react-rxinput
Advanced tools
Comparing version 1.0.4 to 1.1.0
988
lib/index.js
@@ -1,18 +0,50 @@ | ||
/* | ||
Copyright (c) 2016, Nurul Choudhury | ||
'use strict'; | ||
Permission to use, copy, modify, and/or distribute this software for any | ||
purpose with or without fee is hereby granted, provided that the above | ||
copyright notice and this permission notice appear in all copies. | ||
exports.__esModule = true; | ||
exports.RxInput = exports.RxInputBase = undefined; | ||
THE SOFTWARE IS PROVIDED "AS IS" AND THE AUTHOR DISCLAIMS ALL WARRANTIES | ||
WITH REGARD TO THIS SOFTWARE INCLUDING ALL IMPLIED WARRANTIES OF | ||
MERCHANTABILITY AND FITNESS. IN NO EVENT SHALL THE AUTHOR BE LIABLE FOR | ||
ANY SPECIAL, DIRECT, INDIRECT, OR CONSEQUENTIAL DAMAGES OR ANY DAMAGES | ||
WHATSOEVER RESULTING FROM LOSS OF USE, DATA OR PROFITS, WHETHER IN AN | ||
ACTION OF CONTRACT, NEGLIGENCE OR OTHER TORTIOUS ACTION, ARISING OUT OF | ||
OR IN CONNECTION WITH THE USE OR PERFORMANCE OF THIS SOFTWARE. | ||
var _extends = Object.assign || function (target) { for (var i = 1; i < arguments.length; i++) { var source = arguments[i]; for (var key in source) { if (Object.prototype.hasOwnProperty.call(source, key)) { target[key] = source[key]; } } } return target; }; | ||
*/ | ||
exports.hashStr = hashStr; | ||
var _react = require('react'); | ||
var _react2 = _interopRequireDefault(_react); | ||
var _reactBootstrap = require('react-bootstrap'); | ||
var _incrRegexPackage = require('incr-regex-package'); | ||
var _incrRegexPackage2 = _interopRequireDefault(_incrRegexPackage); | ||
function _interopRequireDefault(obj) { return obj && obj.__esModule ? obj : { default: obj }; } | ||
function _objectWithoutProperties(obj, keys) { var target = {}; for (var i in obj) { if (keys.indexOf(i) >= 0) continue; if (!Object.prototype.hasOwnProperty.call(obj, i)) continue; target[i] = obj[i]; } return target; } | ||
function _classCallCheck(instance, Constructor) { if (!(instance instanceof Constructor)) { throw new TypeError("Cannot call a class as a function"); } } | ||
function _possibleConstructorReturn(self, call) { if (!self) { throw new ReferenceError("this hasn't been initialised - super() hasn't been called"); } return call && (typeof call === "object" || typeof call === "function") ? call : self; } | ||
function _inherits(subClass, superClass) { if (typeof superClass !== "function" && superClass !== null) { throw new TypeError("Super expression must either be null or a function, not " + typeof superClass); } subClass.prototype = Object.create(superClass && superClass.prototype, { constructor: { value: subClass, enumerable: false, writable: true, configurable: true } }); if (superClass) Object.setPrototypeOf ? Object.setPrototypeOf(subClass, superClass) : subClass.__proto__ = superClass; } //component.js | ||
// const RX = require("incr-regex-package"); | ||
// const {convertMask,contract,RXInputMask,isMeta} = RX; | ||
/** | ||
* Copyright (c) 2016, Nurul Choudhury | ||
* | ||
* Permission to use, copy, modify, and/or distribute this software for any | ||
* purpose with or without fee is hereby granted, provided that the above | ||
* copyright notice and this permission notice appear in all copies. | ||
* | ||
* THE SOFTWARE IS PROVIDED "AS IS" AND THE AUTHOR DISCLAIMS ALL WARRANTIES | ||
* WITH REGARD TO THIS SOFTWARE INCLUDING ALL IMPLIED WARRANTIES OF | ||
* MERCHANTABILITY AND FITNESS. IN NO EVENT SHALL THE AUTHOR BE LIABLE FOR | ||
* ANY SPECIAL, DIRECT, INDIRECT, OR CONSEQUENTIAL DAMAGES OR ANY DAMAGES | ||
* WHATSOEVER RESULTING FROM LOSS OF USE, DATA OR PROFITS, WHETHER IN AN | ||
* ACTION OF CONTRACT, NEGLIGENCE OR OTHER TORTIOUS ACTION, ARISING OUT OF | ||
* OR IN CONNECTION WITH THE USE OR PERFORMANCE OF THIS SOFTWARE. | ||
* | ||
*/ | ||
// | ||
@@ -27,72 +59,96 @@ // Modified from https://github.com/insin/react-maskedinput | ||
'use strict'; | ||
var _extends = Object.assign || function (target) { for (var i = 1; i < arguments.length; i++) { var source = arguments[i]; for (var key in source) { if (Object.prototype.hasOwnProperty.call(source, key)) { target[key] = source[key]; } } } return target; }; | ||
//const convertMask = convertMask; | ||
var rxPlaceHolder = new RegExp((0, _incrRegexPackage.convertMask)('[?*]')); | ||
var KEYCODE_Z = 90; | ||
var KEYCODE_Y = 89; | ||
function _interopRequireDefault(obj) { return obj && obj.__esModule ? obj : { 'default': obj }; } | ||
function except(object, list) { | ||
var result = {}; | ||
var keys = list; | ||
function _objectWithoutProperties(obj, keys) { var target = {}; for (var i in obj) { if (keys.indexOf(i) >= 0) continue; if (!Object.prototype.hasOwnProperty.call(obj, i)) continue; target[i] = obj[i]; } return target; } | ||
for (var key in object) { | ||
if (keys.indexOf(key) === -1) { | ||
result[key] = object[key]; | ||
} | ||
} | ||
var _react = require('react'); | ||
return result; | ||
} | ||
var _react2 = _interopRequireDefault(_react); | ||
function isUndo(e) { | ||
return (e.ctrlKey || e.metaKey) && e.keyCode === (e.shiftKey ? KEYCODE_Y : KEYCODE_Z); | ||
} | ||
var _except = require('except'); | ||
function isRedo(e) { | ||
return (e.ctrlKey || e.metaKey) && e.keyCode === (e.shiftKey ? KEYCODE_Z : KEYCODE_Y); | ||
} | ||
var _except2 = _interopRequireDefault(_except); | ||
function getSelection(el) { | ||
var start = void 0, | ||
end = void 0, | ||
rangeEl = void 0, | ||
clone = void 0; | ||
var _reactBootstrap = require('react-bootstrap'); | ||
if (el.selectionStart !== undefined) { | ||
start = el.selectionStart; | ||
end = el.selectionEnd; | ||
} else { | ||
try { | ||
el.focus(); | ||
rangeEl = el.createTextRange(); | ||
clone = rangeEl.duplicate(); | ||
var _reactLibReactInputSelection = require('react/lib/ReactInputSelection'); | ||
rangeEl.moveToBookmark(document.selection.createRange().getBookmark()); | ||
clone.setEndPoint('EndToStart', rangeEl); | ||
var RX = require("incr-regex-package"); | ||
start = clone.text.length; | ||
end = start + rangeEl.text.length; | ||
} catch (e) {/* not focused or not visible */} | ||
} | ||
var KEYCODE_Z = 90; | ||
var KEYCODE_Y = 89; | ||
function isUndo(e) { | ||
return (e.ctrlKey || e.metaKey) && e.keyCode === (e.shiftKey ? KEYCODE_Y : KEYCODE_Z); | ||
return { start: start, end: end }; | ||
} | ||
function isRedo(e) { | ||
return (e.ctrlKey || e.metaKey) && e.keyCode === (e.shiftKey ? KEYCODE_Z : KEYCODE_Y); | ||
function setSelection(el, selection) { | ||
var rangeEl = void 0; | ||
try { | ||
if (el.selectionStart !== undefined) { | ||
el.focus(); | ||
el.setSelectionRange(selection.start, selection.end); | ||
} else { | ||
el.focus(); | ||
rangeEl = el.createTextRange(); | ||
rangeEl.collapse(true); | ||
rangeEl.moveStart('character', selection.start); | ||
rangeEl.moveEnd('character', selection.end - selection.start); | ||
rangeEl.select(); | ||
} | ||
} catch (e) {/* not focused or not visible */} | ||
} | ||
function supportArrowNavigation(mask) { | ||
return RX.contract.isFunc(mask.arrowAction); | ||
return _incrRegexPackage.contract.isFunc(mask.arrowAction); | ||
} | ||
function asStr(anObj) { | ||
return JSON.stringify(anObj); | ||
return JSON.stringify(anObj); | ||
} | ||
function eqSel(sel1, sel2) { | ||
if (sel1 === sel2) return true; | ||
if (sel1 === undefined || sel2 === undefined) return false; | ||
return sel1.start === sel2.start && sel1.end === sel2.end; | ||
if (sel1 === sel2) return true; | ||
if (sel1 === undefined || sel2 === undefined) return false; | ||
return sel1.start === sel2.start && sel1.end === sel2.end; | ||
} | ||
function selAt(sel, x) { | ||
if (!sel && x === 0) return true; | ||
return sel.start === x && (sel.end === undefined || sel.end === x); | ||
if (!sel && x === 0) return true; | ||
return sel.start === x && (sel.end === undefined || sel.end === x); | ||
} | ||
function strCmp1(a, b) { | ||
var nameA = a.toUpperCase(); // ignore upper and lowercase | ||
var nameB = b.toUpperCase(); // ignore upper and lowercase | ||
if (nameA < nameB) { | ||
return -1; | ||
} | ||
if (nameA > nameB) { | ||
return 1; | ||
} | ||
// names must be equal | ||
return 0; | ||
} | ||
var mapImg = { | ||
"DONE": [_react2['default'].createElement('span', { className: 'glyphicon glyphicon-ok form-control-feedback' }), ""], | ||
"MORE": [_react2['default'].createElement('span', { className: 'glyphicon glyphicon-arrow-right form-control-feedback' }), " has-warning"], | ||
"OK": [_react2['default'].createElement('span', { className: 'glyphicon glyphicon-option-horizontal form-control-feedback' }), ""] | ||
"DONE": [_react2.default.createElement('span', { className: 'glyphicon glyphicon-ok form-control-feedback' }), ""], | ||
"MORE": [_react2.default.createElement('span', { className: 'glyphicon glyphicon-arrow-right form-control-feedback' }), " has-warning"], | ||
"OK": [_react2.default.createElement('span', { className: 'glyphicon glyphicon-option-horizontal form-control-feedback' }), ""] | ||
}; | ||
@@ -102,356 +158,534 @@ | ||
var LOG = function LOG(x) { | ||
return x; | ||
return x; | ||
}; | ||
var RxInput = _react2['default'].createClass({ | ||
displayName: 'RxInput', | ||
var RxStatus = function (_Component) { | ||
_inherits(RxStatus, _Component); | ||
propTypes: { | ||
mask: _react2['default'].PropTypes.object.isRequired, | ||
name: _react2['default'].PropTypes.string.isRequired, | ||
popover: _react2['default'].PropTypes.string, | ||
selection: _react2['default'].PropTypes.object, | ||
value: _react2['default'].PropTypes.string | ||
}, | ||
function RxStatus() { | ||
_classCallCheck(this, RxStatus); | ||
getDefaultProps: function getDefaultProps() { | ||
return { | ||
value: '' | ||
}; | ||
}, | ||
return _possibleConstructorReturn(this, _Component.apply(this, arguments)); | ||
} | ||
getInitialState: function getInitialState() { | ||
var options = { | ||
pattern: this.props.mask, | ||
value: this.props.value | ||
}; | ||
return { focus: false, | ||
value: this.props.value, | ||
selection: this.props.selection, | ||
mask: new RX.RXInputMask(options) | ||
}; | ||
}, | ||
RxStatus.prototype.render = function render() { | ||
function printElem(_ref) { | ||
var code = _ref[0], | ||
typ = _ref[1]; | ||
componentWillReceiveProps: function componentWillReceiveProps(nextProps) { | ||
if (this.props.mask.toString() !== nextProps.mask.toString()) { | ||
//this.state.mask.setPattern(nextProps.mask, {value: this.state.mask.getRawValue()}); | ||
this.state.mask.setPattern(nextProps.mask, { value: nextProps.value, selection: this.state.mask.selection }); | ||
this.setState({ selection: this.state.selection, value: nextProps.value }); | ||
} else if (this.props.value !== nextProps.value) { | ||
this.state.mask.setValue(nextProps.value); | ||
} | ||
}, | ||
if (typ === undefined) return code; | ||
return _react2.default.createElement( | ||
'b', | ||
null, | ||
code | ||
); | ||
} | ||
var t = this.props.mask.pattern.getInputTracker() || []; | ||
return _react2.default.createElement( | ||
'div', | ||
null, | ||
t.map(printElem) | ||
); | ||
}; | ||
_updateMaskSelection: function _updateMaskSelection() { | ||
this.state.mask.selection = (0, _reactLibReactInputSelection.getSelection)(this.input); | ||
}, | ||
return RxStatus; | ||
}(_react.Component); | ||
_updateInputSelection: function _updateInputSelection() { | ||
if (!eqSel((0, _reactLibReactInputSelection.getSelection)(this.input), this.state.mask.selection)) (0, _reactLibReactInputSelection.setSelection)(this.input, this.state.mask.selection); | ||
}, | ||
function minV(minVal) { | ||
return function (val) { | ||
return Math.max(minVal, val); | ||
}; | ||
} | ||
_onFocus: function _onFocus(e) {}, | ||
function hashStr(str) { | ||
var hashVal = 5381, | ||
i = str.length; | ||
_onBlurr: function _onBlurr() {}, | ||
while (i) { | ||
hashVal = hashVal * 33 ^ str.charCodeAt(--i); | ||
}return (hashVal >>> 0) + 12; | ||
} | ||
_onChange: function _onChange(e) { | ||
// console.log('onChange', asStr(getSelection(this.input)), e.target.value) | ||
var mask = this.state.mask; | ||
var maskValue = mask.getValue(); | ||
if (e.target.value !== maskValue) { | ||
// Cut or delete operations will have shortened the value | ||
if (e.target.value.length < maskValue.length) { | ||
var sizeDiff = maskValue.length - e.target.value.length; | ||
this._updateMaskSelection(); | ||
mask.selection.end = mask.selection.start + sizeDiff; | ||
mask.backspace(); | ||
//console.log("Fix maskValue", maskValue, "diff:", sizeDiff, "target value: ", e.target.value); | ||
} | ||
var value = this._getDisplayValue(); | ||
e.target.value = value; | ||
if (value) { | ||
this._updateInputSelection(); | ||
} | ||
} | ||
this.setState({ selection: this.mask.selection }); | ||
if (this.props.onChange) { | ||
var opt = { target: { value: this.getValue() } }; | ||
this.props.onChange(opt); | ||
} | ||
// console.log("on change", e) | ||
}, | ||
var RxInputBase = exports.RxInputBase = function (_Component2) { | ||
_inherits(RxInputBase, _Component2); | ||
_onKeyDown: function _onKeyDown(e) { | ||
var _this = this; | ||
function RxInputBase(props) { | ||
_classCallCheck(this, RxInputBase); | ||
var mask = this.state.mask; | ||
var isKey = function isKey(keyV) { | ||
return function (e) { | ||
return e.key === keyV; | ||
}; | ||
}; | ||
var _this2 = _possibleConstructorReturn(this, _Component2.call(this, props)); | ||
var _C = function _C(test, action) { | ||
if (!test(e)) return false; | ||
e.preventDefault(); | ||
_this._updateMaskSelection(); | ||
if (action()) { | ||
var oldVal = e.target.value; | ||
var value = _this._getDisplayValue(); | ||
e.target.value = value; | ||
//console.log(action+":getDisplayValue", value); | ||
if (value) { | ||
_this._updateInputSelection(); | ||
} | ||
if (_this.props.onChange && oldVal != value) { | ||
var opt = { target: { value: mask._getValue() } }; | ||
_this.props.onChange(opt); | ||
//console.log("on change", e) | ||
} | ||
// console.log("on change1", e) | ||
} | ||
_this.setState({ selection: mask.selection }); | ||
return true; | ||
}; | ||
//console.log('onKeyDown',mask, asStr(getSelection(this.input))+"/"+asStr(mask.selection), e.key, e.keyCode, e.target.value) | ||
if (_C(isUndo, function () { | ||
return mask.undo(); | ||
}) || _C(isRedo, function () { | ||
return mask.redo(); | ||
}) || _C(isKey("Backspace"), function () { | ||
return mask.backspace(); | ||
}) || _C(isKey("Delete"), function () { | ||
return mask.del(); | ||
})) return; | ||
_this2.state = _this2.getInitialState(); | ||
_this2._onChange = _this2._onChange.bind(_this2); | ||
_this2._onKeyDown = _this2._onKeyDown.bind(_this2); | ||
_this2._onKeyPress = _this2._onKeyPress.bind(_this2); | ||
_this2._onPaste = _this2._onPaste.bind(_this2); | ||
_this2._onFocus = _this2._onFocus.bind(_this2); | ||
_this2._onBlur = _this2._onBlur.bind(_this2); | ||
_this2.input = null; | ||
return _this2; | ||
} | ||
// propTypes: { | ||
// mask: React.PropTypes.object.isRequired, | ||
// name: React.PropTypes.string.isRequired, | ||
// popover: React.PropTypes.string, | ||
// selection: React.PropTypes.object, | ||
// value: React.PropTypes.string, | ||
// }, | ||
if (e.metaKey || e.altKey || e.ctrlKey || e.shiftKey || e.key === 'Enter') { | ||
return; | ||
} | ||
if (e.key === 'ArrowLeft' || e.key == 'ArrowRight') { | ||
// Check if mask supports arrow support | ||
var sel = (0, _reactLibReactInputSelection.getSelection)(this.input); | ||
//mask.selection = sel; | ||
if (sel.start === sel.end && mask.left !== undefined) { | ||
e.preventDefault(); | ||
if (e.key === 'ArrowLeft') mask.left(sel);else mask.right(sel); | ||
this._updateInputSelection(); | ||
//this.refs.debug.props.forceUpdate(); | ||
} | ||
// get input() { | ||
// return this.myRef.current; | ||
// } | ||
//console.log("Arrow Action support:", supportArrowNavigation(mask), " value:",this._getDisplayValue(), " selection: ", asStr(getSelection(this.input)), asStr(mask.selection)); | ||
} | ||
}, | ||
RxInputBase.prototype.getInitialState = function getInitialState() { | ||
var options = { | ||
pattern: this.props.mask || /.*/, | ||
value: this.props.value || '' | ||
}; | ||
return { | ||
focus: false, | ||
value: this.props.value || '', | ||
selection: this.props.selection, | ||
mask: new _incrRegexPackage.RXInputMask(options) | ||
}; | ||
}; | ||
_onKeyPress: function _onKeyPress(e) { | ||
var mask = this.state.mask; | ||
//console.log('onKeyPress', asStr(getSelection(this.input)),asStr(mask.selection), e.key, e.target.value) | ||
RxInputBase.prototype.componentWillReceiveProps = function componentWillReceiveProps(nextProps) { | ||
if (this.props.mask.toString() !== nextProps.mask.toString()) { | ||
//this.state.mask.setPattern(nextProps.mask, {value: this.state.mask.getRawValue()}); | ||
this.state.mask.setPattern(nextProps.mask, { value: nextProps.value, selection: this.state.mask.selection }); | ||
this.setState({ selection: this.state.selection, value: nextProps.value }); | ||
} else if (this.props.value !== nextProps.value) { | ||
this.state.mask.setValue(nextProps.value); | ||
} | ||
}; | ||
// Ignore modified key presses | ||
// Ignore enter key to allow form submission | ||
if (e.metaKey || e.altKey || e.ctrlKey || e.key === 'Enter') { | ||
return; | ||
} | ||
var selX = (0, _reactLibReactInputSelection.getSelection)(this.input); | ||
var oldMaskX = mask.getSelection(); | ||
e.preventDefault(); | ||
this._updateMaskSelection(); | ||
// static getDerivedStateFromProps(nextProps, state) { | ||
// //if (this.props.mask.toString() !== nextProps.mask.toString()) { | ||
// let value = nextProps.value||''; | ||
// console.log('getDerivedStateFromProps',{oldValue: state.value, value, oldmask:state.mask.pattern.toString(),mask: nextProps.mask.toString() }); | ||
// if (state.mask.pattern.toString() !== nextProps.mask.toString()) { | ||
// //this.state.mask.setPattern(nextProps.mask, {value: this.state.mask.getRawValue()}); | ||
// state.mask.setPattern(nextProps.mask, {value, selection: state.mask.selection}); | ||
// //this.setState({ selection: this.state.selection, value: nextProps.value}); | ||
// return {...state, value: nextProps.value}; | ||
// } | ||
// else if (state.value !== value) { | ||
// state.mask.setValue(nextProps.value); | ||
// console.log("value change"); | ||
// return {...state }; | ||
// } | ||
// return null; | ||
// } | ||
if (insert(e.key)) { | ||
var oldVal = e.target.value; | ||
var value = mask.getValue(); | ||
e.target.value = value; | ||
//console.log("keyPress:getDisplayValue", this._getDisplayValue(), " selection: ", asStr(selX)+"/"+asStr(mask.selection)+"<"+asStr(oldMaskX)); | ||
this._updateInputSelection(); | ||
this.setState({ selection: mask.selection }); | ||
if (this.props.onChange && oldVal != value) { | ||
var opt = { target: { value: mask._getValue() } }; | ||
this.props.onChange(opt); | ||
} | ||
//console.log("on change", e) | ||
} | ||
function insert(ch) { | ||
if (mask.input(ch)) return true; | ||
if (ch !== ch.toUpperCase()) return mask.input(ch.toUpperCase());else if (ch != ch.toLowerCase()) return mask.input(ch.toLowerCase()); | ||
return false; | ||
} | ||
}, | ||
RxInputBase.prototype._updateMaskSelection = function _updateMaskSelection() { | ||
this.state.mask.selection = getSelection(this.input); | ||
}; | ||
_onPaste: function _onPaste(e) { | ||
var mask = this.state.mask; | ||
//console.log('onPaste', asStr(getSelection(this.input)), e.clipboardData.getData('Text'), e.target.value) | ||
RxInputBase.prototype._updateInputSelection = function _updateInputSelection() { | ||
if (!eqSel(getSelection(this.input), this.state.mask.selection)) setSelection(this.input, this.state.mask.selection); | ||
}; | ||
e.preventDefault(); | ||
this._updateMaskSelection(); | ||
// getData value needed for IE also works in FF & Chrome | ||
//console.log("paste: ", e.clipboardData.getData('Text')); | ||
if (mask.paste(e.clipboardData.getData('Text'))) { | ||
e.target.value = mask.getValue(); | ||
//console.log("undo:getDisplayValue", this._getDisplayValue()); | ||
// Timeout needed for IE | ||
setTimeout(this._updateInputSelection, 0); | ||
//this.props.onChange(e); | ||
this.setState({ selection: mask.selection }); | ||
} | ||
}, | ||
RxInputBase.prototype._onFocus = function _onFocus(e) { | ||
if (this.props.onFocus) this.props.onFocus(e); | ||
}; | ||
_getMaskList: function _getMaskList(flag) { | ||
var list = this.state.mask.minCharsList(!!flag); | ||
if (list && list.length < 20) return list; | ||
return this.state.mask.minCharsList(); | ||
}, | ||
RxInputBase.prototype._onBlur = function _onBlur(e) { | ||
this.fireChange(e); | ||
}; | ||
_getDisplayValue: function _getDisplayValue() { | ||
var value = this.state.mask.getValue(); | ||
return value === this.state.mask.emptyValue ? '' : value; | ||
}, | ||
RxInputBase.prototype.fireChange = function fireChange(e) { | ||
if (this.props.onChange) { | ||
var opt = { value: this.state.mask._getValue(), target: e.target, name: this.props.name, mask: this.state.mask }; | ||
//this.props.onChange(opt); | ||
this.props.onChange({ target: opt }); | ||
} | ||
}; | ||
selected: function selected(str, e) { | ||
//console.log("Selected: "+str); | ||
if (!str.split('').find(function (c) { | ||
return RX.isMeta(c) ? c : undefined; | ||
})) { | ||
var mask = this.state.mask; | ||
mask.setValue(str); | ||
this.setState({ mask: mask }); | ||
//console.log("Selected(done): "+str); | ||
} | ||
}, | ||
RxInputBase.prototype._onChange = function _onChange(e) { | ||
// console.log('onChange', asStr(getSelection(this.input)), e.target.value) | ||
var mask = this.state.mask; | ||
var maskValue = mask.getValue(); | ||
if (e.target.value !== maskValue) { | ||
// Cut or delete operations will have shortened the value | ||
if (e.target.value.length < maskValue.length) { | ||
var sizeDiff = maskValue.length - e.target.value.length; | ||
this._updateMaskSelection(); | ||
mask.selection.end = mask.selection.start + sizeDiff; | ||
mask.backspace(); | ||
//console.log("Fix maskValue", maskValue, "diff:", sizeDiff, "target value: ", e.target.value); | ||
} | ||
var value = this._getDisplayValue(); | ||
e.target.value = value; | ||
if (value) { | ||
this._updateInputSelection(); | ||
} | ||
} | ||
this.setState({ selection: this.mask.selection }); | ||
this.fireChange(e); | ||
// console.log("on change", e) | ||
}; | ||
_createPopover: function _createPopover(valueList, headers, maxWidth) { | ||
var _this2 = this; | ||
RxInputBase.prototype._onKeyDown = function _onKeyDown(e) { | ||
var _this3 = this; | ||
var strip = function strip(s) { | ||
return LOG(s.replace(/\u0332/g, ""), "strip:"); | ||
}; | ||
maxWidth = Math.max(150, maxWidth || 12 * Math.max.apply(null, valueList.map(function (a) { | ||
return Math.min(25, strip(a).length); | ||
}))); | ||
LOG(maxWidth, "MAX WIDTH:"); | ||
var MAXWIDTH = maxWidth || 200; | ||
var SPANSTYLE = { width: MAXWIDTH - 50, maxWidth: MAXWIDTH - 50 }; | ||
var TS = undefined, | ||
PADDING = undefined; | ||
if (!valueList || valueList.length <= 1) return _react2['default'].createElement('div', null); | ||
if (valueList.length > 20) { | ||
TS = { height: "400px", display: "block", overflow: "auto" }; | ||
PADDING = _react2['default'].createElement( | ||
'div', | ||
null, | ||
' ' | ||
); | ||
} | ||
function hash(str) { | ||
var hashVal = 5381, | ||
i = str.length; | ||
var mask = this.state.mask; | ||
var isKey = function isKey(keyV) { | ||
return function (e) { | ||
return e.key === keyV; | ||
}; | ||
}; | ||
while (i) hashVal = hashVal * 33 ^ str.charCodeAt(--i); | ||
return (hashVal >>> 0) + 12; | ||
} | ||
var me = this; | ||
return _react2['default'].createElement( | ||
_reactBootstrap.Popover, | ||
{ id: this.props.name + "myPopover", className: 'col-xs-10 col-md-10', style: { width: MAXWIDTH, maxWidth: MAXWIDTH, fontSize: "10px", marginTop: "10px", marginBottom: "10px" } }, | ||
_react2['default'].createElement( | ||
'table', | ||
{ key: this.props.name + "myPopover1", className: 'table-responsive table-striped table-hover table-condensed col-xs-10 col-md-10', style: SPANSTYLE }, | ||
_react2['default'].createElement( | ||
'thead', | ||
null, | ||
_react2['default'].createElement( | ||
'tr', | ||
null, | ||
headers.map(function (e) { | ||
return _react2['default'].createElement( | ||
'th', | ||
{ key: _this2.props.name + e }, | ||
e | ||
); | ||
}) | ||
) | ||
), | ||
_react2['default'].createElement( | ||
'tbody', | ||
{ style: TS }, | ||
valueList.sort(strCmp1).map(function (l) { | ||
return _react2['default'].createElement( | ||
'tr', | ||
{ onClick: function (e) { | ||
return me.selected(l, e); | ||
}, key: _this2.props.name + "L" + hash(l) }, | ||
_react2['default'].createElement( | ||
'td', | ||
{ onClick: function (e) { | ||
return me.selected(l, e); | ||
} }, | ||
l | ||
) | ||
); | ||
}) | ||
) | ||
), | ||
PADDING | ||
); | ||
}, | ||
var _C = function _C(test, action) { | ||
if (!test(e)) return false; | ||
e.preventDefault(); | ||
_this3._updateMaskSelection(); | ||
if (action()) { | ||
var oldVal = e.target.value; | ||
var value = _this3._getDisplayValue(); | ||
e.target.value = value; | ||
//console.log(action+":getDisplayValue", value); | ||
if (value) { | ||
_this3._updateInputSelection(); | ||
} | ||
if (_this3.props.onChange && oldVal != value) { | ||
//let opt = {target: {value: mask._getValue()}}; | ||
//this.props.onChange(opt); | ||
_this3.fireChange(e); | ||
//console.log("on change", e) | ||
} | ||
// console.log("on change1", e) | ||
} | ||
_this3.setState({ selection: mask.selection }); | ||
return true; | ||
}; | ||
//console.log('onKeyDown',mask, asStr(getSelection(this.input))+"/"+asStr(mask.selection), e.key, e.keyCode, e.target.value) | ||
if (_C(isUndo, function () { | ||
return mask.undo(); | ||
}) || _C(isRedo, function () { | ||
return mask.redo(); | ||
}) || _C(isKey("Backspace"), function () { | ||
return mask.backspace(); | ||
}) || _C(isKey("Delete"), function () { | ||
return mask.del(); | ||
})) return; | ||
render: function render() { | ||
var _this3 = this; | ||
if (e.metaKey || e.altKey || e.ctrlKey || e.shiftKey || e.key === 'Enter' || e.key === 'Tab') { | ||
return; | ||
} | ||
if (e.key === 'ArrowLeft' || e.key == 'ArrowRight') { | ||
// Check if mask supports arrow support | ||
var sel = getSelection(this.input); | ||
//mask.selection = sel; | ||
if (sel.start === sel.end && mask.left !== undefined) { | ||
e.preventDefault(); | ||
if (e.key === 'ArrowLeft') mask.left(sel);else mask.right(sel); | ||
this._updateInputSelection(); | ||
//this.refs.debug.props.forceUpdate(); | ||
} | ||
var _props = this.props; | ||
var mask = _props.mask; | ||
var size = _props.size; | ||
var placeholder = _props.placeholder; | ||
//console.log("Arrow Action support:", supportArrowNavigation(mask), " value:",this._getDisplayValue(), " selection: ", asStr(getSelection(this.input)), asStr(mask.selection)); | ||
} | ||
}; | ||
var props = _objectWithoutProperties(_props, ['mask', 'size', 'placeholder']); | ||
RxInputBase.prototype._onKeyPress = function _onKeyPress(e) { | ||
var mask = this.state.mask; | ||
//console.log('onKeyPress', asStr(getSelection(this.input)),asStr(mask.selection), e.key, e.target.value) | ||
var inpProps = (0, _except2['default'])(this.props, ['popover', 'mask', 'selection']); | ||
//console.log("PROPS:", inpProps); | ||
var OK = undefined; | ||
var pat = this.state.mask.pattern; | ||
var patternLength = pat.length; | ||
//console.log(`about to render name:'${this.props.name}' - ${this.state.mask.isDone()}`); | ||
var myPopover = this.props.popover ? this._createPopover(this._getMaskList(), ['Possible Values']) : _react2['default'].createElement('span', null); | ||
//console.log("about to render - " + this.state.mask.isDone()); | ||
var ok = this.state.mask.isDone(); | ||
if (ok) OK = mapImg[this.state.mask.isDone()]; //<span className="input-group-addon">.00</span>; // | ||
// Ignore modified key presses | ||
// Ignore enter key to allow form submission | ||
if (e.metaKey || e.altKey || e.ctrlKey || e.key === 'Enter') { | ||
return; | ||
} | ||
var selX = getSelection(this.input); | ||
var oldMaskX = mask.getSelection(); | ||
e.preventDefault(); | ||
this._updateMaskSelection(); | ||
var warningStyle = { marginBotton: "0px", fontSize: "70%", color: 'red', fontStyle: 'italic' }; | ||
var selDisplay = ""; | ||
var inputField = _react2['default'].createElement( | ||
'div', | ||
{ style: { marginBotton: "0px", paddingLeft: "100px" } }, | ||
_react2['default'].createElement( | ||
'div', | ||
{ style: warningStyle }, | ||
ok, | ||
' ' | ||
), | ||
_react2['default'].createElement( | ||
'div', | ||
{ className: "form-group has-feedback" + OK[1] }, | ||
_react2['default'].createElement( | ||
_reactBootstrap.OverlayTrigger, | ||
{ trigger: 'focus', style: { marginBotton: "0px" }, ref: 'mypop', placement: 'bottom', overlay: myPopover }, | ||
_react2['default'].createElement('input', _extends({}, inpProps, { | ||
className: 'form-control', | ||
ref: function (r) { | ||
return _this3.input = r; | ||
}, | ||
maxLength: patternLength, | ||
onChange: this._onChange, | ||
onKeyDown: this._onKeyDown, | ||
onKeyPress: this._onKeyPress, | ||
onPaste: this._onPaste, | ||
placeholder: placeholder || this.state.mask.emptyValue, | ||
size: size || patternLength, | ||
value: this._getDisplayValue(), | ||
style: { padding: "3px 0px 3px 0px" } | ||
})) | ||
), | ||
OK[0] | ||
) | ||
); | ||
return inputField; | ||
} | ||
}); | ||
module.exports = RxInput; | ||
if (insert(e.key)) { | ||
var oldVal = e.target.value; | ||
var value = mask.getValue(); | ||
e.target.value = value; | ||
//console.log("keyPress:getDisplayValue", this._getDisplayValue(), " selection: ", asStr(selX)+"/"+asStr(mask.selection)+"<"+asStr(oldMaskX)); | ||
this._updateInputSelection(); | ||
this.setState({ selection: mask.selection }); | ||
if (this.props.onChange && oldVal != value) { | ||
var opt = { target: { value: mask._getValue() } }; | ||
this.props.onChange(opt); | ||
} | ||
//console.log("on change", e) | ||
} | ||
function insert(ch) { | ||
if (mask.input(ch)) return true; | ||
if (ch !== ch.toUpperCase()) return mask.input(ch.toUpperCase());else if (ch != ch.toLowerCase()) return mask.input(ch.toLowerCase()); | ||
return false; | ||
} | ||
}; | ||
RxInputBase.prototype._onPaste = function _onPaste(e) { | ||
var mask = this.state.mask; | ||
//console.log('onPaste', asStr(getSelection(this.input)), e.clipboardData.getData('Text'), e.target.value) | ||
e.preventDefault(); | ||
this._updateMaskSelection(); | ||
// getData value needed for IE also works in FF & Chrome | ||
//console.log("paste: ", e.clipboardData.getData('Text')); | ||
if (mask.paste(e.clipboardData.getData('Text'))) { | ||
e.target.value = mask.getValue(); | ||
//console.log("undo:getDisplayValue", this._getDisplayValue()); | ||
// Timeout needed for IE | ||
setTimeout(this._updateInputSelection, 0); | ||
//this.props.onChange(e); | ||
this.setState({ selection: mask.selection }); | ||
} | ||
}; | ||
RxInputBase.prototype._getMaskList = function _getMaskList(flag) { | ||
var list = this.state.mask.minCharsList(!!flag); | ||
if (list && list.length < 20) return list; | ||
return this.state.mask.minCharsList(); | ||
}; | ||
RxInputBase.prototype._getDisplayValue = function _getDisplayValue() { | ||
var value = this.state.mask.getValue(); | ||
return value === this.state.mask.emptyValue ? '' : value; | ||
}; | ||
RxInputBase.prototype.selected = function selected(str, e) { | ||
//console.log("Selected: "+str); | ||
if (!str.split('').find(function (c) { | ||
return (0, _incrRegexPackage.isMeta)(c) ? c : undefined; | ||
})) { | ||
var mask = this.state.mask; | ||
mask.setValue(str); | ||
this.setState({ mask: mask }); | ||
//console.log("Selected(done): "+str); | ||
} | ||
}; | ||
RxInputBase.prototype.getMaxWidth = function getMaxWidth(valueList, maxWidth) { | ||
var dflt = arguments.length > 2 && arguments[2] !== undefined ? arguments[2] : 200; | ||
if (maxWidth) return Math.max(maxWidth, dflt); | ||
if (!valueList || !valueList.length) return dflt; | ||
var len = function len(s) { | ||
return s.replace(/\u0332/g, "").length; | ||
}; | ||
var lenList = valueList.map(len).map(minV(20)); | ||
return 12 * Math.max.apply(null, lenList); | ||
}; | ||
RxInputBase.prototype.getMapImg = function getMapImg() { | ||
return mapImg; | ||
}; | ||
RxInputBase.prototype.getRxPlaceHolder = function getRxPlaceHolder() { | ||
return rxPlaceHolder; | ||
}; | ||
RxInputBase.prototype.getInput = function getInput(input) { | ||
return input; | ||
}; | ||
RxInputBase.prototype.inputClassName = function inputClassName() { | ||
return "form-control"; | ||
}; | ||
RxInputBase.prototype.getPopoverData = function getPopoverData(valueList, headers, maxWidth, placeholder) { | ||
var _this4 = this; | ||
var MAXWIDTH = this.getMaxWidth(valueList, maxWidth, 300); | ||
if (!valueList || valueList.length <= 1) { | ||
if (!placeholder) return undefined; //{valueList: [""], headers, MAXWIDTH, hasSmallHeader: false} ; | ||
else valueList = [placeholder]; | ||
} | ||
var val = this._getDisplayValue() || ''; | ||
var ph = placeholder || this.state.mask.emptyValue; | ||
var popList = [val, ph].concat(valueList); | ||
var hasSmallHeader = popList.find(function (v) { | ||
return v.match(_this4.getRxPlaceHolder()); | ||
}); | ||
return { valueList: valueList, headers: headers, MAXWIDTH: MAXWIDTH, hasSmallHeader: hasSmallHeader }; | ||
}; | ||
RxInputBase.prototype.render = function render() { | ||
var _this5 = this; | ||
var _props = this.props, | ||
mask = _props.mask, | ||
size = _props.size, | ||
placeholder = _props.placeholder, | ||
popover = _props.popover, | ||
selection = _props.selection, | ||
showAll = _props.showAll, | ||
props = _objectWithoutProperties(_props, ['mask', 'size', 'placeholder', 'popover', 'selection', 'showAll']); | ||
var patternLength = this.state.mask.pattern.length; | ||
var setRef = function setRef(aDomElem) { | ||
_this5.input = aDomElem; | ||
}; | ||
var input = _react2.default.createElement('input', _extends({ | ||
style: { padding: "3px 0px 3px 0px" }, | ||
className: this.inputClassName() | ||
}, props, { | ||
ref: setRef, | ||
maxLength: patternLength, | ||
onChange: this._onChange, | ||
onKeyDown: this._onKeyDown, | ||
onKeyPress: this._onKeyPress, | ||
onPaste: this._onPaste, | ||
onFocus: this._onFocus, | ||
onBlur: this._onBlur, | ||
placeholder: placeholder || this.state.mask.emptyValue, | ||
size: size || patternLength, | ||
value: this._getDisplayValue() | ||
})); | ||
return this.getInput(input, placeholder); | ||
}; | ||
return RxInputBase; | ||
}(_react.Component); | ||
//const LOG = (first, ...params) => {console.log(first, ...params); return first; } | ||
function strCmp1(a, b) { | ||
var nameA = a.toUpperCase(); // ignore upper and lowercase | ||
var nameB = b.toUpperCase(); // ignore upper and lowercase | ||
if (nameA < nameB) { | ||
return -1; | ||
} | ||
if (nameA > nameB) { | ||
return 1; | ||
} | ||
// names must be equal | ||
return 0; | ||
} | ||
var RxInput = exports.RxInput = function (_RxInputBase) { | ||
_inherits(RxInput, _RxInputBase); | ||
function RxInput() { | ||
_classCallCheck(this, RxInput); | ||
return _possibleConstructorReturn(this, _RxInputBase.apply(this, arguments)); | ||
} | ||
RxInput.prototype._createPopover = function _createPopover(props) { | ||
var _this7 = this; | ||
if (!props) return _react2.default.createElement('span', null); | ||
var MAXWIDTH = props.MAXWIDTH, | ||
hasSmallHeader = props.hasSmallHeader, | ||
valueList = props.valueList, | ||
headers = props.headers; | ||
var smallHeader = hasSmallHeader ? smallHeader = _react2.default.createElement( | ||
'pre', | ||
{ className: 'text-muted small-text' }, | ||
(0, _incrRegexPackage.convertMask)('? - optional, * - zero or more') | ||
) : ""; | ||
var SPANSTYLE = { width: MAXWIDTH - 50, maxWidth: MAXWIDTH - 50 }; | ||
var TS = void 0, | ||
PADDING = void 0; | ||
console.log("valueList", valueList); | ||
if (valueList.length > 20) { | ||
TS = { height: "400px", display: "block", overflow: "auto" }; | ||
PADDING = _react2.default.createElement( | ||
'div', | ||
null, | ||
' \xA0\xA0\xA0\xA0\xA0\xA0\xA0\xA0\xA0\xA0\xA0\xA0' | ||
); | ||
} | ||
var me = this; | ||
return _react2.default.createElement( | ||
_reactBootstrap.Popover, | ||
{ id: this.props.name + "myPopover", className: 'col-xs-10 col-md-10', style: { width: MAXWIDTH, maxWidth: MAXWIDTH, fontSize: "10px", marginTop: "10px", marginBottom: "10px" } }, | ||
smallHeader, | ||
_react2.default.createElement( | ||
'table', | ||
{ key: this.props.name + "myPopover1", className: 'table-responsive table-striped table-hover table-condensed col-xs-10 col-md-10', style: SPANSTYLE }, | ||
_react2.default.createElement( | ||
'thead', | ||
null, | ||
_react2.default.createElement( | ||
'tr', | ||
null, | ||
headers.map(function (e) { | ||
return _react2.default.createElement( | ||
'th', | ||
{ key: _this7.props.name + e }, | ||
e | ||
); | ||
}) | ||
) | ||
), | ||
_react2.default.createElement( | ||
'tbody', | ||
{ style: TS }, | ||
valueList.sort(strCmp1).map(function (l) { | ||
return _react2.default.createElement( | ||
'tr', | ||
{ onClick: function onClick(e) { | ||
return me.selected(l, e); | ||
}, key: _this7.props.name + "L" + hashStr(l) }, | ||
_react2.default.createElement( | ||
'td', | ||
{ onClick: function onClick(e) { | ||
return me.selected(l, e); | ||
} }, | ||
l | ||
) | ||
); | ||
}) | ||
) | ||
), | ||
PADDING | ||
); | ||
}; | ||
RxInput.prototype.getInput = function getInput(input, placeholder) { | ||
var OK = void 0; | ||
var warningStyle = { marginBotton: "0px", fontSize: "70%", color: 'red', fontStyle: 'italic' }; | ||
var mapImg = this.getMapImg(); | ||
//let status = <RxStatus mask={this.state.mask}; | ||
var status = ""; | ||
var popOverData = this.getPopoverData(this._getMaskList(this.props.showAll !== 'no'), ['Possible Values'], undefined, placeholder); | ||
var myPopover = this.props.popover ? this._createPopover(popOverData) : _react2.default.createElement('span', null); | ||
var ok = this.state.mask.isDone(); | ||
if (ok) OK = mapImg[this.state.mask.isDone()]; //<span className="input-group-addon">.00</span>; // | ||
return _react2.default.createElement( | ||
'div', | ||
{ style: { marginBotton: "0px", paddingLeft: "100px" } }, | ||
_react2.default.createElement( | ||
'div', | ||
{ style: warningStyle }, | ||
ok, | ||
' \xA0' | ||
), | ||
status, | ||
_react2.default.createElement( | ||
'div', | ||
{ className: "form-group has-feedback" + OK[1] }, | ||
_react2.default.createElement( | ||
_reactBootstrap.OverlayTrigger, | ||
{ trigger: 'focus', style: { marginBotton: "0px" }, placement: 'bottom', overlay: myPopover }, | ||
input | ||
), | ||
OK[0] | ||
) | ||
); | ||
}; | ||
return RxInput; | ||
}(RxInputBase); |
{ | ||
"name": "react-rxinput", | ||
"version": "1.0.4", | ||
"description": "react-rxinput React component, extends <input> element to validate against a regular expression as you type input (incremental regex matcher)", | ||
"version": "1.1.0", | ||
"description": "react-rxinput React extends input element to validate against a regular expression as you type input (incremental regex matcher)", | ||
"main": "lib/index.js", | ||
"jsnext:main": "es6/index.js", | ||
"jsnext:main": "es/index.js", | ||
"files": [ | ||
"css", | ||
"es6", | ||
"es", | ||
"lib", | ||
@@ -14,25 +14,42 @@ "umd" | ||
"scripts": { | ||
"build": "nwb build && cp demo/dist/demo.* ../nurulc.github.io/", | ||
"clean": "nwb clean", | ||
"start": "nwb serve", | ||
"test": "nwb test", | ||
"test:watch": "nwb test --server" | ||
"buildex": "nwb build-react-component && shx cp demo/dist/demo.*.js ../nurulc.github.io/demo.js && shx cp demo/dist/demo.*.css ../nurulc.github.io/demo.css && shx cp demo/dist/runtime.*.js ../nurulc.github.io/runtime.js", | ||
"build": "nwb build-react-component", | ||
"clean": "nwb clean-module && nwb clean-demo", | ||
"prepublishOnly": "npm run build", | ||
"start": "nwb serve-react-demo", | ||
"test": "nwb test-react", | ||
"test:coverage": "nwb test-react --coverage", | ||
"test:watch": "nwb test-react --server", | ||
"webpack": "webpack" | ||
}, | ||
"dependencies": { | ||
"except": "^0.1.3", | ||
"incr-regex-package": "^0.9.6", | ||
"react-bootstrap": "^0.30.2" | ||
}, | ||
"dependencies": {}, | ||
"peerDependencies": { | ||
"react": "15.x" | ||
"incr-regex-package": ">=1", | ||
"react": ">=15", | ||
"react-dom": ">=15", | ||
"react-bootstrap": ">=1" | ||
}, | ||
"devDependencies": { | ||
"html-webpack-plugin": "^2.22.0", | ||
"nwb": "0.11.x", | ||
"react": "^15.3.0", | ||
"react-dom": "^15.3.0" | ||
"@babel/cli": ">=7.7", | ||
"@babel/core": ">=7.7", | ||
"@babel/plugin-transform-react-jsx": ">=7.7", | ||
"@babel/preset-env": ">=7.7", | ||
"@babel/preset-react": ">=7.7", | ||
"@babel/register": ">=7.7", | ||
"babel-loader": ">=8", | ||
"clean-webpack-plugin": ">=3", | ||
"except": "^0.1.3", | ||
"incr-regex-package": ">=1", | ||
"react": ">=15", | ||
"react-bootstrap": ">=1.0.0-beta.16", | ||
"react-dom": "^16.12.0", | ||
"shelljs": "^0.8.3", | ||
"shx": "^0.3.2", | ||
"terser-webpack-plugin": "^2.3.0", | ||
"webpack": ">=4", | ||
"webpack-cli": ">=3" | ||
}, | ||
"author": "Nurul Choudhury", | ||
"license": "ISC", | ||
"homepage": "", | ||
"homepage": "https://nurulc.github..io", | ||
"repository": { | ||
@@ -44,2 +61,6 @@ "type": "git", | ||
"react-component", | ||
"react", | ||
"autocomplete", | ||
"partial", | ||
"suggestion", | ||
"regular-expression", | ||
@@ -49,4 +70,5 @@ "dynamic", | ||
"validation", | ||
"input" | ||
"input", | ||
"RegExp" | ||
] | ||
} |
# react-rxinput | ||
[![Travis][build-badge]][build] | ||
[![npm package][npm-badge]][npm] | ||
[![Coveralls][coveralls-badge]][coveralls] | ||
## Changes | ||
### v2.0 | ||
* Removed bootstrap dependency and made the component UI neural and the expense of reduced visual functionality. | ||
* The functionality can be reintroduced through customization | ||
[build-badge]: https://img.shields.io/travis/user/repo/master.svg?style=flat-square | ||
[build]: https://travis-ci.org/user/repo | ||
## Summary | ||
[npm-badge]: https://img.shields.io/npm/v/npm-package.svg?style=flat-square | ||
[npm]: https://www.npmjs.org/package/npm-package | ||
* React Component | ||
* Input component | ||
* RegExp to define - validation, masks, ... | ||
* Not require coding to for most validation | ||
[coveralls-badge]: https://img.shields.io/coveralls/user/repo/master.svg?style=flat-square | ||
[coveralls]: https://coveralls.io/github/user/repo | ||
# react-rxinput | ||
## Introduction | ||
## Summary | ||
I stared with a simple challange, how can I make input validation both easy to usefor developes and anso easy to use for the end user.So lets start with very simple usecase usecase: | ||
A flexible input validation widget that validates you input as you type. You use a regular expression to validate the input. | ||
- Enter a phone number: 3 digit area-code, 3 digit exchange and 4 digit number | ||
[Demo](https://nurulc.github.io/) | ||
* Options | ||
1. No validation - Just use an input box and allow user to enter anything | ||
2. Application validation - allow user to enter anything, the application checks the format when the form is submitted | ||
3. Form validation in Javascript - allow user to enter anything, trap the onsubmit event and use a regular expression to validate the text in the input field; perhaps a regular expression like this ___/\d{3} \d{3} \d{4}/___, just as a reminder \d will match any digit, and \d{3} will match 3 digits. | ||
See [Wiki for more technical details](https://github.com/nurulc/react-rxinput/wiki) | ||
A flexible input validation widget that validates you input as you type. You use a regular expression to validate the input. As all tools this little component scratches an itch - needed an form input component that would allow only valid input, guide the user as he or she in typing in the input text, perform full/partial auto complete. Most of all it should use validation components that is standard, and there is nothing more standard that regular expressions for string matching. The only problem is that JavaScript RegExp requires the entire input before it can validate. Use **incr-regex-package** implements a stream (one character at a time RegExp matching). _Note: not intenders to replace JavaScript RegExp, an alternative to regexp for these use cases._ | ||
This component was inspired by [react-maskinput](https://github.com/insin/react-maskedinput) a component to support fixed masked input, e.g. phone number, credit card, etc. The good thing is that it does validation as you type, but the validation is very limited (you can create your custom validation code - but you have to code). The description of the mask is easy but not very flexible and is specific to this component. | ||
[Demo](https://nurulc.github.io/rxinput.html) | ||
## Highlights: | ||
- Easy to use (__my opinin__) | ||
- Use regular expression to define 'masked input' [react-maskinput](project inspired by https://github.com/insin/react-maskinput) | ||
- Good support for fixed format entry (mask input) - example Phone number, date, credit card number | ||
- Semi-fixed format, for example URL, email | ||
- Mix of from a fixed list (dropdown list) and user entry formats | ||
- Easy to use (__my opinion__) | ||
- Use regular expression to define 'masked input' [react-maskinput](https://github.com/insin/react-maskedinput) | ||
- RegExp matching/validation as you type | ||
@@ -48,7 +66,7 @@ - Auto complete | ||
[Please try out the demo](https://nurulc.github.io/) | ||
[Please try out the demo](https://nurulc.github.io/rxinput.html) | ||
## Screenshot | ||
[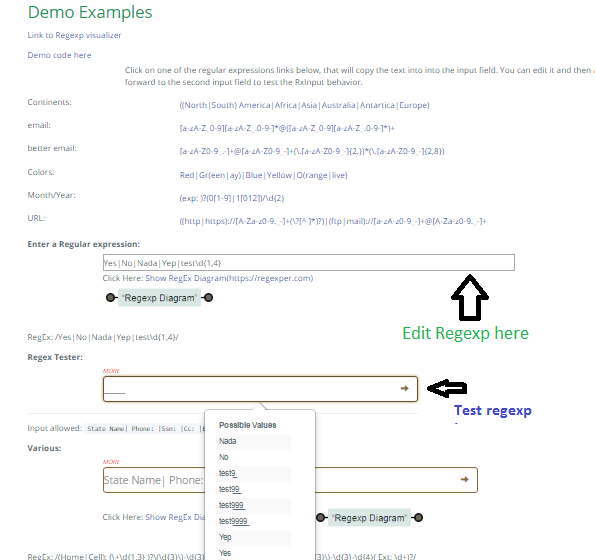](https://nurulc.github.io/) | ||
[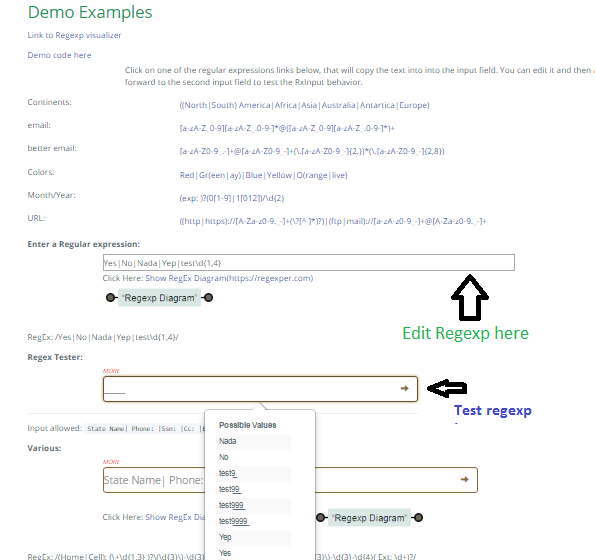](https://nurulc.github.io/rxinput.html) | ||
@@ -59,7 +77,7 @@ ## Introduction | ||
I needed a regular expression matcher that would work incrementally; By that I mean that it should let you know if a string matches the beginning part of a regular expression (good so far, but needs more input scenario). I tried to figure out if that was possible using JavaScript's regular expression matcher. I could not figure out any easy to do that. I decided that I would write an incremental regular expression matcher. I was much more difficult that I expected. But I have build an npm package that does perform incremental regular expression matching. | ||
I needed a regular expression matcher that would work incrementally; by that I mean that it should let you know if a string matches the beginning part of a regular expression (good so far, but needs more input scenario). I tried to figure out if that was possible using JavaScript's regular expression matcher. I could not figure out any easy to do that. I decided that I would write an incremental regular expression matcher. I was much more difficult that I expected. But I have build an npm package that does perform incremental regular expression matching. | ||
- npm [incr-regex-package](https://github.com/nurulc/incr-regex-package) | ||
The widget was inspired by another github project (https://github.com/insin/react-maskinput) that provides mask input for things like phone number, credit card number, date and so on. Although the capability is very nice, but it was limited. THe input mask you could enter has very little flexibility, wile a regular expression has all the flexibility you could need (even regexp has its limitations, cannot match recursive patterns, but that is for another day). | ||
The widget was inspired by another github project (https://github.com/insin/react-maskedinput) that provides mask input for things like phone number, credit card number, date and so on. Although the capability is very nice, but it was limited. THe input mask you could enter has very little flexibility, wile a regular expression has all the flexibility you could need (even regexp has its limitations, cannot match recursive patterns, but that is for another day). | ||
@@ -74,3 +92,3 @@ While building the widget it became obvious that it could be a swiss army knife and provide the following (so I implemented them): | ||
- Force upper case | ||
- Limit the character you can enter | ||
- Limit the character you can enter based on matching the regular expression | ||
- Only allow valid input as you type | ||
@@ -80,19 +98,22 @@ | ||
- Does not support look back | ||
- Does not fupport look forward | ||
- Is not a replacement JavaScript RegExp | ||
- Not sure of all RegExp edge cases are handles | ||
- Assome all reagep are anchored to the beging of input (meaning the regexp matching always starts at the begining | ||
- Currently only works as React component, requiring **bootstrap 3** and also **react-boorstrap** | ||
- Working on lifting the limitation and offering jQuery plugin (i have never built a jquery plugin, so it might take a little time). Further I would like to offer a version that does not depend on any external js library. | ||
* Currently only works as React component, requiring **bootstrap 3** and also **react-bootstrap** | ||
* Working on lifting the limitation and offering jQuery plugin (i have never built a jquery plugin, so it might take a little time). * Further I would like to offer a version that does not depend on any external js library. | ||
* __RegExp__ _(incr-regex-package)_ | ||
* Does not support look back | ||
* Does not support look forward | ||
* Is not a replacement JavaScript RegExp | ||
* Tries to have test cases for quite a lot of regexp variotions, but cannot be sure of all RegExp edge cases are handles, use the demo app to try out your regexp. | ||
* Assumes all reagep are anchored to the beging of input (meaning the regexp matching always starts at the begining | ||
## Known bugs | ||
- Rare: but deleting input text in the middle of the input box (very complex regular expressions) sometimes misbehaves. The algorithm for handling this is rather complex. I am trying to figure out how to imp-lement the capability more elegantly. | ||
* Rare: but deleting input text in the middle of the input box (very complex regular expressions) sometimes misbehaves. | ||
* The algorithm for handling this is rather complex. I am trying to figure out how to imp-lement the capability more elegantly. | ||
### Installation | ||
``` | ||
npm install react-rxinput --save | ||
``` | ||
git: | ||
@@ -99,0 +120,0 @@ |
/*! | ||
* react-rxinput 1.0.4 | ||
* react-rxinput v1.1.0 - https://nurulc.github..io | ||
* ISC Licensed | ||
*/ | ||
!function(t,e){"object"==typeof exports&&"object"==typeof module?module.exports=e(require("react"),require("react-bootstrap")):"function"==typeof define&&define.amd?define(["react","react-bootstrap"],e):"object"==typeof exports?exports.RxInput=e(require("react"),require("react-bootstrap")):t.RxInput=e(t.React,t.ReactBootstrap)}(this,function(t,e){return function(t){function e(r){if(n[r])return n[r].exports;var i=n[r]={exports:{},id:r,loaded:!1};return t[r].call(i.exports,i,i.exports,e),i.loaded=!0,i.exports}var n={};return e.m=t,e.c=n,e.p="",e(0)}([function(t,e,n){"use strict";function r(t){return t&&t.__esModule?t:{"default":t}}function i(t,e){var n={};for(var r in t)e.indexOf(r)>=0||Object.prototype.hasOwnProperty.call(t,r)&&(n[r]=t[r]);return n}function a(t){return(t.ctrlKey||t.metaKey)&&t.keyCode===(t.shiftKey?g:m)}function o(t){return(t.ctrlKey||t.metaKey)&&t.keyCode===(t.shiftKey?m:g)}function s(t,e){return t===e||void 0!==t&&void 0!==e&&(t.start===e.start&&t.end===e.end)}function u(t,e){var n=t.toUpperCase(),r=e.toUpperCase();return n<r?-1:n>r?1:0}var c=Object.assign||function(t){for(var e=1;e<arguments.length;e++){var n=arguments[e];for(var r in n)Object.prototype.hasOwnProperty.call(n,r)&&(t[r]=n[r])}return t},l=n(19),h=r(l),f=n(6),p=r(f),d=n(20),v=n(16),y=n(12),m=90,g=89,_={DONE:[h.default.createElement("span",{className:"glyphicon glyphicon-ok form-control-feedback"}),""],MORE:[h.default.createElement("span",{className:"glyphicon glyphicon-arrow-right form-control-feedback"})," has-warning"],OK:[h.default.createElement("span",{className:"glyphicon glyphicon-option-horizontal form-control-feedback"}),""]},k=function(t){return t},E=h.default.createClass({displayName:"RxInput",propTypes:{mask:h.default.PropTypes.object.isRequired,name:h.default.PropTypes.string.isRequired,popover:h.default.PropTypes.string,selection:h.default.PropTypes.object,value:h.default.PropTypes.string},getDefaultProps:function(){return{value:""}},getInitialState:function(){var t={pattern:this.props.mask,value:this.props.value};return{focus:!1,value:this.props.value,selection:this.props.selection,mask:new y.RXInputMask(t)}},componentWillReceiveProps:function(t){this.props.mask.toString()!==t.mask.toString()?(this.state.mask.setPattern(t.mask,{value:t.value,selection:this.state.mask.selection}),this.setState({selection:this.state.selection,value:t.value})):this.props.value!==t.value&&this.state.mask.setValue(t.value)},_updateMaskSelection:function(){this.state.mask.selection=(0,v.getSelection)(this.input)},_updateInputSelection:function(){s((0,v.getSelection)(this.input),this.state.mask.selection)||(0,v.setSelection)(this.input,this.state.mask.selection)},_onFocus:function(t){},_onBlurr:function(){},_onChange:function(t){var e=this.state.mask,n=e.getValue();if(t.target.value!==n){if(t.target.value.length<n.length){var r=n.length-t.target.value.length;this._updateMaskSelection(),e.selection.end=e.selection.start+r,e.backspace()}var i=this._getDisplayValue();t.target.value=i,i&&this._updateInputSelection()}if(this.setState({selection:this.mask.selection}),this.props.onChange){var a={target:{value:this.getValue()}};this.props.onChange(a)}},_onKeyDown:function(t){var e=this,n=this.state.mask,r=function(t){return function(e){return e.key===t}},i=function(r,i){if(!r(t))return!1;if(t.preventDefault(),e._updateMaskSelection(),i()){var a=t.target.value,o=e._getDisplayValue();if(t.target.value=o,o&&e._updateInputSelection(),e.props.onChange&&a!=o){var s={target:{value:n._getValue()}};e.props.onChange(s)}}return e.setState({selection:n.selection}),!0};if(!(i(a,function(){return n.undo()})||i(o,function(){return n.redo()})||i(r("Backspace"),function(){return n.backspace()})||i(r("Delete"),function(){return n.del()})||t.metaKey||t.altKey||t.ctrlKey||t.shiftKey||"Enter"===t.key||"ArrowLeft"!==t.key&&"ArrowRight"!=t.key)){var s=(0,v.getSelection)(this.input);s.start===s.end&&void 0!==n.left&&(t.preventDefault(),"ArrowLeft"===t.key?n.left(s):n.right(s),this._updateInputSelection())}},_onKeyPress:function(t){function e(t){return!!n.input(t)||(t!==t.toUpperCase()?n.input(t.toUpperCase()):t!=t.toLowerCase()&&n.input(t.toLowerCase()))}var n=this.state.mask;if(!(t.metaKey||t.altKey||t.ctrlKey||"Enter"===t.key)){(0,v.getSelection)(this.input),n.getSelection();if(t.preventDefault(),this._updateMaskSelection(),e(t.key)){var r=t.target.value,i=n.getValue();if(t.target.value=i,this._updateInputSelection(),this.setState({selection:n.selection}),this.props.onChange&&r!=i){var a={target:{value:n._getValue()}};this.props.onChange(a)}}}},_onPaste:function(t){var e=this.state.mask;t.preventDefault(),this._updateMaskSelection(),e.paste(t.clipboardData.getData("Text"))&&(t.target.value=e.getValue(),setTimeout(this._updateInputSelection,0),this.setState({selection:e.selection}))},_getMaskList:function(t){var e=this.state.mask.minCharsList(!!t);return e&&e.length<20?e:this.state.mask.minCharsList()},_getDisplayValue:function(){var t=this.state.mask.getValue();return t===this.state.mask.emptyValue?"":t},selected:function(t,e){if(!t.split("").find(function(t){return y.isMeta(t)?t:void 0})){var n=this.state.mask;n.setValue(t),this.setState({mask:n})}},_createPopover:function(t,e,n){function r(t){for(var e=5381,n=t.length;n;)e=33*e^t.charCodeAt(--n);return(e>>>0)+12}var i=this,a=function(t){return k(t.replace(/\u0332/g,""),"strip:")};n=Math.max(150,n||12*Math.max.apply(null,t.map(function(t){return Math.min(25,a(t).length)}))),k(n,"MAX WIDTH:");var o=n||200,s={width:o-50,maxWidth:o-50},c=void 0,l=void 0;if(!t||t.length<=1)return h.default.createElement("div",null);t.length>20&&(c={height:"400px",display:"block",overflow:"auto"},l=h.default.createElement("div",null," "));var f=this;return h.default.createElement(d.Popover,{id:this.props.name+"myPopover",className:"col-xs-10 col-md-10",style:{width:o,maxWidth:o,fontSize:"10px",marginTop:"10px",marginBottom:"10px"}},h.default.createElement("table",{key:this.props.name+"myPopover1",className:"table-responsive table-striped table-hover table-condensed col-xs-10 col-md-10",style:s},h.default.createElement("thead",null,h.default.createElement("tr",null,e.map(function(t){return h.default.createElement("th",{key:i.props.name+t},t)}))),h.default.createElement("tbody",{style:c},t.sort(u).map(function(t){return h.default.createElement("tr",{onClick:function(e){return f.selected(t,e)},key:i.props.name+"L"+r(t)},h.default.createElement("td",{onClick:function(e){return f.selected(t,e)}},t))}))),l)},render:function(){var t=this,e=this.props,n=(e.mask,e.size),r=e.placeholder,a=(i(e,["mask","size","placeholder"]),(0,p.default)(this.props,["popover","mask","selection"])),o=void 0,s=this.state.mask.pattern,u=s.length,l=this.props.popover?this._createPopover(this._getMaskList(),["Possible Values"]):h.default.createElement("span",null),f=this.state.mask.isDone();f&&(o=_[this.state.mask.isDone()]);var v={marginBotton:"0px",fontSize:"70%",color:"red",fontStyle:"italic"},y=h.default.createElement("div",{style:{marginBotton:"0px",paddingLeft:"100px"}},h.default.createElement("div",{style:v},f," "),h.default.createElement("div",{className:"form-group has-feedback"+o[1]},h.default.createElement(d.OverlayTrigger,{trigger:"focus",style:{marginBotton:"0px"},ref:"mypop",placement:"bottom",overlay:l},h.default.createElement("input",c({},a,{className:"form-control",ref:function(e){return t.input=e},maxLength:u,onChange:this._onChange,onKeyDown:this._onKeyDown,onKeyPress:this._onKeyPress,onPaste:this._onPaste,placeholder:r||this.state.mask.emptyValue,size:n||u,value:this._getDisplayValue(),style:{padding:"3px 0px 3px 0px"}}))),o[0]));return y}});t.exports=E},function(t,e){"use strict";function n(t,e){if(!(t instanceof e))throw new TypeError("Cannot call a class as a function")}function r(t){if(!t)return t;for(var e=1,n=arguments.length;e<n;e++){var r=arguments[e];if("function"==typeof r||"object"===("undefined"==typeof r?"undefined":z(r))&&null!==r)for(var i,a=-1,o=Object.keys(r),s=o?o.length:0;++a<s;)i=o[a],t[i]=r[i]}return t}function i(t){return r({},t)}function o(t,e){return!!t[e]}function s(t,e){var n,i=this;if("function"!=typeof i)throw new Error("Parent must be a constructor function");return n=o(t,"constructor")?t.constructor:function(){return i.apply(this,arguments)},r(n,i,e),n.prototype=Object.create(i.prototype),t&&r(n.prototype,t),n.prototype.constructor=n,n}function u(t){return t}function c(t){var e=[];return e.concat.apply(e,t)}function l(t,e){if(t===e)return!0;if(!t||!e)return!1;if(e.length!==t.length)return!1;for(var n=0,r=e.length;n<r;n++)if(e[n]instanceof Array&&t[n]instanceof Array){if(!l(t[n],e[n]))return!1}else if(e[n]!==t[n])return!1;return!0}function h(t){for(var e={},n=[],r=0;r<t.length;r++)t[r]in e||(n.push(t[r]),e[t[r]]=!0);return n}function f(t,e){for(var n=0;n<e.length;n++)if(t(e[n]))return e[n]}function p(t,e,n){if(!t||!e)return!1;var r=t.length,i=e.length;if(n+i>r)return!1;for(var a=n,o=0;o<i;a++,o++)if(e[o]instanceof Array&&t[a]instanceof Array){if(!p(t[a],e[o],0))return!1}else if(t[a]!==e[o])return!1;return!0}function d(t,e){for(var n=0;n<e.length;n++)t.push(e[n]);return t}function v(t){for(var e=[],n=0,r=t.length;n<=r;n++)e.push(t.charAt(r-n));return e.join("")}function y(t,e){for(var n=0;n<t.length&&n<e.length;n++)if(t.charAt(n)!==e.charAt(n))return t.substring(0,n);return t.length<e.length?t:e}function m(t,e){return v(y(v(t),v(e)))}function g(t,e){return t.length<e?t:t.substr(0,e)}function _(t,e){return t.length<e?t:t.substr(t.length-e,e)}function k(t,e){function n(t,e,n){for(var r=t.length-n,i=0;i<n;r++,i++)if(t.charAt(r)!==e.charAt(i))return!1;return!0}for(var r=Math.min(t.length,e.length),i=r;i>0;i--)if(n(t,e,i))return t.substr(0,t.length-i)+e;return t+e}function E(){function t(t){return t.split("").map(function(t){return"\\"+t}).join("|")}function e(t){return t.split("").join("|")}var n="\\[(?:\\\\u|\\\\\\]|\\\\\\\\|(\\\\)?\\[|[^\\]\\[\\\\])*?\\]",r=t(".|+*?()^$"),i="\\(\\?:|\\?\\?|\\*\\?|\\+\\?",a=e("dDsSbBwW"),o=t("[]{}\\"),s=[r,a,o].join("|"),u="\\\\(?:"+s+")",c="\\{(?:\\d+,\\d+|\\d+|\\d+,|,\\d+)\\}",l="[^.+?{}\\]\\[|()\\\\]";return[n,c,u,i,r,l].map(function(t){return"(?:"+t+")"}).join("|")}function O(){return new RegExp(E(),"g")}function b(t){var e=t.match(/\{(\d+)(,(\d*))?\}/),n=Number(e[1]);return{min:Number(e[1]),max:e[3]?Number(e[3]):e[2]?void 0:n}}function x(t){return(1&+t)>0}function S(t,e){return void 0===t?e:(t.push(e),t)}function w(t,e,n){return new B(t,e)}function R(t){if(!t)throw Error("n_head of empty list");return t.head}function I(t){if(!t)throw Error("n_tail of empty list");return t.tail}function A(t){var e=arguments.length<=1||void 0===arguments[1]?null:arguments[1];return t?A(I(t),w(R(t),e)):e}function N(t){return A(t.reduce(function(t,e){return w(e,t)},null))}function M(t){return N(t.split(""))}function C(t){return V(function(t,e){return t.push(e),t},t,[])}function D(t){return C(t).join("")}function P(t,e){return t&&t?w(R(t),P(I(t),e)):e}function L(t,e,n){return n=n||0,e?w(t(R(e),n,e),L(t,I(e),n+1)):e}function T(t,e,n){return e?(n=n||0,t(R(e),n,e)?w(R(e),T(t,I(e),n+1)):T(t,I(e),n+1)):e}function j(t,e,n){return n=n||0,e?t(R(e),n,e)?e:j(t,I(e),n+1):null}function V(t,e,n){return e?V(t,I(e),t(n,R(e))):n}function K(t,e,n){return 2===arguments.length&&(n=e,e=t,t=function(t,e){return t===e}),T(function(e){return!j(function(n){return t(n,e)},n)},e)}Object.defineProperty(e,"__esModule",{value:!0});var F=function(){function t(t,e){for(var n=0;n<e.length;n++){var r=e[n];r.enumerable=r.enumerable||!1,r.configurable=!0,"value"in r&&(r.writable=!0),Object.defineProperty(t,r.key,r)}}return function(e,n,r){return n&&t(e.prototype,n),r&&t(e,r),e}}(),z="function"==typeof Symbol&&"symbol"==typeof Symbol.iterator?function(t){return typeof t}:function(t){return t&&"function"==typeof Symbol&&t.constructor===Symbol?"symbol":typeof t};e.assign=r,e.copy=i,e.extend=s,e.ID=u,e.flatten=c,e.array_eq=l,e.arr_uniq=h,e.arr_find=f,e.array_match=p,e.array_append=d,e.sreverse=v,e.sprefix=y,e.rprefix=m,e.shead=g,e.stail=_,e.sRightMerge=k,e.parseMulti=b,e.odd=x,e.arr_push=S,e.n_cons=w,e.n_head=R,e.n_tail=I,e.n_reverse=A,e.arrayToList=N,e.stringToList=M,e.listToArray=C,e.listToString=D,e.n_concat=P,e.n_map=L,e.n_filter=T,e.n_find=j,e.n_reduce=V,e.n_removeAll=K;e.contract=function(){var t=Function.prototype.call,e=(t.bind([].slice),t.bind({}.toString)),n=function(t){return"undefined"==typeof t},r=function(t){var n="[object "+t+"]";return function(t){return e(t)==n}},i=function(t){var n="[object "+t+"]";return function(r){if(e(r)!==n)throw new TypeError("Expected "+t);return r}},a=r("Array"),o=i("Array"),s=function(t){return function(e){return("undefined"==typeof e?"undefined":z(e))==t}},u=function(t){return function(e){if(("undefined"==typeof e?"undefined":z(e))!==t)throw new TypeError("Expected a"+("object"===t?"n":"")+t+".");return e}},c=u("function"),l=s("function"),h=function(t){return function(e){if(!(e instanceof t))throw new TypeError("Expected an instance of "+t);return e}},f=function(t){if((0|t)!==t)throw new TypeError("Expected a 32-bit natural.");return t},p=function(t){if((0|t)!==t||t<0)throw new TypeError("Expected a 32-bit natural.");return t};return{int32:f,nat32:p,func:c,isFunc:l,typeOf:u,isTypeOf:s,arr:o,isArr:a,classOf:i,isClassOf:r,instanceOf:h,isUndef:n}}();"function"!=typeof Object.assign&&!function(){Object.assign=function(t){if(void 0===t||null===t)throw new TypeError("Cannot convert undefined or null to object");for(var e=Object(t),n=1;n<arguments.length;n++){var r=arguments[n];if(void 0!==r&&null!==r)for(var i in r)r.hasOwnProperty(i)&&(e[i]=r[i])}return e}}();var B=(e.TOKINIZATION_RX=O(),e.StackDedup=function(){function t(e){n(this,t),this.length=0,this.data=[],e&&this.push(e),this.maxLen=0}return F(t,[{key:"forEach",value:function(t){for(var e=this.data,n=0;n<this.length;n++)t(e[n],n,this);return this}},{key:"reduce",value:function(t,e){for(var n=this.data,r=0;r<this.length;r++)e=t(e,n[r],r,this);return e}},{key:"filter",value:function(e){var n=new t,r=this.data;n.maxLen=this.maxLen;for(var i=0;i<this.length;i++)e(r[i],i,this)&&n.push(r[i]);return n}},{key:"map",value:function(e){var n=new t,r=this.data;n.maxLen=this.maxLen;for(var i=0;i<this.length;i++)n.push(e(r[i],i,this));return n}},{key:"toArray",value:function(){return this.reduce(S,[])}},{key:"reset",value:function(){return this.length=0,this.maxLen=0,this}},{key:"push",value:function(t){if(void 0===t)return this;for(var e=this.data,n=this.length,r=0;r<n;r++)if(e[r]===t)return this;return e[this.length++]=t,this.length>this.maxLen&&(this.maxLen=this.length),this}},{key:"pop",value:function(){return this.length<=0?this:(length--,this)}},{key:"top",value:function(){if(!(this.length<=0))return data[this.length-1]}},{key:"addAll",value:function(t){var e=this;return t?(t.forEach(function(t){return e.push(t)}),this):this}}]),t}(),function(){function t(e,r){n(this,t),this.head=e,this.tail=r}return F(t,[{key:"equals",value:function(t){return null!==t&&(this.head===t.head&&this.tail===t.tail)}},{key:"addAll",value:function(t){return t?A(a).reduce(function(t,e){return w(e,t)},this):this}},{key:"map",value:function(t){return L(t,this)}},{key:"reduce",value:function(t,e){return V(t,this,e)}},{key:"reverse",value:function(t){return A(this,t)}}]),t}())},function(t,e,n){"use strict";function r(t,e){if(!(t instanceof e))throw new TypeError("Cannot call a class as a function")}function i(){}function a(){}function o(){}function s(){}function u(t){return t&&"U"==t.type&&"MULTIRANGE"==t.op}function c(t){return function(e){return[!(e===W||void 0!==e&&!e.match(t)),void 0]}}function l(t){return[t!==W,void 0]}function h(t){return function(e){return[!(e===W||void 0!==e&&e!==t),t]}}function f(t){return/[^a-zA-Z0-9]/.test(t||".")}function p(t,e){var n=f(t),r=f(e);return[n&&!r||!n&&r,void 0]}function d(){return!1}function v(t,e){if((m(t)||g(t))&&e)return t.nextNode=e,t;if(_(t)){var n=e?v(t.right,e):t.right;return v(t.left,n),t}if(k(t)&&e)return t.left===F?t.left=e:v(t.left,e),t.right===F?t.right=e:v(t.right,e),t;if(E(t)&&e)return v(t.left,e),t.nextNode=e,t;if(O(t)&&e){var r=t;return v(t.left,r),t.nextNode=e,t}return t}function y(t,e){var n=function(){return e||t.val};return t.toString=n,t}function m(t){return t&&!t.oper&&t.multi!==s&&t.match}function g(t){return t&&!t.oper&&t.multi===s&&t.match}function _(t){return t&&t.oper===Y}function k(t){return t&&t.oper===U}function E(t){return t&&t.oper===H}function O(t){return t&&t.oper===X}function b(t){return t&&"N"===t.type&&"ANY"===t.op}function x(t){return t&&"N"===t.type&&("CHARSET"===t.op||"SPECIAL-CHARSET"===t.op)}function S(t){return"<SKIP>"==t?F:"("==t||"(?:"==t?z:")"==t?B:"."==t?{type:"N",val:"(.)",multi:i,op:"ANY",match:l}:"[\\b]"==t?{type:"N",val:"\b",multi:a,op:"SINGLE",match:c(/[\b]/)}:"^"==t||"$"==t?{type:"N",val:t,multi:s,op:"BOUNDARY",match:d}:"\\b"==t||"\\B"==t?{type:"N",val:t,multi:s,op:"BOUNDARY",match:p}:/^\[.*\]$/.test(t)?{type:"N",val:t,multi:i,op:"CHARSET",match:c(new RegExp(t))}:"|"==t?U:/^[?+*]\??$/.test(t)?q[t.substring(0,1)]:/^\{[^}]*\}$/.test(t)?{type:"U",val:t,op:"MULTIRANGE",fn:(0,K.parseMulti)(t)}:/^\\[dDsSwW]$/.test(t)?{type:"N",val:t,multi:i,op:"SPECIAL-CHARSET",match:c($[t])}:/^\\[trn]$/.test(t)?{type:"N",val:J[t.substring(1,2)],multi:a,op:"NON-PRINTING",match:c("\\"+t.substring(1))}:/^\\[.?+*{}()$^\\:|\][]$/.test(t)?{type:"N",val:t.substring(1,2),multi:a,op:"SINGLE",match:h(t.substring(1))}:{type:"N",val:t,multi:a,op:"SINGLE",match:h(t)}}function w(t){if(void 0!==t){if(t===W)return W;if("N"===t.type&&void 0===t.oper)return{type:"N",val:t.val,multi:t.multi,op:t.op,match:t.match};if(t.left&&(_(t)||E(t)||O(t)||k(t)))return t.right?{oper:t.oper,left:w(t.left),right:w(t.right)}:{oper:t.oper,left:w(t.left)};throw new Error("Copy of an invalid node "+t)}}function R(t,e,n){return t===Y?I(e,n):t===U?A(e,n):n?{oper:t,left:e,right:n}:e}function I(t,e){return t===F?e:e===F?t:e?{oper:Y,left:t,right:e}:t}function A(t,e){return e?{oper:U,left:t,right:e}:t}function N(t){return{oper:X,left:t}}function M(t,e){return!(t<e)&&(t>e||(0,K.odd)(t))}function C(t,e){for(var n=0;n<Q.length;n++)if((0,K.array_match)(t,Q[n].match,e))return Q[n]}function D(t){for(var e=[],n=0,r=t.length;n<r;n++){var i=C(t,n);i?((0,K.array_append)(e,i.put),n+=i.match.length-1):e.push(t[n])}return e}function P(t){t&&("|"==t[t.length-1]&&(t=t.concat("<SKIP>")),"|"==t[0]&&(t=["<SKIP>"].concat(t)));for(var e=D(t);!(0,K.array_eq)(t,e);)t=e,e=D(t);return t}function L(t,e){return e&&t&&t.oper?"("+L(t)+")":t&&t.oper?"B"==t.oper.type?"."==t.oper.op?"("+L(t.left)+"."+L(t.right)+")":"("+L(t.left)+"|"+L(t.right)+")":"U"==t.oper.type?"("+L(t.left,!1)+t.oper.val+")":void 0:t===W?"<DONE>":t.val}function T(t,e){return e&&t&&t.oper?"("+T(t)+")":t&&t.oper?"B"==t.oper.type?"."==t.oper.op?"("+T(t.left)+"."+T(t.right)+")":"("+T(t.left)+"|"+T(t.right)+")":"U"==t.oper.type?"("+T(t.left,!1)+t.oper.val+")":void 0:t===W?"<DONE>":"'"+t.val+"'"}function j(t,e){return e&&t&&t.oper?"("+L(t)+")":t&&t.oper?"B"==t.oper.type?"."==t.oper.op?t.nextNode?"("+j(t.left)+"."+j(t.nextNode)+")":j(t.left):"("+j(t.left)+"|"+j(t.right)+")":"U"==t.oper.type?t.nextNode?"(("+L(t.left,!1)+t.oper.val+")."+j(t.nextNode)+")":"("+L(t.left,!1)+t.oper.val+")":void 0:t===W?"<DONE>":t&&t.nextNode?"("+t.val+"."+j(t.nextNode)+")":t?t.val:""}Object.defineProperty(e,"__esModule",{value:!0}),e.RxParser=e.RXTREE=e.FAILED=e.MAYBE=e.MORE=e.DONE=void 0;var V=function(){function t(t,e){for(var n=0;n<e.length;n++){var r=e[n];r.enumerable=r.enumerable||!1,r.configurable=!0,"value"in r&&(r.writable=!0),Object.defineProperty(t,r.key,r)}}return function(e,n,r){return n&&t(e.prototype,n),r&&t(e,r),e}}();e.MANY=i,e.TERM=a,e.PERHAPS_MORE=o,e.BOUNDARY=s,e.makeFSM=v,e.matchable=m,e.boundary=g,e.dot=_,e.or=k,e.zero_or_one=E,e.zero_or_more=O,e.anychar=b,e.charset=x,e.copyNode=w,e.printExpr=L,e.printExprQ=T,e.printExprN=j;var K=n(1);if(!K.array_append)throw new Error("array_append is undefined");var F=y({type:"N",val:"<SKIP>",multi:a,op:"SKIP",match:l}),z=(y({type:"N",val:"\b",multi:a,op:"SINGLE",match:c(/[\b]/)}),y({type:"L",val:")"})),B=y({type:"R",val:")"}),U=y({type:"B",val:"|",op:"OR"}),H=y({type:"U",val:"?",op:"MULTI"}),X=y({type:"U",val:"*",op:"MULTI"}),Z=y({type:"U",val:"+",op:"MULTI"}),Y=y({type:"B",val:".",op:"."}),G=function(){return!1},W=e.DONE=y({type:"N",val:"DONE",multi:s,op:"DONE",match:G}),q=(e.MORE=y({type:"N",val:"MORE",multi:s,op:"MORE",match:G}),e.MAYBE=y({type:"N",val:"MAYBE",multi:s,op:"MAYBE",match:G}),e.FAILED=y({type:"N",val:"FAILED",multi:s,op:"FAILED",match:G}),e.RXTREE={matchable:m,boundary:g,dot:_,or:k,zero_or_one:E,zero_or_more:O,OR:U,ZERO_OR_ONE:H,ZERO_OR_MORE:X,ONE_OR_MORE:Z,DOT:Y,FALSE:G},{"*":X,"+":Z,"?":H}),$={"\\d":/\d/,"\\D":/\D/,"\\s":/\s/,"\\S":/\S/,"\\w":/\w/,"\\W":/\W/},J={t:"\t",n:"\n",r:"\r"},Q=[{match:["(","|",")"],put:["(",")"]},{match:["<SKIP>","|","<SKIP>"],put:["<SKIP>"]},{match:["<SKIP>","<SKIP>"],put:["<SKIP>"]},{match:["(","<SKIP>",")"],put:["<SKIP>"]},{match:["<SKIP>","*"],put:["<SKIP>"]},{match:["<SKIP>","+"],put:["<SKIP>"]},{match:["(","|"],put:["(","<SKIP>","|"]},{match:["|",")"],put:["|","<SKIP>",")"]},{match:["|","|"],put:["|","<SKIP>","|"]},{match:["(",")"],put:["<SKIP>"]}];e.RxParser=function(){function t(){r(this,t),this.operand=[],this.operator=[],this.basePrec=0,this.wasOp=!0,this.lastop=void 0}return V(t,[{key:"toString",value:function(){return"{ operand: "+this.operand.map(L)+" operator: "+this.operator.map(function(t){return t.toString()})+" prec: "+this.BasePrec+" wasOp: "+this.wasOp+"}"}},{key:"opState",value:function(t,e,n){var r=function(t){return t?"OPERATOR":"OPERAND"};if(this.lastop=n,this.wasOp!=t)throw new Error("RegExp parsing expected: "+r(t)+" but was: "+r(this.wasOp));this.wasOp=e}},{key:"addToken",value:function(t){if(!t)return this.finishUp();var e=S(t);switch("N"!=e.type&&"L"!=e.type||this.wasOp||(this.pushOp(Y,this.basePrec+4),this.opState(!1,!0)),e.type){case"L":this.opState(!0,!0,z),this.basePrec+=10;break;case"R":if(this.opState(!1,!1,B),this.basePrec-=10,this.basePrec<0)throw Error("Syntax error "+this.basePrec);break;case"":case"B":this.pushOp(e,this.basePrec+2),this.opState(!1,!0,e);break;case"U":this.pushOp(e,this.basePrec+7),this.opState(!1,!1,e);break;case"N":this.operand.push(e),this.opState(!0,!1,e);break;default:throw Error("Syntax error")}return this}},{key:"pushOp",value:function(t,e){for(var n=this.topV()||{prec:-100};n&&M(n.prec,e);){var r,i;i=this.popOper(),n.op.type&&"B"!=n.op.type?u(n.op)?this.operand.push(this.applyMulti(n.op,i)):n.op===Z?this.oneOrMore(i):this.unaryOp(n.op,i):(r=this.popOper(),this.operand.push(R(n.op,r,i))),this.operator.pop(),n=this.topV()}e>=0&&this.operator.push({op:t,prec:e})}},{key:"finishUp",value:function(){return void 0===this.wasOp?this:(this.wasOp?this.pushOp(void 0,-1):(this.pushOp(Y,0),this.operand.push(W),this.pushOp(void 0,-1)),this.wasOp=void 0,this)}},{key:"val",value:function(){return 0===this.operand.length?void 0:this.operand[this.operand.length-1]}},{key:"topV",value:function(){return 0===this.operator.length?void 0:this.operator[this.operator.length-1]}},{key:"popOper",value:function(){return this.operand.pop()}},{key:"applyMulti",value:function(t,e){var n,r=t.fn.min,i=t.fn.max;if(g(e))throw new SyntaxError("repetition of boundary element: '"+e.val+"'' has no meaning");var a=function(t,e,r){if(0===r)return t;for(n=0;n<r;n++)e=w(e),t=t?I(t,e):e;return t||e};return 0===r?void 0===i?{oper:X,left:e}:a(void 0,{oper:H,left:e},i):void 0===i?a(a(void 0,e,r),{oper:X,left:e},1):a(a(e,e,r-1),{oper:H,left:w(e)},i-r)}},{key:"oneOrMore",value:function(t){if(g(t))throw new SyntaxError("repetition of boundary element: "+t.val+" has no meaning");this.operand.push(I(t,N(w(t))))}},{key:"unaryOp",value:function(t,e){if(g(e))throw new SyntaxError("modifier ("+t.val+") of boundary element: "+e.val+" has no meaning");this.operand.push({oper:t,left:e})}}],[{key:"parse",value:function(e){"string"!=typeof e&&(e=e.toString().replace(/\\\//g,"/").replace(/^\/|\/$/g,""));var n=P(e.match(K.TOKINIZATION_RX));n=n||[];var r=n.reduce(function(t,e){return t.addToken(e)},new t);return r.val()&&r.pushOp(Y,0),r.operand.push(W),r.pushOp(void 0,-1),v(r.val())}}]),t}()},function(t,e,n){"use strict";function r(t,e){if(!(t instanceof e))throw new TypeError("Cannot call a class as a function")}function i(t,e){return new M(t,e)}function a(t){return t.split("").map(function(t){return"*"===t?x:"?"===t?w:"_"===t?S:t}).join("")}function o(t){return t==S||s(t)}function s(t){return t==w||t==x}function u(t){return t===S}function c(t){for(var e=void 0,n=[],r=0;r<t.length;r++){var i=t.charAt(r);s(i)&&e===i||(e=i,n.push(i))}return n.join("")}function l(t,e){for(var n=0;n<t.length;n++)if(!e(t.charAt(n)))return t.substring(n+1,t.length);return t}function h(t,e){for(var n=(t.length<e.length?t.length:e.length,l((0,O.rprefix)(t,e),s)),r="",i=1,a=2,o=function(t){var e=0;return function(n){switch(n){case i:return e<t.length?t.charAt(e):void 0;case a:e++;break;default:return e<t.length}}},u=function(t,e){return t==e||s(t)&&s(e)},c=function(t,e){return t===x?t:e},h=o(t),f=o(e);h()&&f();){var p=h(i),d=f(i),v=s(p)||s(d);u(p,d)?(r+=c(p,d),h(a),f(a)):v?s(p)?(r+=p,h(a)):(r+=d,f(a)):(r+=S,h(a),f(a))}return(0,O.sRightMerge)(r,n)}function f(t){var e=t.charCodeAt(0);return e>=97&&e<=122}function p(t){return void 0!==t&&t.filter(function(t){return t===b.DONE}).length===t.length}function d(t,e,n,r,i){function a(s,u,c){if(!s)return t(u);if(c&&s===(0,O.n_head)(c))return a(s.nextNode,o(s,u),(0,O.n_tail)(c));if(s===b.DONE)return t(u);if((0,b.dot)(s))return a(s.left,u,c);if((0,b.or)(s)){var l=a(s.left,u,c),h=a(s.right,u,c);return n(l,h)}if((0,b.zero_or_one)(s))return r?r(s,u,a,c):a(s.nextNode,e(u,w),c);if((0,b.zero_or_more)(s))return r?r(s,u,a,c):a(s.nextNode,e(u,x),c);if((0,b.matchable)(s)){var f=(0,b.matchable)(s)(void 0),p=f[1]||(i?i(s,S):S);return a(s.nextNode,e(u,p),c)}return(0,b.boundary)(s)?a(s.nextNode,u,c):t(u)}var o=function(t,n){return(0,b.zero_or_more)(t)?e(n,x):n};return a}function v(t,e,n){function r(r,i){return r.reduce(function(n,r){return e(n,t(r,i))},n())}return r}function y(t,e){if(!t)return e;switch(t.val){case"[0-9]":case"\\d":return"9̲";case"[A-Za-z]":case"[a-zA-Z]":case"[a-z]":return"a̲";case"[A-Z]":return"A̲";case"[0-9A-Za-z]":case"[A-Z0-9a-z]":case"[A-Za-z0-9]":case"[0-9a-zA-Z]":case"[a-z0-9A-Z]":case"[a-zA-Z0-9]":return"z̲";default:return e}}function m(t){if(t&&t!==b.DONE){if((0,b.dot)(t))return m(t.left);if((0,b.matchable)(t)){var e=(0,b.matchable)(t)(void 0);return e[1]}if((0,b.or)(t)){var n=m(t.left),r=m(t.right);return n===r?n:void 0}(0,b.zero_or_one)(t)||(0,b.zero_or_more)(t)||(0,b.boundary)(t)}}function g(t){var e=t&&t.length>0?m(t.data[0]):void 0;if(void 0!==e)return t.reduce(function(t,e){return t===m(e)?t:void 0},e)}function _(t,e){return t===-1||e===-1?-1:t+e}function k(t){if(!t)return 0;if(t===b.DONE)return 0;if((0,b.dot)(t))return _(k(t.left),k(t.right));if((0,b.or)(t)){var e=k(t.left);return e>=0&&e===k(t.right)?e:-1}if((0,b.zero_or_one)(t)||(0,b.zero_or_more)(t))return-1;if((0,b.matchable)(t)){var n=(0,b.matchable)(t)(void 0);return n[1]}return(0,b.boundary)(t)?k(t.left):0}Object.defineProperty(e,"__esModule",{value:!0}),e.IREGEX=e.HOLDER_ZERO_OR_ONE=e.HOLDER_ANY=e.HOLDER_ZERO_OR_MORE=void 0;var E=function(){function t(t,e){for(var n=0;n<e.length;n++){var r=e[n];r.enumerable=r.enumerable||!1,r.configurable=!0,"value"in r&&(r.writable=!0),Object.defineProperty(t,r.key,r)}}return function(e,n,r){return n&&t(e.prototype,n),r&&t(e,r),e}}();e.incrRegEx=i,e.convertMask=a,e.isMeta=o,e.isOptional=s,e.isHolder=u,e.__isDoneN=p,e.makeRxInfo=d;var O=n(1),b=n(2),x=e.HOLDER_ZERO_OR_MORE="⋯",S=e.HOLDER_ANY="_",w=e.HOLDER_ZERO_OR_ONE="◑",R=function(t,e){var n=function(t){return void 0===t?[]:[t]},r=function(t,e){return t+e},i=function(t,e){return(0,O.flatten)([t,e])},a=function(t,e){return(0,O.flatten)((0,O.arr_push)(t,e))},o=function(t,e,n,r){if(t.left){var a=n(t.nextNode,e,r),o=n(t.left,e,(0,O.n_cons)(t,r));return i(a,o)}},s=e?o:void 0;return v(d(n,r,i,s,t),a,n)},I=R(y,!0),A=R(y,!1),N=function(){var t=function(t){return t},e=function(t,e){return t+e},n=function(t,e){return h(t,e)},r=function(t,e){return h(t||e,e)},i=v(d(t,e,n),r,t);return function(t){return c(i(t,""))}}(),M=e.IREGEX=function(){function t(e,n){r(this,t);var i=30;e||n?(!n&&e&&(n=b.RxParser.parse(e)),this.str=e,this.base=n,this.tracker=[],this.current=new O.StackDedup(n),this.one=this.current,this.two=new O.StackDedup,this.lastCh=void 0,this.maxLen=0,this.mask=void 0,this._len=30):(this.str="",this.base=void 0,this.tracker=void 0,this.current=void 0,this.one=this.current,this.two=void 0,this.lastCh=void 0,this.maxLen=0,this._mask=void 0,i=k(this.base),i<=0&&(i=30),this._len=i)}return E(t,[{key:"toString",value:function(){return this.str}},{key:"getInputLength",value:function(){return this.tracker.length}},{key:"isDone",value:function(t){return(t>=this.tracker.length||void 0===t)&&this.state()===b.DONE}},{key:"getTree",value:function(){return this.base}},{key:"minChars",value:function(){return this._mask=N(this.current),this._mask}},{key:"minCharsList",value:function(t){var e=t?I:A;return(0,O.arr_uniq)(e(this.current,this.inputStr()))}},{key:"match",value:function(t){var e=g(this.current),n=this.test(t===S?void 0:t);return void 0===n&&t&&f(t)&&(n=this.test(t.toUpperCase()),t=t.toUpperCase()),this._update(n,t,e)}},{key:"matchStr",value:function(t){var e=t.length,n=!0,r=0,i=0;for(i=0;i<e;i++){var a=t[i];if(!this.match(a)){n=!1;break}this.lastCh=a,r++}return[n,r,t.substring(0,i)]}},{key:"state",value:function(){return this._state=this._state||this._stateCompute(),this._state}},{key:"stateStr",value:function(){var t=this.state();return t===b.MORE?"MORE":t===b.MAYBE?"OK":"DONE"}},{key:"inputStr",value:function(){return this.tracker.map(function(t){return t[0]}).join("")}},{key:"fixed",value:function(){return g(this.current)}},{key:"getCurrentStates",value:function(){return this.current.map(O.ID)}},{key:"reset",value:function(){return this.tracker=[],this.current.reset(),this.current.push(this.base),this.lastCh=void 0,this._state=void 0,this._mask=void 0,this}},{key:"clone",value:function(){var t=i();return t.str=this.str,t.base=this.base,t.tracker=this.tracker.slice(0),t.one=this.one.map(O.ID),t.two=this.two.map(O.ID),t.current=this.current==this.one?t.one:t.two,t.lastCh=this.lastCh,t._state=this._state,t._mask=void 0,t._len=this.length,t}},{key:"getInputTracker",value:function(){return this.tracker.map(O.ID)}},{key:"_after",value:function(t,e){if(e){var n=this.tracker.filter(function(n,r){return r>=e&&(t||void 0===n[1])});return n.map(function(t){return t[0]}).join("")}var r=t?this.tracker:this.tracker.filter(function(t){return void 0===t[1]});return r.map(function(t){return t[0]}).join("")}},{key:"_getArr",value:function(){return this.current===this.one?this.two.reset():this.one.reset()}},{key:"action",value:function(t,e,n,r){if(t===b.DONE)return e===b.DONE?(n.push(b.DONE),b.DONE):b.FAILED;if((0,b.dot)(t))return this.action(t.left,e,n,r);if((0,b.or)(t)){var i=this.action(t.left,e,n,r),a=this.action(t.right,e,n,r);return this._result(i,a)}if((0,b.zero_or_one)(t)||(0,b.zero_or_more)(t)){var o=(0,b.boundary)(t.left)?b.DONE:this.action(t.left,e,n,!0),s=this.action(t.nextNode,e,n,r);return this._result(o,s)}if((0,b.matchable)(t)){var u=t.match(e);return u[0]&&n.push(t.nextNode),u[0]?t.nextNode===b.DONE?b.DONE:b.MORE:b.FAILED}if((0,b.boundary)(t)){if(r)return b.FAILED;var c=t.match(this.lastCh,e);return c[0]?(t=t.nextNode,c=this.action(t,e,n)):b.FAILED}return b.FAILED}},{key:"_result",value:function(t,e){return t===e?t:t===b.MORE||e===b.MORE?b.MORE:void 0}},{key:"test",value:function(t,e){var n=this;e=e||this.current;var r=b.FAILED,i=this._getArr();if(e.forEach(function(e){r=n._result(n.action(e,t,i),r)}),r!==b.FAILED&&0!==i.length)return i}},{key:"_update",value:function(t,e,n){return void 0!==t&&(this.tracker.push([void 0===e?S:e,n]),t.maxLen>this.maxLen&&(this.maxLen=t.maxLen), | ||
this.current=t,this.lastCh=e,this._state=void 0,this._mask=void 0),void 0!==t}},{key:"_stateCompute",value:function(){var t=this.test(void 0);if(void 0===t)return b.DONE;var e=this.test(b.DONE);return p(e)?b.MAYBE:b.MORE}},{key:"length",get:function(){return this._len}}]),t}()},function(t,e){"use strict";var n=!("undefined"==typeof window||!window.document||!window.document.createElement),r={canUseDOM:n,canUseWorkers:"undefined"!=typeof Worker,canUseEventListeners:n&&!(!window.addEventListener&&!window.attachEvent),canUseViewport:n&&!!window.screen,isInWorker:!n};t.exports=r},function(t,e,n){"use strict";function r(t,e){if(!(t instanceof e))throw new TypeError("Cannot call a class as a function")}Object.defineProperty(e,"__esModule",{value:!0}),e.RxMatcher=void 0;var i=function(){function t(t,e){for(var n=0;n<e.length;n++){var r=e[n];r.enumerable=r.enumerable||!1,r.configurable=!0,"value"in r&&(r.writable=!0),Object.defineProperty(t,r.key,r)}}return function(e,n,r){return n&&t(e.prototype,n),r&&t(e,r),e}}(),a=(n(1),n(3)),o=n(2);e.RxMatcher=function(){function t(e){r(this,t),this.matcher=e,this._lastEditableIndex=void 0}return i(t,[{key:"getFirstEditableAtOrAfter",value:function(t){for(var e=t,n=this.getInputTracker();e<n.length;e++)if(void 0===n[e][1])return e;var r=this.minChars();e=n.length;for(var i=0;i<r.length&&!(0,a.isMeta)(r.charAt(i));i++,e++);return e}},{key:"getFirstEditableAtOrBefore",value:function(t){var e=this.getInputTracker();for(t>=e.length&&(t=e.length-1);t>0;t--)if(void 0===e[t][1])return t;return 0}},{key:"getInputLength",value:function(){return this.matcher.getInputLength()}},{key:"setPos",value:function(t){if(void 0!==t){var e=this.getInputTracker(),n=e.map(function(t){return t[0]}).join("").substr(0,t);for(this.reset(),this.matchStr(n),e=this.getInputTracker();e.length<t&&void 0!==this.fixed();)if(!this.match(this.fixed())){t=this.getInputLength();break}this._resetCache()}return t}},{key:"toString",value:function(){return this.matcher.toString()}},{key:"after",value:function(t){return this.matcher._after(!0,t)}},{key:"valueWithMask",value:function(){return this.matcher.valueWithMask()}},{key:"rawValue",value:function(t){return this.matcher.rawValue(t)}},{key:"_after",value:function(t,e){return this.matcher._after(t,e)}},{key:"isDone",value:function(t){return this.matcher.isDone(t)}},{key:"setToFirstEditableAfter",value:function(t){return void 0===t&&(t=this.getInputLength()),this.setPos(this.getFirstEditableAtOrAfter(t))}},{key:"lastEditableIndex",value:function(){if(void 0===this._lastEditableIndex){var t=this.getInputTracker(),e=this.clone(),n=this.getFirstEditableAtOrAfter(t.length);e.setPos(n),e.state()===o.DONE&&(n=t.length),this._lastEditableIndex=n}return this._lastEditableIndex}},{key:"getTree",value:function(){return this.matcher.getTree()}},{key:"minChars",value:function(t){if(void 0===t)return this.matcher.minChars();var e=this.matcher.clone(),n=this.matcher._after(!0,0).substring(0,t);e.reset();var r=e.matchStr(n);if(!r[0])throw new Error("Unexpected error (matchStr failed) from "+e.constructor.name||"IREGEX");return e.minChars()}},{key:"minCharsList",value:function(t){return this.matcher.minCharsList(t)}},{key:"emptyAt",value:function(t){var e=this.getInputTracker();return t<e.length&&(0,a.isHolder)(e[t][0])}},{key:"match",value:function(t){return this._resetCache(),this.matcher.match(t)}},{key:"matchStr",value:function(t){return this._resetCache(),this.matcher.matchStr(t)}},{key:"state",value:function(){return this.matcher.state()}},{key:"fixed",value:function(){return this.matcher.fixed()}},{key:"reset",value:function(){return this.matcher.reset(),this._resetCache(),this}},{key:"clone",value:function(){return new t(this.matcher.clone())}},{key:"getInputTracker",value:function(){return this.matcher.tracker}},{key:"getFullTracker",value:function(){var t=this.getInputTracker(),e=this.matcher.minChars().map(function(t){return(0,a.isMeta)(t)?[t,void 0]:[t,t]});return[].concat(t,e)}},{key:"_resetCache",value:function(){this._lastEditableIndex=void 0}},{key:"stateStr",value:function(){return this.matcher.stateStr()}},{key:"length",get:function(){return this.matcher.length}}]),t}()},function(t,e,n){"use strict";function r(t){var e={},n=a.apply(i,o.call(arguments,1));for(var r in t)s(n,r)===-1&&(e[r]=t[r]);return e}var i=Array.prototype,a=i.concat,o=i.slice,s=n(14);t.exports=r},function(t,e,n){"use strict";function r(t,e){return!(!t||!e)&&(t===e||!i(t)&&(i(e)?r(t,e.parentNode):"contains"in t?t.contains(e):!!t.compareDocumentPosition&&!!(16&t.compareDocumentPosition(e))))}var i=n(11);t.exports=r},function(t,e){"use strict";function n(t){try{t.focus()}catch(e){}}t.exports=n},function(t,e){"use strict";function n(){if("undefined"==typeof document)return null;try{return document.activeElement||document.body}catch(t){return document.body}}t.exports=n},function(t,e){"use strict";function n(t){return!(!t||!("function"==typeof Node?t instanceof Node:"object"==typeof t&&"number"==typeof t.nodeType&&"string"==typeof t.nodeName))}t.exports=n},function(t,e,n){"use strict";function r(t){return i(t)&&3==t.nodeType}var i=n(10);t.exports=r},function(t,e,n){"use strict";Object.defineProperty(e,"__esModule",{value:!0}),e.isHolder=e.isOptional=e.isMeta=e.convertMask=e.IREGEX=e.zero_or_more=e.zero_or_one=e.or=e.dot=e.matchable=e.RxMatcher=e.contract=e.RXInputMask=e.RxParser=e.printExpr=e.incrRegEx=e.FAILED=e.MAYBE=e.MORE=e.DONE=void 0;var r=n(1),i=n(2),a=n(3),o=n(13),s=n(5);if(void 0===a.incrRegEx)throw new Error("incrRegEx not defined");if(void 0===o.RXInputMask)throw new Error("RXInputMask not defined");if(void 0===s.RxMatcher)throw new Error("RxMatcher not defined");e.DONE=i.DONE,e.MORE=i.MORE,e.MAYBE=i.MAYBE,e.FAILED=i.FAILED,e.incrRegEx=a.incrRegEx,e.printExpr=i.printExpr,e.RxParser=i.RxParser,e.RXInputMask=o.RXInputMask,e.contract=r.contract,e.RxMatcher=s.RxMatcher,e.matchable=i.matchable,e.dot=i.dot,e.or=i.or,e.zero_or_one=i.zero_or_one,e.zero_or_more=i.zero_or_more,e.IREGEX=a.IREGEX,e.convertMask=a.convertMask,e.isMeta=a.isMeta,e.isOptional=a.isOptional,e.isHolder=a.isHolder},function(t,e,n){"use strict";function r(t,e){if(!(t instanceof e))throw new TypeError("Cannot call a class as a function")}function i(t,e){return e=e||t,{start:Math.min(t,e),end:Math.max(t,e)}}function a(t,e,n){return o(i(t.start+e,t.end+(void 0===n?e:n)))}function o(t,e,n,r){n=n||function(t){return t},r=r||n;var i=function(t,e,n){return n(Math.max(Math.min(t,e.end),e.start))};return e?{start:i(t.start,e,r),end:i(t.end,e,n)}:t}function s(t){return!t}function u(t){return t?t.end-t.start:0}function c(t){return s(u(t))}function l(t,e){return t.start>e.start}function h(t,e){return t===e}function f(t){for(var e=t.minChars(),n=0;e.length>n&&!(0,k.isMeta)(e.charAt(0));n++){if(!t.match(e.charAt(0)))return n>0;e=t.minChars()}return n>0}function p(t,e){var n=t.minChars();if(e=!!e,e!==!0&&n.length>1&&(0,k.isOptional)(n.charAt(0))&&!(0,k.isMeta)(n.charAt(1))){if(t.match(n.charAt(1)))return!0}else if(n.length>0&&!(0,k.isMeta)(n.charAt(0)))return t.match(n.charAt(0));return!1}function d(t,e){if(t.match(e))return!0;for(var n=t.clone();p(t,!1);)if(t.match(e))return!0;return t.reset(),t.matchStr(y(n,!0,0)),!1}function v(t){for(var e=t.length-1;e>=0&&(0,k.isHolder)(t.charAt(e));e--);return t.substring(0,e+1)}function y(t,e,n){var r=t.getInputTracker();if(n){var i=r.filter(function(t,r){return r>=n&&(e||void 0===t[1])});return i.map(function(t){return t[0]}).join("")}var a=e?r:r.filter(function(t){return void 0===t[1]});return a.map(function(t){return t[0]}).join("")}Object.defineProperty(e,"__esModule",{value:!0}),e.RXInputMask=void 0;var m=function(){function t(t,e){var n=[],r=!0,i=!1,a=void 0;try{for(var o,s=t[Symbol.iterator]();!(r=(o=s.next()).done)&&(n.push(o.value),!e||n.length!==e);r=!0);}catch(u){i=!0,a=u}finally{try{!r&&s.return&&s.return()}finally{if(i)throw a}}return n}return function(e,n){if(Array.isArray(e))return e;if(Symbol.iterator in Object(e))return t(e,n);throw new TypeError("Invalid attempt to destructure non-iterable instance")}}(),g=function(){function t(t,e){for(var n=0;n<e.length;n++){var r=e[n];r.enumerable=r.enumerable||!1,r.configurable=!0,"value"in r&&(r.writable=!0),Object.defineProperty(t,r.key,r)}}return function(e,n,r){return n&&t(e.prototype,n),r&&t(e,r),e}}();e.trimHolder=v,e._after=y;var _=n(1),k=n(3),E=n(5),O=(n(2),e.RXInputMask=function(){function t(e){if(r(this,t),e=(0,_.assign)({pattern:null,selection:{start:0,end:0},value:"",history:{data:[],index:null,lastOp:null}},e),null===e.pattern)throw new Error("RXInputMask: you must provide a pattern.");this.setPattern(e.pattern,{value:e.value,selection:e.selection,history:e.history})}return g(t,[{key:"getState",value:function(){({pattern:this.pattern.clone(),selection:a(this.selection,0),value:this._getValue(),history:{data:[],index:null,lastOp:null}})}},{key:"input",value:function(t){if(c(this.selection)&&this.pattern.isDone(this.selection.start))return!1;var e=this._input(t,this.selection,this.pattern),n=m(e,3),r=n[0],i=n[1],a=n[2];if(!r)return!1;var o=this._getValue(),s=this.selection,u=this.pattern;return this._lastOp="input",this.selection=i,this.pattern=a,this.value=this.getValue(),null!==this._historyIndex&&(this._history.splice(this._historyIndex,this._history.length-this._historyIndex),this._historyIndex=null),("input"!==this._lastOp||!c(s)||null!==this._lastSelection&&s.start!==this._lastSelection.start)&&this._history.push({value:o,selection:s,lastOp:this._lastOp,pattern:u}),this._lastSelection=s,!0}},{key:"_input",value:function(t,e,n){c(e)&&n.emptyAt(e.start)&&(e=a(e,1));var r=n.clone();e=this._updateSelection(e,r.getFirstEditableAtOrAfter(e.start));var o=r.getFirstEditableAtOrAfter(e.end),s=y(r,!1,o),u=e.start;if(r.setPos(u),void 0!==t&&!d(r,t))return[!1,e,r];e=this._updateSelection(e,r.getInputLength()),o=Math.max(o,e.end);var l=r.getFirstEditableAtOrAfter(r.getInputLength()),h=this._insertRest(s,r,u,o+1);return f(h||r),[!0,i(l,l),h||r]}},{key:"_insertRest",value:function(t,e,n,r){function i(t,e,n,r,a){if(t>=e.length)return n;if(r>a||n.isDone())return n;var o=n.clone();if(d(o,e.charAt(t))){var s=i(t+1,e,o,o.getInputLength()-1,a);if(void 0!==s)return s}for(;p(n,!1););return n.match(void 0),i(t,e,n,r+1,a)}var a=i(0,t,e,n,r+t.length);return a}},{key:"backspace",value:function(){var t=this.pattern.getFirstEditableAtOrAfter(0);if(this.selection.start<t||this.selection.end<t)return!1;var e=(0,_.copy)(this.selection),n=this._getValue(),r=this.selection,a=r.start,o=r.end;if(a===o){if(a=this.pattern.getFirstEditableAtOrBefore(a-1),o=a+1,a<t)return}else if(a=this.pattern.getFirstEditableAtOrBefore(o<a?o:a),o===a&&o++,o<=t)return;var s=this._input(void 0,i(a,o),this.pattern),u=this.pattern;return!!s[0]&&(this.pattern=s[2],this.selection.start=this.selection.end=a,null!==this._historyIndex&&this._history.splice(this._historyIndex,this._history.length-this._historyIndex),("backspace"!==this._lastOp||e.start!==e.end||null!==this._lastSelection&&e.start!==this._lastSelection.start)&&this._history.push({value:n,selection:e,lastOp:this._lastOp,pattern:u}),this._lastOp="backspace",this._lastSelection=(0,_.copy)(e),!0)}},{key:"del",value:function(){return c(this.selection)?(this.right(this.selection),this.backspace()):this.backspace()}},{key:"paste",value:function(t){var e={value:this.value.slice(),selection:(0,_.copy)(this.selection),_lastOp:this._lastOp,_history:this._history.slice(),_historyIndex:this._historyIndex,_lastSelection:(0,_.copy)(this._lastSelection),pattern:this.pattern.clone()},n=this.getRawValueAt(this.selection.end);this.pattern.setPos(this.selection.start);var r=this._setValueFrom(this.selection.start,t);return this.selection.end=this.pattern.getInputLength(),!(!r||!this._setValueFrom(this.selection.end,n))||((0,_.assign)(this,e),!1)}},{key:"undo",value:function(){if(0===this._history.length||0===this._historyIndex)return!1;var t;if(null===this._historyIndex){this._historyIndex=this._history.length-1,t=this._history[this._historyIndex];var e=this._getValue();t.value===e&&t.selection.start===this.selection.start&&t.selection.end===this.selection.end||this._history.push({value:e,selection:(0,_.copy)(this.selection),lastOp:this._lastOp,startUndo:!0,pattern:this.pattern.clone()})}else t=this._history[--this._historyIndex];return this.pattern=t.pattern,this.setValue(t.value),this.selection=t.selection,this._lastOp=t.lastOp,!0}},{key:"redo",value:function(){if(0===this._history.length||null===this._historyIndex)return!1;var t=this._history[++this._historyIndex];return this._historyIndex===this._history.length-1&&(this._historyIndex=null,t.startUndo&&this._history.pop()),this.pattern=t.pattern.clone(),this.setValue(t.value),this.selection=t.selection,this._lastOp=t.lastOp,!0}},{key:"left",value:function(t){var e=(0,_.copy)(t);return e&&c(e)&&(e.start=e.end=t.start-1,this.setSelection(e)),this}},{key:"right",value:function(t){var e=(0,_.copy)(t);return e&&e.start===e.end&&(e.start=e.end=t.start+1,this.setSelection(e)),this}},{key:"setPattern",value:function(t,e){for(e=(0,_.assign)({value:""},e),this.pattern=new E.RxMatcher((0,k.incrRegEx)(t)),this.setValue(e.value),this.emptyValue=this.pattern.minChars(),this.setSelection(e.selection);this.skipFixed(!0););if(c(this.selection)&&0!==this.pattern.getInputTracker().length){var n=this._getValue();this.setValue(n),this.selection.start=this.selection.end=n.length}return this._resetHistory(),this}},{key:"select",value:function(t,e){return this.selection=e<t?i(e,t):i(t,e),this}},{key:"setSelection",value:function(t){var e=this,n=t===this.selection?this.selection:(0,_.copy)(t),r=this.selection||n,a=function(t){return e.pattern.getFirstEditableAtOrAfter(t)},s=function(t){return e.pattern.getFirstEditableAtOrBefore(t)};this.selection=r;var u=a(0),h=this.pattern.lastEditableIndex(),f=i(u,h);return c(n)?this.selection=o(n,f,l(this.selection,n)?s:a):this.selection=o(n,f,a,s),this}},{key:"_adjustSelection",value:function(t,e){return c(t)?i(e?this.pattern.getFirstEditableAtOrAfter(t.start):this.pattern.getFirstEditableAtOrBefore(t.start)):i(this.pattern.getFirstEditableAtOrBefore(t.start),this.pattern.getFirstEditableAtOrAfter(t.end))}},{key:"_setValueFrom",value:function(t,e){var n=this.pattern.clone(),r=!0;void 0!==t&&n.setPos(t);for(var i=t,a=0;a<e.length&&r;i++,a++){var o=e.charAt(a);r&=d(n,o)}return r&&(f(n),this.pattern=n),r}},{key:"setValue",value:function(t){var e=new O("RXInputMask:");if(this.getValue()===t)return!0;var n=this.pattern.clone();null===t&&(t=""),n.reset(),e.println("iterate over",t,"length:",t.length);for(var r=0;r<t.length;r++){var i=t.charAt(r);if(e.print("index: ",r,"char:",i,"minChars:",n.minChars(),"sameAsRest",h(t.substring(r),n.minChars()),"---"),h(t.substring(r),n.minChars()))break;if((0,k.isHolder)(i)&&(i=void 0),!d(n,i))return!1}return this.pattern=n,this.value=this.getValue(),!0}},{key:"minCharsList",value:function(t){return this.pattern.minCharsList(t)}},{key:"getSelection",value:function(){return(0,_.copy)(this.selection)}},{key:"_getValue",value:function(){return y(this.pattern,!0,0)}},{key:"getValue",value:function(){return y(this.pattern,!0,0)+this.pattern.minChars()}},{key:"getRawValue",value:function(){return y(this.pattern,!1,0)}},{key:"getRawValueAt",value:function(t){return y(this.pattern,!1,t)}},{key:"reset",value:function(){return this.pattern.reset(),this._resetHistory(),this.value=this.getValue(),this.selection.start=this.selection.end=0,this.setSelection(this.selection),this}},{key:"_resetHistory",value:function(){return this._history=[],this._historyIndex=null,this._lastOp=null,this._lastSelection=(0,_.copy)(this.selection),this}},{key:"_updateSelection",value:function(t,e){var n=(0,_.copy)(t);return n.start=e,e>n.end&&(n.end=e),n}},{key:"skipFixed",value:function(t){return p(this.pattern,t)}},{key:"isDone",value:function(){var t=this.pattern.clone(),e=this.getValue(),n=e.split("");if((0,_.arr_find)(function(t){return(0,k.isHolder)(t)},n))return"MORE";t.reset();for(var r=0;r<n.length;r++)if(!(0,k.isMeta)(n[r])&&!t.match(n[r]))return"MORE";return t.stateStr()}}]),t}(),function(){function t(e){r(this,t),this.content=e||""}return g(t,[{key:"print",value:function(){for(var t=arguments.length,e=Array(t),n=0;n<t;n++)e[n]=arguments[n];return this.content+=","+(e||[]).map(function(t){return JSON.stringify(t)}).join(" "),this}},{key:"println",value:function(){for(var t=arguments.length,e=Array(t),n=0;n<t;n++)e[n]=arguments[n];return this.print.apply(this,e),this.content+="\n",this}},{key:"flush",value:function(){console.log(this.content),this.content=""}}]),t}())},function(t,e){var n=[].indexOf;t.exports=function(t,e){if(n)return t.indexOf(e);for(var r=0;r<t.length;++r)if(t[r]===e)return r;return-1}},function(t,e,n){"use strict";function r(t,e,n,r){return t===n&&e===r}function i(t){var e=document.selection,n=e.createRange(),r=n.text.length,i=n.duplicate();i.moveToElementText(t),i.setEndPoint("EndToStart",n);var a=i.text.length,o=a+r;return{start:a,end:o}}function a(t){var e=window.getSelection&&window.getSelection();if(!e||0===e.rangeCount)return null;var n=e.anchorNode,i=e.anchorOffset,a=e.focusNode,o=e.focusOffset,s=e.getRangeAt(0);try{s.startContainer.nodeType,s.endContainer.nodeType}catch(u){return null}var c=r(e.anchorNode,e.anchorOffset,e.focusNode,e.focusOffset),l=c?0:s.toString().length,h=s.cloneRange();h.selectNodeContents(t),h.setEnd(s.startContainer,s.startOffset);var f=r(h.startContainer,h.startOffset,h.endContainer,h.endOffset),p=f?0:h.toString().length,d=p+l,v=document.createRange();v.setStart(n,i),v.setEnd(a,o);var y=v.collapsed;return{start:y?d:p,end:y?p:d}}function o(t,e){var n,r,i=document.selection.createRange().duplicate();void 0===e.end?(n=e.start,r=n):e.start>e.end?(n=e.end,r=e.start):(n=e.start,r=e.end),i.moveToElementText(t),i.moveStart("character",n),i.setEndPoint("EndToStart",i),i.moveEnd("character",r-n),i.select()}function s(t,e){if(window.getSelection){var n=window.getSelection(),r=t[l()].length,i=Math.min(e.start,r),a=void 0===e.end?i:Math.min(e.end,r);if(!n.extend&&i>a){var o=a;a=i,i=o}var s=c(t,i),u=c(t,a);if(s&&u){var h=document.createRange();h.setStart(s.node,s.offset),n.removeAllRanges(),i>a?(n.addRange(h),n.extend(u.node,u.offset)):(h.setEnd(u.node,u.offset),n.addRange(h))}}}var u=n(4),c=n(17),l=n(18),h=u.canUseDOM&&"selection"in document&&!("getSelection"in window),f={getOffsets:h?i:a,setOffsets:h?o:s};t.exports=f},function(t,e,n){"use strict";function r(t){return a(document.documentElement,t)}var i=n(15),a=n(7),o=n(8),s=n(9),u={hasSelectionCapabilities:function(t){var e=t&&t.nodeName&&t.nodeName.toLowerCase();return e&&("input"===e&&"text"===t.type||"textarea"===e||"true"===t.contentEditable)},getSelectionInformation:function(){var t=s();return{focusedElem:t,selectionRange:u.hasSelectionCapabilities(t)?u.getSelection(t):null}},restoreSelection:function(t){var e=s(),n=t.focusedElem,i=t.selectionRange;e!==n&&r(n)&&(u.hasSelectionCapabilities(n)&&u.setSelection(n,i),o(n))},getSelection:function(t){var e;if("selectionStart"in t)e={start:t.selectionStart,end:t.selectionEnd};else if(document.selection&&t.nodeName&&"input"===t.nodeName.toLowerCase()){var n=document.selection.createRange();n.parentElement()===t&&(e={start:-n.moveStart("character",-t.value.length),end:-n.moveEnd("character",-t.value.length)})}else e=i.getOffsets(t);return e||{start:0,end:0}},setSelection:function(t,e){var n=e.start,r=e.end;if(void 0===r&&(r=n),"selectionStart"in t)t.selectionStart=n,t.selectionEnd=Math.min(r,t.value.length);else if(document.selection&&t.nodeName&&"input"===t.nodeName.toLowerCase()){var a=t.createTextRange();a.collapse(!0),a.moveStart("character",n),a.moveEnd("character",r-n),a.select()}else i.setOffsets(t,e)}};t.exports=u},function(t,e){"use strict";function n(t){for(;t&&t.firstChild;)t=t.firstChild;return t}function r(t){for(;t;){if(t.nextSibling)return t.nextSibling;t=t.parentNode}}function i(t,e){for(var i=n(t),a=0,o=0;i;){if(3===i.nodeType){if(o=a+i.textContent.length,a<=e&&o>=e)return{node:i,offset:e-a};a=o}i=n(r(i))}}t.exports=i},function(t,e,n){"use strict";function r(){return!a&&i.canUseDOM&&(a="textContent"in document.documentElement?"textContent":"innerText"),a}var i=n(4),a=null;t.exports=r},function(e,n){e.exports=t},function(t,n){t.exports=e}])}); | ||
!function(t,e){"object"==typeof exports&&"object"==typeof module?module.exports=e(require("react"),require("incr-regex-package"),require("react-bootstrap")):"function"==typeof define&&define.amd?define(["react","incr-regex-package","react-bootstrap"],e):"object"==typeof exports?exports.RxInput=e(require("react"),require("incr-regex-package"),require("react-bootstrap")):t.RxInput=e(t.React,t.iRX,t.ReactBootstrap)}(window,function(t,e,n){return function(t){var e={};function n(r){if(e[r])return e[r].exports;var o=e[r]={i:r,l:!1,exports:{}};return t[r].call(o.exports,o,o.exports,n),o.l=!0,o.exports}return n.m=t,n.c=e,n.d=function(t,e,r){n.o(t,e)||Object.defineProperty(t,e,{enumerable:!0,get:r})},n.r=function(t){"undefined"!=typeof Symbol&&Symbol.toStringTag&&Object.defineProperty(t,Symbol.toStringTag,{value:"Module"}),Object.defineProperty(t,"__esModule",{value:!0})},n.t=function(t,e){if(1&e&&(t=n(t)),8&e)return t;if(4&e&&"object"==typeof t&&t&&t.__esModule)return t;var r=Object.create(null);if(n.r(r),Object.defineProperty(r,"default",{enumerable:!0,value:t}),2&e&&"string"!=typeof t)for(var o in t)n.d(r,o,function(e){return t[e]}.bind(null,o));return r},n.n=function(t){var e=t&&t.__esModule?function(){return t.default}:function(){return t};return n.d(e,"a",e),e},n.o=function(t,e){return Object.prototype.hasOwnProperty.call(t,e)},n.p="",n(n.s=3)}([function(e,n){e.exports=t},function(t,n){t.exports=e},function(t,e){t.exports=n},function(t,e,n){t.exports=n(4)},function(t,e,n){"use strict";n.r(e),n.d(e,"hashStr",function(){return g}),n.d(e,"RxInputBase",function(){return k}),n.d(e,"RxInput",function(){return _});var r=n(0),o=n.n(r),a=n(2),i=n(1),s=Object.assign||function(t){for(var e=1;e<arguments.length;e++){var n=arguments[e];for(var r in n)Object.prototype.hasOwnProperty.call(n,r)&&(t[r]=n[r])}return t};function u(t,e){if(!(t instanceof e))throw new TypeError("Cannot call a class as a function")}function l(t,e){if(!t)throw new ReferenceError("this hasn't been initialised - super() hasn't been called");return!e||"object"!=typeof e&&"function"!=typeof e?t:e}function c(t,e){if("function"!=typeof e&&null!==e)throw new TypeError("Super expression must either be null or a function, not "+typeof e);t.prototype=Object.create(e&&e.prototype,{constructor:{value:t,enumerable:!1,writable:!0,configurable:!0}}),e&&(Object.setPrototypeOf?Object.setPrototypeOf(t,e):t.__proto__=e)}var p=new RegExp(Object(i.convertMask)("[?*]")),f=90,h=89;function m(t){return(t.ctrlKey||t.metaKey)&&t.keyCode===(t.shiftKey?h:f)}function d(t){return(t.ctrlKey||t.metaKey)&&t.keyCode===(t.shiftKey?f:h)}function y(t){var e=void 0,n=void 0,r=void 0,o=void 0;if(void 0!==t.selectionStart)e=t.selectionStart,n=t.selectionEnd;else try{t.focus(),o=(r=t.createTextRange()).duplicate(),r.moveToBookmark(document.selection.createRange().getBookmark()),o.setEndPoint("EndToStart",r),n=(e=o.text.length)+r.text.length}catch(t){}return{start:e,end:n}}var v={DONE:[o.a.createElement("span",{className:"glyphicon glyphicon-ok form-control-feedback"}),""],MORE:[o.a.createElement("span",{className:"glyphicon glyphicon-arrow-right form-control-feedback"})," has-warning"],OK:[o.a.createElement("span",{className:"glyphicon glyphicon-option-horizontal form-control-feedback"}),""]};!function(t){function e(){return u(this,e),l(this,t.apply(this,arguments))}c(e,t),e.prototype.render=function(){var t=this.props.mask.pattern.getInputTracker()||[];return o.a.createElement("div",null,t.map(function(t){var e=t[0];return void 0===t[1]?e:o.a.createElement("b",null,e)}))}}(r.Component);function g(t){for(var e=5381,n=t.length;n;)e=33*e^t.charCodeAt(--n);return 12+(e>>>0)}var k=function(t){function e(n){u(this,e);var r=l(this,t.call(this,n));return r.state=r.getInitialState(),r._onChange=r._onChange.bind(r),r._onKeyDown=r._onKeyDown.bind(r),r._onKeyPress=r._onKeyPress.bind(r),r._onPaste=r._onPaste.bind(r),r._onFocus=r._onFocus.bind(r),r._onBlur=r._onBlur.bind(r),r.input=null,r}return c(e,t),e.prototype.getInitialState=function(){var t={pattern:this.props.mask||/.*/,value:this.props.value||""};return{focus:!1,value:this.props.value||"",selection:this.props.selection,mask:new i.RXInputMask(t)}},e.prototype.componentWillReceiveProps=function(t){this.props.mask.toString()!==t.mask.toString()?(this.state.mask.setPattern(t.mask,{value:t.value,selection:this.state.mask.selection}),this.setState({selection:this.state.selection,value:t.value})):this.props.value!==t.value&&this.state.mask.setValue(t.value)},e.prototype._updateMaskSelection=function(){this.state.mask.selection=y(this.input)},e.prototype._updateInputSelection=function(){(function(t,e){return t===e||void 0!==t&&void 0!==e&&t.start===e.start&&t.end===e.end})(y(this.input),this.state.mask.selection)||function(t,e){var n=void 0;try{void 0!==t.selectionStart?(t.focus(),t.setSelectionRange(e.start,e.end)):(t.focus(),(n=t.createTextRange()).collapse(!0),n.moveStart("character",e.start),n.moveEnd("character",e.end-e.start),n.select())}catch(t){}}(this.input,this.state.mask.selection)},e.prototype._onFocus=function(t){this.props.onFocus&&this.props.onFocus(t)},e.prototype._onBlur=function(t){this.fireChange(t)},e.prototype.fireChange=function(t){if(this.props.onChange){var e={value:this.state.mask._getValue(),target:t.target,name:this.props.name,mask:this.state.mask};this.props.onChange({target:e})}},e.prototype._onChange=function(t){var e=this.state.mask,n=e.getValue();if(t.target.value!==n){if(t.target.value.length<n.length){var r=n.length-t.target.value.length;this._updateMaskSelection(),e.selection.end=e.selection.start+r,e.backspace()}var o=this._getDisplayValue();t.target.value=o,o&&this._updateInputSelection()}this.setState({selection:this.mask.selection}),this.fireChange(t)},e.prototype._onKeyDown=function(t){var e=this,n=this.state.mask,r=function(t){return function(e){return e.key===t}},o=function(r,o){if(!r(t))return!1;if(t.preventDefault(),e._updateMaskSelection(),o()){var a=t.target.value,i=e._getDisplayValue();t.target.value=i,i&&e._updateInputSelection(),e.props.onChange&&a!=i&&e.fireChange(t)}return e.setState({selection:n.selection}),!0};if(!(o(m,function(){return n.undo()})||o(d,function(){return n.redo()})||o(r("Backspace"),function(){return n.backspace()})||o(r("Delete"),function(){return n.del()})||t.metaKey||t.altKey||t.ctrlKey||t.shiftKey||"Enter"===t.key||"Tab"===t.key||"ArrowLeft"!==t.key&&"ArrowRight"!=t.key)){var a=y(this.input);a.start===a.end&&void 0!==n.left&&(t.preventDefault(),"ArrowLeft"===t.key?n.left(a):n.right(a),this._updateInputSelection())}},e.prototype._onKeyPress=function(t){var e=this.state.mask;if(!(t.metaKey||t.altKey||t.ctrlKey||"Enter"===t.key)){y(this.input),e.getSelection();if(t.preventDefault(),this._updateMaskSelection(),function(t){if(e.input(t))return!0;if(t!==t.toUpperCase())return e.input(t.toUpperCase());if(t!=t.toLowerCase())return e.input(t.toLowerCase());return!1}(t.key)){var n=t.target.value,r=e.getValue();if(t.target.value=r,this._updateInputSelection(),this.setState({selection:e.selection}),this.props.onChange&&n!=r){var o={target:{value:e._getValue()}};this.props.onChange(o)}}}},e.prototype._onPaste=function(t){var e=this.state.mask;t.preventDefault(),this._updateMaskSelection(),e.paste(t.clipboardData.getData("Text"))&&(t.target.value=e.getValue(),setTimeout(this._updateInputSelection,0),this.setState({selection:e.selection}))},e.prototype._getMaskList=function(t){var e=this.state.mask.minCharsList(!!t);return e&&e.length<20?e:this.state.mask.minCharsList()},e.prototype._getDisplayValue=function(){var t=this.state.mask.getValue();return t===this.state.mask.emptyValue?"":t},e.prototype.selected=function(t,e){if(!t.split("").find(function(t){return Object(i.isMeta)(t)?t:void 0})){var n=this.state.mask;n.setValue(t),this.setState({mask:n})}},e.prototype.getMaxWidth=function(t,e){var n=arguments.length>2&&void 0!==arguments[2]?arguments[2]:200;if(e)return Math.max(e,n);if(!t||!t.length)return n;var r=t.map(function(t){return t.replace(/\u0332/g,"").length}).map(function(t){return function(e){return Math.max(t,e)}}(20));return 12*Math.max.apply(null,r)},e.prototype.getMapImg=function(){return v},e.prototype.getRxPlaceHolder=function(){return p},e.prototype.getInput=function(t){return t},e.prototype.inputClassName=function(){return"form-control"},e.prototype.getPopoverData=function(t,e,n,r){var o=this,a=this.getMaxWidth(t,n,300);if(!t||t.length<=1){if(!r)return;t=[r]}return{valueList:t,headers:e,MAXWIDTH:a,hasSmallHeader:[this._getDisplayValue()||"",r||this.state.mask.emptyValue].concat(t).find(function(t){return t.match(o.getRxPlaceHolder())})}},e.prototype.render=function(){var t=this,e=this.props,n=(e.mask,e.size),r=e.placeholder,a=(e.popover,e.selection,e.showAll,function(t,e){var n={};for(var r in t)e.indexOf(r)>=0||Object.prototype.hasOwnProperty.call(t,r)&&(n[r]=t[r]);return n}(e,["mask","size","placeholder","popover","selection","showAll"])),i=this.state.mask.pattern.length,u=o.a.createElement("input",s({style:{padding:"3px 0px 3px 0px"},className:this.inputClassName()},a,{ref:function(e){t.input=e},maxLength:i,onChange:this._onChange,onKeyDown:this._onKeyDown,onKeyPress:this._onKeyPress,onPaste:this._onPaste,onFocus:this._onFocus,onBlur:this._onBlur,placeholder:r||this.state.mask.emptyValue,size:n||i,value:this._getDisplayValue()}));return this.getInput(u,r)},e}(r.Component);function b(t,e){var n=t.toUpperCase(),r=e.toUpperCase();return n<r?-1:n>r?1:0}var _=function(t){function e(){return u(this,e),l(this,t.apply(this,arguments))}return c(e,t),e.prototype._createPopover=function(t){var e=this;if(!t)return o.a.createElement("span",null);var n=t.MAXWIDTH,r=t.hasSmallHeader,s=t.valueList,u=t.headers,l=r?l=o.a.createElement("pre",{className:"text-muted small-text"},Object(i.convertMask)("? - optional, * - zero or more")):"",c={width:n-50,maxWidth:n-50},p=void 0,f=void 0;console.log("valueList",s),s.length>20&&(p={height:"400px",display:"block",overflow:"auto"},f=o.a.createElement("div",null," "));var h=this;return o.a.createElement(a.Popover,{id:this.props.name+"myPopover",className:"col-xs-10 col-md-10",style:{width:n,maxWidth:n,fontSize:"10px",marginTop:"10px",marginBottom:"10px"}},l,o.a.createElement("table",{key:this.props.name+"myPopover1",className:"table-responsive table-striped table-hover table-condensed col-xs-10 col-md-10",style:c},o.a.createElement("thead",null,o.a.createElement("tr",null,u.map(function(t){return o.a.createElement("th",{key:e.props.name+t},t)}))),o.a.createElement("tbody",{style:p},s.sort(b).map(function(t){return o.a.createElement("tr",{onClick:function(e){return h.selected(t,e)},key:e.props.name+"L"+g(t)},o.a.createElement("td",{onClick:function(e){return h.selected(t,e)}},t))}))),f)},e.prototype.getInput=function(t,e){var n=void 0,r=this.getMapImg(),i=this.getPopoverData(this._getMaskList("no"!==this.props.showAll),["Possible Values"],void 0,e),s=this.props.popover?this._createPopover(i):o.a.createElement("span",null),u=this.state.mask.isDone();return u&&(n=r[this.state.mask.isDone()]),o.a.createElement("div",{style:{marginBotton:"0px",paddingLeft:"100px"}},o.a.createElement("div",{style:{marginBotton:"0px",fontSize:"70%",color:"red",fontStyle:"italic"}},u," "),"",o.a.createElement("div",{className:"form-group has-feedback"+n[1]},o.a.createElement(a.OverlayTrigger,{trigger:"focus",style:{marginBotton:"0px"},placement:"bottom",overlay:s},t),n[0]))},e}(k)}]).default}); | ||
//# sourceMappingURL=react-rxinput.min.js.map |
Sorry, the diff of this file is too big to display
Sorry, the diff of this file is not supported yet
Major refactor
Supply chain riskPackage has recently undergone a major refactor. It may be unstable or indicate significant internal changes. Use caution when updating to versions that include significant changes.
Found 1 instance in 1 package
No website
QualityPackage does not have a website.
Found 1 instance in 1 package
189
133794
18
1915
2
1
+ Added@babel/code-frame@7.12.11(transitive)
+ Added@babel/helper-validator-identifier@7.22.20(transitive)
+ Added@babel/highlight@7.24.2(transitive)
+ Added@eslint/eslintrc@0.4.3(transitive)
+ Added@humanwhocodes/config-array@0.5.0(transitive)
+ Added@humanwhocodes/object-schema@1.2.1(transitive)
+ Added@popperjs/core@2.11.8(transitive)
+ Added@react-aria/ssr@3.9.2(transitive)
+ Added@restart/hooks@0.4.16(transitive)
+ Added@restart/ui@1.6.8(transitive)
+ Added@swc/helpers@0.5.11(transitive)
+ Added@types/prop-types@15.7.12(transitive)
+ Added@types/react@18.3.1(transitive)
+ Added@types/react-transition-group@4.4.10(transitive)
+ Added@types/warning@3.0.3(transitive)
+ Addedacorn@7.4.1(transitive)
+ Addedacorn-jsx@5.3.2(transitive)
+ Addedajv@6.12.68.12.0(transitive)
+ Addedansi-colors@4.1.3(transitive)
+ Addedansi-regex@5.0.1(transitive)
+ Addedansi-styles@3.2.14.3.0(transitive)
+ Addedargparse@1.0.10(transitive)
+ Addedastral-regex@2.0.0(transitive)
+ Addedbalanced-match@1.0.2(transitive)
+ Addedbrace-expansion@1.1.11(transitive)
+ Addedcallsites@3.1.0(transitive)
+ Addedchalk@2.4.24.1.2(transitive)
+ Addedcolor-convert@1.9.32.0.1(transitive)
+ Addedcolor-name@1.1.31.1.4(transitive)
+ Addedconcat-map@0.0.1(transitive)
+ Addedcore_d@2.0.0(transitive)
+ Addedcross-spawn@7.0.3(transitive)
+ Addedcsstype@3.1.3(transitive)
+ Addeddebug@4.3.4(transitive)
+ Addeddeep-is@0.1.4(transitive)
+ Addeddequal@2.0.3(transitive)
+ Addeddoctrine@3.0.0(transitive)
+ Addeddom-helpers@5.2.1(transitive)
+ Addedemoji-regex@8.0.0(transitive)
+ Addedenquirer@2.4.1(transitive)
+ Addedescape-string-regexp@1.0.54.0.0(transitive)
+ Addedeslint@7.32.0(transitive)
+ Addedeslint-scope@5.1.1(transitive)
+ Addedeslint-utils@2.1.0(transitive)
+ Addedeslint-visitor-keys@1.3.02.1.0(transitive)
+ Addedeslint_d@9.1.2(transitive)
+ Addedespree@7.3.1(transitive)
+ Addedesprima@4.0.1(transitive)
+ Addedesquery@1.5.0(transitive)
+ Addedesrecurse@4.3.0(transitive)
+ Addedestraverse@4.3.05.3.0(transitive)
+ Addedesutils@2.0.3(transitive)
+ Addedfast-deep-equal@3.1.3(transitive)
+ Addedfast-json-stable-stringify@2.1.0(transitive)
+ Addedfast-levenshtein@2.0.6(transitive)
+ Addedfile-entry-cache@6.0.1(transitive)
+ Addedflat-cache@3.2.0(transitive)
+ Addedflatted@3.3.1(transitive)
+ Addedfs.realpath@1.0.0(transitive)
+ Addedfunctional-red-black-tree@1.0.1(transitive)
+ Addedglob@7.2.3(transitive)
+ Addedglob-parent@5.1.2(transitive)
+ Addedglobals@13.24.0(transitive)
+ Addedhas-flag@3.0.04.0.0(transitive)
+ Addedignore@4.0.6(transitive)
+ Addedimport-fresh@3.3.0(transitive)
+ Addedimurmurhash@0.1.4(transitive)
+ Addedincr-regex-package@1.0.4(transitive)
+ Addedinflight@1.0.6(transitive)
+ Addedinherits@2.0.4(transitive)
+ Addedis-extglob@2.1.1(transitive)
+ Addedis-fullwidth-code-point@3.0.0(transitive)
+ Addedis-glob@4.0.3(transitive)
+ Addedisexe@2.0.0(transitive)
+ Addedjs-yaml@3.14.1(transitive)
+ Addedjson-buffer@3.0.1(transitive)
+ Addedjson-schema-traverse@0.4.11.0.0(transitive)
+ Addedjson-stable-stringify-without-jsonify@1.0.1(transitive)
+ Addedkeyv@4.5.4(transitive)
+ Addedlevn@0.4.1(transitive)
+ Addedlodash.merge@4.6.2(transitive)
+ Addedlodash.truncate@4.4.2(transitive)
+ Addedlru-cache@6.0.0(transitive)
+ Addedminimatch@3.1.2(transitive)
+ Addedms@2.1.2(transitive)
+ Addednanolru@1.0.0(transitive)
+ Addednatural-compare@1.4.0(transitive)
+ Addedonce@1.4.0(transitive)
+ Addedoptionator@0.9.4(transitive)
+ Addedparent-module@1.0.1(transitive)
+ Addedpath-is-absolute@1.0.1(transitive)
+ Addedpath-key@3.1.1(transitive)
+ Addedpicocolors@1.0.0(transitive)
+ Addedprelude-ls@1.2.1(transitive)
+ Addedprogress@2.0.3(transitive)
+ Addedprop-types-extra@1.1.1(transitive)
+ Addedpunycode@2.3.1(transitive)
+ Addedreact-bootstrap@2.10.2(transitive)
+ Addedreact-lifecycles-compat@3.0.4(transitive)
+ Addedreact-transition-group@4.4.5(transitive)
+ Addedregexpp@3.2.0(transitive)
+ Addedrequire-from-string@2.0.2(transitive)
+ Addedresolve-from@4.0.0(transitive)
+ Addedrimraf@3.0.2(transitive)
+ Addedsemver@7.6.0(transitive)
+ Addedshebang-command@2.0.0(transitive)
+ Addedshebang-regex@3.0.0(transitive)
+ Addedslice-ansi@4.0.0(transitive)
+ Addedsprintf-js@1.0.3(transitive)
+ Addedstring-width@4.2.3(transitive)
+ Addedstrip-ansi@6.0.1(transitive)
+ Addedstrip-json-comments@3.1.1(transitive)
+ Addedsupports-color@5.5.07.2.0(transitive)
+ Addedtable@6.8.2(transitive)
+ Addedtext-table@0.2.0(transitive)
+ Addedtslib@2.6.2(transitive)
+ Addedtype-check@0.4.0(transitive)
+ Addedtype-fest@0.20.2(transitive)
+ Addeduncontrollable@7.2.18.0.4(transitive)
+ Addeduri-js@4.4.1(transitive)
+ Addedv8-compile-cache@2.4.0(transitive)
+ Addedwarning@4.0.3(transitive)
+ Addedwhich@2.0.2(transitive)
+ Addedword-wrap@1.2.5(transitive)
+ Addedwrappy@1.0.2(transitive)
+ Addedyallist@4.0.0(transitive)
- Removedexcept@^0.1.3
- Removedincr-regex-package@^0.9.6
- Removedreact-bootstrap@^0.30.2
- Removedasap@2.0.6(transitive)
- Removedassertion-error@1.1.0(transitive)
- Removedbabel-runtime@6.26.0(transitive)
- Removedchai@3.5.0(transitive)
- Removedcore-js@1.2.72.6.12(transitive)
- Removedcreate-react-class@15.7.0(transitive)
- Removeddeep-eql@0.1.3(transitive)
- Removeddom-helpers@3.4.0(transitive)
- Removedencoding@0.1.13(transitive)
- Removedexcept@0.1.3(transitive)
- Removedfbjs@0.8.18(transitive)
- Removediconv-lite@0.6.3(transitive)
- Removedincr-regex-package@0.9.9(transitive)
- Removedindexof@0.0.1(transitive)
- Removedis-stream@1.1.0(transitive)
- Removedisomorphic-fetch@2.2.1(transitive)
- Removedkeycode@2.2.1(transitive)
- Removednode-fetch@1.7.3(transitive)
- Removedpromise@7.3.1(transitive)
- Removedreact@15.7.0(transitive)
- Removedreact-bootstrap@0.30.10(transitive)
- Removedreact-overlays@0.6.12(transitive)
- Removedreact-prop-types@0.4.0(transitive)
- Removedregenerator-runtime@0.11.1(transitive)
- Removedsafer-buffer@2.1.2(transitive)
- Removedsetimmediate@1.0.5(transitive)
- Removedtype-detect@0.1.11.0.0(transitive)
- Removedua-parser-js@0.7.37(transitive)
- Removeduncontrollable@4.1.0(transitive)
- Removedwarning@3.0.0(transitive)
- Removedwhatwg-fetch@3.6.20(transitive)