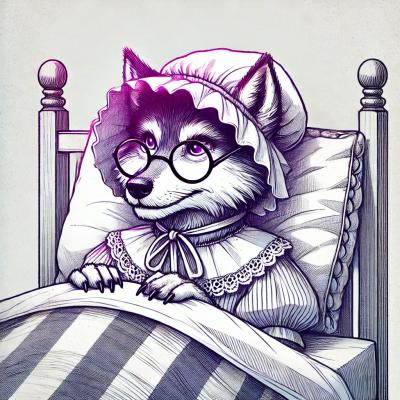
Security News
GitHub Removes Malicious Pull Requests Targeting Open Source Repositories
GitHub removed 27 malicious pull requests attempting to inject harmful code across multiple open source repositories, in another round of low-effort attacks.
The readline npm package is a core Node.js module that provides an interface for reading data from a Readable stream (like process.stdin) one line at a time. It is particularly useful for creating command-line interfaces and reading large files line by line.
Reading Input from stdin
This code sets up a readline interface to read user input from the standard input (stdin). It asks the user 'What is your name?' and prints a greeting with the user's input.
const readline = require('readline');
const rl = readline.createInterface({
input: process.stdin,
output: process.stdout
});
rl.question('What is your name? ', (answer) => {
console.log(`Hello, ${answer}!`);
rl.close();
});
Reading a File Line by Line
This example demonstrates how to use readline to read a file line by line. It creates a readline interface with a file stream as input and logs each line to the console as it is read.
const fs = require('fs');
const readline = require('readline');
const rl = readline.createInterface({
input: fs.createReadStream('file.txt'),
output: process.stdout,
terminal: false
});
rl.on('line', (line) => {
console.log(line);
});
readline-sync is an npm package that provides synchronous readline capabilities. Unlike the asynchronous nature of readline, readline-sync blocks the event loop until input is received. This is useful for CLI applications where synchronous operations are preferred.
Inquirer.js is a powerful library for building interactive command-line interfaces. It offers more features than readline, such as checkboxes, lists, confirmations, and more. It is more suitable for complex CLI applications that require more than just line-by-line input.
Read a file line by line.
npm install linebyline
npm install .
npm test
Simple streaming readline module for NodeJS. Reads a file and buffer new lines emitting a line event for each line.
var readline = require('linebyline'),
rl = readline('./somefile.txt');
rl.on('line', function(line, lineCount, byteCount) {
// do something with the line of text
})
.on('error', function(e) {
// something went wrong
});
##API
BSD © Craig Brookes
FAQs
Simple streaming readline module.
The npm package readline receives a total of 798,468 weekly downloads. As such, readline popularity was classified as popular.
We found that readline demonstrated a not healthy version release cadence and project activity because the last version was released a year ago. It has 1 open source maintainer collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
GitHub removed 27 malicious pull requests attempting to inject harmful code across multiple open source repositories, in another round of low-effort attacks.
Security News
RubyGems.org has added a new "maintainer" role that allows for publishing new versions of gems. This new permission type is aimed at improving security for gem owners and the service overall.
Security News
Node.js will be enforcing stricter semver-major PR policies a month before major releases to enhance stability and ensure reliable release candidates.