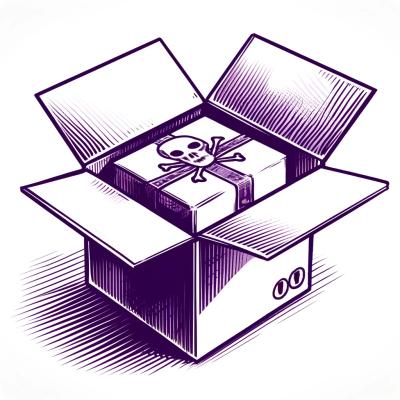
Security News
Research
Data Theft Repackaged: A Case Study in Malicious Wrapper Packages on npm
The Socket Research Team breaks down a malicious wrapper package that uses obfuscation to harvest credentials and exfiltrate sensitive data.
read-package-up
Advanced tools
The 'read-package-up' npm package is a utility that helps you read the closest package.json file, starting from a specified directory and traversing upwards. It is useful for tasks that require information from the package.json file, such as versioning, dependency management, and configuration.
Read the closest package.json
This feature allows you to read the closest package.json file starting from the current directory and moving upwards. The result includes the path to the package.json file and its contents.
const readPackageUp = require('read-package-up');
(async () => {
const result = await readPackageUp();
console.log(result);
})();
Specify a starting directory
This feature allows you to specify a starting directory from which the search for the closest package.json file will begin. This is useful if you want to start the search from a specific location rather than the current working directory.
const readPackageUp = require('read-package-up');
(async () => {
const result = await readPackageUp({ cwd: '/path/to/start/directory' });
console.log(result);
})();
The 'find-up' package is a utility for finding a file or directory by walking up parent directories. It is more general-purpose compared to 'read-package-up' as it can be used to find any file, not just package.json. However, it requires additional steps to read and parse the package.json file.
The 'pkg-up' package is similar to 'read-package-up' in that it finds the closest package.json file by traversing upwards. However, it only returns the path to the package.json file and does not read its contents. You would need to use another package like 'fs' to read the file.
The 'read-pkg' package reads the package.json file from a specified path. Unlike 'read-package-up', it does not traverse upwards to find the closest package.json file. It is useful when you already know the exact path to the package.json file you want to read.
Read the closest package.json file
npm install read-package-up
import {readPackageUp} from 'read-package-up';
console.log(await readPackageUp());
/*
{
packageJson: {
name: 'awesome-package',
version: '1.0.0',
…
},
path: '/Users/sindresorhus/dev/awesome-package/package.json'
}
*/
Returns a Promise<object>
, or Promise<undefined>
if no package.json
was found.
Returns the result object, or undefined
if no package.json
was found.
Type: object
Type: URL | string
Default: process.cwd()
The directory to start looking for a package.json file.
Type: boolean
Default: true
Normalize the package data.
FAQs
Read the closest package.json file
We found that read-package-up demonstrated a not healthy version release cadence and project activity because the last version was released a year ago. It has 1 open source maintainer collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
Research
The Socket Research Team breaks down a malicious wrapper package that uses obfuscation to harvest credentials and exfiltrate sensitive data.
Research
Security News
Attackers used a malicious npm package typosquatting a popular ESLint plugin to steal sensitive data, execute commands, and exploit developer systems.
Security News
The Ultralytics' PyPI Package was compromised four times in one weekend through GitHub Actions cache poisoning and failure to rotate previously compromised API tokens.