React File Upload Widget
(With Integrated Cloud Storage)
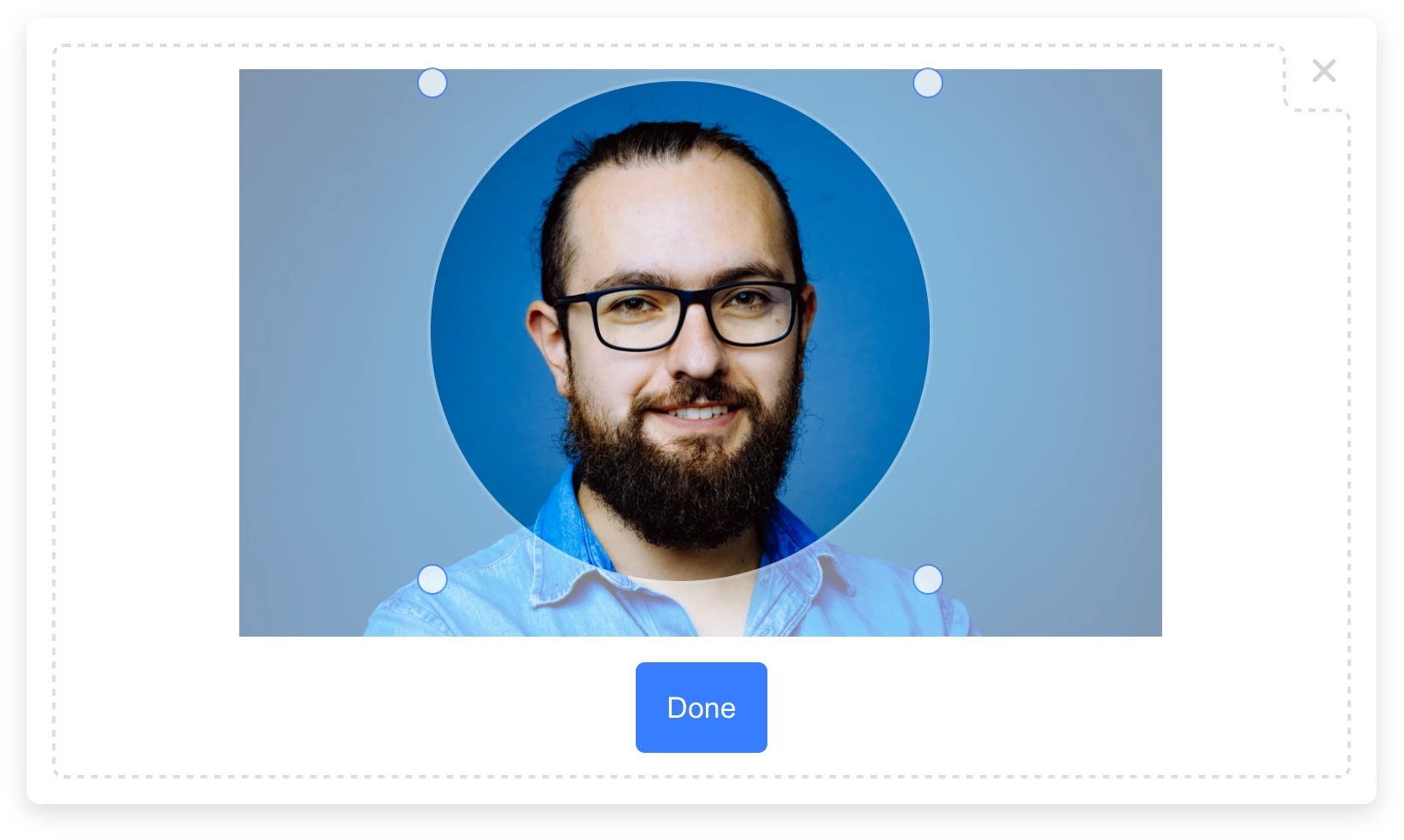
100% Serverless File Upload Widget
Powered by Upload.io
DMCA Compliant โข GDPR Compliant โข 99.9% Uptime SLA
Supports: Rate Limiting, Volume Limiting, File Size & Type Limiting, JWT Auth, and more...
Installation
Install via NPM:
npm install react-uploader
Or via YARN:
yarn add react-uploader
Or via a <script>
tag:
<script src="https://js.upload.io/react-uploader/v3"></script>
Usage
The UploadButton
component uses a render prop to provide an onClick
callback to your button element.
When clicked, a file upload modal will appear:
import { Uploader } from "uploader";
import { UploadButton } from "react-uploader";
const uploader = Uploader({
apiKey: "free"
});
const options = { multi: true };
const MyApp = () => (
<UploadButton uploader={uploader}
options={options}
onComplete={files => alert(files.map(x => x.fileUrl).join("\n"))}>
{({onClick}) =>
<button onClick={onClick}>
Upload a file...
</button>
}
</UploadButton>
);
Required props:
Optional props:
The UploadDropzone
component renders an inline drag-and-drop file upload dropzone:
import { Uploader } from "uploader";
import { UploadButton } from "react-uploader";
const uploader = Uploader({
apiKey: "free"
});
const options = { multi: true };
const MyApp = () => (
<UploadDropzone uploader={uploader}
options={options}
onUpdate={files => alert(files.map(x => x.fileUrl).join("\n"))}
width="600px"
height="375px" />
);
Required props:
Optional props:
options
onUpdate
width
height
The Result
The callbacks receive a Array<UploadWidgetResult>
:
{
fileUrl: "https://upcdn.io/FW25...",
filePath: "/uploads/example.jpg",
editedFile: undefined,
originalFile: {
fileUrl: "https://upcdn.io/FW25...",
filePath: "/uploads/example.jpg",
accountId: "FW251aX",
originalFileName: "example.jpg",
file: { ... },
size: 12345,
lastModified: 1663410542397,
mime: "image/jpeg",
metadata: {
...
},
tags: [
"tag1",
"tag2",
...
]
}
}
Image Processing (Resize, Crop, etc.)
The Upload.io platform includes a built-in Image Processing API, which supports the following:
Original Image
Here's an example using a photo of Chicago:
https://upcdn.io/W142hJk/raw/example/city-landscape.jpg
Processed Image
You can use the Image Processing API to convert the above photo into this processed image:
https://upcdn.io/W142hJk/image/example/city-landscape.jpg
?w=900
&h=600
&fit=crop
&f=webp
&q=80
&blur=4
&text=WATERMARK
&layer-opacity=80
&blend=overlay
&layer-rotate=315
&font-size=100
&padding=10
&font-weight=900
&color=ffffff
&repeat=true
&text=Chicago
&gravity=bottom
&padding-x=50
&padding-bottom=20
&font=/example/fonts/Lobster.ttf
&color=ffe400
Full Documentation
React Uploader Documentation ยป
Need a Headless (no UI) File Upload Library?
Try Upload.js ยป
Can I use my own storage?
Yes! Upload.io supports custom S3 buckets on Upload Plus plans.
For ease and simplicity, your files are stored in Upload.io's internal S3 buckets by default. You can change this on a folder-by-folder basis โ to use your existing S3 bucket(s) โ in the Upload Dashboard.
๐ Create your Upload.io Account
React Uploader is the React file upload component for Upload.io: the file upload service for web apps.
Create an Upload.io account ยป
Building From Source
BUILD.md
License
MIT