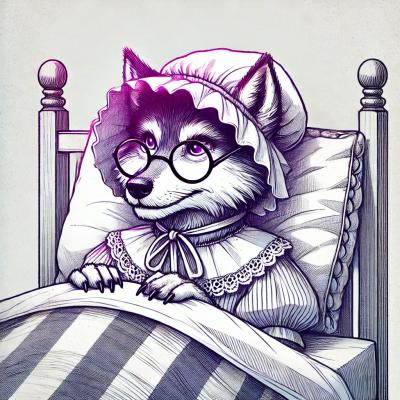
Security News
GitHub Removes Malicious Pull Requests Targeting Open Source Repositories
GitHub removed 27 malicious pull requests attempting to inject harmful code across multiple open source repositories, in another round of low-effort attacks.
reakit-utils
Advanced tools
The reakit-utils package provides a collection of utility functions that are useful for building accessible and reusable React components. These utilities help with DOM manipulation, event handling, and other common tasks in React development.
DOM Utilities
DOM utilities help with common DOM manipulation tasks. For example, `isVisibleInViewport` checks if an element is visible within the viewport.
const element = document.getElementById('myElement');
const isVisible = isVisibleInViewport(element);
Event Utilities
Event utilities simplify event handling. The `addEventListener` function, for instance, adds an event listener to a DOM element.
import { addEventListener } from 'reakit-utils';
const handleClick = () => console.log('Element clicked');
addEventListener(document.getElementById('myElement'), 'click', handleClick);
Array Utilities
Array utilities provide functions for common array operations. `removeItemFromArray` removes a specified item from an array.
import { removeItemFromArray } from 'reakit-utils';
const array = [1, 2, 3, 4];
const newArray = removeItemFromArray(array, 3); // [1, 2, 4]
String Utilities
String utilities offer functions for string manipulation. The `capitalize` function capitalizes the first letter of a string.
import { capitalize } from 'reakit-utils';
const text = 'hello world';
const capitalizedText = capitalize(text); // 'Hello world'
Lodash is a popular utility library that provides a wide range of functions for common programming tasks, including array manipulation, object manipulation, and more. It is more comprehensive and widely used compared to reakit-utils.
Ramda is a functional programming library for JavaScript that provides utility functions for working with arrays, objects, and other data types. It emphasizes immutability and pure functions, making it a good choice for functional programming paradigms.
Date-fns is a utility library for working with dates in JavaScript. It provides a comprehensive set of functions for date manipulation and formatting. While it focuses specifically on dates, it offers more specialized functionality compared to reakit-utils.
This is experimental and may have breaking changes in minor versions.
npm:
npm i reakit-utils
Yarn:
yarn add reakit-utils
It's true
if it is running in a browser environment or false
if it is not (SSR).
import { canUseDOM } from "reakit-utils";
const title = canUseDOM ? document.title : "";
Checks if a given string exists in the user agent string.
string
stringReturns element.ownerDocument || document
.
Returns Document
Returns element.ownerDocument.defaultView || window
.
element
Element?Returns Window
Returns element.ownerDocument.activeElement
.
Similar to Element.prototype.contains
, but a little bit faster when
element
is the same as child
.
import { contains } from "reakit-utils";
contains(document.getElementById("parent"), document.getElementById("child"));
Returns boolean
Checks whether element
is a native HTML button element.
import { isButton } from "reakit-utils";
isButton(document.querySelector("button")); // true
isButton(document.querySelector("input[type='button']")); // true
isButton(document.querySelector("div")); // false
isButton(document.querySelector("input[type='text']")); // false
isButton(document.querySelector("div[role='button']")); // false
Returns boolean
Ponyfill for Element.prototype.matches
Returns boolean
Ponyfill for Element.prototype.closest
element
Elementselectors
Kimport { closest } from "reakit-utils";
closest(document.getElementById("id"), "div");
// same as
document.getElementById("id").closest("div");
Returns any
Check whether the given element is a text field, where text field is defined by the ability to select within the input, or that it is contenteditable.
element
HTMLElementimport { isTextField } from "reakit-utils";
isTextField(document.querySelector("div")); // false
isTextField(document.querySelector("input")); // true
isTextField(document.querySelector("input[type='button']")); // false
isTextField(document.querySelector("textarea")); // true
isTextField(document.querySelector("div[contenteditable='true']")); // true
Returns boolean
Returns the native tag name of the passed element. If the element is not
provided, the second argument defaultType
will be used, but only if it's
a string.
import { getNativeElementType } from "reakit-utils";
getNativeElementType(document.querySelector("div")); // "div"
getNativeElementType(document.querySelector("button")); // "button"
getNativeElementType(null, "button"); // "button"
getNativeElementType(null, SomeComponent); // undefined
Returns true
if event
has been fired within a React Portal element.
event
SyntheticEventReturns boolean
Returns true
if event.target
and event.currentTarget
are the same.
event
SyntheticEventReturns boolean
Creates an event. Supports IE 11.
element
HTMLElementtype
stringeventInit
EventInit?import { createEvent } from "reakit-utils";
const element = document.getElementById("id");
createEvent(element, "blur", { bubbles: false });
Returns Event
Creates and dispatches an event. Supports IE 11.
element
HTMLElementtype
stringeventInit
EventInitimport { fireEvent } from "reakit-utils";
fireEvent(document.getElementById("id"), "blur", {
bubbles: true,
cancelable: true,
});
Creates and dispatches a blur event. Supports IE 11.
element
HTMLElementeventInit
FocusEventInit?import { fireBlurEvent } from "reakit-utils";
fireBlurEvent(document.getElementById("id"));
Creates and dispatches a keyboard event. Supports IE 11.
element
HTMLElementtype
stringeventInit
KeyboardEventInitimport { fireKeyboardEvent } from "reakit-utils";
fireKeyboardEvent(document.getElementById("id"), "keydown", {
key: "ArrowDown",
shiftKey: true,
});
Cross-browser method that returns the next active element (the element that is receiving focus) after a blur event is dispatched. It receives the blur event object as the argument.
event
(ReactFocusEvent | FocusEvent)import { getNextActiveElementOnBlur } from "reakit-utils";
const element = document.getElementById("id");
element.addEventListener("blur", (event) => {
const nextActiveElement = getNextActiveElementOnBlur(event);
});
Transforms an array with multiple levels into a flattened one.
array
Array<T>import { flatten } from "reakit-utils";
flatten([0, 1, [2, [3, 4], 5], 6]);
// => [0, 1, 2, 3, 4, 5, 6]
Checks whether element
is focusable or not.
element
Elementimport { isFocusable } from "reakit-utils";
isFocusable(document.querySelector("input")); // true
isFocusable(document.querySelector("input[tabindex='-1']")); // true
isFocusable(document.querySelector("input[hidden]")); // false
isFocusable(document.querySelector("input:disabled")); // false
Returns boolean
Checks whether element
is tabbable or not.
element
Elementimport { isTabbable } from "reakit-utils";
isTabbable(document.querySelector("input")); // true
isTabbable(document.querySelector("input[tabindex='-1']")); // false
isTabbable(document.querySelector("input[hidden]")); // false
isTabbable(document.querySelector("input:disabled")); // false
Returns boolean
Returns all the focusable elements in container
.
container
ElementReturns the first focusable element in container
.
container
ElementReturns (Element | null)
Returns all the tabbable elements in container
, including the container
itself.
container
ElementfallbackToFocusable
boolean? If true
, it'll return focusable elements if there are no tabbable ones.Returns the first tabbable element in container
, including the container
itself if it's tabbable.
container
ElementfallbackToFocusable
boolean? If true
, it'll return the first focusable element if there are no tabbable ones.Returns (Element | null)
Returns the last tabbable element in container
, including the container
itself if it's tabbable.
container
ElementfallbackToFocusable
boolean? If true
, it'll return the last focusable element if there are no tabbable ones.Returns (Element | null)
Returns the next tabbable element in container
.
container
ElementfallbackToFocusable
boolean? If true
, it'll return the next focusable element if there are no tabbable ones.Returns (Element | null)
Returns the previous tabbable element in container
.
container
ElementfallbackToFocusable
boolean? If true
, it'll return the previous focusable element if there are no tabbable ones.Returns (Element | null)
Returns the closest focusable element.
element
(T | null)?container
ElementReturns (Element | null)
Checks if element
has focus. Elements that are referenced by
aria-activedescendant
are also considered.
element
Elementimport { hasFocus } from "reakit-utils";
hasFocus(document.getElementById("id"));
Returns boolean
Checks if element
has focus within. Elements that are referenced by
aria-activedescendant
are also considered.
element
Elementimport { hasFocusWithin } from "reakit-utils";
hasFocusWithin(document.getElementById("id"));
Returns boolean
Ensures element
will receive focus if it's not already.
element
HTMLElement$1
EnsureFocusOptions (optional, default {}
)
$1.preventScroll
$1.isActive
(optional, default hasFocus
)import { ensureFocus } from "reakit-utils";
ensureFocus(document.activeElement); // does nothing
const element = document.querySelector("input");
ensureFocus(element); // focuses element
ensureFocus(element, { preventScroll: true }); // focuses element preventing scroll jump
function isActive(el) {
return el.dataset.active === "true";
}
ensureFocus(document.querySelector("[data-active='true']"), { isActive }); // does nothing
Returns number requestAnimationFrame
call ID so it can be passed to cancelAnimationFrame
if needed.
React.useLayoutEffect
that fallbacks to React.useEffect
on server side.
Returns a value that never changes even if the argument is updated.
value
InitialState<T>import { useInitialValue } from "reakit-utils";
function Component({ prop }) {
const initialProp = useInitialValue(prop);
}
Returns a value that is lazily initiated and never changes.
init
function (): Timport { useLazyRef } from "reakit-utils";
function Component() {
const set = useLazyRef(() => new Set());
}
Creates a React.RefObject
that is constantly updated with the incoming
value.
value
Timport { useLiveRef } from "reakit-utils";
function Component({ prop }) {
const propRef = useLiveRef(prop);
}
Creates a memoized callback function that is constantly updated with the incoming callback.
callback
T?import { useEffect } from "react";
import { useEventCallback } from "reakit-utils";
function Component(props) {
const onClick = useEventCallback(props.onClick);
useEffect(() => {}, [onClick]);
}
Merges React Refs into a single memoized function ref so you can pass it to an element.
import { forwardRef, useRef } from "react";
import { useForkRef } from "reakit-utils";
const Component = forwardRef((props, ref) => {
const internalRef = useRef();
return <div {...props} ref={useForkRef(internalRef, ref)} />;
});
Returns the native tag name of an element by parsing its ref object. If the
second argument defaultType
is provided, it's going to be used by default
on the first render, before React runs the effects.
import * as React from "react";
import { useNativeElementType } from "reakit-utils";
function Component(props) {
const ref = React.useRef();
// button on the first render, div on the second render
const type = useNativeElementType(ref, "button");
return <div ref={ref} {...props} />;
}
A React.useEffect
that will not run on the first render.
effect
EffectCallbackdeps
(ReadonlyArray<any> | undefined)?Compares two objects.
a
AnyObject?b
AnyObject?import { shallowEqual } from "reakit-utils";
shallowEqual({ a: "a" }, {}); // false
shallowEqual({ a: "a" }, { b: "b" }); // false
shallowEqual({ a: "a" }, { a: "a" }); // true
shallowEqual({ a: "a" }, { a: "a", b: "b" }); // false
Transforms arg
into an array if it's not already.
arg
Timport { toArray } from "reakit-utils";
toArray("a"); // ["a"]
toArray(["a"]); // ["a"]
Receives a setState
argument and calls it with currentValue
if it's a
function. Otherwise return the argument as the new value.
argument
SetStateAction<T>currentValue
Timport { applyState } from "reakit-utils";
applyState((value) => value + 1, 1); // 2
applyState(2, 1); // 2
Checks whether arg
is an object or not.
arg
anyReturns boolean
Checks whether arg
is a plain object or not.
arg
anyReturns boolean
Checks whether arg
is a promise or not.
arg
(T | Promise<T>)Returns boolean
Checks whether arg
is empty or not.
arg
anyimport { isEmpty } from "reakit-utils";
isEmpty([]); // true
isEmpty(["a"]); // false
isEmpty({}); // true
isEmpty({ a: "a" }); // false
isEmpty(); // true
isEmpty(null); // true
isEmpty(undefined); // true
isEmpty(""); // true
Returns boolean
Checks whether arg
is an integer or not.
arg
anyimport { isInteger } from "reakit-utils";
isInteger(1); // true
isInteger(1.5); // false
isInteger("1"); // true
isInteger("1.5"); // false
Returns boolean
Immutably removes an index from an array.
array
Tindex
numberimport { removeIndexFromArray } from "reakit-utils";
removeIndexFromArray(["a", "b", "c"], 1); // ["a", "c"]
Returns Array A new array without the item in the passed index.
Immutably removes an item from an array.
array
Aitem
anyimport { removeItemFromArray } from "reakit-utils";
removeItemFromArray(["a", "b", "c"], "b"); // ["a", "c"]
// This only works by reference
const obj = {};
removeItemFromArray([obj], {}); // [obj]
removeItemFromArray([obj], obj); // []
Returns Array A new array without the passed item.
Omits specific keys from an object.
object
Tpaths
(ReadonlyArray<K> | Array<K>)import { omit } from "reakit-utils";
omit({ a: "a", b: "b" }, ["a"]); // { b: "b" }
Returns Omit<T, K>
Picks specific keys from an object.
object
Tpaths
(ReadonlyArray<K> | Array<K>)import { pick } from "reakit-utils";
pick({ a: "a", b: "b" }, ["a"]); // { a: "a" }
Render prop type
Type: function (props: P): React.ReactElement<any>
"as" prop
Type: React.ElementType<P>
Type: any
Returns only the HTML attributes inside P
type OnlyId = ExtractHTMLAttributes<{ id: string; foo: string }>;
type HTMLAttributes = ExtractHTMLAttributes<any>;
Type: Pick<HTMLAttributesWithRef, Extract<any, any>>
Transforms "a" | "b"
into "a" & "b"
Type: any
Generic component props with "as" prop
Type: any
Returns the type of the items in an array
Type: any
Any function
Type: function (...args: Array<any>): any
Any object
Type: Record<any, any>
State hook setter.
Type: React.Dispatch<React.SetStateAction<T>>
Initial state.
Type: (T | function (): T)
MIT © Diego Haz
FAQs
Reakit utils
The npm package reakit-utils receives a total of 0 weekly downloads. As such, reakit-utils popularity was classified as not popular.
We found that reakit-utils demonstrated a not healthy version release cadence and project activity because the last version was released a year ago. It has 1 open source maintainer collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
GitHub removed 27 malicious pull requests attempting to inject harmful code across multiple open source repositories, in another round of low-effort attacks.
Security News
RubyGems.org has added a new "maintainer" role that allows for publishing new versions of gems. This new permission type is aimed at improving security for gem owners and the service overall.
Security News
Node.js will be enforcing stricter semver-major PR policies a month before major releases to enhance stability and ensure reliable release candidates.