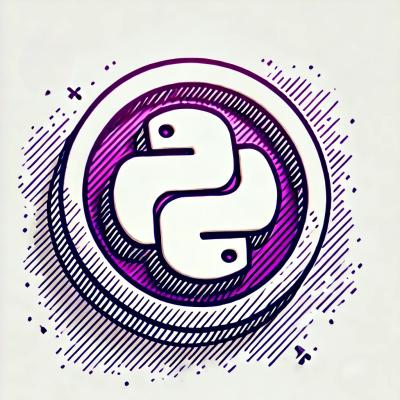
Security News
PyPI Introduces Digital Attestations to Strengthen Python Package Security
PyPI now supports digital attestations, enhancing security and trust by allowing package maintainers to verify the authenticity of Python packages.
react-redux-lifecycle
Advanced tools
A higher-order component to dispatch actions on react lifecycle methods
Often, actions need to be dispatched as part of the React lifecycle. In many cases, the only reason to extend React.Component
instead of using functional components is to do this.
Well, no more! Now you can just wrap your component in this higher-order component and remain in your functional bliss.
npm install --save react-redux-lifecycle
This assumes that you’re using npm package manager with a module bundler like Webpack or Browserify to consume CommonJS modules.
import { withLifecycleActions } from 'react-redux-lifecycle'
const myAction = { type: 'MY_ACTION' }
const MyComponent = ({ message }) => <div>{message}</div>
export default withLifecycleActions({ componentDidMount: myAction })(MyComponent)
...
import MyComponent from './MyComponent'
<MyComponent message="Hello World!" />
import { withLifecycleActions } from 'react-redux-lifecycle'
const myActionCreator = () => ({ type: 'MY_ACTION' })
const MyComponent = ({ message }) => <div>{message}</div>
export default withLifecycleActions({ componentDidMount: myActionCreator })(MyComponent)
...
import MyComponent from './MyComponent'
<MyComponent message="Hello World!" />
The component props are passed to any functions that are provided
import { withLifecycleActions } from 'react-redux-lifecycle'
const myActionCreator = ({ id }) => ({ type: 'MY_ACTION', id })
const MyComponent = ({ message }) => <div>{message}</div>
export default withLifecycleActions({ componentDidMount: myActionCreator })(MyComponent)
...
import MyComponent from './MyComponent'
<MyComponent id={123} message="Hello World!" />
import { withLifecycleActions } from 'react-redux-lifecycle'
const myAction = () => { type: 'MY_ACTION' }
const myActionCreator = () => myAction
const MyComponent = ({ message }) => <div>{message}</div>
export default withLifecycleActions({ componentDidMount: [myAction, myActionCreator] })(MyComponent)
...
import MyComponent from './MyComponent'
<MyComponent message="Hello World!" />
Helper functions are available if you only need to dispatch the action on a single lifecycle method.
import { onComponentDidMount } from 'react-redux-lifecycle'
const myAction = { type: 'MY_ACTION' }
const MyComponent = ({ message }) => <div>{message}</div>
export default onComponentDidMount(myAction)(MyComponent)
...
import MyComponent from './MyComponent'
<MyComponent message="Hello World!" />
Basic action, action creators and multiple actions are all supported in the same manner as withLifecycleActions
.
The following lifecycle methods are supported:
componentWillMount
(helper: onComponentWillMount
)componentDidMount
(helper: onComponentDidMount
)componentWillReceiveProps
(helper: onComponentWillReceiveProps
)componentWillUpdate
(helper: onComponentWillUpdate
)componentDidUpdate
(helper: onComponentDidUpdate
)componentWillUnmount
(helper: onComponentWillUnmount
)componentDidCatch
(helper: onComponentDidCatch
)FAQs
A higher-order component to dispatch actions on react lifecycle methods
The npm package react-redux-lifecycle receives a total of 353 weekly downloads. As such, react-redux-lifecycle popularity was classified as not popular.
We found that react-redux-lifecycle demonstrated a not healthy version release cadence and project activity because the last version was released a year ago. It has 1 open source maintainer collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
PyPI now supports digital attestations, enhancing security and trust by allowing package maintainers to verify the authenticity of Python packages.
Security News
GitHub removed 27 malicious pull requests attempting to inject harmful code across multiple open source repositories, in another round of low-effort attacks.
Security News
RubyGems.org has added a new "maintainer" role that allows for publishing new versions of gems. This new permission type is aimed at improving security for gem owners and the service overall.