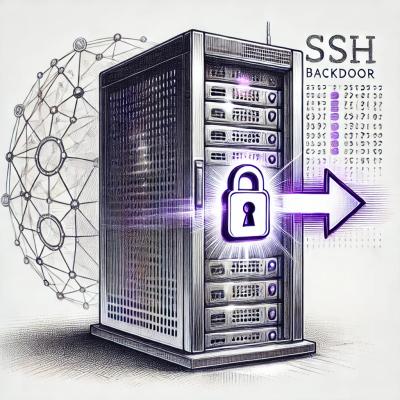
Research
Security News
Malicious npm Packages Inject SSH Backdoors via Typosquatted Libraries
Socket’s threat research team has detected six malicious npm packages typosquatting popular libraries to insert SSH backdoors.
react-reactive-class
Advanced tools
With React Reactive Class, you can create Reactive Components, which subscribe Rx.Observables and re-render themselves.
Reactive DOM elements: A set of reactive DOM elements (button, div, span, etc).
Reactive wrapper: A higher order component to wrap your existing component to be a Reactive Component.
npm install --save react-reactive-class
import React from 'react';
import Rx from 'rx';
import {dom} from 'react-reactive-class';
const { div:Div, span:Span } = dom;
window.style$ = new Rx.Subject();
window.text$ = new Rx.Subject();
class App extends React.Component {
render() {
console.log('App rendered.');
return (
<div>
<h1>Demo</h1>
<Div style={window.style$}>Hello</Div>
<Span>{window.text$}</Span>
</div>
);
}
}
React.render(<App />, document.getElementById('app'));
// notice that App will not re-render, nice!
window.style$.onNext({color: 'blue'});
window.text$.onNext('Reactive!');
// you can open your console and play around
import {reactive} from 'react-reactive-class';
class Text extends React.Component {
render() {
console.log('Text rendered.');
return (
<div>{this.props.children}</div>
);
}
}
const XText = reactive(Text);
import {reactive} from 'react-reactive-class';
@reactive
class XText extends React.Component {
render() {
console.log('Text rendered.');
return (
<div>{this.props.children}</div>
);
}
}
Reactively compose components!
// Unmount this component if length of incoming text is 0.
<Span mount={ text$.map(text => text.length) }>
{text$}
</Span>
Source must be the only child when using observable as child component.
// This will not work
<Span>
Hello {name$}, how are you?
</Span>
// This will work
<span>
Hello <Span>{name$}</Span>, how are you?
</span>
Feel free to ask questions or submit pull requests!
The MIT License (MIT)
FAQs
create reactive react classes
The npm package react-reactive-class receives a total of 0 weekly downloads. As such, react-reactive-class popularity was classified as not popular.
We found that react-reactive-class demonstrated a not healthy version release cadence and project activity because the last version was released a year ago. It has 1 open source maintainer collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Research
Security News
Socket’s threat research team has detected six malicious npm packages typosquatting popular libraries to insert SSH backdoors.
Security News
MITRE's 2024 CWE Top 25 highlights critical software vulnerabilities like XSS, SQL Injection, and CSRF, reflecting shifts due to a refined ranking methodology.
Security News
In this segment of the Risky Business podcast, Feross Aboukhadijeh and Patrick Gray discuss the challenges of tracking malware discovered in open source softare.