJS component
npm install react-particles
or
yarn add react-particles
Starting from version 1.17.0 there are two official create-react-app
templates:
cra-template-particles
: Simple ReactJS template with full screen particles, using JavaScriptcra-template-particles-typescript
: Simple ReactJS template with full screen particles, using TypeScriptYou can simply install them using the create-react-app
command like this:
$ create-react-app your_app --template particles
or
$ create-react-app your_app --template particles-typescript
Examples:
import { useCallback } from "react";
import Particles from "react-particles";
//import { loadFull } from "tsparticles"; // if you are going to use `loadFull`, install the "tsparticles" package too.
import { loadSlim } from "tsparticles-slim"; // if you are going to use `loadSlim`, install the "tsparticles-slim" package too.
const App = () => {
const particlesInit = useCallback(async engine => {
console.log(engine);
// you can initiate the tsParticles instance (engine) here, adding custom shapes or presets
// this loads the tsparticles package bundle, it's the easiest method for getting everything ready
// starting from v2 you can add only the features you need reducing the bundle size
//await loadFull(engine);
await loadSlim(engine);
}, []);
const particlesLoaded = useCallback(async container => {
await console.log(container);
}, []);
return (
<Particles id="tsparticles" url="http://foo.bar/particles.json" init={particlesInit} loaded={particlesLoaded} />
);
};
import { useCallback } from "react";
import Particles from "react-particles";
import type { Container, Engine } from "tsparticles-engine";
//import { loadFull } from "tsparticles"; // if you are going to use `loadFull`, install the "tsparticles" package too.
import { loadSlim } from "tsparticles-slim"; // if you are going to use `loadSlim`, install the "tsparticles-slim" package too.
const App = () => {
const particlesInit = useCallback(async (engine: Engine) => {
console.log(engine);
// you can initialize the tsParticles instance (engine) here, adding custom shapes or presets
// this loads the tsparticles package bundle, it's the easiest method for getting everything ready
// starting from v2 you can add only the features you need reducing the bundle size
//await loadFull(engine);
await loadSlim(engine);
}, []);
const particlesLoaded = useCallback(async (container: Container | undefined) => {
await console.log(container);
}, []);
return (
<Particles id="tsparticles" url="http://foo.bar/particles.json" init={particlesInit} loaded={particlesLoaded} />
);
};
import { useCallback } from "react";
import Particles from "react-particles";
//import { loadFull } from "tsparticles"; // if you are going to use `loadFull`, install the "tsparticles" package too.
import { loadSlim } from "tsparticles-slim"; // if you are going to use `loadSlim`, install the "tsparticles-slim" package too.
const App = () => {
const particlesInit = useCallback(async engine => {
console.log(engine);
// you can initiate the tsParticles instance (engine) here, adding custom shapes or presets
// this loads the tsparticles package bundle, it's the easiest method for getting everything ready
// starting from v2 you can add only the features you need reducing the bundle size
//await loadFull(engine);
await loadSlim(engine);
}, []);
const particlesLoaded = useCallback(async container => {
await console.log(container);
}, []);
return (
<Particles
id="tsparticles"
init={particlesInit}
loaded={particlesLoaded}
options={{
background: {
color: {
value: "#0d47a1",
},
},
fpsLimit: 120,
interactivity: {
events: {
onClick: {
enable: true,
mode: "push",
},
onHover: {
enable: true,
mode: "repulse",
},
resize: true,
},
modes: {
push: {
quantity: 4,
},
repulse: {
distance: 200,
duration: 0.4,
},
},
},
particles: {
color: {
value: "#ffffff",
},
links: {
color: "#ffffff",
distance: 150,
enable: true,
opacity: 0.5,
width: 1,
},
move: {
direction: "none",
enable: true,
outModes: {
default: "bounce",
},
random: false,
speed: 6,
straight: false,
},
number: {
density: {
enable: true,
area: 800,
},
value: 80,
},
opacity: {
value: 0.5,
},
shape: {
type: "circle",
},
size: {
value: { min: 1, max: 5 },
},
},
detectRetina: true,
}}
/>
);
};
import { useCallback } from "react";
import type { Container, Engine } from "tsparticles-engine";
import Particles from "react-particles";
//import { loadFull } from "tsparticles"; // if you are going to use `loadFull`, install the "tsparticles" package too.
import { loadSlim } from "tsparticles-slim"; // if you are going to use `loadSlim`, install the "tsparticles-slim" package too.
const App = () => {
const particlesInit = useCallback(async (engine: Engine) => {
console.log(engine);
// you can initialize the tsParticles instance (engine) here, adding custom shapes or presets
// this loads the tsparticles package bundle, it's the easiest method for getting everything ready
// starting from v2 you can add only the features you need reducing the bundle size
//await loadFull(engine);
await loadSlim(engine);
}, []);
const particlesLoaded = useCallback(async (container: Container | undefined) => {
await console.log(container);
}, []);
return (
<Particles
id="tsparticles"
init={particlesInit}
loaded={particlesLoaded}
options={{
background: {
color: {
value: "#0d47a1",
},
},
fpsLimit: 120,
interactivity: {
events: {
onClick: {
enable: true,
mode: "push",
},
onHover: {
enable: true,
mode: "repulse",
},
resize: true,
},
modes: {
push: {
quantity: 4,
},
repulse: {
distance: 200,
duration: 0.4,
},
},
},
particles: {
color: {
value: "#ffffff",
},
links: {
color: "#ffffff",
distance: 150,
enable: true,
opacity: 0.5,
width: 1,
},
move: {
direction: "none",
enable: true,
outModes: {
default: "bounce",
},
random: false,
speed: 6,
straight: false,
},
number: {
density: {
enable: true,
area: 800,
},
value: 80,
},
opacity: {
value: 0.5,
},
shape: {
type: "circle",
},
size: {
value: { min: 1, max: 5 },
},
},
detectRetina: true,
}}
/>
);
};
Prop | Type | Definition |
---|---|---|
id | string | The id of the element. |
width | string | The width of the canvas. |
height | string | The height of the canvas. |
options | object | The options of the particles instance. |
url | string | The remote options url, called using an AJAX request |
style | object | The style of the canvas element. |
className | string | The class name of the canvas wrapper. |
canvasClassName | string | the class name of the canvas. |
container | object | The instance of the particles container |
init | function | This function is called after the tsParticles instance initialization, the instance is the parameter and you can load custom presets or shapes here |
loaded | function | This function is called when particles are correctly loaded in canvas, the current container is the parameter and you can customize it here |
Find all configuration options here.
You can find sample configurations here 📖
Preset demos can be found here
There's also a CodePen collection actively maintained and updated here
Report bugs and issues here
FAQs
Official tsParticles React Component - Easily create highly customizable particle, confetti and fireworks animations and use them as animated backgrounds for your website. Ready to use components available also for Web Components, Vue.js (2.x and 3.x), An
The npm package react-particles receives a total of 12,402 weekly downloads. As such, react-particles popularity was classified as popular.
We found that react-particles demonstrated a not healthy version release cadence and project activity because the last version was released a year ago. It has 2 open source maintainers collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
Browserslist-rs now uses static data to reduce binary size by over 1MB, improving memory use and performance for Rust-based frontend tools.
Research
Security News
Eight new malicious Firefox extensions impersonate games, steal OAuth tokens, hijack sessions, and exploit browser permissions to spy on users.
Security News
The official Go SDK for the Model Context Protocol is in development, with a stable, production-ready release expected by August 2025.