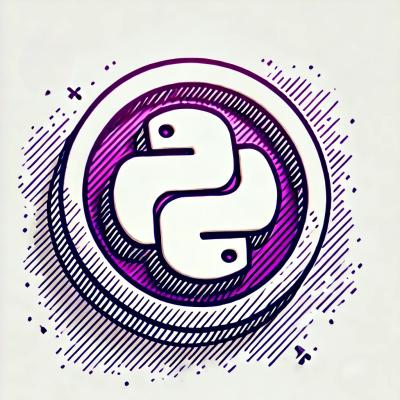
Security News
PyPI Introduces Digital Attestations to Strengthen Python Package Security
PyPI now supports digital attestations, enhancing security and trust by allowing package maintainers to verify the authenticity of Python packages.
react-popper-tooltip
Advanced tools
The react-popper-tooltip package is a React wrapper for Popper.js, which is a library used to position tooltips and popovers in web applications. It provides a simple and flexible way to create tooltips that are dynamically positioned based on the user's viewport and other elements on the page.
Basic Tooltip
This code demonstrates how to create a basic tooltip using the react-popper-tooltip package. The tooltip appears when the user hovers over the button.
import React from 'react';
import { usePopperTooltip } from 'react-popper-tooltip';
import 'react-popper-tooltip/dist/styles.css';
const BasicTooltip = () => {
const { getTooltipProps, setTooltipRef, setTriggerRef, visible } = usePopperTooltip();
return (
<div>
<button ref={setTriggerRef}>Hover me</button>
{visible && (
<div ref={setTooltipRef} {...getTooltipProps({ className: 'tooltip-container' })}>
Tooltip content
</div>
)}
</div>
);
};
export default BasicTooltip;
Custom Tooltip
This code demonstrates how to create a custom tooltip that appears on click and is positioned to the right of the trigger element.
import React from 'react';
import { usePopperTooltip } from 'react-popper-tooltip';
import 'react-popper-tooltip/dist/styles.css';
const CustomTooltip = () => {
const { getTooltipProps, setTooltipRef, setTriggerRef, visible } = usePopperTooltip({
placement: 'right',
trigger: 'click',
});
return (
<div>
<button ref={setTriggerRef}>Click me</button>
{visible && (
<div ref={setTooltipRef} {...getTooltipProps({ className: 'tooltip-container' })}>
Custom Tooltip content
</div>
)}
</div>
);
};
export default CustomTooltip;
Tooltip with Arrow
This code demonstrates how to create a tooltip with an arrow using the react-popper-tooltip package. The tooltip appears when the user hovers over the button and includes an arrow pointing to the trigger element.
import React from 'react';
import { usePopperTooltip } from 'react-popper-tooltip';
import 'react-popper-tooltip/dist/styles.css';
const TooltipWithArrow = () => {
const { getArrowProps, getTooltipProps, setTooltipRef, setTriggerRef, visible } = usePopperTooltip({
arrow: true,
});
return (
<div>
<button ref={setTriggerRef}>Hover me</button>
{visible && (
<div ref={setTooltipRef} {...getTooltipProps({ className: 'tooltip-container' })}>
Tooltip with arrow
<div {...getArrowProps({ className: 'tooltip-arrow' })} />
</div>
)}
</div>
);
};
export default TooltipWithArrow;
react-tooltip is a popular package for creating tooltips in React applications. It offers a wide range of customization options and supports various trigger events. Compared to react-popper-tooltip, react-tooltip is more feature-rich but may have a steeper learning curve.
react-bootstrap is a popular UI library for React that includes a Tooltip component. It is built on top of Bootstrap and provides a set of pre-styled components. Compared to react-popper-tooltip, react-bootstrap is more opinionated and may be easier to use for developers already familiar with Bootstrap.
React tooltip component based on react-popper, the React wrapper around popper.js library.
https://react-popper-tooltip.netlify.app
https://codesandbox.io/s/pykkz77z5j
npm install react-popper-tooltip
or
yarn add react-popper-tooltip
import React from 'react';
import {render} from 'react-dom';
import TooltipTrigger from 'react-popper-tooltip';
const Tooltip = ({
arrowRef,
tooltipRef,
getArrowProps,
getTooltipProps,
placement
}) => (
<div
{...getTooltipProps({
ref: tooltipRef,
className: 'tooltip-container'
/* your props here */
})}
>
<div
{...getArrowProps({
ref: arrowRef,
className: 'tooltip-arrow',
'data-placement': placement
/* your props here */
})}
/>
Hello, World!
</div>
);
const Trigger = ({getTriggerProps, triggerRef}) => (
<span
{...getTriggerProps({
ref: triggerRef,
className: 'trigger'
/* your props here */
})}
>
Click Me!
</span>
);
render(
<TooltipTrigger placement="right" trigger="click" tooltip={Tooltip}>
{Trigger}
</TooltipTrigger>,
document.getElementById('root')
);
TooltipTrigger
is the only component exposed by the package. It's just a positioning engine. What to render is left completely to the user, which can be provided using render props. Your props should be passed through getTriggerProps
, getTooltipProps
and getArrowProps
.
Read more about render prop pattern if you're not familiar with this approach.
To fiddle with our example recipes, run:
> npm install
> npm run docs
or
> yarn
> yarn docs
and open up localhost:3000 in your browser.
function({})
| required
This is called with an object. Read more about the properties of this object in the section "Children and tooltip functions".
function({})
| required
This is called with an object. Read more about the properties of this object in the section "Children and tooltip functions".
boolean
| defaults tofalse
This is the initial visibility state of the tooltip.
function(tooltipShown: boolean)
Called with the tooltip state, when the visibility of the tooltip changes
boolean
| control prop
Use this prop if you want to control the visibility state of the tooltip.
react-popper-tooltip
manages its own state internally. You can use this prop to pass the
visibility state of the tooltip from the outside. You will be required to keep this state up to
date (this is where onVisibilityChange
becomes useful), but you can also control the state
from anywhere, be that state from other components, redux
, react-router
, or anywhere else.
number
| defaults to0
Delay in showing the tooltip (ms).
number
| defaults to0
Delay in hiding the tooltip (ms).
string
| defaults toright
The tooltip placement. Valid placements are:
auto
top
right
bottom
left
Each placement can have a variation from this list:
-start
-end
string
orstring[]
| defaults to"hover"
The event(s) that trigger the tooltip. One of click
, right-click
, hover
, focus
, and none
, or an array of them.
function(HTMLElement) => void
Function that can be used to obtain a trigger element reference.
boolean
| defaults totrue
Whether to close the tooltip when it's trigger is out of the boundary.
boolean
| defaults totrue
Whether to use React.createPortal
for creating tooltip.
HTMLElement
| defaults todocument.body
Element to be used as portal container
boolean
| defaults tofalse
Whether to spawn the tooltip at the cursor position.
Recommended usage with hover trigger and no arrow element
object
Modifiers passed directly to the underlying popper.js instance. For more information, refer to Popper.js’ modifier docs
object
Options to MutationObserver
, used internally for updating tooltip position based on its DOM changes.
For more information, refer to MutationObserver docs.
Default options:
{
childList: true,
subtree: true
}
This is where you render whatever you want. react-popper-tooltip
uses two render props children
and tooltip
. Children
prop is used to trigger the appearance of the tooltip and tooltip
displays the tooltip itself.
You use it like so:
const tooltip = (
<TooltipTrigger tooltip={tooltip => <div>{/* more jsx here */}</div>}>
{trigger => <div>{/* more jsx here */}</div>}
</TooltipTrigger>
);
These functions are used to apply props to the elements that you render.
It's advisable to pass all your props to that function rather than applying them on the element
yourself to avoid your props being overridden (or overriding the props returned). For example
<button {...getTriggerProps({onClick: event => console.log(event))}>Click me</button>
property | type | description |
---|---|---|
triggerRef | function ref | returns the react ref you should apply to the trigger element. |
getTriggerProps | function({}) | returns the props you should apply to the trigger element you render. |
property | type | description |
---|---|---|
arrowRef | function ref | return the react ref you should apply to the tooltip arrow element. |
tooltipRef | function ref | return the react ref you should apply to the tooltip element. |
getArrowProps | function({}) | return the props you should apply to the tooltip arrow element. |
getTooltipProps | function({}) | returns the props you should apply to the tooltip element you render. |
placement | string | return the dynamic placement of the tooltip. |
This library is based on react-popper, the official react wrapper around Popper.js.
Using of render props, prop getters and doc style of this library are heavily inspired by downshift.
FAQs
React tooltip library built around react-popper
The npm package react-popper-tooltip receives a total of 133,749 weekly downloads. As such, react-popper-tooltip popularity was classified as popular.
We found that react-popper-tooltip demonstrated a not healthy version release cadence and project activity because the last version was released a year ago. It has 2 open source maintainers collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
PyPI now supports digital attestations, enhancing security and trust by allowing package maintainers to verify the authenticity of Python packages.
Security News
GitHub removed 27 malicious pull requests attempting to inject harmful code across multiple open source repositories, in another round of low-effort attacks.
Security News
RubyGems.org has added a new "maintainer" role that allows for publishing new versions of gems. This new permission type is aimed at improving security for gem owners and the service overall.