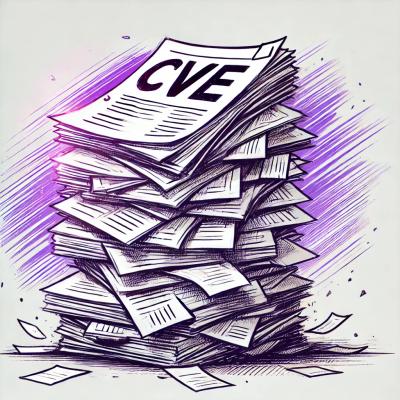
Security News
Node.js EOL Versions CVE Dubbed the "Worst CVE of the Year" by Security Experts
Critics call the Node.js EOL CVE a misuse of the system, sparking debate over CVE standards and the growing noise in vulnerability databases.
A stubbing tool that leads to safer and more maintainable React unit tests.
You create a stub component out of a real component. The stub is just a normal React component -- you can render it, nest it, inspect its properties, do whatever you want. Example:
// Pretend this is something in your app:
import Login from 'components/login';
import reactStub from 'react-stub';
let LoginStub = reactStub(Login);
npm install --save-dev react-stub
Stubs and mocks are generally risky in dynamic languages such as JavaScript because you have to keep track of interface refactoring in your head and that's hard. This leads to a maintainance burden and in the worst case scenario you might have a passing test suite yet a failing application. React Stub attempts to solve these problems.
Here is an overview of error feedback you get when running tests:
A good case for stubbed components is when you have one component that depends on another but you want to unit test each of them independently. Stubbing out the one you're not testing helps you focus on the interface of the dependent component, which is typically its properties. This also helps keep your test suite lean and fast.
Imagine you have an App
component that relies on a Login
component. If you want
to test App
in isolation, the first thing you need to do is
re-design your App
class to accept a stubbed dependency:
import React, { Component, PropTypes } from 'react';
// Import the real component for reference.
import DefaultLogin from 'components/login';
export default class App extends Component {
static propTypes = {
Login: PropTypes.func.isRequired,
}
static defaultProps = {
// When not testing, the real Login is used.
Login: DefaultLogin,
}
render() {
let Login = this.props.Login;
return <Login/>;
}
}
This component design allows you to inject a stub component while testing, like this:
// Pretend these are custom components for your app.
import App from 'components/app';
import Login from 'components/login';
import ReactTestUtils from 'react-addons-test-utils';
import reactStub from 'react-stub';
let LoginStub = reactStub(Login);
ReactTestUtils.renderIntoDocument(
<App Login={LoginStub} />
);
If the stub gets used incorrectly, you'll see an exception when the top level component gets rendered. For example, if you pass an unexpected attribute, you'll see an error:
ReactTestUtils.renderIntoDocument(
<LoginStub userId={321} />
);
...
reactStub:Login does not accept property userId
If you want to go beyond simply verifying the PropTypes of the stubbed component, you can use typical React testing approaches. For example:
// Pretend these are custom components for your app.
import App from 'components/app';
import Login from 'components/login';
import ReactTestUtils from 'react-addons-test-utils';
import reactStub from 'react-stub';
let LoginStub = reactStub(Login);
let renderedApp = ReactTestUtils.renderIntoDocument(
<App Login={LoginStub} />
);
let renderedLogin = ReactTestUtils.findRenderedComponentWithType(
renderedApp, LoginStub
);
// You could now make assertions about `renderedLogin.props` ...
Clone the source, install NodeJS, and run this from the source directory to install the dependency modules:
npm install
Make sure you have grunt installed and on your path. Otherwise, run this:
npm install -g grunt
To run the tests each time you edit a file, run:
grunt watch-test
To run a test suite once, run:
grunt test
To check for lint errors, run:
grunt eslint
To build a distribution from source, run:
grunt build
To create a new release:
package.json
.README.md
is up to date.git tag 0.0.1
) and push the tag with
git push --tags
.grunt build
to create a common JS distribution (in the dist
folder).npm publish
.0.0.2
(2015-12-14)
0.0.1
(2015-12-14)
FAQs
A stubbing tool that leads to safer and more maintainable React unit tests
The npm package react-stub receives a total of 2 weekly downloads. As such, react-stub popularity was classified as not popular.
We found that react-stub demonstrated a not healthy version release cadence and project activity because the last version was released a year ago. It has 1 open source maintainer collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
Critics call the Node.js EOL CVE a misuse of the system, sparking debate over CVE standards and the growing noise in vulnerability databases.
Security News
cURL and Go security teams are publicly rejecting CVSS as flawed for assessing vulnerabilities and are calling for more accurate, context-aware approaches.
Security News
Bun 1.2 enhances its JavaScript runtime with 90% Node.js compatibility, built-in S3 and Postgres support, HTML Imports, and faster, cloud-first performance.