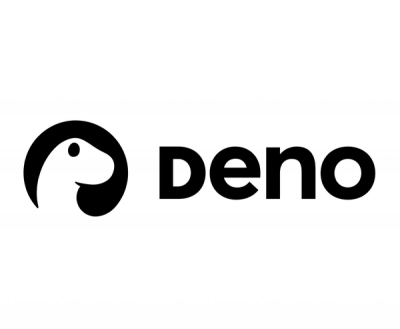
Security News
Deno 2.2 Improves Dependency Management and Expands Node.js Compatibility
Deno 2.2 enhances Node.js compatibility, improves dependency management, adds OpenTelemetry support, and expands linting and task automation for developers.
react-simple-keyboard
Advanced tools
An easily customisable and responsive on-screen virtual keyboard for React.js projects.
Want the vanilla js version? Get simple-keyboard instead!
npm install react-simple-keyboard --save
import React, {Component} from 'react';
import Keyboard from 'react-simple-keyboard';
import 'simple-keyboard/build/css/index.css';
class App extends Component {
onChange = (input) => {
console.log("Input changed", input);
}
onKeyPress = (button) => {
console.log("Button pressed", button);
}
render(){
return (
<Keyboard
onChange={input =>
this.onChange(input)}
onKeyPress={button =>
this.onKeyPress(button)}
/>
);
}
}
export default App;
Need a more extensive example? Click here.
You can customize the Keyboard by passing options (props) to it. Here are the available options (the code examples are the defaults):
Modify the keyboard layout
layout={{
'default': [
'` 1 2 3 4 5 6 7 8 9 0 - = {bksp}',
'{tab} q w e r t y u i o p [ ] \\',
'{lock} a s d f g h j k l ; \' {enter}',
'{shift} z x c v b n m , . / {shift}',
'.com @ {space}'
],
'shift': [
'~ ! @ # $ % ^ & * ( ) _ + {bksp}',
'{tab} Q W E R T Y U I O P { } |',
'{lock} A S D F G H J K L : " {enter}',
'{shift} Z X C V B N M < > ? {shift}',
'.com @ {space}'
]
}}
Specifies which layout should be used.
layoutName={"default"}
Replaces variable buttons (such as
{bksp}
) with a human-friendly name (e.g.: "delete").
display={{
'{bksp}': 'delete',
'{enter}': '< enter',
'{shift}': 'shift',
'{s}': 'shift',
'{tab}': 'tab',
'{lock}': 'caps',
'{accept}': 'Submit',
'{space}': ' ',
'{//}': ' '
}}
A prop to add your own css classes. You can add multiple classes separated by a space.
theme={"hg-theme-default"}
Runs a console.log every time a key is pressed. Displays the buttons pressed and the current input.
debug={false}
Specifies whether clicking the "ENTER" button will input a newline (
\n
) or not.
newLineOnEnter={false}
Allows you to use a single simple-keyboard instance for several inputs.
inputName={"default"}
Executes the callback function on key press. Returns button layout name (i.e.: "{shift}").
onKeyPress={(button) => console.log(button)}
Executes the callback function on input change. Returns the current input's string.
onChange={(input) => console.log(input)}
Executes the callback function on input change. Returns the input object with all defined inputs. This is useful if you're handling several inputs with simple-keyboard, as specified in the "Using several inputs" guide.
onChangeAll={(inputs) => console.log(inputs)}
simple-keyboard has a few methods you can use to further control it's behavior.
To access these functions, you need a ref
of the simple-keyboard component, like so:
<Keyboard
ref={r => this.keyboard = r}
[...]
/>
// Then, on componentDidMount ..
this.keyboard.methodName(params);
Clear the keyboard's input.
// For default input (i.e. if you have only one)
this.keyboard.clearInput();
// For specific input
// Must have been previously set using the "inputName" prop.
this.keyboard.clearInput("inputName");
Get the keyboard's input (You can also get it from the onChange prop).
// For default input (i.e. if you have only one)
let input = this.keyboard.getInput();
// For specific input
// Must have been previously set using the "inputName" prop.
let input = this.keyboard.getInput("inputName");
Set the keyboard's input. Useful if you want the keybord to initialize with a default value, for example.
// For default input (i.e. if you have only one)
this.keyboard.setInput("Hello World!");
// For specific input
// Must have been previously set using the "inputName" prop.
this.keyboard.setInput("Hello World!", "inputName");
It returns a promise, so if you want to run something after it's applied, call it as so:
let inputSetPromise = this.keyboard.setInput("Hello World!");
inputSetPromise.then((result) => {
console.log("Input set");
});
Set the inputName option for each input you want to handle with simple-keyboard.
For example:
// Tell simple-keyboard which input is active
setActiveInput = (event) => {
this.setState({
inputName: event.target.id
});
}
// When the inputs are changed
// (retrieves all inputs as an object instead of just the current input's string)
onChangeAll = (input) => {
this.setState({
input: input
}, () => {
console.log("Inputs changed", input);
});
}
render(){
return (
<div>
<input id="input1" onFocus={this.setActiveInput} value={this.state.input['input1'] || ""}/>
<input id="input2" onFocus={this.setActiveInput} value={this.state.input['input2'] || ""}/>
<Keyboard
ref={r => this.keyboard = r}
inputName={this.state.inputName}
onChangeAll={inputs => this.onChangeAll(inputs)}
layoutName={this.state.layoutName}
/>
</div>
);
https://franciscohodge.com/simple-keyboard/demo
npm install
npm start
This is a work in progress. Feel free to submit any issues you have at: https://github.com/hodgef/react-simple-keyboard/issues
FAQs
React.js Virtual Keyboard
The npm package react-simple-keyboard receives a total of 32,611 weekly downloads. As such, react-simple-keyboard popularity was classified as popular.
We found that react-simple-keyboard demonstrated a healthy version release cadence and project activity because the last version was released less than a year ago. It has 0 open source maintainers collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
Deno 2.2 enhances Node.js compatibility, improves dependency management, adds OpenTelemetry support, and expands linting and task automation for developers.
Security News
React's CRA deprecation announcement sparked community criticism over framework recommendations, leading to quick updates acknowledging build tools like Vite as valid alternatives.
Security News
Ransomware payment rates hit an all-time low in 2024 as law enforcement crackdowns, stronger defenses, and shifting policies make attacks riskier and less profitable.