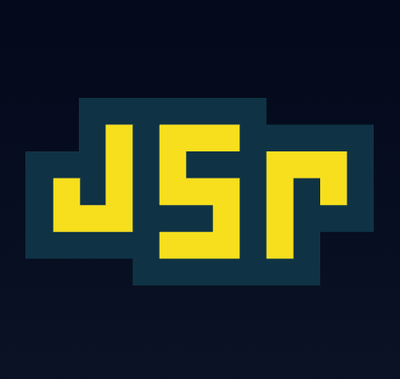
Security News
JSR Working Group Kicks Off with Ambitious Roadmap and Plans for Open Governance
At its inaugural meeting, the JSR Working Group outlined plans for an open governance model and a roadmap to enhance JavaScript package management.
react-use
Advanced tools
The react-use package is a collection of essential React hooks that provide a variety of functionalities to manage state, lifecycle, and side effects in functional components. It simplifies the process of creating complex components by abstracting common behavior into reusable hooks.
State Management
Manage state with local storage persistence using useLocalStorage hook.
const [state, setState] = useLocalStorage('my-key', initialValue);
Lifecycle and Side Effects
Handle component lifecycle events with useEffectOnce hook.
useEffectOnce(() => { console.log('Component mounted'); return () => console.log('Component will unmount'); });
Sensors
Access sensor data like battery status using useBattery hook.
const state = useBattery();
Animations
Create animations with useSpring hook.
const [style, play] = useSpring({ from: { opacity: 0 }, to: { opacity: 1 } });
UI State
Control UI state such as fullscreen mode with useFullscreen hook.
const [isFullscreen, toggleFullscreen] = useFullscreen(ref);
Recompose is a React utility belt for function components and higher-order components. It's similar to react-use in that it provides a set of helpers for creating and manipulating components, but it does not focus exclusively on hooks.
React-powerhooks offers a set of hooks that can be used to manage state and lifecycle in React components, similar to react-use. However, react-use has a broader range of hooks, including those for sensors and UI state management.
libreact
.
npm i react-use
useBattery
— tracks device battery state. useGeolocation
— tracks geo location state of user's device. useHover
and useHoverDirty
— tracks mouse hover state of some element. useHash
— tracks location hash value. useIdle
— tracks whether user is being inactive.useIntersection
— tracks an HTML element's intersection. useKey
, useKeyPress
, useKeyboardJs
, and useKeyPressEvent
— track keys. useLocation
and useSearchParam
— tracks page navigation bar location state.useLongPress
— tracks long press gesture of some element.useMedia
— tracks state of a CSS media query. useMediaDevices
— tracks state of connected hardware devices.useMotion
— tracks state of device's motion sensor.useMouse
and useMouseHovered
— tracks state of mouse position. useMouseWheel
— tracks deltaY of scrolled mouse wheel. useNetworkState
— tracks the state of browser's network connection. useOrientation
— tracks state of device's screen orientation.usePageLeave
— triggers when mouse leaves page boundaries.useScratch
— tracks mouse click-and-scrub state.useScroll
— tracks an HTML element's scroll position. useScrolling
— tracks whether HTML element is scrolling.useStartTyping
— detects when user starts typing.useWindowScroll
— tracks Window
scroll position. useWindowSize
— tracks Window
dimensions. useMeasure
and useSize
— tracks an HTML element's dimensions. createBreakpoint
— tracks innerWidth
useScrollbarWidth
— detects browser's native scrollbars width. usePinchZoom
— tracks pointer events to detect pinch zoom in and out status. useAudio
— plays audio and exposes its controls. useClickAway
— triggers callback when user clicks outside target area.useCss
— dynamically adjusts CSS.useDrop
and useDropArea
— tracks file, link and copy-paste drops.useFullscreen
— display an element or video full-screen. useSlider
— provides slide behavior over any HTML element. useSpeech
— synthesizes speech from a text string. useVibrate
— provide physical feedback using the Vibration API. useVideo
— plays video, tracks its state, and exposes playback controls. useRaf
— re-renders component on each requestAnimationFrame
.useInterval
and useHarmonicIntervalFn
— re-renders component on a set interval using setInterval
.useSpring
— interpolates number over time according to spring dynamics.useTimeout
— re-renders component after a timeout.useTimeoutFn
— calls given function after a timeout. useTween
— re-renders component, while tweening a number from 0 to 1. useUpdate
— returns a callback, which re-renders component when called.
useAsync
, useAsyncFn
, and useAsyncRetry
— resolves an async
function.useBeforeUnload
— shows browser alert when user try to reload or close the page.useCookie
— provides way to read, update and delete a cookie. useCopyToClipboard
— copies text to clipboard.useDebounce
— debounces a function. useError
— error dispatcher. useFavicon
— sets favicon of the page.useLocalStorage
— manages a value in localStorage
.useLockBodyScroll
— lock scrolling of the body element.useRafLoop
— calls given function inside the RAF loop.useSessionStorage
— manages a value in sessionStorage
.useThrottle
and useThrottleFn
— throttles a function. useTitle
— sets title of the page.usePermission
— query permission status for browser APIs.
useEffectOnce
— a modified useEffect
hook that only runs once.useEvent
— subscribe to events.useLifecycles
— calls mount
and unmount
callbacks.useMountedState
and useUnmountPromise
— track if component is mounted.usePromise
— resolves promise only while component is mounted.useLogger
— logs in console as component goes through life-cycles.useMount
— calls mount
callbacks.useUnmount
— calls unmount
callbacks.useUpdateEffect
— run an effect
only on updates.useIsomorphicLayoutEffect
— useLayoutEffect
that that works on server.useDeepCompareEffect
, useShallowCompareEffect
, and useCustomCompareEffect
createMemo
— factory of memoized hooks.createReducer
— factory of reducer hooks with custom middleware.createReducerContext
and createStateContext
— factory of hooks for a sharing state between components.useDefault
— returns the default value when state is null
or undefined
.useGetSet
— returns state getter get()
instead of raw state.useGetSetState
— as if useGetSet
and useSetState
had a baby.useLatest
— returns the latest state or propsusePrevious
— returns the previous state or props. usePreviousDistinct
— like usePrevious
but with a predicate to determine if previous
should update.useObservable
— tracks latest value of an Observable
.useRafState
— creates setState
method which only updates after requestAnimationFrame
. useSetState
— creates setState
method which works like this.setState
. useStateList
— circularly iterates over an array. useToggle
and useBoolean
— tracks state of a boolean. useCounter
and useNumber
— tracks state of a number. useList
useUpsert
useMap
— tracks state of an object. useSet
— tracks state of a Set. useQueue
— implements simple queue.useStateValidator
— tracks state of an object. useStateWithHistory
— stores previous state values and provides handles to travel through them. useMultiStateValidator
— alike the useStateValidator
, but tracks multiple states at a time. useMediatedState
— like the regular useState
but with mediation by custom function. useFirstMountState
— check if current render is first. useRendersCount
— count component renders. createGlobalState
— cross component shared state.useMethods
— neat alternative to useReducer
. useEnsuredForwardedRef
and ensuredForwardRef
— use a React.forwardedRef safely.
Usage — how to import.
Unlicense — public domain.
Support — add yourself to backer list below.
FAQs
Collection of React Hooks
The npm package react-use receives a total of 1,284,958 weekly downloads. As such, react-use popularity was classified as popular.
We found that react-use demonstrated a healthy version release cadence and project activity because the last version was released less than a year ago. It has 0 open source maintainers collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
At its inaugural meeting, the JSR Working Group outlined plans for an open governance model and a roadmap to enhance JavaScript package management.
Security News
Research
An advanced npm supply chain attack is leveraging Ethereum smart contracts for decentralized, persistent malware control, evading traditional defenses.
Security News
Research
Attackers are impersonating Sindre Sorhus on npm with a fake 'chalk-node' package containing a malicious backdoor to compromise developers' projects.