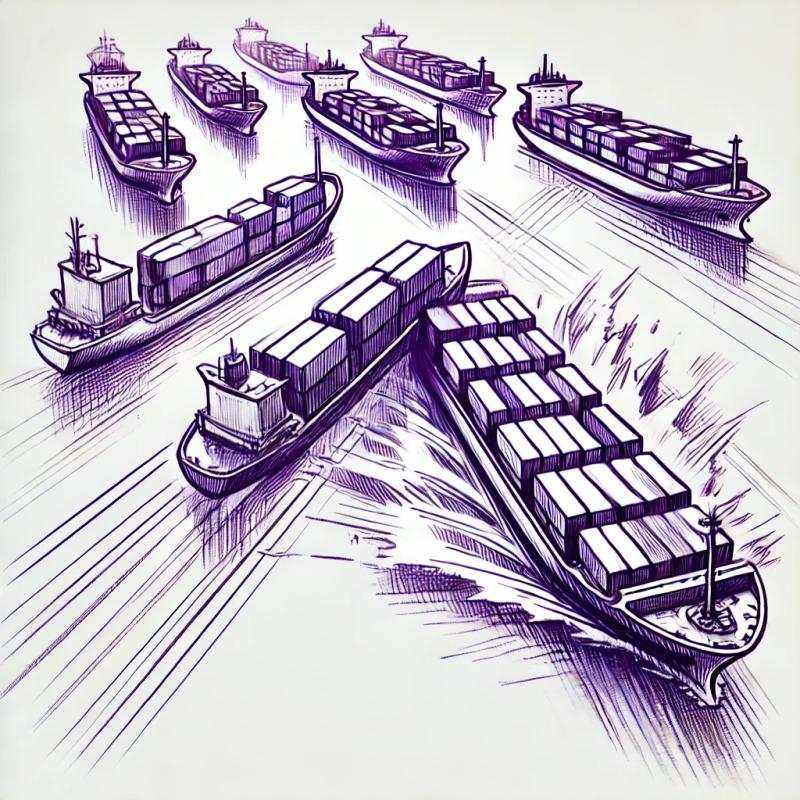
Security News
Namecheap Takes Down Polyfill.io Service Following Supply Chain Attack
Polyfill.io has been serving malware for months via its CDN, after the project's open source maintainer sold the service to a company based in China.
react-simple-captcha
Advanced tools
Readme
React Simple Captcha is a very powerful and easy to use captcha for React JS.
Demo Demo can be seen here.
Install
$ npm install react-simple-captcha
Usage Guide
Just follow these 6 easy steps to use the react simple captcha:
**react-html-parser**
Install 'react-html-parser'
$ npm install react-html-parser
Import 'react-html-parser'
import ReactHtmlParser from 'react-html-parser';
react-simple-captcha
Import all functions from react-simple-captcha
import { loadcaptchaenginge, LoadCanvasTemplate, LoadCanvasTemplateNoReload, validateCaptcha } from 'react-simple-captcha';
Place **LoadCanvasTemplate** or **LoadCanvasTemplateNoReload** *(if you do not want 'Reload Captcha' functionality)* in your render code in the function ReactHtmlParser()
render() {
return (<div>
{ReactHtmlParser(LoadCanvasTemplate)}
</div>);
};
OR
render() {
return (<div>
{ReactHtmlParser(LoadCanvasTemplateNoReload)}
</div>);
};
Paste **loadcaptchaenginge(6)** *(You can change 6 to number of captcha charcters you want)* in **componentDidMount**
componentDidMount () {
loadcaptchaenginge(6);
};
Validate captcha by using **validateCaptcha(user_captcha_value)**
doSubmit = () => {
let user_captcha_value = document.getElementById('captcha').value;
if (validateCaptcha(user_captcha_value)==true) {
alert('Captcha Matched');
}
else {
alert('Captcha Does Not Match');
}
};
OR
If you don't watch captcha to be reloaded if user enter the wrong value then set second parameter to *false* **validateCaptcha(user_captcha_value, false)**
doSubmit = () => {
let user_captcha_value = document.getElementById('captcha').value;
if (validateCaptcha(user_captcha_value, false)==true) {
alert('Captcha Matched');
}
else {
alert('Captcha Does Not Match');
}
};
Listed are all the options available for react-simple-captcha
Name | Description |
---|---|
LoadCanvasTemplate | It will load the captcha with 'Reload Captcha' functionality. Place between your render code, usage example {ReactHtmlParser(LoadCanvasTemplate)} |
LoadCanvasTemplateNoReload | It will load the captcha without 'Reload Captcha' functionality. Place between your render code, usage example {ReactHtmlParser(LoadCanvasTemplateNoReload)} |
loadcaptchaenginge(Number_Of_Captcha_Charcters); | Simply paste it in componentDidMount(). Pass number of captcha characters you want to display. |
validateCaptcha(User_Submitted_Value) | Will return true if user submitted value matches with captcha otherwise false. Also will reload captcha if user submitted value is false |
validateCaptcha(User_Submitted_Value, false) | Will return true if user submitted value matches with captcha otherwise false. Will not reload captcha if user submitted value is false |
Let's create a class name CaptchaTest with react simple captcha functionality:
import React, { Component } from 'react';
import ReactHtmlParser from 'react-html-parser';
import { loadcaptchaenginge, LoadCanvasTemplate, LoadCanvasTemplateNoReload, validateCaptcha } from 'react-simple-captcha';
class CaptchaTest extends Component {
componentDidMount () {
loadcaptchaenginge(6);
};
doSubmit = () => {
let user_captcha = document.getElementById('captcha').value;
if (validateCaptcha(user_captcha)==true) {
alert('Captcha Matched');
loadcaptchaenginge(6);
document.getElementById('captcha').value = "";
}
else {
alert('Captcha Does Not Match');
document.getElementById('captcha').value = "";
}
};
render() {
return (<div>
<div className="container">
<div className="form-group">
<div className="col mt-3">
{ReactHtmlParser(LoadCanvasTemplate)}
</div>
<div className="col mt-3">
<div><input placeholder="Enter Captcha Value" id="captcha" name="captcha" type="text"></input></div>
</div>
<div className="col mt-3">
<div><button class="btn btn-primary" onClick={() => this.doSubmit()}>Submit</button></div>
</div>
</div>
</div>
</div>);
};
}
export default CaptchaTest;
Import CaptchaTest in index.js
import CaptchaTest from './captcha_test';
Now replace ReactDOM.render with
ReactDOM.render(<CaptchaTest />, document.getElementById('root'));
Works in every modern browser which has support for canvas element.
react-simple-captcha is licensed under the MIT license.
Name: Masroor Ejaz
Linkedin: https://www.linkedin.com/in/masroorejaz/
Twitter: https://twitter.com/masroorejaz
FAQs
A very simple,powerful and highly customizable captcha for ReactJS
The npm package react-simple-captcha receives a total of 4,922 weekly downloads. As such, react-simple-captcha popularity was classified as popular.
We found that react-simple-captcha demonstrated a healthy version release cadence and project activity because the last version was released less than a year ago. It has 1 open source maintainer collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
Polyfill.io has been serving malware for months via its CDN, after the project's open source maintainer sold the service to a company based in China.
Security News
OpenSSF is warning open source maintainers to stay vigilant against reputation farming on GitHub, where users artificially inflate their status by manipulating interactions on closed issues and PRs.
Security News
A JavaScript library maintainer is under fire after merging a controversial PR to support legacy versions of Node.js.