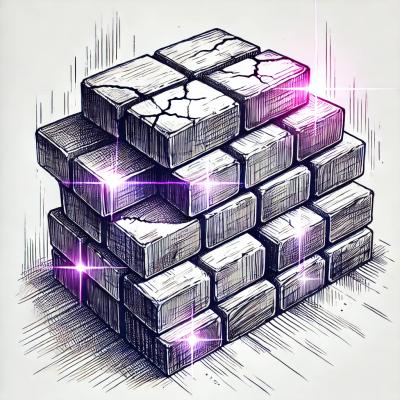
Security News
Input Validation Vulnerabilities Dominate MITRE's 2024 CWE Top 25 List
MITRE's 2024 CWE Top 25 highlights critical software vulnerabilities like XSS, SQL Injection, and CSRF, reflecting shifts due to a refined ranking methodology.
react-tooltip-controller
Advanced tools
This is a feature-rich React component for controlling tooltips. Not only for tooltips, but you can use it for various interaction requirements.
Visit the examples page to discover all the functionalities.
Basic Tooltip | Animated Tooltip | Advanced Tooltip |
---|---|---|
![]() | ![]() | ![]() |
click
, hover
, hover-hold
and hover-interactable
detection.npm install react-tooltip-controller
After installing the module, import it:
import ToolTipController from 'react-tooltip-controller'
<button className="random-classname">Click me</button>
<ToolTipController
select="random-classname"
detect="click"
offsetY={20}>
<TooltipComponent/>
</ToolTipController>
Anything wrapped by <ToolTipController>
is portalled to the bottom of the <body>
tag in the DOM.
You can either wrap a component or JSX Markup with <ToolTipController>
.
By referring to the className
property of the <button>
, <TooltipComponent/>
is attached to <button>
and set to be triggered by a click
action.
By default, the tooltip wrapped is positioned relative to the left-bottom of the selected element.
Props | Description | Possible Values | Default | Data Type |
---|---|---|---|---|
id | Assigns an ID number to the tooltip container class to be able to distinguish between multiple tooltips | E.g. "1" , "2" | null | String |
select | className of the element that will control the tooltip | - | - | String |
detect | Determines how to trigger the tooltip. Note that timeOut and id props should be defined in order to use the "hover-interactable” option | "click" ,"hover" ,"hover-hold" ,"hover-interactable” | "click" | String |
closeOnClick | Determines whether the tooltip closes when clicked on it | true , false | true | Boolean |
triggerClose | A Boolean value of true closes the tooltip manually | Boolean variable | - | Boolean |
returnState | Returns the state of the tooltip - If it’s open or not | Function | - | Function |
timeOut | Determines if the tooltip closes automatically after a certain amount of time in milliseconds. | positive integers | null | Integer |
offsetX | Determines the offset along the X axis relative to left bottom of the element | positive/negative integers | 0 | Integer |
offsetY | Determines the offset along the Y axis relative to left bottom of the element. If set to "center" along with width prop, automatically aligns to middle of the triggering element | positive/negative integers or "center" | 0 | Integer, String |
width | Width value of the tooltip Required for offsetX = "center" | positive integers | null | Integer |
animation | Determines the name of the animation class | - | null | String |
duration | Determines the duration of the animation in units of milliseconds(ms) or seconds(s) | E.g. "500ms" or "0.5s" | null | String |
timing | Determines the timing function of the animation. You can use standard CSS timing functions such as "linear" , "ease" or can define a specific Cubic Bezier curve | E.g. "linear" or "ease" | null | String |
properties | Determines the properties to be animated. Can be a string or an array of strings | E.g. "all" or ["opacity", "transform"] | [ ] | String, Array |
import React, { Component } from 'react'
import ToolTipController from 'react-tooltip-controller'
import './styles/main.css'
const ToolTip = () =>
<div className="toolTip">
Tooltip
</div>
class Example extends Component {
render() {
return (
<div className="App">
<button className="btn">Hello There</button>
<ToolTipController
select="btn"
detect="hover">
<ToolTip/>
</ToolTipController>
</div>
)
}
}
export default Example
Stylus File
.react-tooltip-1
opacity: 0
transform: translateY(10px)
&.fadeIn
opacity: 1
transform: translateY(0)
JSX file
import React, { Component } from 'react'
import ToolTipController from 'react-tooltip-controller'
import './styles/main.css'
const ToolTip = () =>
<div className="toolTip">
Tooltip
</div>
class Example extends Component {
render() {
return (
<div className="App">
<button className="btn">Hello There</button>
<ToolTipController
id="1"
select="btn"
detect="hover"
animation="fadeIn"
duration=".3s"
timing="ease"
properties={["opacity", "transform"]}>
<ToolTip/>
</ToolTipController>
</div>
)
}
}
export default Example
Note that react-tooltip
is a built-in class name and since the id
prop is set to "1"
, we refer to this tooltip with the specific class name of react-tooltip-1
.
Always set the id
prop for the animated tooltips in order to prevent any class name conflicts.
Side note: If you don't set the properties
prop, all the properties for the tooltip animates. This is prominent when you resize the browser window as the tooltip is open.
triggerClose
propimport React, { Component } from 'react'
import ToolTipController from 'react-tooltip-controller'
import './styles/main.css'
const ToolTip = (props) =>
<div className="toolTip">
Tooltip
<button onClick={null}></button>
<button onClick={props.closeTriggerFunction}></button>
</div>
class Example extends Component {
state = {
trigger: false
}
close = () => {
this.setState({trigger: true})
}
render() {
return (
<div className="App">
<button className="btn">Hello There</button>
<ToolTipController
select="btn"
detect="click"
closeOnClick={false}
triggerClose={this.state.trigger}>
<ToolTip closeTriggerFunction={this.close}/>
</ToolTipController>
</div>
)
}
}
export default Example
By using the triggerClose
prop, the tooltip can be closed manually. To do so, variable passed to triggerClose
prop should be set to true
.
This example demonstrates how to close the tooltip by setting the state of the triggering variable to true
. To prevent the other click actions on the tooltip from closing it, closeOnClick
is set to false
. Note that clicking outside of the tooltip closes it independent of the triggerClose
prop.
returnState
propimport React, { Component } from 'react'
import ToolTipController from 'react-tooltip-controller'
import './styles/main.css'
const ToolTip = (props) =>
<div className="toolTip">
Tooltip
</div>
class Example extends Component {
state = {
tooltipState: false
}
getTooltipState = (data) => {
this.setState({tooltipState: data})
}
render() {
return (
<div className="App">
<button className="btn">Hello There</button>
<ToolTipController
select="btn"
detect="hover"
returnState={this.getTooltipState}>
<ToolTip/>
</ToolTipController>
</div>
)
}
}
export default Example
You can pass a function as a prop through returnState
in order to get the state of the tooltip, whether it's open or not.
MIT License
FAQs
A feature-rich React component for controlling tooltips / pop-up menus
The npm package react-tooltip-controller receives a total of 7 weekly downloads. As such, react-tooltip-controller popularity was classified as not popular.
We found that react-tooltip-controller demonstrated a not healthy version release cadence and project activity because the last version was released a year ago. It has 1 open source maintainer collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
MITRE's 2024 CWE Top 25 highlights critical software vulnerabilities like XSS, SQL Injection, and CSRF, reflecting shifts due to a refined ranking methodology.
Security News
In this segment of the Risky Business podcast, Feross Aboukhadijeh and Patrick Gray discuss the challenges of tracking malware discovered in open source softare.
Research
Security News
A threat actor's playbook for exploiting the npm ecosystem was exposed on the dark web, detailing how to build a blockchain-powered botnet.