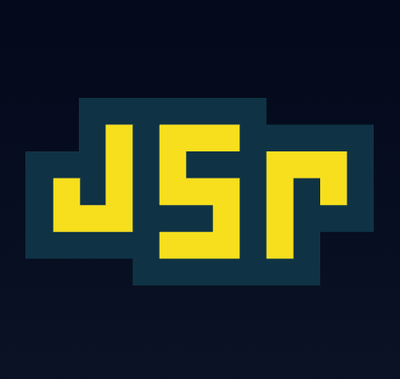
Security News
JSR Working Group Kicks Off with Ambitious Roadmap and Plans for Open Governance
At its inaugural meeting, the JSR Working Group outlined plans for an open governance model and a roadmap to enhance JavaScript package management.
The readline2 npm package is a Node.js module that provides an interface for reading data from a Readable stream (such as process.stdin) one line at a time. It is a more feature-rich and flexible alternative to the built-in readline module in Node.js.
Basic Line Reading
This feature allows you to read a line of input from the user and then process it. The example code demonstrates how to prompt the user for their name and then print a greeting.
const readline = require('readline2');
const rl = readline.createInterface({
input: process.stdin,
output: process.stdout
});
rl.question('What is your name? ', (answer) => {
console.log(`Hello, ${answer}!`);
rl.close();
});
Handling Multiple Lines
This feature allows you to handle multiple lines of input. The example code demonstrates how to listen for 'line' events and process each line of input as it is received.
const readline = require('readline2');
const rl = readline.createInterface({
input: process.stdin,
output: process.stdout
});
rl.on('line', (input) => {
console.log(`Received: ${input}`);
});
rl.on('close', () => {
console.log('Interface closed');
});
Custom Completer
This feature allows you to provide custom tab completion for user input. The example code demonstrates how to set up a completer that suggests commands like 'help', 'error', and 'exit'.
const readline = require('readline2');
const rl = readline.createInterface({
input: process.stdin,
output: process.stdout,
completer: (line) => {
const completions = 'help error exit'.split(' ');
const hits = completions.filter((c) => c.startsWith(line));
return [hits.length ? hits : completions, line];
}
});
rl.question('Type a command: ', (answer) => {
console.log(`You typed: ${answer}`);
rl.close();
});
The built-in readline module in Node.js provides similar functionality for reading lines of input from a Readable stream. However, readline2 offers additional features and flexibility, making it a more powerful alternative.
The prompt package is another alternative for getting user input in Node.js. It provides a more user-friendly interface for prompting users for input, including support for validation and default values. However, it may not offer the same level of control over the input stream as readline2.
Inquirer is a popular package for creating interactive command-line interfaces. It provides a higher-level abstraction for prompting users with various types of questions, such as lists, checkboxes, and password inputs. While it offers more advanced features for user interaction, it may be overkill for simple line reading tasks that readline2 handles well.
Node.js (v0.8 and v0.10) had some bugs and issues with the default Readline module.
This module include fixes seen in later version (0.11) and ease some undesirable behavior one could see using the readline to create interatives prompts. This means readline2
change some behaviors and as so is not meant to be a drop-in replacement.
This project is extracted from the core of Inquirer.js interactive prompt interface to be available as a standalone module.
Installation: npm install --save readline2
Present the same API as Node.js readline.createInterface()
options.input
as process.stdin
options.output
as process.stdout
interface.stdout
is wrapped in a MuteStreamup
and down
keys from moving through history inside the readlineInterface
prompt contains ANSI colorsCopyright (c) 2012 Simon Boudrias (twitter: @vaxilart) Licensed under the MIT license.
FAQs
Readline Façade fixing bugs and issues found in releases 0.8 and 0.10
We found that readline2 demonstrated a not healthy version release cadence and project activity because the last version was released a year ago. It has 1 open source maintainer collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
At its inaugural meeting, the JSR Working Group outlined plans for an open governance model and a roadmap to enhance JavaScript package management.
Security News
Research
An advanced npm supply chain attack is leveraging Ethereum smart contracts for decentralized, persistent malware control, evading traditional defenses.
Security News
Research
Attackers are impersonating Sindre Sorhus on npm with a fake 'chalk-node' package containing a malicious backdoor to compromise developers' projects.