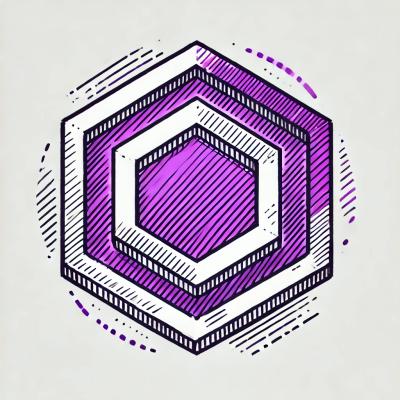
Security News
ESLint is Now Language-Agnostic: Linting JSON, Markdown, and Beyond
ESLint has added JSON and Markdown linting support with new officially-supported plugins, expanding its versatility beyond JavaScript.
reactivity
Advanced tools
##The Native Reactivity Standard for Javascript
Native Reactivity is a very simple "hack" that allows native functions and expressions in Javascript to become Reactive. Which is a fancy way of saying that they can notify consumers when their result changes.
Using Native Reactivity gives you one very important feature for free: Changes are propagated transparently up the call stack. Native Reactivity is automatically transitive - any function that depends on a reactive function is reactive itself.
This means that there is no need to explicitly declare dependencies.
The only "catch" is that everyone has to use the same implementation. This is the reason behind the reactivity.io effort. It defines an API and provides a cannonical implementation.
npm install reactivity
var reactivity = require('reactivity')
Include the following JS file
<script src="https://raw.github.com/aldonline/reactivity.js/master/dist/reactivity.min.js"></script>
In the browser, the global reactivity object is attached to the root scope ( window )
var reactivity = window.reactivity
If the object is already present then the library won't mess things up. It will proxy calls to the pre-existing implementation.
Functions throw an invalidation event up the stack. However, because the stack is transient and won't exist in the future, functions that wish to notify a change need to request a callback so they can throw the event in the future.
Requesting this callback will make sure that any consuming functions get a chance to ask for notifiers on the other side.
function time(){
var notifier = reactivity.notifier() // request a notifier
setTimeout( notifier, 1000 ) // call it in 1000MS
return new Date().getTime()
}
The caller of the function will have an opportunity to register a listener to be notified when any of the functions that participated in the evaluation are invalidated. At this point you can decide to re-evaluate the expression.
// run the function in a reactive context
// instead of returning the result or throwing an error
// it will return an object with three properties: result, error and monitor
var r = reactivity.run( time )
// we are interested in the result
var time = r.result
// and the monitor
var monitor = r.monitor
// the monitor is null unless the function is reactive
// ( or depends on a reactive function - reactivity is transitive )
// in this case we know that time() is reactive since we created it ourselves
// but we still check for illustrative purposes
if ( monitor != null ){
// and we can now wait to be notifier of a change
monitor.onChange( function(){
console.log( "we should reevaluate the expression" )
})
}
There are two different APIs. One for each side of the problem ( consuming VS publishing )
signature | shortcut | description |
---|---|---|
reactivity.run( f:Function ):Result | reactivity(f) | runs code in a reactive context |
Result::result | x | x |
Result::error | x | x |
Runs a reactive function and returns a Result object
Shortcut:
reactivity(func)
Runs a reactive function repeatedly.
Shortcut:
reactivity( reactiveFunction, callback )
// example
reactivity( reactiveFunction, function(error, result, monitor){
// ...
})
Transform any function into a reactive function by repeatedly evaluating it and comparing its result.
Using this method is considered bad practice in general.
var reactiveFunc = reactivity.poll( func, 500 )
Shortcut:
reactivity( func, 500 )
The Result object has three properties:
states: ready, changed, destroyed, cancelled
Registers a callback that will be called whenever the monitored function changes.
Returns a Notifier
Shortcut:
reactivity()
Shortcut
notifier()
States: ready, cancelled, fired, destroyed
In order to be able to combine libraries developed by different people at different times we need to agree on a common way of notifying changes up the stack.
This means ( at the least ) sharing a global object where invalidators and notifiers meet each other. reactivity.js provides a microlibrary and a set of conventions. If we all follow them, Javascript automatically becomes a MUCH more powerful language.
Because an expression may depend on several reactive functions, the Invalidation event you catch at the top of the stack may come from any of them. The value of this specific function is not important. What's important is the result of evaluating the complete expression.
Like all good ideas and patterns in software they have been discovered and rediscovered over and over again. The author of this library can attest that its first encounter with this pattern was as part of a strategy to invalidate caches when calling complex stored procedures probably 15 years ago. The implementation details were a bit different but the principle was the same. Naturally, this idea was ported to Javascript over a decade ago.
Lately it has popped up in several places. The most notable of them all is probably the Meteor.js framework, where it is tightly coupled with the framework.
FAQs
Native Reactivity for Javascript
The npm package reactivity receives a total of 55 weekly downloads. As such, reactivity popularity was classified as not popular.
We found that reactivity demonstrated a not healthy version release cadence and project activity because the last version was released a year ago. It has 1 open source maintainer collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
ESLint has added JSON and Markdown linting support with new officially-supported plugins, expanding its versatility beyond JavaScript.
Security News
Members Hub is conducting large-scale campaigns to artificially boost Discord server metrics, undermining community trust and platform integrity.
Security News
NIST has failed to meet its self-imposed deadline of clearing the NVD's backlog by the end of the fiscal year. Meanwhile, CVE's awaiting analysis have increased by 33% since June.