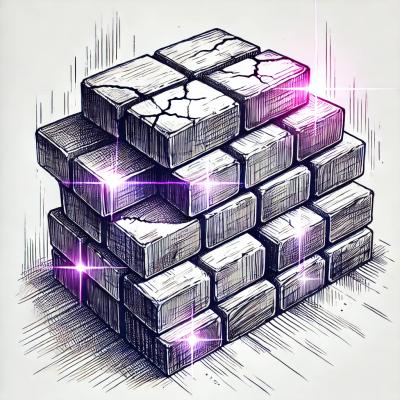
Security News
Input Validation Vulnerabilities Dominate MITRE's 2024 CWE Top 25 List
MITRE's 2024 CWE Top 25 highlights critical software vulnerabilities like XSS, SQL Injection, and CSRF, reflecting shifts due to a refined ranking methodology.
react-timing-hooks
Advanced tools
React hooks for setTimeout, setInterval, requestAnimationFrame, requestIdleCallback
This package contains a bunch of hooks that allow you to make use of setTimeout
,
setInterval
, setIdleCallback
and requestAnimationFrame
in your react-components without
having to worry about handling "IDs" or the clean up of leaking timers etc. Apart from that
the hooks are quite easy to use.
Oh, and the lib is super light-weight, too, since it doesn't include any other dependencies!
# via npm
npm i react-timing-hooks
# via yarn
yarn add react-timing-hooks
useTimeout(callback, timeout)
callback
- a function that will be invoked as soon as the timeout expires
timeout
- the timeout in milliseconds
Example:
// Hide something after 2 seconds
const hideDelayed = useTimeout(() => setHide(true), 2000)
return <button onClick={hideDelayed}>Hide!</button>
useTimeoutEffect(effectCallback, deps)
effectCallback
- will receive one argument timeout(f, timeout)
that has the
same signature as a native setTimeout
deps
- is your regular useEffect
dependency array
This works like a regular useEffect
hook, except that it adds a setTimeout
like function
to the callback args.
Example:
// Delay the transition of a color by one second everytime it changes
useTimeoutEffect(timeout => {
if (color) {
timeout(() => transitionTo(color), 1000)
}
}, [color])
useInterval(intervalCallback, delay)
intervalCallback
- will be run every [delay] (second arg) seconds
delay
- is the delay at which the callback will be run. If delay is null
the interval will be suspended.
Example:
// Increase count every 200 milliseconds
const [count, setCount] = useState(0)
useInterval(() => setCount(count + 1), 200)
useAnimationFrame(callback)
callback
- a function that will be invoked on the next animation frameuseAnimationFrameLoop(callback)
callback
- a function that will be invoked in an animation frame loopExample:
// Update canvas on every frame
const updateCanvas () => {
// ...
}
useAnimationFrameLoop(updateCanvas)
useIdleCallback(callback, options)
callback
- a function that will be invoked as soon as the browser decides to run the idle callback
options
- options for requestIdleCallback
Example:
// Track button click when idle
const trackClickWhenIdle = useIdleCallback(trackClick)
return <button onClick={trackClickWhenIdle}>Track me!</button>
useIdleCallbackEffect(effectCallback, deps)
effectCallback
- will receive one argument requestIdleCallback(f, opts)
that has the
same signature as the native requestIdleCallback
deps
- is your regular useEffect
dependency array
This works like a regular useEffect
hook, except that it adds a requestIdleCallbackEffect
like function
to the callback args.
Note: This hook will print a warning if the browser doesn't support requestIdleCallback
.
Example:
// Track page view when browser is idle
useIdleCallbackEffect(onIdle => {
if (page) {
onIdle(() => trackPageView(page))
}
}, [page])
Writing a timeout or anything similar requires a lot of boilerplate (if you don't do it quick and dirty). This library is supposed to give you easy access to those functionalities while keeping your code clean.
For example: You might have a timeout that runs under a certain condition. In this case a cleanup
has to be done in a separate useEffect
call that cleans everything up (but only on unmount).
Your code could look like this:
import { useEffect } from 'react'
// ...
const timeoutId = useRef(null)
useEffect(() => {
if (depA && depB) {
timeoutId.current = setTimeout(() => doSomething(), 1000)
}
}, [depA, depB])
useEffect(() => {
return function onUnmount() {
if (timeoutId.current !== null) {
clearTimeout(timeoutId.current)
}
}
}, [timeoutId])
With react-timing-hooks
you can just write:
import { useTimeoutEffect } from 'react-timing-hooks'
// ...
useTimeoutEffect((timeout) => {
if (depA && depB) {
timeout(() => doSomething(), 1000)
}
}, [depA, depB])
In this case react-timing-hooks
automatically took care of cleaning up the timeout for you (if the component is mounted for less than a second for instance).
FAQs
React hooks for setTimeout, setInterval, requestAnimationFrame, requestIdleCallback
The npm package react-timing-hooks receives a total of 829 weekly downloads. As such, react-timing-hooks popularity was classified as not popular.
We found that react-timing-hooks demonstrated a healthy version release cadence and project activity because the last version was released less than a year ago. It has 0 open source maintainers collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
MITRE's 2024 CWE Top 25 highlights critical software vulnerabilities like XSS, SQL Injection, and CSRF, reflecting shifts due to a refined ranking methodology.
Security News
In this segment of the Risky Business podcast, Feross Aboukhadijeh and Patrick Gray discuss the challenges of tracking malware discovered in open source softare.
Research
Security News
A threat actor's playbook for exploiting the npm ecosystem was exposed on the dark web, detailing how to build a blockchain-powered botnet.