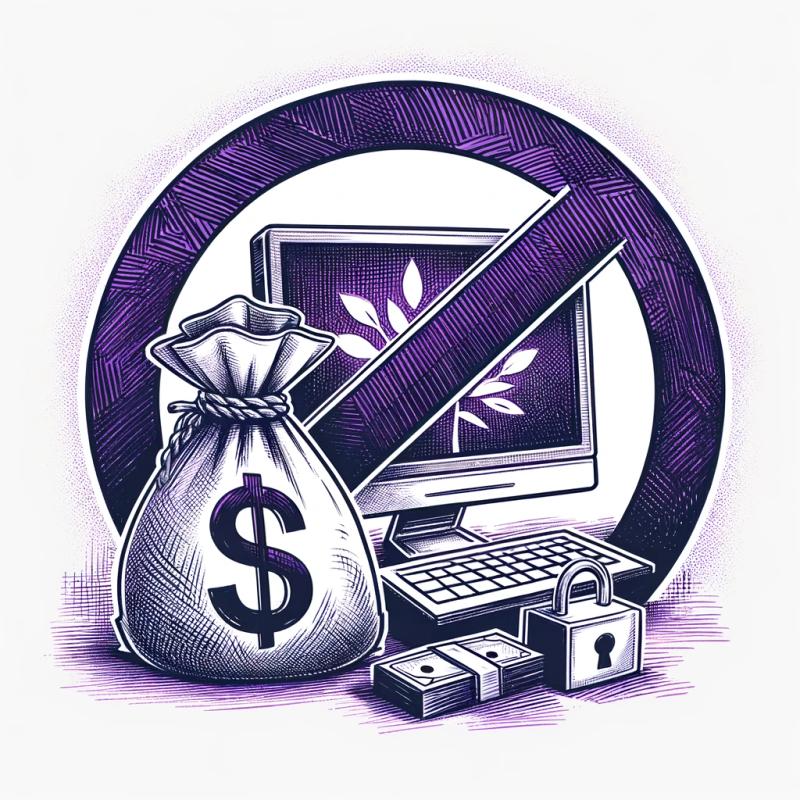
Security News
The Push to Ban Ransom Payments Is Gaining Momentum
Ransomware costs victims an estimated $30 billion per year and has gotten so out of control that global support for banning payments is gaining momentum.
react-tech-tree
Advanced tools
Readme
Create highly customizable tech trees (or generic graphical trees)
Light
Responsive
Image Support
Customizable
npm install --save react-tech-tree
yarn add react-tech-tree
import React from "react";
import { Tree } from "react-tech-tree";
import "react-tech-tree/dist/index.css";
const nodes = [
[
{ id: "A0", name: "A" },
{ id: "B0", name: "B" }
]
];
const links = [{ from: "A0", to: "B0" }];
function ExampleComponent() {
return <Tree nodes={nodes} links={links} />;
}
import { Tree } from "react-tech-tree";
Prop | Type | Description |
---|---|---|
id | string | id property (should be unique). E.g: uuuid |
nodes | {id:string, name: string}[][] | 2d array with nodes information |
links | {from:string, to: string}[] | array with links information |
NodeElement | ReactElement | (optional) React Element to be used as Node. Defaults to Node . |
nodeProps | object | (optional) Properties to pass down to Node elements. See Node . |
linkProps | object | (optional) See below. |
Prop | Type | Description |
---|---|---|
pathMaker | (r0: Rect,r1: Rect, props?: object) => string | (optional) Function to generate string paths. Defaults to simplePathMaker |
import { Node } from "react-tech-tree";
Prop | Type | Description |
---|---|---|
onClick | (e: MouseEvent) => void | (optional) Event Handler fired when the Node is clicked. Defaults to nodeClickHandler |
import { Sprite } from "react-tech-tree";
Prop | Type | Description |
---|---|---|
name | string | name of spritesheet entry or image name for this Sprite |
styleName | () => object | function to style the Sprite from its name. Example |
import { Link } from "react-tech-tree";
Prop | Type | Description |
---|---|---|
pathData | string | data to build links. E.g: M 0 0 L 1 1 |
import { nodeClickHandler } from "react-tech-tree";
clickHandler function used by Node
internally.
Use it when you are trying to build a custom NodeElement
that you pass to a Tree
.
It will:
Node
with class active
Node's children
with class next-active
//...
function MyNodeElement({ name, id }) {
return (
<button id={id} onClick={nodeClickHandler}>
{name}
</button>
);
}
function ExampleComponent() {
return <Tree nodes={nodes} links={links} NodeElement={MyNodeElement} />;
}
import { prepareSpritesheetStyle } from "react-tech-tree";
When using spritesheets, you may need to style each Sprite
based on its name.
If you are using TexturePacker, you can use this function as follows:
//...
import spriteInformation from "./data/spritesheet.json";
import spriteImage from "./data/spritesheet.png";
//...
const nodeProps = {
styleName: prepareSpritesheetStyle(spriteImage, spriteInformation)
};
function ExampleComponent() {
return <Tree nodes={nodes} links={links} nodeProps={nodeProps} />;
}
import { simplePathMaker } from "react-tech-tree";
Pure function that return a string path between the center of two rectangles.
const rect0 = { x: 0, y: 24, width: 4, height: 4 };
const rect1 = { x: 8, y: 32, width: 2, height: 2 };
const path = simplePathMaker(rect0, rect1);
// "M 2 26 L 9 33"
It is used at the default value for building links between Nodes
.
It can be passed down in linkProps
to a Tree
. See example
//...
const linkProps = { pathMaker: simplePathMaker };
function ExampleComponent() {
return <Tree nodes={nodes} links={links} linkProps={linkProps} />;
}
For further usage, go to the examples page
MIT © ldd
FAQs
React tech tree component
The npm package react-tech-tree receives a total of 3 weekly downloads. As such, react-tech-tree popularity was classified as not popular.
We found that react-tech-tree demonstrated a not healthy version release cadence and project activity because the last version was released a year ago. It has 1 open source maintainer collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
Ransomware costs victims an estimated $30 billion per year and has gotten so out of control that global support for banning payments is gaining momentum.
Application Security
New SEC disclosure rules aim to enforce timely cyber incident reporting, but fear of job loss and inadequate resources lead to significant underreporting.
Security News
The Python Software Foundation has secured a 5-year sponsorship from Fastly that supports PSF's activities and events, most notably the security and reliability of the Python Package Index (PyPI).