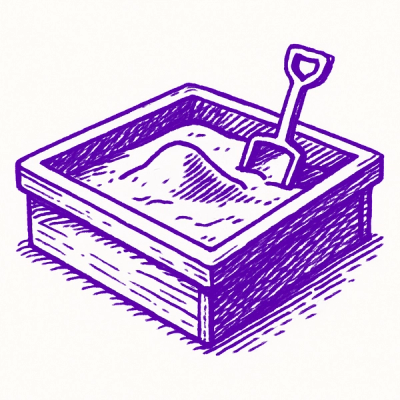
Research
/Security News
Critical Vulnerability in NestJS Devtools: Localhost RCE via Sandbox Escape
A flawed sandbox in @nestjs/devtools-integration lets attackers run code on your machine via CSRF, leading to full Remote Code Execution (RCE).
react-router
Advanced tools
The react-router npm package is a declarative routing library for React, allowing you to add navigation functionality to your React applications. It enables you to handle URL routing, match routes to your React components, and manage navigation state in a single-page application (SPA) environment.
Basic Routing
This code demonstrates how to set up basic routing in a React application using react-router. It includes navigation links and route components that render different components based on the URL path.
import { BrowserRouter as Router, Route, Link } from 'react-router-dom';
function App() {
return (
<Router>
<div>
<nav>
<ul>
<li>
<Link to='/'>Home</Link>
</li>
<li>
<Link to='/about'>About</Link>
</li>
</ul>
</nav>
<Route exact path='/' component={Home} />
<Route path='/about' component={About} />
</div>
</Router>
);
}
function Home() {
return <h2>Home</h2>;
}
function About() {
return <h2>About</h2>;
}
Dynamic Routing
This code snippet shows how to implement dynamic routing with path parameters. The User component will render with the appropriate user ID based on the URL.
import { BrowserRouter as Router, Route, Link } from 'react-router-dom';
function App() {
return (
<Router>
<div>
<ul>
<li>
<Link to='/users/1'>User 1</Link>
</li>
<li>
<Link to='/users/2'>User 2</Link>
</li>
</ul>
<Route path='/users/:id' component={User} />
</div>
</Router>
);
}
function User({ match }) {
return <h2>User ID: {match.params.id}</h2>;
}
Nested Routing
Nested routing allows you to create routes within routes. This example shows a Layout component with a nested Dashboard route.
import { BrowserRouter as Router, Route, Link, Switch } from 'react-router-dom';
function App() {
return (
<Router>
<Route path='/' component={Layout} />
</Router>
);
}
function Layout({ match }) {
return (
<div>
<nav>
<Link to={`${match.url}dashboard`}>Dashboard</Link>
</nav>
<Switch>
<Route path={`${match.path}dashboard`} component={Dashboard} />
</Switch>
</div>
);
}
function Dashboard() {
return <h2>Dashboard</h2>;
}
Protected Routes
Protected routes are used to restrict access to certain parts of your application. This example shows a route that renders a component only if the user is authenticated, otherwise it redirects to a login page.
import { BrowserRouter as Router, Route, Redirect } from 'react-router-dom';
function App() {
return (
<Router>
<Route path='/protected' render={() => (
isAuthenticated() ? (
<ProtectedComponent />
) : (
<Redirect to='/login' />
)
)} />
</Router>
);
}
function isAuthenticated() {
// Authentication logic here
return true;
}
function ProtectedComponent() {
return <h2>Protected</h2>;
}
Vue-router is the official router for Vue.js. It provides similar functionalities for Vue applications as react-router does for React applications, including nested routes, dynamic segments, and navigation guards. However, it is designed to work seamlessly with Vue's reactivity system.
Reach Router is another routing library for React, which aims to be more accessible and simpler to use than react-router. It has a smaller API surface area and focuses on accessibility by managing focus after route transitions. However, as of my knowledge cutoff in 2023, Reach Router has been officially merged with React Router, and its features have been integrated into React Router v6.
react-router
is the primary package in the React Router project.
npm i react-router
v7.7.1
Date: 2025-07-24
@react-router/dev
- Update to Prettier v3 for formatting when running react-router reveal --no-typescript
(#14049)⚠️ Unstable features are not recommended for production use
react-router
- RSC Data Mode: fix bug where routes with errors weren't forced to revalidate when shouldRevalidate
returned false
(#14026)react-router
- RSC Data Mode: fix Matched leaf route at location "/..." does not have an element or Component
warnings when error boundaries are rendered (#14021)Full Changelog: v7.7.0...v7.7.1
FAQs
Declarative routing for React
The npm package react-router receives a total of 15,909,398 weekly downloads. As such, react-router popularity was classified as popular.
We found that react-router demonstrated a healthy version release cadence and project activity because the last version was released less than a year ago. It has 4 open source maintainers collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Research
/Security News
A flawed sandbox in @nestjs/devtools-integration lets attackers run code on your machine via CSRF, leading to full Remote Code Execution (RCE).
Product
Customize license detection with Socket’s new license overlays: gain control, reduce noise, and handle edge cases with precision.
Product
Socket now supports Rust and Cargo, offering package search for all users and experimental SBOM generation for enterprise projects.