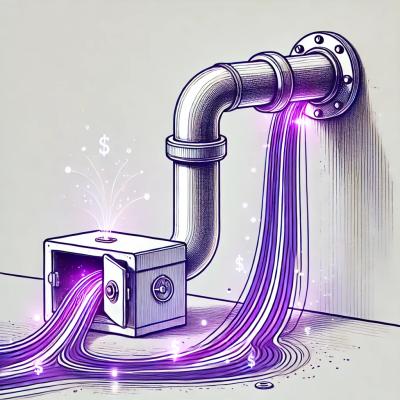
Research
Security News
Malicious npm Package Targets Solana Developers and Hijacks Funds
A malicious npm package targets Solana developers, rerouting funds in 2% of transactions to a hardcoded address.
react-router-carousel
Advanced tools
React carousel with the ability to switch routes, both with the usual swipe, and with the ability to add zones for swipe on the site
<Route path="*" component={FallbackPage} />
- ignores routes in the carousel. Please add the fallbackRoute prop for RouterCarousel with the 404 page component, as in the example belownpm install --save react-router-carousel
Property | Type | Description | Default | Example |
---|---|---|---|---|
sliderMode | bool | Standart carousel mode. Router will not switch | false | |
swipeLeftClassName | string | Custom className for swipe left zone | null | |
swipeRightClassName | string | Custom className for swipe right zone | null | |
fallbackRoute | component | If the entered route is not found this is the component that will be displayed | null | <FallbackPage /> |
Property | Type | Description | Default |
---|---|---|---|
index | number | Set active slider. Work only props sliderMode | 1 |
swipeleft | bool | Enable swipe left zone. If uses with props sliderMode add true or false - swipeleft="true" | false |
swiperight | bool | Enable swipe right zone. If uses with props sliderMode add true or false - swiperight="true" | false |
Demo - https://denisoed.github.io/react-router-carousel
Look at the page url
import React, { Component } from 'react';
import RouterCarousel from 'react-router-carousel';
import {
BrowserRouter,
Route,
NavLink,
Switch
} from 'react-router-dom';
import AuthHoc from './AuthHoc';
// Components
const Home = () => (
<div style={{ width: '100%', height: 540 }}>
<h1>Home page</h1>
<p>
Lorem ipsum dolor sit amet, consectetur adipiscing elit, sed do eiusmod
tempor incididunt ut labore et dolore magna aliqua. Ut enim ad minim
veniam, quis nostrud exercitation ullamco laboris nisi ut aliquip ex ea
commodo consequat.
</p>
</div>
);
const About = () => (
<div style={{ width: '100%', height: 540 }}>
<h1>About page</h1>
<p>
Lorem ipsum dolor sit amet, consectetur adipiscing elit, sed do eiusmod
tempor incididunt ut labore et dolore magna aliqua. Ut enim ad minim
veniam, quis nostrud exercitation ullamco laboris nisi ut aliquip ex ea
commodo consequat.
</p>
<NavLink to='/map' activeClassName='activeRoute'>
Map
</NavLink>
</div>
);
const Contact = () => (
<div style={{ width: '100%', height: 540, position: 'relative' }}>
<h1>Contact page</h1>
<p>
Lorem ipsum dolor sit amet, consectetur adipiscing elit, sed do eiusmod
tempor incididunt ut labore et dolore magna aliqua. Ut enim ad minim
veniam, quis nostrud exercitation ullamco laboris nisi ut aliquip ex ea
commodo consequat.
</p>
<section
style={{
width: '70%',
height: 70,
position: 'relative',
margin: '0 auto'
}}
>
<RouterCarousel
sliderMode
index="1"
swipeLeftClassName={'router-carousel-zone router-carousel-zone--left'}
swipeRightClassName={'router-carousel-zone router-carousel-zone--right'}
>
<h2 swipeleft='false' swiperight='true'>
EMail
</h2>
<h2>Phone number</h2>
<h2>Address</h2>
</RouterCarousel>
</section>
</div>
);
const Profile = () => (
<div style={{ width: '100%', height: 540 }}>
<h1>Profile page</h1>
<p>
Lorem ipsum dolor sit amet, consectetur adipiscing elit, sed do eiusmod
tempor incididunt ut labore et dolore magna aliqua. Ut enim ad minim
veniam, quis nostrud exercitation ullamco laboris nisi ut aliquip ex ea
commodo consequat.
</p>
</div>
);
const Map = () => (
<div style={{ width: '100%', height: 540 }}>
<h1>Map page</h1>
<p>
Lorem ipsum dolor sit amet, consectetur adipiscing elit, sed do eiusmod
tempor incididunt ut labore et dolore magna aliqua. Ut enim ad minim
veniam, quis nostrud exercitation ullamco laboris nisi ut aliquip ex ea
commodo consequat.
</p>
</div>
);
const Login = () => (
<div style={{ width: '100%', height: 540 }}>
<h1>Login page</h1>
<h3>React Hoc is works!</h3>
<p>
If the user is not authorized, it will be transferred to the authorization
page
</p>
</div>
);
const FallbackPage = () => (
<div style={{ width: '100%', height: 540 }}>
<h1>404 page</h1>
<p>
Lorem ipsum dolor sit amet, consectetur adipiscing elit, sed do eiusmod
tempor incididunt ut labore et dolore magna aliqua. Ut enim ad minim
veniam, quis nostrud exercitation ullamco laboris nisi ut aliquip ex ea
commodo consequat.
</p>
</div>
);
const Carousel = () => (
<RouterCarousel
swipeLeftClassName={'router-carousel-zone router-carousel-zone--left'}
swipeRightClassName={'router-carousel-zone router-carousel-zone--right'}
fallbackRoute={<FallbackPage />}
>
<Route exact path='/' component={Home} />
<Route path='/about' component={About} />
<Route path='/contact' component={Contact} swipeleft swiperight />
<Route path='/profile' component={AuthHoc(Profile)} />
</RouterCarousel>
);
const App = () => {
return (
<BrowserRouter>
<h1>React Router Carousel</h1>
<div
style={{
width: '98%',
height: 540,
position: 'relative',
margin: '0 auto',
padding: 20,
boxSizing: 'border-box',
overflow: 'hidden',
borderRadius: 10,
border: '1px solid #222',
textAlign: 'center'
}}
>
<Switch>
<Route path='/map' component={Map} />
<Route path='/login' component={Login} />
<Route path='*' component={Carousel} />
</Switch>
<div className='menu'>
<NavLink exact to='/' activeClassName='activeRoute'>
Home
</NavLink>
<NavLink to='/about' activeClassName='activeRoute'>
About
</NavLink>
<NavLink to='/contact' activeClassName='activeRoute'>
Contact
</NavLink>
<NavLink to='/profile' activeClassName='activeRoute'>
Profile
</NavLink>
</div>
</div>
</BrowserRouter>
);
};
Example can be found in the example/ folder
Boilerplate for create react plugins - https://github.com/transitive-bullshit/create-react-library
Local development is broken into two parts (ideally using two tabs).
First, run rollup to watch your src/
module and automatically recompile it into dist/
whenever you make changes.
npm start # runs rollup with watch flag
The second part will be running the example/
create-react-app that's linked to the local version of your module.
# (in another tab)
cd example
npm start # runs create-react-app dev server
Now, anytime you make a change to your library in src/
or to the example app's example/src
, create-react-app
will live-reload your local dev server so you can iterate on your component in real-time.
npm publish
This builds commonjs
and es
versions of your module to dist/
and then publishes your module to npm
.
Make sure that any npm modules you want as peer dependencies are properly marked as peerDependencies
in package.json
. The rollup config will automatically recognize them as peers and not try to bundle them in your module.
npm run deploy
This creates a production build of the example create-react-app
that showcases your library and then runs gh-pages
to deploy the resulting bundle.
If you use react-hooks in your project, when you debug your example you may run into an exception Invalid Hook Call Warning. This issue explains the reason, your lib and example use a different instance, one solution is rewrite the react
path in your example's package.json
to 'file:../node_modules/react' or 'link:../node_modules/react'.
FAQs
React Router Carousel
We found that react-router-carousel demonstrated a not healthy version release cadence and project activity because the last version was released a year ago. It has 1 open source maintainer collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Research
Security News
A malicious npm package targets Solana developers, rerouting funds in 2% of transactions to a hardcoded address.
Security News
Research
Socket researchers have discovered malicious npm packages targeting crypto developers, stealing credentials and wallet data using spyware delivered through typosquats of popular cryptographic libraries.
Security News
Socket's package search now displays weekly downloads for npm packages, helping developers quickly assess popularity and make more informed decisions.