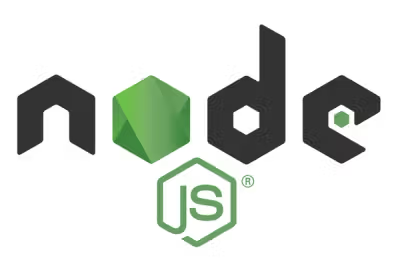
Security News
require(esm) Backported to Node.js 20, Paving the Way for ESM-Only Packages
require(esm) backported to Node.js 20, easing the transition to ESM-only packages and reducing complexity for developers as Node 18 nears end-of-life.
A React component that synthesis text into speech using Web Speech API
A React component that synthesis text into speech using Web Speech API.
This project scaffold can be found at compulim/react-component-template
.
Try out the demo at https://compulim.github.io/react-say/.
First, run npm install react-say
for production build. Or run npm install react-say@master
for latest development build.
The following will speak the text immediately upon showing up. Some browsers may not speak the text until the user interacted with the page.
import Say from 'react-say';
export default props =>
<Say speak="A quick brown fox jumped over the lazy dogs." />
You may want to customize the speech by varying pitch, rate, and volume. You can use <Composer>
and <Say>
to say your text.
import Say from 'react-say';
export default props =>
<Say
pitch={ 1.1 }
rate={ 1.5 }
speak="A quick brown fox jumped over the lazy dogs."
volume={ .8 }
/>
Note: variation will take effect for new/modified
<Say>
You may want to say something after the user interacted with the page, for example, after clicking on a button. We have built <SayButton>
that speak immediately after clicking on it. Some browsers may requires "priming" with a button.
import { SayButton } from 'react-say';
export default props =>
<SayButton
onClick={ event => console.log(event) }
speak="A quick brown fox jumped over the lazy dogs."
>
Tell me a story
</SayButton>
Instead of passing a SpeechSynthesisVoice
object, you can pass a function (voices: SpeechSynthesisVoice[]) => SpeechSynthesisVoice
to select the voice just before the text is synthesized.
import Say from 'react-say';
export default props =>
<Say
speak="A quick brown fox jumped over the lazy dogs."
voice={ voices => [].find.call(voices, v => v.lang === 'zh-HK') }
/>
Note: it also works with
<SayButton>
.
<Say>
or <SayButton>
is unmounted, the utterance will continue to speak
Like us? Star us.
Want to make it better? File us an issue.
Don't like something you see? Submit a pull request.
FAQs
[](https://badge.fury.io/js/react-say) [](https://travis-ci.org/compulim/react-say)
We found that react-say demonstrated a not healthy version release cadence and project activity because the last version was released a year ago. It has 2 open source maintainers collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
require(esm) backported to Node.js 20, easing the transition to ESM-only packages and reducing complexity for developers as Node 18 nears end-of-life.
Security News
PyPI now supports iOS and Android wheels, making it easier for Python developers to distribute mobile packages.
Security News
Create React App is officially deprecated due to React 19 issues and lack of maintenance—developers should switch to Vite or other modern alternatives.