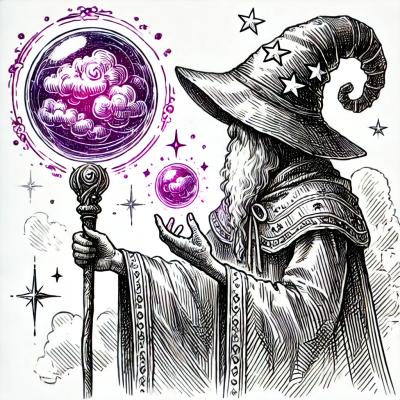
Security News
Cloudflare Adds Security.txt Setup Wizard
Cloudflare has launched a setup wizard allowing users to easily create and manage a security.txt file for vulnerability disclosure on their websites.
@humanwhocodes/object-schema
Advanced tools
The @humanwhocodes/object-schema package is a utility for validating JavaScript objects against a predefined schema. It allows developers to ensure that objects match a specific structure, types, and constraints, making it easier to enforce data integrity throughout applications.
Type Validation
This feature allows for the validation of object properties against specified types. In the example, the schema is defined to expect a property 'name' of type string. The validate method then checks if the provided object matches this schema.
{"const schema = new Schema({name: Schema.string()}); const result = schema.validate({name: 'John Doe'}); if (result.valid) { console.log('Valid!'); } else { console.log('Invalid:', result.errors); }"}
Required Properties
This feature ensures that certain properties are present in the object being validated. In the code sample, the 'name' property is marked as required, and the validation will fail if it is missing.
{"const schema = new Schema({name: Schema.string().required()}); const result = schema.validate({}); if (result.valid) { console.log('Valid!'); } else { console.log('Invalid:', result.errors); }"}
Default Values
This feature allows specifying default values for properties that are not present in the validated object. In the example, if the 'age' property is missing, it will default to 18.
{"const schema = new Schema({age: Schema.number().default(18)}); const result = schema.validate({}); console.log(result.value); // { age: 18 }"}
Joi is a powerful schema description language and data validator for JavaScript. Compared to @humanwhocodes/object-schema, Joi offers a more extensive set of features for describing and validating data structures, making it suitable for more complex validation scenarios.
Yup is a JavaScript schema builder for value parsing and validation. It adopts a similar approach to @humanwhocodes/object-schema but provides a more fluent interface and additional methods for transforming values, making it a good choice for both client-side and server-side validation.
Ajv is a fast JSON schema validator. It supports the latest JSON Schema standards and is known for its performance. Compared to @humanwhocodes/object-schema, Ajv is more focused on validating JSON data structures according to the JSON Schema standard, offering a different approach to schema validation.
If you find this useful, please consider supporting my work with a donation.
A JavaScript object merge/validation utility where you can define a different merge and validation strategy for each key. This is helpful when you need to validate complex data structures and then merge them in a way that is more complex than Object.assign()
.
You can install using either npm:
npm install @humanwhocodes/object-schema
Or Yarn:
yarn add @humanwhocodes/object-schema
Use CommonJS to get access to the ObjectSchema
constructor:
const { ObjectSchema } = require("@humanwhocodes/object-schema");
const schema = new ObjectSchema({
// define a definition for the "downloads" key
downloads: {
required: true,
merge(value1, value2) {
return value1 + value2;
},
validate(value) {
if (typeof value !== "number") {
throw new Error("Expected downloads to be a number.");
}
}
},
// define a strategy for the "versions" key
version: {
required: true,
merge(value1, value2) {
return value1.concat(value2);
},
validate(value) {
if (!Array.isArray(value)) {
throw new Error("Expected versions to be an array.");
}
}
}
});
const record1 = {
downloads: 25,
versions: [
"v1.0.0",
"v1.1.0",
"v1.2.0"
]
};
const record2 = {
downloads: 125,
versions: [
"v2.0.0",
"v2.1.0",
"v3.0.0"
]
};
// make sure the records are valid
schema.validate(record1);
schema.validate(record2);
// merge together (schema.merge() accepts any number of objects)
const result = schema.merge(record1, record2);
// result looks like this:
const result = {
downloads: 75,
versions: [
"v1.0.0",
"v1.1.0",
"v1.2.0",
"v2.0.0",
"v2.1.0",
"v3.0.0"
]
};
Instead of specifying a merge()
method, you can specify one of the following strings to use a default merge strategy:
"assign"
- use Object.assign()
to merge the two values into one object."overwrite"
- the second value always replaces the first."replace"
- the second value replaces the first if the second is not undefined
.For example:
const schema = new ObjectSchema({
name: {
merge: "replace",
validate() {}
}
});
Instead of specifying a validate()
method, you can specify one of the following strings to use a default validation strategy:
"array"
- value must be an array."boolean"
- value must be a boolean."number"
- value must be a number."object"
- value must be an object."object?"
- value must be an object or null."string"
- value must be a string."string!"
- value must be a non-empty string.For example:
const schema = new ObjectSchema({
name: {
merge: "replace",
validate: "string"
}
});
If you are defining a key that is, itself, an object, you can simplify the process by using a subschema. Instead of defining merge()
and validate()
, assign a schema
key that contains a schema definition, like this:
const schema = new ObjectSchema({
name: {
schema: {
first: {
merge: "replace",
validate: "string"
},
last: {
merge: "replace",
validate: "string"
}
}
}
});
schema.validate({
name: {
first: "n",
last: "z"
}
});
If the merge strategy for a key returns undefined
, then the key will not appear in the final object. For example:
const schema = new ObjectSchema({
date: {
merge() {
return undefined;
},
validate(value) {
Date.parse(value); // throws an error when invalid
}
}
});
const object1 = { date: "5/5/2005" };
const object2 = { date: "6/6/2006" };
const result = schema.merge(object1, object2);
console.log("date" in result); // false
If you'd like the presence of one key to require the presence of another key, you can use the requires
property to specify an array of other properties that any key requires. For example:
const schema = new ObjectSchema();
const schema = new ObjectSchema({
date: {
merge() {
return undefined;
},
validate(value) {
Date.parse(value); // throws an error when invalid
}
},
time: {
requires: ["date"],
merge(first, second) {
return second;
},
validate(value) {
// ...
}
}
});
// throws error: Key "time" requires keys "date"
schema.validate({
time: "13:45"
});
In this example, even though date
is an optional key, it is required to be present whenever time
is present.
BSD 3-Clause
FAQs
An object schema merger/validator
The npm package @humanwhocodes/object-schema receives a total of 24,755,747 weekly downloads. As such, @humanwhocodes/object-schema popularity was classified as popular.
We found that @humanwhocodes/object-schema demonstrated a healthy version release cadence and project activity because the last version was released less than a year ago. It has 1 open source maintainer collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
Cloudflare has launched a setup wizard allowing users to easily create and manage a security.txt file for vulnerability disclosure on their websites.
Security News
The Socket Research team breaks down a malicious npm package targeting the legitimate DOMPurify library. It uses obfuscated code to hide that it is exfiltrating browser and crypto wallet data.
Security News
ENISA’s 2024 report highlights the EU’s top cybersecurity threats, including rising DDoS attacks, ransomware, supply chain vulnerabilities, and weaponized AI.