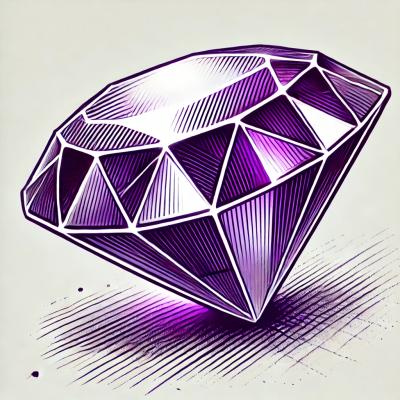
Security News
RubyGems.org Adds New Maintainer Role
RubyGems.org has added a new "maintainer" role that allows for publishing new versions of gems. This new permission type is aimed at improving security for gem owners and the service overall.
read-json-sync
Advanced tools
A Node.js module to read and parse a JSON file synchronously
const readJsonSync = require('read-json-sync');
readJsonSync('package.json'); //=> {name: 'read-json-sync', version: '1.0.0', ...}
Node.js built-in require
and import
can do almost the same thing, but this module doesn't cache results.
npm install read-json-sync
const readJsonSync = require('read-json-sync');
path: string
Buffer
URL
(JSON filename) or integer
(file descriptor)
options: Object
string
(fs.readFile
options or an encoding of the file)
Return: any
(parsed JSON data)
It automatically ignores the leading byte order mark.
// with-bom.json: '\uFEFF{"a": 1}'
JSON.parse('\uFEFF{"a": 1}'); // throws a SyntaxError
readJsonSync('with-bom.json'); //=> {a: 1}
ISC License © 2017 - 2018 Shinnosuke Watanabe
FAQs
Read and parse a JSON file synchronously
The npm package read-json-sync receives a total of 33,191 weekly downloads. As such, read-json-sync popularity was classified as popular.
We found that read-json-sync demonstrated a not healthy version release cadence and project activity because the last version was released a year ago. It has 1 open source maintainer collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
RubyGems.org has added a new "maintainer" role that allows for publishing new versions of gems. This new permission type is aimed at improving security for gem owners and the service overall.
Security News
Node.js will be enforcing stricter semver-major PR policies a month before major releases to enhance stability and ensure reliable release candidates.
Security News
Research
Socket's threat research team has detected five malicious npm packages targeting Roblox developers, deploying malware to steal credentials and personal data.