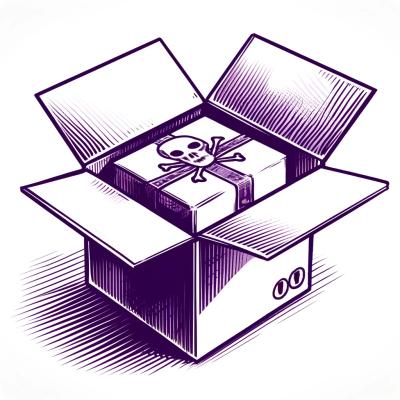
Security News
Research
Data Theft Repackaged: A Case Study in Malicious Wrapper Packages on npm
The Socket Research Team breaks down a malicious wrapper package that uses obfuscation to harvest credentials and exfiltrate sensitive data.
react-toast-notifications
Advanced tools
A configurable, composable, toast notification system for react.
This was a great project to learn from and fulfilled the requirements it set out to. Unfortunately, I can no-longer give this project the time it needs. Consider react-hot-toast as an alternative, or look at the source and make your own 🎉 (there really isn't much to it).
A configurable, composable, toast notification system for react.
https://jossmac.github.io/react-toast-notifications
yarn add react-toast-notifications
Wrap your app in the ToastProvider
, which provides context for the Toast
descendants.
import { ToastProvider, useToasts } from 'react-toast-notifications';
const FormWithToasts = () => {
const { addToast } = useToasts();
const onSubmit = async value => {
const { error } = await dataPersistenceLayer(value);
if (error) {
addToast(error.message, { appearance: 'error' });
} else {
addToast('Saved Successfully', { appearance: 'success' });
}
};
return <form onSubmit={onSubmit}>...</form>;
};
const App = () => (
<ToastProvider>
<FormWithToasts />
</ToastProvider>
);
For brevity:
PlacementType
is equal to 'bottom-left' | 'bottom-center' | 'bottom-right' | 'top-left' | 'top-center' | 'top-right'
.TransitionState
is equal to 'entering' | 'entered' | 'exiting' | 'exited'
.Property | Description |
---|---|
autoDismissTimeout number | Default 5000 . The time until a toast will be dismissed automatically, in milliseconds. |
autoDismiss boolean | Default: false . Whether or not to dismiss the toast automatically after a timeout. |
children Node | Required. Your app content. |
components { ToastContainer, Toast } | Replace the underlying components. |
newestOnTop boolean | Default false . When true, insert new toasts at the top of the stack. |
placement PlacementType | Default top-right . Where, in relation to the viewport, to place the toasts. |
portalTargetSelector string | Which element to attach the container's portal to. Uses document.body when not provided. |
transitionDuration number | Default 220 . The duration of the CSS transition on the Toast component. |
Property | Description |
---|---|
appearance | Used by the default toast. One of success , error , warning , info . |
children | Required. The content of the toast notification. |
autoDismiss boolean | Inherited from ToastProvider if not provided. |
autoDismissTimeout number | Inherited from ToastProvider . |
onDismiss: Id => void | Passed in dynamically. Can be called in a custom toast to dismiss it. |
placement PlacementType | Inherited from ToastProvider . |
transitionDuration number | Inherited from ToastProvider . |
transitionState: TransitionState | Passed in dynamically. |
The useToast
hook has the following signature:
const {
addToast,
removeToast,
removeAllToasts,
updateToast,
toastStack,
} = useToasts();
The addToast
method has three arguments:
Node
.Options
object, which can take any shape you like. Options.appearance
is required when using the DefaultToast
. When departing from the default shape, you must provide an alternative, compliant Toast
component.ID
.The removeToast
method has two arguments:
ID
of the toast to remove.The removeAllToasts
method has no arguments.
The updateToast
method has three arguments:
ID
of the toast to update.Options
object, which differs slightly from the add method because it accepts a content
property.ID
.The toastStack
is an array of objects representing the current toasts, e.g.
[
{
content: 'Something went wrong',
id: 'generated-string',
appearance: 'error',
},
{ content: 'Item saved', id: 'generated-string', appearance: 'success' },
];
To bring each toast notification inline with your app, you can provide alternative components to the ToastProvider
:
import { ToastProvider } from 'react-toast-notifications';
const MyCustomToast = ({ appearance, children }) => (
<div style={{ background: appearance === 'error' ? 'red' : 'green' }}>
{children}
</div>
);
const App = () => (
<ToastProvider components={{ Toast: MyCustomToast }}>...</ToastProvider>
);
To customize the existing component instead of creating a new one, you may import DefaultToast
:
import { DefaultToast } from 'react-toast-notifications';
export const MyCustomToast = ({ children, ...props }) => (
<DefaultToast {...props}>
<SomethingSpecial>{children}</SomethingSpecial>
</DefaultToast>
);
This library may not meet your needs. Here are some alternative I came across whilst searching for this solution:
FAQs
A configurable, composable, toast notification system for react.
The npm package react-toast-notifications receives a total of 67,089 weekly downloads. As such, react-toast-notifications popularity was classified as popular.
We found that react-toast-notifications demonstrated a not healthy version release cadence and project activity because the last version was released a year ago. It has 1 open source maintainer collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
Research
The Socket Research Team breaks down a malicious wrapper package that uses obfuscation to harvest credentials and exfiltrate sensitive data.
Research
Security News
Attackers used a malicious npm package typosquatting a popular ESLint plugin to steal sensitive data, execute commands, and exploit developer systems.
Security News
The Ultralytics' PyPI Package was compromised four times in one weekend through GitHub Actions cache poisoning and failure to rotate previously compromised API tokens.