react-ultimate-pagination
Advanced tools
Comparing version
@@ -130,73 +130,45 @@ (function webpackUniversalModuleDefinition(root, factory) { | ||
'use strict'; | ||
Object.defineProperty(exports, "__esModule", { | ||
value: true | ||
}); | ||
exports.ITEM_KEYS = exports.ITEM_TYPES = undefined; | ||
exports.getPaginationModel = getPaginationModel; | ||
var _ultimatePaginationConstants = __webpack_require__(3); | ||
Object.defineProperty(exports, 'ITEM_TYPES', { | ||
enumerable: true, | ||
get: function get() { | ||
return _ultimatePaginationConstants.ITEM_TYPES; | ||
} | ||
}); | ||
Object.defineProperty(exports, 'ITEM_KEYS', { | ||
enumerable: true, | ||
get: function get() { | ||
return _ultimatePaginationConstants.ITEM_KEYS; | ||
} | ||
}); | ||
var _ultimatePaginationUtils = __webpack_require__(4); | ||
var _ultimatePaginationItemFactories = __webpack_require__(5); | ||
function _toConsumableArray(arr) { if (Array.isArray(arr)) { for (var i = 0, arr2 = Array(arr.length); i < arr.length; i++) { arr2[i] = arr[i]; } return arr2; } else { return Array.from(arr); } } | ||
"use strict"; | ||
var ultimate_pagination_utils_1 = __webpack_require__(3); | ||
var ultimate_pagination_item_factories_1 = __webpack_require__(4); | ||
function getPaginationModel(options) { | ||
var currentPage = options.currentPage; | ||
var totalPages = options.totalPages; | ||
var paginationModel = []; | ||
var createPage = (0, _ultimatePaginationItemFactories.createPageFunctionFactory)(options); | ||
// Calculate group of central pages | ||
var mainPagesStart = Math.max(currentPage - 1, 3) - Math.max(0, currentPage + 3 - totalPages); | ||
var mainPagesEnd = Math.min(currentPage + 1, totalPages - 2) + Math.max(0, 4 - currentPage); | ||
var mainPages = (0, _ultimatePaginationUtils.createRange)(mainPagesStart, mainPagesEnd).map(createPage); | ||
paginationModel.push((0, _ultimatePaginationItemFactories.createFirstPageLink)(options)); | ||
paginationModel.push((0, _ultimatePaginationItemFactories.createPreviousPageLink)(options)); | ||
// Always add the first page | ||
paginationModel.push(createPage(1)); | ||
// Show '...' or second page between the last page and main pages group if needed | ||
if (mainPagesStart > 3) { | ||
paginationModel.push((0, _ultimatePaginationItemFactories.createFirstEllipsis)(mainPagesStart - 1)); | ||
} else { | ||
paginationModel.push(createPage(2)); | ||
} | ||
// Add pages +/- from the current page | ||
paginationModel.push.apply(paginationModel, _toConsumableArray(mainPages)); | ||
// Show '...' or penult page between main pages group and the last page if needed | ||
if (mainPagesEnd < totalPages - 2) { | ||
paginationModel.push((0, _ultimatePaginationItemFactories.createSecondEllipsis)(mainPagesEnd + 1)); | ||
} else { | ||
paginationModel.push(createPage(totalPages - 1)); | ||
} | ||
// Always add the last page | ||
paginationModel.push(createPage(totalPages)); | ||
paginationModel.push((0, _ultimatePaginationItemFactories.createNextPageLink)(options)); | ||
paginationModel.push((0, _ultimatePaginationItemFactories.createLastPageLink)(options)); | ||
return paginationModel; | ||
var currentPage = options.currentPage, totalPages = options.totalPages; | ||
var paginationModel = []; | ||
var createPage = ultimate_pagination_item_factories_1.createPageFunctionFactory(options); | ||
// Calculate group of central pages | ||
var mainPagesStart = Math.max(2, Math.max(currentPage - 1, 3) - Math.max(0, currentPage + 3 - totalPages)); | ||
var mainPagesEnd = Math.min(totalPages - 1, Math.min(currentPage + 1, totalPages - 2) + Math.max(0, 4 - currentPage)); | ||
var mainPages = ultimate_pagination_utils_1.createRange(mainPagesStart, mainPagesEnd).map(createPage); | ||
paginationModel.push(ultimate_pagination_item_factories_1.createFirstPageLink(options)); | ||
paginationModel.push(ultimate_pagination_item_factories_1.createPreviousPageLink(options)); | ||
// Always add the first page | ||
paginationModel.push(createPage(1)); | ||
// Show '...' or second page between the last page and main pages group if needed | ||
if (mainPagesStart > 3) { | ||
paginationModel.push(ultimate_pagination_item_factories_1.createFirstEllipsis(mainPagesStart - 1)); | ||
} | ||
else if (mainPagesStart !== 2) { | ||
paginationModel.push(createPage(2)); | ||
} | ||
// Add pages +/- from the current page | ||
paginationModel.push.apply(paginationModel, mainPages); | ||
// Show '...' or penult page between main pages group and the last page if needed | ||
if (mainPagesEnd < totalPages - 2) { | ||
paginationModel.push(ultimate_pagination_item_factories_1.createSecondEllipsis(mainPagesEnd + 1)); | ||
} | ||
else if (mainPagesEnd !== totalPages - 1) { | ||
paginationModel.push(createPage(totalPages - 1)); | ||
} | ||
if (totalPages > 1) { | ||
paginationModel.push(createPage(totalPages)); | ||
} | ||
paginationModel.push(ultimate_pagination_item_factories_1.createNextPageLink(options)); | ||
paginationModel.push(ultimate_pagination_item_factories_1.createLastPageLink(options)); | ||
return paginationModel; | ||
} | ||
exports.getPaginationModel = getPaginationModel; | ||
var ultimate_pagination_constants_1 = __webpack_require__(5); | ||
exports.ITEM_TYPES = ultimate_pagination_constants_1.ITEM_TYPES; | ||
exports.ITEM_KEYS = ultimate_pagination_constants_1.ITEM_KEYS; | ||
//# sourceMappingURL=ultimate-pagination.js.map | ||
@@ -207,134 +179,107 @@ /***/ }, | ||
'use strict'; | ||
Object.defineProperty(exports, "__esModule", { | ||
value: true | ||
}); | ||
var ITEM_TYPES = exports.ITEM_TYPES = { | ||
PAGE: 'PAGE', | ||
ELLIPSIS: 'ELLIPSIS', | ||
FIRST_PAGE_LINK: 'FIRST_PAGE_LINK', | ||
PREVIOS_PAGE_LINK: 'PREVIOS_PAGE_LINK', | ||
NEXT_PAGE_LINK: 'NEXT_PAGE_LINK', | ||
LAST_PAGE_LINK: 'LAST_PAGE_LINK' | ||
}; | ||
var ITEM_KEYS = exports.ITEM_KEYS = { | ||
FIRST_ELLIPSIS: -1, | ||
SECOND_ELLISPIS: -2, | ||
FIRST_PAGE_LINK: -3, | ||
PREVIOS_PAGE_LINK: -4, | ||
NEXT_PAGE_LINK: -5, | ||
LAST_PAGE_LINK: -6 | ||
}; | ||
/***/ }, | ||
/* 4 */ | ||
/***/ function(module, exports) { | ||
"use strict"; | ||
Object.defineProperty(exports, "__esModule", { | ||
value: true | ||
}); | ||
exports.createRange = createRange; | ||
function createRange(start, end) { | ||
var range = []; | ||
for (var i = start; i <= end; i++) { | ||
range.push(i); | ||
} | ||
return range; | ||
var range = []; | ||
for (var i = start; i <= end; i++) { | ||
range.push(i); | ||
} | ||
return range; | ||
} | ||
exports.createRange = createRange; | ||
//# sourceMappingURL=ultimate-pagination-utils.js.map | ||
/***/ }, | ||
/* 5 */ | ||
/* 4 */ | ||
/***/ function(module, exports, __webpack_require__) { | ||
'use strict'; | ||
Object.defineProperty(exports, "__esModule", { | ||
value: true | ||
}); | ||
exports.createPageFunctionFactory = exports.createLastPageLink = exports.createNextPageLink = exports.createPreviousPageLink = exports.createFirstPageLink = exports.createSecondEllipsis = exports.createFirstEllipsis = undefined; | ||
var _ultimatePaginationConstants = __webpack_require__(3); | ||
var createFirstEllipsis = exports.createFirstEllipsis = function createFirstEllipsis(value) { | ||
return { | ||
type: _ultimatePaginationConstants.ITEM_TYPES.ELLIPSIS, | ||
key: _ultimatePaginationConstants.ITEM_KEYS.FIRST_ELLIPSIS, | ||
value: value, | ||
isActive: false | ||
}; | ||
"use strict"; | ||
var ultimate_pagination_constants_1 = __webpack_require__(5); | ||
exports.createFirstEllipsis = function (pageNumber) { | ||
return { | ||
type: ultimate_pagination_constants_1.ITEM_TYPES.ELLIPSIS, | ||
key: ultimate_pagination_constants_1.ITEM_KEYS.FIRST_ELLIPSIS, | ||
value: pageNumber, | ||
isActive: false | ||
}; | ||
}; | ||
var createSecondEllipsis = exports.createSecondEllipsis = function createSecondEllipsis(value) { | ||
return { | ||
type: _ultimatePaginationConstants.ITEM_TYPES.ELLIPSIS, | ||
key: _ultimatePaginationConstants.ITEM_KEYS.SECOND_ELLISPIS, | ||
value: value, | ||
isActive: false | ||
}; | ||
exports.createSecondEllipsis = function (pageNumber) { | ||
return { | ||
type: ultimate_pagination_constants_1.ITEM_TYPES.ELLIPSIS, | ||
key: ultimate_pagination_constants_1.ITEM_KEYS.SECOND_ELLISPIS, | ||
value: pageNumber, | ||
isActive: false | ||
}; | ||
}; | ||
var createFirstPageLink = exports.createFirstPageLink = function createFirstPageLink(_ref) { | ||
var currentPage = _ref.currentPage; | ||
return { | ||
type: _ultimatePaginationConstants.ITEM_TYPES.FIRST_PAGE_LINK, | ||
key: _ultimatePaginationConstants.ITEM_KEYS.FIRST_PAGE_LINK, | ||
value: 1, | ||
isActive: currentPage === 1 | ||
}; | ||
exports.createFirstPageLink = function (options) { | ||
var currentPage = options.currentPage; | ||
return { | ||
type: ultimate_pagination_constants_1.ITEM_TYPES.FIRST_PAGE_LINK, | ||
key: ultimate_pagination_constants_1.ITEM_KEYS.FIRST_PAGE_LINK, | ||
value: 1, | ||
isActive: currentPage === 1 | ||
}; | ||
}; | ||
var createPreviousPageLink = exports.createPreviousPageLink = function createPreviousPageLink(_ref2) { | ||
var currentPage = _ref2.currentPage; | ||
var totalPages = _ref2.totalPages; | ||
return { | ||
type: _ultimatePaginationConstants.ITEM_TYPES.PREVIOS_PAGE_LINK, | ||
key: _ultimatePaginationConstants.ITEM_KEYS.PREVIOS_PAGE_LINK, | ||
value: Math.max(1, currentPage - 1), | ||
isActive: currentPage === 1 | ||
}; | ||
exports.createPreviousPageLink = function (options) { | ||
var currentPage = options.currentPage; | ||
return { | ||
type: ultimate_pagination_constants_1.ITEM_TYPES.PREVIOS_PAGE_LINK, | ||
key: ultimate_pagination_constants_1.ITEM_KEYS.PREVIOS_PAGE_LINK, | ||
value: Math.max(1, currentPage - 1), | ||
isActive: currentPage === 1 | ||
}; | ||
}; | ||
var createNextPageLink = exports.createNextPageLink = function createNextPageLink(_ref3) { | ||
var currentPage = _ref3.currentPage; | ||
var totalPages = _ref3.totalPages; | ||
return { | ||
type: _ultimatePaginationConstants.ITEM_TYPES.NEXT_PAGE_LINK, | ||
key: _ultimatePaginationConstants.ITEM_KEYS.NEXT_PAGE_LINK, | ||
value: Math.min(totalPages, currentPage + 1), | ||
isActive: currentPage === totalPages | ||
}; | ||
exports.createNextPageLink = function (options) { | ||
var currentPage = options.currentPage, totalPages = options.totalPages; | ||
return { | ||
type: ultimate_pagination_constants_1.ITEM_TYPES.NEXT_PAGE_LINK, | ||
key: ultimate_pagination_constants_1.ITEM_KEYS.NEXT_PAGE_LINK, | ||
value: Math.min(totalPages, currentPage + 1), | ||
isActive: currentPage === totalPages | ||
}; | ||
}; | ||
var createLastPageLink = exports.createLastPageLink = function createLastPageLink(_ref4) { | ||
var currentPage = _ref4.currentPage; | ||
var totalPages = _ref4.totalPages; | ||
return { | ||
type: _ultimatePaginationConstants.ITEM_TYPES.LAST_PAGE_LINK, | ||
key: _ultimatePaginationConstants.ITEM_KEYS.LAST_PAGE_LINK, | ||
value: totalPages, | ||
isActive: currentPage === totalPages | ||
}; | ||
}; | ||
var createPageFunctionFactory = exports.createPageFunctionFactory = function createPageFunctionFactory(_ref5) { | ||
var currentPage = _ref5.currentPage; | ||
return function (pageNumber) { | ||
exports.createLastPageLink = function (options) { | ||
var currentPage = options.currentPage, totalPages = options.totalPages; | ||
return { | ||
type: _ultimatePaginationConstants.ITEM_TYPES.PAGE, | ||
key: pageNumber, | ||
value: pageNumber, | ||
isActive: pageNumber === currentPage | ||
type: ultimate_pagination_constants_1.ITEM_TYPES.LAST_PAGE_LINK, | ||
key: ultimate_pagination_constants_1.ITEM_KEYS.LAST_PAGE_LINK, | ||
value: totalPages, | ||
isActive: currentPage === totalPages | ||
}; | ||
}; | ||
}; | ||
exports.createPageFunctionFactory = function (options) { | ||
var currentPage = options.currentPage; | ||
return function (pageNumber) { | ||
return { | ||
type: ultimate_pagination_constants_1.ITEM_TYPES.PAGE, | ||
key: pageNumber, | ||
value: pageNumber, | ||
isActive: pageNumber === currentPage | ||
}; | ||
}; | ||
}; | ||
//# sourceMappingURL=ultimate-pagination-item-factories.js.map | ||
/***/ }, | ||
/* 5 */ | ||
/***/ function(module, exports) { | ||
"use strict"; | ||
exports.ITEM_TYPES = { | ||
PAGE: 'PAGE', | ||
ELLIPSIS: 'ELLIPSIS', | ||
FIRST_PAGE_LINK: 'FIRST_PAGE_LINK', | ||
PREVIOS_PAGE_LINK: 'PREVIOS_PAGE_LINK', | ||
NEXT_PAGE_LINK: 'NEXT_PAGE_LINK', | ||
LAST_PAGE_LINK: 'LAST_PAGE_LINK' | ||
}; | ||
exports.ITEM_KEYS = { | ||
FIRST_ELLIPSIS: -1, | ||
SECOND_ELLISPIS: -2, | ||
FIRST_PAGE_LINK: -3, | ||
PREVIOS_PAGE_LINK: -4, | ||
NEXT_PAGE_LINK: -5, | ||
LAST_PAGE_LINK: -6 | ||
}; | ||
//# sourceMappingURL=ultimate-pagination-constants.js.map | ||
/***/ } | ||
@@ -341,0 +286,0 @@ /******/ ]) |
@@ -1,2 +0,2 @@ | ||
!function(e,t){"object"==typeof exports&&"object"==typeof module?module.exports=t(require("react")):"function"==typeof define&&define.amd?define(["react"],t):"object"==typeof exports?exports.reactUltimatePagination=t(require("react")):e.reactUltimatePagination=t(e.React)}(this,function(e){return function(e){function t(n){if(r[n])return r[n].exports;var a=r[n]={exports:{},id:n,loaded:!1};return e[n].call(a.exports,a,a.exports,t),a.loaded=!0,a.exports}var r={};return t.m=e,t.c=r,t.p="",t(0)}([function(e,t,r){"use strict";function n(e){return e&&e.__esModule?e:{"default":e}}Object.defineProperty(t,"__esModule",{value:!0}),t.ITEM_TYPES=t.createUltimatePagination=void 0;var a=Object.assign||function(e){for(var t=1;t<arguments.length;t++){var r=arguments[t];for(var n in r)Object.prototype.hasOwnProperty.call(r,n)&&(e[n]=r[n])}return e},i=r(5),u=n(i),o=r(4),c=function(e,t,r){var n=function(e){return function(){r&&t!==e&&r(e)}};return function(t){var r=e[t.type],i=n(t.value);return u["default"].createElement(r,a({onClick:i},t))}};t.createUltimatePagination=function(e){var t=e.itemTypeToComponent,r=e.WrapperComponent,n=void 0===r?"div":r,a=function(e){var r=e.currentPage,a=e.totalPages,i=e.onChange,P=(0,o.getPaginationModel)({currentPage:r,totalPages:a}),E=c(t,r,i);return u["default"].createElement(n,null,P.map(E))};return a.propTypes={currentPage:u["default"].PropTypes.number.isRequired,totalPages:u["default"].PropTypes.number.isRequired,onChange:u["default"].PropTypes.func},a};t.ITEM_TYPES=o.ITEM_TYPES},function(e,t){"use strict";Object.defineProperty(t,"__esModule",{value:!0});t.ITEM_TYPES={PAGE:"PAGE",ELLIPSIS:"ELLIPSIS",FIRST_PAGE_LINK:"FIRST_PAGE_LINK",PREVIOS_PAGE_LINK:"PREVIOS_PAGE_LINK",NEXT_PAGE_LINK:"NEXT_PAGE_LINK",LAST_PAGE_LINK:"LAST_PAGE_LINK"},t.ITEM_KEYS={FIRST_ELLIPSIS:-1,SECOND_ELLISPIS:-2,FIRST_PAGE_LINK:-3,PREVIOS_PAGE_LINK:-4,NEXT_PAGE_LINK:-5,LAST_PAGE_LINK:-6}},function(e,t,r){"use strict";Object.defineProperty(t,"__esModule",{value:!0}),t.createPageFunctionFactory=t.createLastPageLink=t.createNextPageLink=t.createPreviousPageLink=t.createFirstPageLink=t.createSecondEllipsis=t.createFirstEllipsis=void 0;var n=r(1);t.createFirstEllipsis=function(e){return{type:n.ITEM_TYPES.ELLIPSIS,key:n.ITEM_KEYS.FIRST_ELLIPSIS,value:e,isActive:!1}},t.createSecondEllipsis=function(e){return{type:n.ITEM_TYPES.ELLIPSIS,key:n.ITEM_KEYS.SECOND_ELLISPIS,value:e,isActive:!1}},t.createFirstPageLink=function(e){var t=e.currentPage;return{type:n.ITEM_TYPES.FIRST_PAGE_LINK,key:n.ITEM_KEYS.FIRST_PAGE_LINK,value:1,isActive:1===t}},t.createPreviousPageLink=function(e){var t=e.currentPage;e.totalPages;return{type:n.ITEM_TYPES.PREVIOS_PAGE_LINK,key:n.ITEM_KEYS.PREVIOS_PAGE_LINK,value:Math.max(1,t-1),isActive:1===t}},t.createNextPageLink=function(e){var t=e.currentPage,r=e.totalPages;return{type:n.ITEM_TYPES.NEXT_PAGE_LINK,key:n.ITEM_KEYS.NEXT_PAGE_LINK,value:Math.min(r,t+1),isActive:t===r}},t.createLastPageLink=function(e){var t=e.currentPage,r=e.totalPages;return{type:n.ITEM_TYPES.LAST_PAGE_LINK,key:n.ITEM_KEYS.LAST_PAGE_LINK,value:r,isActive:t===r}},t.createPageFunctionFactory=function(e){var t=e.currentPage;return function(e){return{type:n.ITEM_TYPES.PAGE,key:e,value:e,isActive:e===t}}}},function(e,t){"use strict";function r(e,t){for(var r=[],n=e;t>=n;n++)r.push(n);return r}Object.defineProperty(t,"__esModule",{value:!0}),t.createRange=r},function(e,t,r){"use strict";function n(e){if(Array.isArray(e)){for(var t=0,r=Array(e.length);t<e.length;t++)r[t]=e[t];return r}return Array.from(e)}function a(e){var t=e.currentPage,r=e.totalPages,a=[],i=(0,o.createPageFunctionFactory)(e),c=Math.max(t-1,3)-Math.max(0,t+3-r),P=Math.min(t+1,r-2)+Math.max(0,4-t),E=(0,u.createRange)(c,P).map(i);return a.push((0,o.createFirstPageLink)(e)),a.push((0,o.createPreviousPageLink)(e)),a.push(i(1)),c>3?a.push((0,o.createFirstEllipsis)(c-1)):a.push(i(2)),a.push.apply(a,n(E)),r-2>P?a.push((0,o.createSecondEllipsis)(P+1)):a.push(i(r-1)),a.push(i(r)),a.push((0,o.createNextPageLink)(e)),a.push((0,o.createLastPageLink)(e)),a}Object.defineProperty(t,"__esModule",{value:!0}),t.ITEM_KEYS=t.ITEM_TYPES=void 0,t.getPaginationModel=a;var i=r(1);Object.defineProperty(t,"ITEM_TYPES",{enumerable:!0,get:function(){return i.ITEM_TYPES}}),Object.defineProperty(t,"ITEM_KEYS",{enumerable:!0,get:function(){return i.ITEM_KEYS}});var u=r(3),o=r(2)},function(t,r){t.exports=e}])}); | ||
!function(e,t){"object"==typeof exports&&"object"==typeof module?module.exports=t(require("react")):"function"==typeof define&&define.amd?define(["react"],t):"object"==typeof exports?exports.reactUltimatePagination=t(require("react")):e.reactUltimatePagination=t(e.React)}(this,function(e){return function(e){function t(n){if(r[n])return r[n].exports;var a=r[n]={exports:{},id:n,loaded:!1};return e[n].call(a.exports,a,a.exports,t),a.loaded=!0,a.exports}var r={};return t.m=e,t.c=r,t.p="",t(0)}([function(e,t,r){"use strict";function n(e){return e&&e.__esModule?e:{"default":e}}Object.defineProperty(t,"__esModule",{value:!0}),t.ITEM_TYPES=t.createUltimatePagination=void 0;var a=Object.assign||function(e){for(var t=1;t<arguments.length;t++){var r=arguments[t];for(var n in r)Object.prototype.hasOwnProperty.call(r,n)&&(e[n]=r[n])}return e},i=r(5),u=n(i),o=r(4),c=function(e,t,r){var n=function(e){return function(){r&&t!==e&&r(e)}};return function(t){var r=e[t.type],i=n(t.value);return u["default"].createElement(r,a({onClick:i},t))}};t.createUltimatePagination=function(e){var t=e.itemTypeToComponent,r=e.WrapperComponent,n=void 0===r?"div":r,a=function(e){var r=e.currentPage,a=e.totalPages,i=e.onChange,E=(0,o.getPaginationModel)({currentPage:r,totalPages:a}),P=c(t,r,i);return u["default"].createElement(n,null,E.map(P))};return a.propTypes={currentPage:u["default"].PropTypes.number.isRequired,totalPages:u["default"].PropTypes.number.isRequired,onChange:u["default"].PropTypes.func},a};t.ITEM_TYPES=o.ITEM_TYPES},function(e,t){"use strict";t.ITEM_TYPES={PAGE:"PAGE",ELLIPSIS:"ELLIPSIS",FIRST_PAGE_LINK:"FIRST_PAGE_LINK",PREVIOS_PAGE_LINK:"PREVIOS_PAGE_LINK",NEXT_PAGE_LINK:"NEXT_PAGE_LINK",LAST_PAGE_LINK:"LAST_PAGE_LINK"},t.ITEM_KEYS={FIRST_ELLIPSIS:-1,SECOND_ELLISPIS:-2,FIRST_PAGE_LINK:-3,PREVIOS_PAGE_LINK:-4,NEXT_PAGE_LINK:-5,LAST_PAGE_LINK:-6}},function(e,t,r){"use strict";var n=r(1);t.createFirstEllipsis=function(e){return{type:n.ITEM_TYPES.ELLIPSIS,key:n.ITEM_KEYS.FIRST_ELLIPSIS,value:e,isActive:!1}},t.createSecondEllipsis=function(e){return{type:n.ITEM_TYPES.ELLIPSIS,key:n.ITEM_KEYS.SECOND_ELLISPIS,value:e,isActive:!1}},t.createFirstPageLink=function(e){var t=e.currentPage;return{type:n.ITEM_TYPES.FIRST_PAGE_LINK,key:n.ITEM_KEYS.FIRST_PAGE_LINK,value:1,isActive:1===t}},t.createPreviousPageLink=function(e){var t=e.currentPage;return{type:n.ITEM_TYPES.PREVIOS_PAGE_LINK,key:n.ITEM_KEYS.PREVIOS_PAGE_LINK,value:Math.max(1,t-1),isActive:1===t}},t.createNextPageLink=function(e){var t=e.currentPage,r=e.totalPages;return{type:n.ITEM_TYPES.NEXT_PAGE_LINK,key:n.ITEM_KEYS.NEXT_PAGE_LINK,value:Math.min(r,t+1),isActive:t===r}},t.createLastPageLink=function(e){var t=e.currentPage,r=e.totalPages;return{type:n.ITEM_TYPES.LAST_PAGE_LINK,key:n.ITEM_KEYS.LAST_PAGE_LINK,value:r,isActive:t===r}},t.createPageFunctionFactory=function(e){var t=e.currentPage;return function(e){return{type:n.ITEM_TYPES.PAGE,key:e,value:e,isActive:e===t}}}},function(e,t){"use strict";function r(e,t){for(var r=[],n=e;t>=n;n++)r.push(n);return r}t.createRange=r},function(e,t,r){"use strict";function n(e){var t=e.currentPage,r=e.totalPages,n=[],u=i.createPageFunctionFactory(e),o=Math.max(2,Math.max(t-1,3)-Math.max(0,t+3-r)),c=Math.min(r-1,Math.min(t+1,r-2)+Math.max(0,4-t)),E=a.createRange(o,c).map(u);return n.push(i.createFirstPageLink(e)),n.push(i.createPreviousPageLink(e)),n.push(u(1)),o>3?n.push(i.createFirstEllipsis(o-1)):2!==o&&n.push(u(2)),n.push.apply(n,E),r-2>c?n.push(i.createSecondEllipsis(c+1)):c!==r-1&&n.push(u(r-1)),r>1&&n.push(u(r)),n.push(i.createNextPageLink(e)),n.push(i.createLastPageLink(e)),n}var a=r(3),i=r(2);t.getPaginationModel=n;var u=r(1);t.ITEM_TYPES=u.ITEM_TYPES,t.ITEM_KEYS=u.ITEM_KEYS},function(t,r){t.exports=e}])}); | ||
//# sourceMappingURL=react-ultimate-pagination.min.js.map |
{ | ||
"name": "react-ultimate-pagination", | ||
"version": "0.7.4", | ||
"version": "0.8.0", | ||
"description": "React.js pagination component based on ultimate-pagination", | ||
@@ -39,3 +39,3 @@ "main": "lib/react-ultimate-pagination.js", | ||
"react-dom": "^15.0.1", | ||
"ultimate-pagination": "0.5.0" | ||
"ultimate-pagination": "0.7.0" | ||
}, | ||
@@ -42,0 +42,0 @@ "devDependencies": { |
@@ -15,4 +15,6 @@ [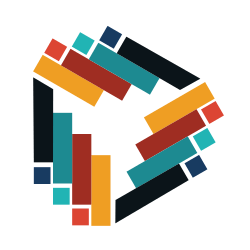](https://github.com/ultimate-pagination/react-ultimate-pagination) | ||
- [Basic Theme](https://github.com/ultimate-pagination/react-ultimate-pagination-basic) | ||
- [Material UI Theme](https://github.com/ultimate-pagination/react-ultimate-pagination-material-ui) | ||
- [Basic Theme](https://github.com/ultimate-pagination/react-ultimate-pagination-basic) ([Demo](http://codepen.io/dmytroyarmak/full/GZwKZJ)) | ||
- [Bootstrap 3 Theme](https://github.com/ultimate-pagination/react-ultimate-pagination-bootstrap-3) ([Demo](http://codepen.io/dmytroyarmak/full/YqBQYw/)) | ||
- [Bootstrap 4 Theme](https://github.com/ultimate-pagination/react-ultimate-pagination-bootstrap-4) ([Demo](http://codepen.io/dmytroyarmak/full/VagMQq/)) | ||
- [Material UI Theme](https://github.com/ultimate-pagination/react-ultimate-pagination-material-ui) ([Demo](http://ultimate-pagination.github.io/react-ultimate-pagination-examples/)) | ||
@@ -19,0 +21,0 @@ ## Installation |
Sorry, the diff of this file is not supported yet
Sorry, the diff of this file is not supported yet
111
1.83%63881
-6.11%322
-6.94%+ Added
- Removed
Updated