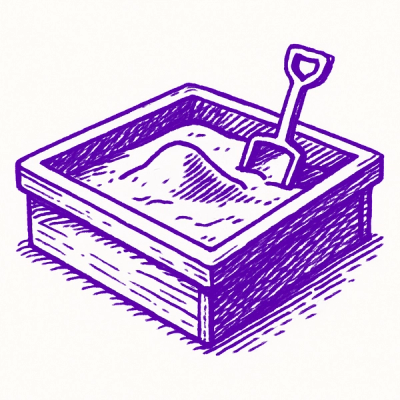
Research
/Security News
Critical Vulnerability in NestJS Devtools: Localhost RCE via Sandbox Escape
A flawed sandbox in @nestjs/devtools-integration lets attackers run code on your machine via CSRF, leading to full Remote Code Execution (RCE).
react-request-fullscreen
Advanced tools
Request fullscreen feature for browsers, used as a react component.
Request fullscreen feature for browsers, used as a react component.
npm i react-request-fullscreen
FulllScreen is a react component, so you can do like this.
import FullScreen from 'react-request-fullscreen';
render() {
return (
<FullScreen />
);
}
After that, use the FulllScreen refs(fullScreen()
) to request or exit fullscreen feature for browsers.
import React from 'react'
import ReactDOM from 'react-dom'
import FullScreen, { fullScreenSupported } from '../index'
// import FullScreen, { fullScreenSupported } from 'react-request-fullscreen'
import './App.less'
class App extends React.Component {
constructor (props) {
super(props)
this.state = {
isFullScreen: false
}
}
onFullScreenChange (isFullScreen) {
this.setState({
isFullScreen
})
}
requestOrExitFullScreen () {
this.fullScreenRef.fullScreen()
}
requestOrExitFullScreenByElement () {
this.elFullScreenRef.fullScreen(this.elRef)
}
render () {
const { isFullScreen } = this.state
return (
<div className='app'>
<p>Browser support fullscreen feature: {`${fullScreenSupported()}`}</p>
<p>Browser is fullscreen: {`${isFullScreen}`}</p>
<FullScreen ref={ref => { this.fullScreenRef = ref }} onFullScreenChange={this.onFullScreenChange.bind(this)}>
<div
className='rq'
onClick={this.requestOrExitFullScreen.bind(this)}
>
{!isFullScreen ? 'Request FullScreen' : 'Exit FullScreen'}
</div>
</FullScreen>
<FullScreen ref={ref => { this.elFullScreenRef = ref }}>
<div
className='el-rq'
ref={ref => { this.elRef = ref }}
onClick={this.requestOrExitFullScreenByElement.bind(this)}
>
{!isFullScreen ? 'Request FullScreen by Element' : 'Exit FullScreen by Element'}
</div>
</FullScreen>
<br />
<a href='https://github.com/TUBB/react-fullscreen'>GITHUB</a>
</div>
)
}
}
ReactDOM.render(
<App />,
document.getElementById('root')
)
Please see Demo project for detail.
FullScreen.fullScreen(element?: HTMLElement)
request or exit fullscreen feature.fullScreenSupported()
whether fullscreen feature is supported for the browser.isFullScreen()
the browser is fullscreen or not.Most code is come from chrisdickinson/fullscreen
FAQs
Request fullscreen feature for browsers, used as a react component.
The npm package react-request-fullscreen receives a total of 195 weekly downloads. As such, react-request-fullscreen popularity was classified as not popular.
We found that react-request-fullscreen demonstrated a not healthy version release cadence and project activity because the last version was released a year ago. It has 1 open source maintainer collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Research
/Security News
A flawed sandbox in @nestjs/devtools-integration lets attackers run code on your machine via CSRF, leading to full Remote Code Execution (RCE).
Product
Customize license detection with Socket’s new license overlays: gain control, reduce noise, and handle edge cases with precision.
Product
Socket now supports Rust and Cargo, offering package search for all users and experimental SBOM generation for enterprise projects.