Comparing version 2.0.1 to 2.1.0
@@ -1,2 +0,2 @@ | ||
'use strict'; | ||
"use strict"; | ||
@@ -6,36 +6,40 @@ Object.defineProperty(exports, "__esModule", { | ||
}); | ||
exports.Notification = undefined; | ||
exports["default"] = exports.Notification = void 0; | ||
var _createClass = function () { function defineProperties(target, props) { for (var i = 0; i < props.length; i++) { var descriptor = props[i]; descriptor.enumerable = descriptor.enumerable || false; descriptor.configurable = true; if ("value" in descriptor) descriptor.writable = true; Object.defineProperty(target, descriptor.key, descriptor); } } return function (Constructor, protoProps, staticProps) { if (protoProps) defineProperties(Constructor.prototype, protoProps); if (staticProps) defineProperties(Constructor, staticProps); return Constructor; }; }(); | ||
var _react = _interopRequireWildcard(require("react")); | ||
var _react = require('react'); | ||
var _propTypes = _interopRequireDefault(require("prop-types")); | ||
var _react2 = _interopRequireDefault(_react); | ||
var _reactRedux = require("react-redux"); | ||
var _propTypes = require('prop-types'); | ||
var _helpers = require("../helpers"); | ||
var _propTypes2 = _interopRequireDefault(_propTypes); | ||
var _notifications = require("../store/notifications"); | ||
var _reactRedux = require('react-redux'); | ||
var _constants = require("../constants"); | ||
var _helpers = require('../helpers'); | ||
function _interopRequireDefault(obj) { return obj && obj.__esModule ? obj : { "default": obj }; } | ||
var _notifications = require('../store/notifications'); | ||
function _getRequireWildcardCache() { if (typeof WeakMap !== "function") return null; var cache = new WeakMap(); _getRequireWildcardCache = function _getRequireWildcardCache() { return cache; }; return cache; } | ||
var _constants = require('../constants'); | ||
function _interopRequireWildcard(obj) { if (obj && obj.__esModule) { return obj; } var cache = _getRequireWildcardCache(); if (cache && cache.has(obj)) { return cache.get(obj); } var newObj = {}; if (obj != null) { var hasPropertyDescriptor = Object.defineProperty && Object.getOwnPropertyDescriptor; for (var key in obj) { if (Object.prototype.hasOwnProperty.call(obj, key)) { var desc = hasPropertyDescriptor ? Object.getOwnPropertyDescriptor(obj, key) : null; if (desc && (desc.get || desc.set)) { Object.defineProperty(newObj, key, desc); } else { newObj[key] = obj[key]; } } } } newObj["default"] = obj; if (cache) { cache.set(obj, newObj); } return newObj; } | ||
function _interopRequireDefault(obj) { return obj && obj.__esModule ? obj : { default: obj }; } | ||
function _typeof(obj) { if (typeof Symbol === "function" && typeof Symbol.iterator === "symbol") { _typeof = function _typeof(obj) { return typeof obj; }; } else { _typeof = function _typeof(obj) { return obj && typeof Symbol === "function" && obj.constructor === Symbol && obj !== Symbol.prototype ? "symbol" : typeof obj; }; } return _typeof(obj); } | ||
function _classCallCheck(instance, Constructor) { if (!(instance instanceof Constructor)) { throw new TypeError("Cannot call a class as a function"); } } | ||
function _possibleConstructorReturn(self, call) { if (!self) { throw new ReferenceError("this hasn't been initialised - super() hasn't been called"); } return call && (typeof call === "object" || typeof call === "function") ? call : self; } | ||
function _defineProperties(target, props) { for (var i = 0; i < props.length; i++) { var descriptor = props[i]; descriptor.enumerable = descriptor.enumerable || false; descriptor.configurable = true; if ("value" in descriptor) descriptor.writable = true; Object.defineProperty(target, descriptor.key, descriptor); } } | ||
function _inherits(subClass, superClass) { if (typeof superClass !== "function" && superClass !== null) { throw new TypeError("Super expression must either be null or a function, not " + typeof superClass); } subClass.prototype = Object.create(superClass && superClass.prototype, { constructor: { value: subClass, enumerable: false, writable: true, configurable: true } }); if (superClass) Object.setPrototypeOf ? Object.setPrototypeOf(subClass, superClass) : subClass.__proto__ = superClass; } | ||
function _createClass(Constructor, protoProps, staticProps) { if (protoProps) _defineProperties(Constructor.prototype, protoProps); if (staticProps) _defineProperties(Constructor, staticProps); return Constructor; } | ||
/** | ||
* Create a timer | ||
* @param {Number} dismissAfter | ||
* @param {Function} callback | ||
* @returns {Function|null} a Timer | ||
*/ | ||
function _possibleConstructorReturn(self, call) { if (call && (_typeof(call) === "object" || typeof call === "function")) { return call; } return _assertThisInitialized(self); } | ||
function _assertThisInitialized(self) { if (self === void 0) { throw new ReferenceError("this hasn't been initialised - super() hasn't been called"); } return self; } | ||
function _getPrototypeOf(o) { _getPrototypeOf = Object.setPrototypeOf ? Object.getPrototypeOf : function _getPrototypeOf(o) { return o.__proto__ || Object.getPrototypeOf(o); }; return _getPrototypeOf(o); } | ||
function _inherits(subClass, superClass) { if (typeof superClass !== "function" && superClass !== null) { throw new TypeError("Super expression must either be null or a function"); } subClass.prototype = Object.create(superClass && superClass.prototype, { constructor: { value: subClass, writable: true, configurable: true } }); if (superClass) _setPrototypeOf(subClass, superClass); } | ||
function _setPrototypeOf(o, p) { _setPrototypeOf = Object.setPrototypeOf || function _setPrototypeOf(o, p) { o.__proto__ = p; return o; }; return _setPrototypeOf(o, p); } | ||
function createTimer(dismissAfter, callback) { | ||
@@ -45,6 +49,9 @@ if (dismissAfter > 0) { | ||
} | ||
return null; | ||
} | ||
var Notification = exports.Notification = function (_Component) { | ||
var Notification = | ||
/*#__PURE__*/ | ||
function (_Component) { | ||
_inherits(Notification, _Component); | ||
@@ -58,8 +65,9 @@ | ||
function Notification(props) { | ||
var _this; | ||
_classCallCheck(this, Notification); | ||
var dismissAfter = props.notification.dismissAfter; | ||
_this = _possibleConstructorReturn(this, _getPrototypeOf(Notification).call(this, props)); | ||
var _this = _possibleConstructorReturn(this, (Notification.__proto__ || Object.getPrototypeOf(Notification)).call(this, props)); | ||
_this._remove = function () { | ||
@@ -69,3 +77,2 @@ var _this$props = _this.props, | ||
id = _this$props.notification.id; | ||
removeNotification(id); | ||
@@ -76,3 +83,2 @@ }; | ||
var timer = _this.state.timer; | ||
timer.pause(); | ||
@@ -83,3 +89,2 @@ }; | ||
var timer = _this.state.timer; | ||
timer.resume(); | ||
@@ -98,4 +103,2 @@ }; | ||
buttons = _this$props2.notification.buttons; | ||
return buttons.map(function (_ref) { | ||
@@ -105,15 +108,9 @@ var name = _ref.name, | ||
primary = _ref.primary; | ||
return _react2.default.createElement( | ||
'button', | ||
{ key: name, className: className.button, onClick: onClick }, | ||
_react2.default.createElement( | ||
'span', | ||
{ className: className.buttonText }, | ||
primary ? _react2.default.createElement( | ||
'b', | ||
null, | ||
name | ||
) : name | ||
) | ||
); | ||
return _react["default"].createElement("button", { | ||
key: name, | ||
className: className.button, | ||
onClick: onClick | ||
}, _react["default"].createElement("span", { | ||
className: className.buttonText | ||
}, primary ? _react["default"].createElement("b", null, name) : name)); | ||
}); | ||
@@ -127,3 +124,2 @@ }; | ||
} | ||
/** | ||
@@ -136,3 +132,3 @@ * Run `onAdd` callback function when component is mounted | ||
_createClass(Notification, [{ | ||
key: 'componentDidMount', | ||
key: "componentDidMount", | ||
value: function componentDidMount() { | ||
@@ -145,3 +141,2 @@ var onAdd = this.props.notification.onAdd; | ||
} | ||
/** | ||
@@ -153,3 +148,3 @@ * Run `onRemove` callback function when component will unmount | ||
}, { | ||
key: 'componentWillUnmount', | ||
key: "componentWillUnmount", | ||
value: function componentWillUnmount() { | ||
@@ -162,3 +157,2 @@ var onRemove = this.props.notification.onRemove; | ||
} | ||
/** | ||
@@ -171,6 +165,5 @@ * Update timer | ||
}, { | ||
key: 'componentWillReceiveProps', | ||
key: "componentWillReceiveProps", | ||
value: function componentWillReceiveProps(nextProps) { | ||
var dismissAfter = nextProps.notification.dismissAfter; | ||
this.setState({ | ||
@@ -180,3 +173,2 @@ timer: createTimer(dismissAfter, this._remove) | ||
} | ||
/** | ||
@@ -188,34 +180,5 @@ * Remove the notification | ||
/** | ||
* Pauses the timer | ||
* @returns {void} | ||
* @private | ||
*/ | ||
/** | ||
* Resumes the timer | ||
* @returns {void} | ||
* @private | ||
*/ | ||
/** | ||
* Wrap content in an object ready for HTML | ||
* @param {String} content a text | ||
* @returns {Object} | ||
* @private | ||
*/ | ||
/** | ||
* Render button(s) | ||
* @returns {*} | ||
*/ | ||
}, { | ||
key: 'render', | ||
key: "render", | ||
/** | ||
@@ -226,15 +189,14 @@ * Render | ||
value: function render() { | ||
var _props = this.props, | ||
className = _props.className, | ||
_props$notification = _props.notification, | ||
title = _props$notification.title, | ||
message = _props$notification.message, | ||
status = _props$notification.status, | ||
dismissible = _props$notification.dismissible, | ||
closeButton = _props$notification.closeButton, | ||
buttons = _props$notification.buttons, | ||
image = _props$notification.image, | ||
allowHTML = _props$notification.allowHTML; | ||
var _this$props3 = this.props, | ||
className = _this$props3.className, | ||
_this$props3$notifica = _this$props3.notification, | ||
title = _this$props3$notifica.title, | ||
message = _this$props3$notifica.message, | ||
status = _this$props3$notifica.status, | ||
dismissible = _this$props3$notifica.dismissible, | ||
closeButton = _this$props3$notifica.closeButton, | ||
buttons = _this$props3$notifica.buttons, | ||
image = _this$props3$notifica.image, | ||
allowHTML = _this$props3$notifica.allowHTML; | ||
var timer = this.state.timer; | ||
var notificationClass = [className.main, className.status(status), className.buttons(buttons.length), dismissible && !closeButton ? className.dismissible : null].join(' '); | ||
@@ -246,44 +208,39 @@ | ||
return _react2.default.createElement( | ||
'div', | ||
{ | ||
className: className.wrapper, | ||
onClick: dismissible && !closeButton ? this._remove : null, | ||
onMouseEnter: timer ? this._pauseTimer : null, | ||
onMouseLeave: timer ? this._resumeTimer : null | ||
}, | ||
_react2.default.createElement( | ||
'div', | ||
{ className: notificationClass }, | ||
image ? _react2.default.createElement( | ||
'div', | ||
{ className: className.imageContainer }, | ||
_react2.default.createElement('span', { className: className.image, style: { backgroundImage: 'url(' + image + ')' } }) | ||
) : _react2.default.createElement('span', { className: className.icon }), | ||
_react2.default.createElement( | ||
'div', | ||
{ className: className.meta }, | ||
title ? allowHTML ? _react2.default.createElement('h4', { className: className.title, dangerouslySetInnerHTML: this._setHTML(title) }) : _react2.default.createElement( | ||
'h4', | ||
{ className: className.title }, | ||
title | ||
) : null, | ||
message ? allowHTML ? _react2.default.createElement('p', { className: className.message, dangerouslySetInnerHTML: this._setHTML(message) }) : _react2.default.createElement( | ||
'p', | ||
{ className: className.message }, | ||
message | ||
) : null | ||
), | ||
dismissible && closeButton ? _react2.default.createElement( | ||
'div', | ||
{ className: className.closeButtonContainer }, | ||
_react2.default.createElement('span', { className: className.closeButton, onClick: this._remove }) | ||
) : null, | ||
buttons.length ? _react2.default.createElement( | ||
'div', | ||
{ className: className.buttons(), onClick: this._remove }, | ||
this._renderButtons() | ||
) : null | ||
) | ||
); | ||
return _react["default"].createElement("div", { | ||
className: className.wrapper, | ||
onClick: dismissible && !closeButton ? this._remove : null, | ||
onMouseEnter: timer ? this._pauseTimer : null, | ||
onMouseLeave: timer ? this._resumeTimer : null | ||
}, _react["default"].createElement("div", { | ||
className: notificationClass | ||
}, image ? _react["default"].createElement("div", { | ||
className: className.imageContainer | ||
}, _react["default"].createElement("span", { | ||
className: className.image, | ||
style: { | ||
backgroundImage: "url(".concat(image, ")") | ||
} | ||
})) : _react["default"].createElement("span", { | ||
className: className.icon | ||
}), _react["default"].createElement("div", { | ||
className: className.meta | ||
}, title ? allowHTML ? _react["default"].createElement("h4", { | ||
className: className.title, | ||
dangerouslySetInnerHTML: this._setHTML(title) | ||
}) : _react["default"].createElement("h4", { | ||
className: className.title | ||
}, title) : null, message ? allowHTML ? _react["default"].createElement("p", { | ||
className: className.message, | ||
dangerouslySetInnerHTML: this._setHTML(message) | ||
}) : _react["default"].createElement("p", { | ||
className: className.message | ||
}, message) : null), dismissible && closeButton ? _react["default"].createElement("div", { | ||
className: className.closeButtonContainer | ||
}, _react["default"].createElement("span", { | ||
className: className.closeButton, | ||
onClick: this._remove | ||
})) : null, buttons.length ? _react["default"].createElement("div", { | ||
className: className.buttons(), | ||
onClick: this._remove | ||
}, this._renderButtons()) : null)); | ||
} | ||
@@ -295,40 +252,46 @@ }]); | ||
exports.Notification = Notification; | ||
Notification.propTypes = { | ||
className: _propTypes2.default.shape({ | ||
main: _propTypes2.default.string.isRequired, | ||
wrapper: _propTypes2.default.string.isRequired, | ||
meta: _propTypes2.default.string.isRequired, | ||
title: _propTypes2.default.string.isRequired, | ||
message: _propTypes2.default.string.isRequired, | ||
imageContainer: _propTypes2.default.string.isRequired, | ||
image: _propTypes2.default.string.isRequired, | ||
icon: _propTypes2.default.string.isRequired, | ||
status: _propTypes2.default.func.isRequired, | ||
dismissible: _propTypes2.default.string.isRequired, | ||
closeButtonContainer: _propTypes2.default.string.isRequired, | ||
closeButton: _propTypes2.default.string.isRequired, | ||
buttons: _propTypes2.default.func.isRequired, | ||
button: _propTypes2.default.string.isRequired, | ||
buttonText: _propTypes2.default.string.isRequired | ||
className: _propTypes["default"].shape({ | ||
main: _propTypes["default"].string.isRequired, | ||
wrapper: _propTypes["default"].string.isRequired, | ||
meta: _propTypes["default"].string.isRequired, | ||
title: _propTypes["default"].string.isRequired, | ||
message: _propTypes["default"].string.isRequired, | ||
imageContainer: _propTypes["default"].string.isRequired, | ||
image: _propTypes["default"].string.isRequired, | ||
icon: _propTypes["default"].string.isRequired, | ||
status: _propTypes["default"].func.isRequired, | ||
dismissible: _propTypes["default"].string.isRequired, | ||
closeButtonContainer: _propTypes["default"].string.isRequired, | ||
closeButton: _propTypes["default"].string.isRequired, | ||
buttons: _propTypes["default"].func.isRequired, | ||
button: _propTypes["default"].string.isRequired, | ||
buttonText: _propTypes["default"].string.isRequired | ||
}).isRequired, | ||
notification: _propTypes2.default.shape({ | ||
id: _propTypes2.default.oneOfType([_propTypes2.default.number, _propTypes2.default.string]).isRequired, | ||
title: _propTypes2.default.string, | ||
message: _propTypes2.default.string, | ||
image: _propTypes2.default.string, | ||
status: _propTypes2.default.string.isRequired, | ||
position: _propTypes2.default.oneOf((0, _helpers.mapObjectValues)(_constants.POSITIONS)), | ||
dismissAfter: _propTypes2.default.number.isRequired, | ||
dismissible: _propTypes2.default.bool.isRequired, | ||
onAdd: _propTypes2.default.func, | ||
onRemove: _propTypes2.default.func, | ||
closeButton: _propTypes2.default.bool.isRequired, | ||
buttons: _propTypes2.default.arrayOf(_propTypes2.default.shape({ | ||
name: _propTypes2.default.string.isRequired, | ||
onClick: _propTypes2.default.func | ||
notification: _propTypes["default"].shape({ | ||
id: _propTypes["default"].oneOfType([_propTypes["default"].number, _propTypes["default"].string]).isRequired, | ||
title: _propTypes["default"].string, | ||
message: _propTypes["default"].string, | ||
image: _propTypes["default"].string, | ||
status: _propTypes["default"].string.isRequired, | ||
position: _propTypes["default"].oneOf((0, _helpers.mapObjectValues)(_constants.POSITIONS)), | ||
dismissAfter: _propTypes["default"].number.isRequired, | ||
dismissible: _propTypes["default"].bool.isRequired, | ||
onAdd: _propTypes["default"].func, | ||
onRemove: _propTypes["default"].func, | ||
closeButton: _propTypes["default"].bool.isRequired, | ||
buttons: _propTypes["default"].arrayOf(_propTypes["default"].shape({ | ||
name: _propTypes["default"].string.isRequired, | ||
onClick: _propTypes["default"].func | ||
})).isRequired, | ||
allowHTML: _propTypes2.default.bool.isRequired | ||
allowHTML: _propTypes["default"].bool.isRequired | ||
}).isRequired, | ||
removeNotification: _propTypes2.default.func.isRequired | ||
removeNotification: _propTypes["default"].func.isRequired | ||
}; | ||
exports.default = (0, _reactRedux.connect)(null, { removeNotification: _notifications.removeNotification })(Notification); | ||
var _default = (0, _reactRedux.connect)(null, { | ||
removeNotification: _notifications.removeNotification | ||
})(Notification); | ||
exports["default"] = _default; |
@@ -1,2 +0,2 @@ | ||
'use strict'; | ||
"use strict"; | ||
@@ -6,49 +6,57 @@ Object.defineProperty(exports, "__esModule", { | ||
}); | ||
exports.NotificationsContainer = undefined; | ||
exports["default"] = exports.NotificationsContainer = void 0; | ||
var _createClass = function () { function defineProperties(target, props) { for (var i = 0; i < props.length; i++) { var descriptor = props[i]; descriptor.enumerable = descriptor.enumerable || false; descriptor.configurable = true; if ("value" in descriptor) descriptor.writable = true; Object.defineProperty(target, descriptor.key, descriptor); } } return function (Constructor, protoProps, staticProps) { if (protoProps) defineProperties(Constructor.prototype, protoProps); if (staticProps) defineProperties(Constructor, staticProps); return Constructor; }; }(); | ||
var _react = _interopRequireWildcard(require("react")); | ||
var _react = require('react'); | ||
var _propTypes = _interopRequireDefault(require("prop-types")); | ||
var _react2 = _interopRequireDefault(_react); | ||
var _TransitionGroup = _interopRequireDefault(require("react-transition-group/TransitionGroup")); | ||
var _propTypes = require('prop-types'); | ||
var _CSSTransition = _interopRequireDefault(require("react-transition-group/CSSTransition")); | ||
var _propTypes2 = _interopRequireDefault(_propTypes); | ||
var _Notification = _interopRequireDefault(require("./Notification")); | ||
var _TransitionGroup = require('react-transition-group/TransitionGroup'); | ||
function _interopRequireDefault(obj) { return obj && obj.__esModule ? obj : { "default": obj }; } | ||
var _TransitionGroup2 = _interopRequireDefault(_TransitionGroup); | ||
function _getRequireWildcardCache() { if (typeof WeakMap !== "function") return null; var cache = new WeakMap(); _getRequireWildcardCache = function _getRequireWildcardCache() { return cache; }; return cache; } | ||
var _CSSTransition = require('react-transition-group/CSSTransition'); | ||
function _interopRequireWildcard(obj) { if (obj && obj.__esModule) { return obj; } var cache = _getRequireWildcardCache(); if (cache && cache.has(obj)) { return cache.get(obj); } var newObj = {}; if (obj != null) { var hasPropertyDescriptor = Object.defineProperty && Object.getOwnPropertyDescriptor; for (var key in obj) { if (Object.prototype.hasOwnProperty.call(obj, key)) { var desc = hasPropertyDescriptor ? Object.getOwnPropertyDescriptor(obj, key) : null; if (desc && (desc.get || desc.set)) { Object.defineProperty(newObj, key, desc); } else { newObj[key] = obj[key]; } } } } newObj["default"] = obj; if (cache) { cache.set(obj, newObj); } return newObj; } | ||
var _CSSTransition2 = _interopRequireDefault(_CSSTransition); | ||
function _typeof(obj) { if (typeof Symbol === "function" && typeof Symbol.iterator === "symbol") { _typeof = function _typeof(obj) { return typeof obj; }; } else { _typeof = function _typeof(obj) { return obj && typeof Symbol === "function" && obj.constructor === Symbol && obj !== Symbol.prototype ? "symbol" : typeof obj; }; } return _typeof(obj); } | ||
var _Notification = require('./Notification'); | ||
function _classCallCheck(instance, Constructor) { if (!(instance instanceof Constructor)) { throw new TypeError("Cannot call a class as a function"); } } | ||
var _Notification2 = _interopRequireDefault(_Notification); | ||
function _defineProperties(target, props) { for (var i = 0; i < props.length; i++) { var descriptor = props[i]; descriptor.enumerable = descriptor.enumerable || false; descriptor.configurable = true; if ("value" in descriptor) descriptor.writable = true; Object.defineProperty(target, descriptor.key, descriptor); } } | ||
function _interopRequireDefault(obj) { return obj && obj.__esModule ? obj : { default: obj }; } | ||
function _createClass(Constructor, protoProps, staticProps) { if (protoProps) _defineProperties(Constructor.prototype, protoProps); if (staticProps) _defineProperties(Constructor, staticProps); return Constructor; } | ||
function _classCallCheck(instance, Constructor) { if (!(instance instanceof Constructor)) { throw new TypeError("Cannot call a class as a function"); } } | ||
function _possibleConstructorReturn(self, call) { if (call && (_typeof(call) === "object" || typeof call === "function")) { return call; } return _assertThisInitialized(self); } | ||
function _possibleConstructorReturn(self, call) { if (!self) { throw new ReferenceError("this hasn't been initialised - super() hasn't been called"); } return call && (typeof call === "object" || typeof call === "function") ? call : self; } | ||
function _assertThisInitialized(self) { if (self === void 0) { throw new ReferenceError("this hasn't been initialised - super() hasn't been called"); } return self; } | ||
function _inherits(subClass, superClass) { if (typeof superClass !== "function" && superClass !== null) { throw new TypeError("Super expression must either be null or a function, not " + typeof superClass); } subClass.prototype = Object.create(superClass && superClass.prototype, { constructor: { value: subClass, enumerable: false, writable: true, configurable: true } }); if (superClass) Object.setPrototypeOf ? Object.setPrototypeOf(subClass, superClass) : subClass.__proto__ = superClass; } | ||
function _getPrototypeOf(o) { _getPrototypeOf = Object.setPrototypeOf ? Object.getPrototypeOf : function _getPrototypeOf(o) { return o.__proto__ || Object.getPrototypeOf(o); }; return _getPrototypeOf(o); } | ||
var NotificationsContainer = exports.NotificationsContainer = function (_Component) { | ||
function _inherits(subClass, superClass) { if (typeof superClass !== "function" && superClass !== null) { throw new TypeError("Super expression must either be null or a function"); } subClass.prototype = Object.create(superClass && superClass.prototype, { constructor: { value: subClass, writable: true, configurable: true } }); if (superClass) _setPrototypeOf(subClass, superClass); } | ||
function _setPrototypeOf(o, p) { _setPrototypeOf = Object.setPrototypeOf || function _setPrototypeOf(o, p) { o.__proto__ = p; return o; }; return _setPrototypeOf(o, p); } | ||
var NotificationsContainer = | ||
/*#__PURE__*/ | ||
function (_Component) { | ||
_inherits(NotificationsContainer, _Component); | ||
function NotificationsContainer() { | ||
var _ref; | ||
var _getPrototypeOf2; | ||
var _temp, _this, _ret; | ||
var _this; | ||
_classCallCheck(this, NotificationsContainer); | ||
for (var _len = arguments.length, args = Array(_len), _key = 0; _key < _len; _key++) { | ||
for (var _len = arguments.length, args = new Array(_len), _key = 0; _key < _len; _key++) { | ||
args[_key] = arguments[_key]; | ||
} | ||
return _ret = (_temp = (_this = _possibleConstructorReturn(this, (_ref = NotificationsContainer.__proto__ || Object.getPrototypeOf(NotificationsContainer)).call.apply(_ref, [this].concat(args))), _this), _this._renderNotifications = function () { | ||
_this = _possibleConstructorReturn(this, (_getPrototypeOf2 = _getPrototypeOf(NotificationsContainer)).call.apply(_getPrototypeOf2, [this].concat(args))); | ||
_this._renderNotifications = function () { | ||
var position = _this.props.position; | ||
@@ -60,5 +68,3 @@ var className = _this.props.theme.notification.className; | ||
leaveTimeout = _this$props$theme$not.leaveTimeout; | ||
var notifications = _this.props.notifications; | ||
// when notifications are displayed at the bottom, | ||
var notifications = _this.props.notifications; // when notifications are displayed at the bottom, | ||
// we display notifications from bottom to top | ||
@@ -71,36 +77,26 @@ | ||
return notifications.map(function (notification) { | ||
return _react2.default.createElement( | ||
_CSSTransition2.default, | ||
{ | ||
key: notification.id, | ||
classNames: { | ||
enter: name.enter, | ||
exit: name.leave | ||
}, | ||
timeout: { | ||
enter: enterTimeout, | ||
exit: leaveTimeout | ||
} | ||
return _react["default"].createElement(_CSSTransition["default"], { | ||
key: notification.id, | ||
classNames: { | ||
enter: name.enter, | ||
exit: name.leave | ||
}, | ||
_react2.default.createElement(_Notification2.default, { | ||
key: notification.id, | ||
notification: notification, | ||
className: className | ||
}) | ||
); | ||
timeout: { | ||
enter: enterTimeout, | ||
exit: leaveTimeout | ||
} | ||
}, _react["default"].createElement(_Notification["default"], { | ||
key: notification.id, | ||
notification: notification, | ||
className: className | ||
})); | ||
}); | ||
}, _temp), _possibleConstructorReturn(_this, _ret); | ||
}; | ||
return _this; | ||
} | ||
/** | ||
* Render notifications | ||
* @private | ||
* @returns {XML} | ||
*/ | ||
_createClass(NotificationsContainer, [{ | ||
key: 'render', | ||
key: "render", | ||
/** | ||
@@ -113,13 +109,5 @@ * Render | ||
var position = this.props.position; | ||
return _react2.default.createElement( | ||
'div', | ||
{ className: className.main + ' ' + className.position(position) }, | ||
_react2.default.createElement( | ||
_TransitionGroup2.default, | ||
null, | ||
this._renderNotifications() | ||
) | ||
); | ||
return _react["default"].createElement("div", { | ||
className: "".concat(className.main, " ").concat(className.position(position)) | ||
}, _react["default"].createElement(_TransitionGroup["default"], null, this._renderNotifications())); | ||
} | ||
@@ -131,19 +119,20 @@ }]); | ||
exports.NotificationsContainer = NotificationsContainer; | ||
NotificationsContainer.propTypes = { | ||
notifications: _propTypes2.default.array.isRequired, | ||
position: _propTypes2.default.string.isRequired, | ||
theme: _propTypes2.default.shape({ | ||
notificationsContainer: _propTypes2.default.shape({ | ||
className: _propTypes2.default.shape({ | ||
main: _propTypes2.default.string.isRequired, | ||
position: _propTypes2.default.func.isRequired | ||
notifications: _propTypes["default"].array.isRequired, | ||
position: _propTypes["default"].string.isRequired, | ||
theme: _propTypes["default"].shape({ | ||
notificationsContainer: _propTypes["default"].shape({ | ||
className: _propTypes["default"].shape({ | ||
main: _propTypes["default"].string.isRequired, | ||
position: _propTypes["default"].func.isRequired | ||
}).isRequired, | ||
transition: _propTypes2.default.shape({ | ||
name: _propTypes2.default.object.isRequired, | ||
enterTimeout: _propTypes2.default.number.isRequired, | ||
leaveTimeout: _propTypes2.default.number.isRequired | ||
transition: _propTypes["default"].shape({ | ||
name: _propTypes["default"].object.isRequired, | ||
enterTimeout: _propTypes["default"].number.isRequired, | ||
leaveTimeout: _propTypes["default"].number.isRequired | ||
}).isRequired | ||
}).isRequired, | ||
notification: _propTypes2.default.shape({ | ||
className: _propTypes2.default.object.isRequired | ||
notification: _propTypes["default"].shape({ | ||
className: _propTypes["default"].object.isRequired | ||
}).isRequired | ||
@@ -155,2 +144,3 @@ }).isRequired | ||
}; | ||
exports.default = NotificationsContainer; | ||
var _default = NotificationsContainer; | ||
exports["default"] = _default; |
@@ -1,2 +0,2 @@ | ||
'use strict'; | ||
"use strict"; | ||
@@ -6,51 +6,68 @@ Object.defineProperty(exports, "__esModule", { | ||
}); | ||
exports.NotificationsSystem = undefined; | ||
exports["default"] = exports.NotificationsSystem = void 0; | ||
var _createClass = function () { function defineProperties(target, props) { for (var i = 0; i < props.length; i++) { var descriptor = props[i]; descriptor.enumerable = descriptor.enumerable || false; descriptor.configurable = true; if ("value" in descriptor) descriptor.writable = true; Object.defineProperty(target, descriptor.key, descriptor); } } return function (Constructor, protoProps, staticProps) { if (protoProps) defineProperties(Constructor.prototype, protoProps); if (staticProps) defineProperties(Constructor, staticProps); return Constructor; }; }(); | ||
var _react = _interopRequireWildcard(require("react")); | ||
var _react = require('react'); | ||
var _propTypes = _interopRequireDefault(require("prop-types")); | ||
var _react2 = _interopRequireDefault(_react); | ||
var _reactRedux = require("react-redux"); | ||
var _propTypes = require('prop-types'); | ||
var _helpers = require("../helpers"); | ||
var _propTypes2 = _interopRequireDefault(_propTypes); | ||
var _NotificationsContainer = _interopRequireDefault(require("./NotificationsContainer")); | ||
var _reactRedux = require('react-redux'); | ||
var _constants = require("../constants"); | ||
var _helpers = require('../helpers'); | ||
function _interopRequireDefault(obj) { return obj && obj.__esModule ? obj : { "default": obj }; } | ||
var _NotificationsContainer = require('./NotificationsContainer'); | ||
function _getRequireWildcardCache() { if (typeof WeakMap !== "function") return null; var cache = new WeakMap(); _getRequireWildcardCache = function _getRequireWildcardCache() { return cache; }; return cache; } | ||
var _NotificationsContainer2 = _interopRequireDefault(_NotificationsContainer); | ||
function _interopRequireWildcard(obj) { if (obj && obj.__esModule) { return obj; } var cache = _getRequireWildcardCache(); if (cache && cache.has(obj)) { return cache.get(obj); } var newObj = {}; if (obj != null) { var hasPropertyDescriptor = Object.defineProperty && Object.getOwnPropertyDescriptor; for (var key in obj) { if (Object.prototype.hasOwnProperty.call(obj, key)) { var desc = hasPropertyDescriptor ? Object.getOwnPropertyDescriptor(obj, key) : null; if (desc && (desc.get || desc.set)) { Object.defineProperty(newObj, key, desc); } else { newObj[key] = obj[key]; } } } } newObj["default"] = obj; if (cache) { cache.set(obj, newObj); } return newObj; } | ||
var _constants = require('../constants'); | ||
function _typeof(obj) { if (typeof Symbol === "function" && typeof Symbol.iterator === "symbol") { _typeof = function _typeof(obj) { return typeof obj; }; } else { _typeof = function _typeof(obj) { return obj && typeof Symbol === "function" && obj.constructor === Symbol && obj !== Symbol.prototype ? "symbol" : typeof obj; }; } return _typeof(obj); } | ||
function _interopRequireDefault(obj) { return obj && obj.__esModule ? obj : { default: obj }; } | ||
function _classCallCheck(instance, Constructor) { if (!(instance instanceof Constructor)) { throw new TypeError("Cannot call a class as a function"); } } | ||
function _possibleConstructorReturn(self, call) { if (!self) { throw new ReferenceError("this hasn't been initialised - super() hasn't been called"); } return call && (typeof call === "object" || typeof call === "function") ? call : self; } | ||
function _defineProperties(target, props) { for (var i = 0; i < props.length; i++) { var descriptor = props[i]; descriptor.enumerable = descriptor.enumerable || false; descriptor.configurable = true; if ("value" in descriptor) descriptor.writable = true; Object.defineProperty(target, descriptor.key, descriptor); } } | ||
function _inherits(subClass, superClass) { if (typeof superClass !== "function" && superClass !== null) { throw new TypeError("Super expression must either be null or a function, not " + typeof superClass); } subClass.prototype = Object.create(superClass && superClass.prototype, { constructor: { value: subClass, enumerable: false, writable: true, configurable: true } }); if (superClass) Object.setPrototypeOf ? Object.setPrototypeOf(subClass, superClass) : subClass.__proto__ = superClass; } | ||
function _createClass(Constructor, protoProps, staticProps) { if (protoProps) _defineProperties(Constructor.prototype, protoProps); if (staticProps) _defineProperties(Constructor, staticProps); return Constructor; } | ||
var NotificationsSystem = exports.NotificationsSystem = function (_Component) { | ||
function _possibleConstructorReturn(self, call) { if (call && (_typeof(call) === "object" || typeof call === "function")) { return call; } return _assertThisInitialized(self); } | ||
function _assertThisInitialized(self) { if (self === void 0) { throw new ReferenceError("this hasn't been initialised - super() hasn't been called"); } return self; } | ||
function _getPrototypeOf(o) { _getPrototypeOf = Object.setPrototypeOf ? Object.getPrototypeOf : function _getPrototypeOf(o) { return o.__proto__ || Object.getPrototypeOf(o); }; return _getPrototypeOf(o); } | ||
function _inherits(subClass, superClass) { if (typeof superClass !== "function" && superClass !== null) { throw new TypeError("Super expression must either be null or a function"); } subClass.prototype = Object.create(superClass && superClass.prototype, { constructor: { value: subClass, writable: true, configurable: true } }); if (superClass) _setPrototypeOf(subClass, superClass); } | ||
function _setPrototypeOf(o, p) { _setPrototypeOf = Object.setPrototypeOf || function _setPrototypeOf(o, p) { o.__proto__ = p; return o; }; return _setPrototypeOf(o, p); } | ||
var NotificationsSystem = | ||
/*#__PURE__*/ | ||
function (_Component) { | ||
_inherits(NotificationsSystem, _Component); | ||
function NotificationsSystem() { | ||
var _ref; | ||
var _getPrototypeOf2; | ||
var _temp, _this, _ret; | ||
var _this; | ||
_classCallCheck(this, NotificationsSystem); | ||
for (var _len = arguments.length, args = Array(_len), _key = 0; _key < _len; _key++) { | ||
for (var _len = arguments.length, args = new Array(_len), _key = 0; _key < _len; _key++) { | ||
args[_key] = arguments[_key]; | ||
} | ||
return _ret = (_temp = (_this = _possibleConstructorReturn(this, (_ref = NotificationsSystem.__proto__ || Object.getPrototypeOf(NotificationsSystem)).call.apply(_ref, [this].concat(args))), _this), _this.state = { | ||
_this = _possibleConstructorReturn(this, (_getPrototypeOf2 = _getPrototypeOf(NotificationsSystem)).call.apply(_getPrototypeOf2, [this].concat(args))); | ||
_this.state = { | ||
windowWidth: window.innerWidth | ||
}, _this._updateWindowWidth = function () { | ||
_this.setState({ windowWidth: window.innerWidth }); | ||
}, _this._renderNotificationsContainers = function () { | ||
}; | ||
_this._updateWindowWidth = function () { | ||
_this.setState({ | ||
windowWidth: window.innerWidth | ||
}); | ||
}; | ||
_this._renderNotificationsContainers = function () { | ||
var _this$props = _this.props, | ||
@@ -60,3 +77,2 @@ theme = _this$props.theme, | ||
var windowWidth = _this.state.windowWidth; | ||
var positions = (0, _helpers.mapObjectValues)(_constants.POSITIONS); | ||
@@ -68,7 +84,7 @@ var containers = []; | ||
notifications = notifications.filter(filter); | ||
} | ||
} // render all notifications in the same container at the top for small screens | ||
// render all notifications in the same container at the top for small screens | ||
if (windowWidth < theme.smallScreenMin) { | ||
return _react2.default.createElement(_NotificationsContainer2.default, { | ||
return _react["default"].createElement(_NotificationsContainer["default"], { | ||
key: theme.smallScreenPosition || _constants.POSITIONS.top, | ||
@@ -85,4 +101,3 @@ position: theme.smallScreenPosition || _constants.POSITIONS.top, | ||
}); | ||
return _react2.default.createElement(_NotificationsContainer2.default, { | ||
return _react["default"].createElement(_NotificationsContainer["default"], { | ||
key: position, | ||
@@ -95,9 +110,10 @@ position: position, | ||
return containers; | ||
}, _temp), _possibleConstructorReturn(_this, _ret); | ||
}; | ||
return _this; | ||
} | ||
_createClass(NotificationsSystem, [{ | ||
key: 'componentDidMount', | ||
key: "componentDidMount", | ||
/** | ||
@@ -110,3 +126,2 @@ * Add resize listener to update window width when the window is resized | ||
} | ||
/** | ||
@@ -118,7 +133,6 @@ * Remove resize listener | ||
}, { | ||
key: 'componentWillUnmount', | ||
key: "componentWillUnmount", | ||
value: function componentWillUnmount() { | ||
window.removeEventListener('resize', this._updateWindowWidth); | ||
} | ||
/** | ||
@@ -130,13 +144,5 @@ * Update window width | ||
/** | ||
* Render notifications containers | ||
* @returns {XML} | ||
* @private | ||
*/ | ||
}, { | ||
key: 'render', | ||
key: "render", | ||
/** | ||
@@ -148,8 +154,5 @@ * Render | ||
var className = this.props.theme.notificationsSystem.className; | ||
return _react2.default.createElement( | ||
'div', | ||
{ className: className }, | ||
this._renderNotificationsContainers() | ||
); | ||
return _react["default"].createElement("div", { | ||
className: className | ||
}, this._renderNotificationsContainers()); | ||
} | ||
@@ -160,3 +163,2 @@ }]); | ||
}(_react.Component); | ||
/** | ||
@@ -169,10 +171,11 @@ * Map state to props | ||
exports.NotificationsSystem = NotificationsSystem; | ||
NotificationsSystem.propTypes = { | ||
notifications: _propTypes2.default.array.isRequired, | ||
filter: _propTypes2.default.func, | ||
theme: _propTypes2.default.shape({ | ||
smallScreenMin: _propTypes2.default.number.isRequired, | ||
smallScreenPosition: _propTypes2.default.oneOf([_constants.POSITIONS.top, _constants.POSITIONS.bottom]), | ||
notificationsSystem: _propTypes2.default.shape({ | ||
className: _propTypes2.default.string | ||
notifications: _propTypes["default"].array.isRequired, | ||
filter: _propTypes["default"].func, | ||
theme: _propTypes["default"].shape({ | ||
smallScreenMin: _propTypes["default"].number.isRequired, | ||
smallScreenPosition: _propTypes["default"].oneOf([_constants.POSITIONS.top, _constants.POSITIONS.bottom]), | ||
notificationsSystem: _propTypes["default"].shape({ | ||
className: _propTypes["default"].string | ||
}) | ||
@@ -184,2 +187,3 @@ }).isRequired | ||
}; | ||
function mapStateToProps(state) { | ||
@@ -191,2 +195,4 @@ return { | ||
exports.default = (0, _reactRedux.connect)(mapStateToProps)(NotificationsSystem); | ||
var _default = (0, _reactRedux.connect)(mapStateToProps)(NotificationsSystem); | ||
exports["default"] = _default; |
@@ -1,2 +0,2 @@ | ||
'use strict'; | ||
"use strict"; | ||
@@ -6,10 +6,15 @@ Object.defineProperty(exports, "__esModule", { | ||
}); | ||
var DEFAULT_STATUS = exports.DEFAULT_STATUS = 'default'; | ||
var INFO_STATUS = exports.INFO_STATUS = 'info'; | ||
var SUCCESS_STATUS = exports.SUCCESS_STATUS = 'success'; | ||
var WARNING_STATUS = exports.WARNING_STATUS = 'warning'; | ||
var ERROR_STATUS = exports.ERROR_STATUS = 'error'; | ||
var STATUS = exports.STATUS = { | ||
default: DEFAULT_STATUS, | ||
exports.DEFAULT_NOTIFICATION = exports.POSITIONS = exports.BOTTOM_RIGHT_POSITION = exports.BOTTOM_LEFT_POSITION = exports.BOTTOM_CENTER = exports.BOTTOM = exports.TOP_RIGHT_POSITION = exports.TOP_LEFT_POSITION = exports.TOP_CENTER = exports.TOP = exports.STATUS = exports.ERROR_STATUS = exports.WARNING_STATUS = exports.SUCCESS_STATUS = exports.INFO_STATUS = exports.DEFAULT_STATUS = void 0; | ||
var DEFAULT_STATUS = 'default'; | ||
exports.DEFAULT_STATUS = DEFAULT_STATUS; | ||
var INFO_STATUS = 'info'; | ||
exports.INFO_STATUS = INFO_STATUS; | ||
var SUCCESS_STATUS = 'success'; | ||
exports.SUCCESS_STATUS = SUCCESS_STATUS; | ||
var WARNING_STATUS = 'warning'; | ||
exports.WARNING_STATUS = WARNING_STATUS; | ||
var ERROR_STATUS = 'error'; | ||
exports.ERROR_STATUS = ERROR_STATUS; | ||
var STATUS = { | ||
"default": DEFAULT_STATUS, | ||
info: INFO_STATUS, | ||
@@ -20,13 +25,20 @@ success: SUCCESS_STATUS, | ||
}; | ||
var TOP = exports.TOP = 't'; | ||
var TOP_CENTER = exports.TOP_CENTER = 'tc'; | ||
var TOP_LEFT_POSITION = exports.TOP_LEFT_POSITION = 'tl'; | ||
var TOP_RIGHT_POSITION = exports.TOP_RIGHT_POSITION = 'tr'; | ||
var BOTTOM = exports.BOTTOM = 'b'; | ||
var BOTTOM_CENTER = exports.BOTTOM_CENTER = 'bc'; | ||
var BOTTOM_LEFT_POSITION = exports.BOTTOM_LEFT_POSITION = 'bl'; | ||
var BOTTOM_RIGHT_POSITION = exports.BOTTOM_RIGHT_POSITION = 'br'; | ||
var POSITIONS = exports.POSITIONS = { | ||
exports.STATUS = STATUS; | ||
var TOP = 't'; | ||
exports.TOP = TOP; | ||
var TOP_CENTER = 'tc'; | ||
exports.TOP_CENTER = TOP_CENTER; | ||
var TOP_LEFT_POSITION = 'tl'; | ||
exports.TOP_LEFT_POSITION = TOP_LEFT_POSITION; | ||
var TOP_RIGHT_POSITION = 'tr'; | ||
exports.TOP_RIGHT_POSITION = TOP_RIGHT_POSITION; | ||
var BOTTOM = 'b'; | ||
exports.BOTTOM = BOTTOM; | ||
var BOTTOM_CENTER = 'bc'; | ||
exports.BOTTOM_CENTER = BOTTOM_CENTER; | ||
var BOTTOM_LEFT_POSITION = 'bl'; | ||
exports.BOTTOM_LEFT_POSITION = BOTTOM_LEFT_POSITION; | ||
var BOTTOM_RIGHT_POSITION = 'br'; | ||
exports.BOTTOM_RIGHT_POSITION = BOTTOM_RIGHT_POSITION; | ||
var POSITIONS = { | ||
top: TOP, | ||
@@ -40,6 +52,6 @@ topCenter: TOP_CENTER, | ||
bottomRight: BOTTOM_RIGHT_POSITION | ||
}; | ||
}; // default value for notifications | ||
// default value for notifications | ||
var DEFAULT_NOTIFICATION = exports.DEFAULT_NOTIFICATION = { | ||
exports.POSITIONS = POSITIONS; | ||
var DEFAULT_NOTIFICATION = { | ||
status: DEFAULT_STATUS, | ||
@@ -51,2 +63,3 @@ position: TOP_RIGHT_POSITION, | ||
closeButton: false | ||
}; | ||
}; | ||
exports.DEFAULT_NOTIFICATION = DEFAULT_NOTIFICATION; |
@@ -1,2 +0,2 @@ | ||
'use strict'; | ||
"use strict"; | ||
@@ -12,12 +12,7 @@ Object.defineProperty(exports, "__esModule", { | ||
var _constants = require('../constants'); | ||
var _constants = require("../constants"); | ||
/** | ||
* Convert status in a understandable status for the Notification component | ||
* @param {String|Number} status | ||
* @returns {String} status an understandable status | ||
*/ | ||
function convertStatus(status) { | ||
var reHttpStatusCode = /^\d{3}$/; | ||
// convert HTTP status code | ||
var reHttpStatusCode = /^\d{3}$/; // convert HTTP status code | ||
if (reHttpStatusCode.test(status)) { | ||
@@ -27,4 +22,6 @@ switch (true) { | ||
return _constants.STATUS.info; | ||
case /^2/.test(status): | ||
return _constants.STATUS.success; | ||
case /^(4|5)/.test(status): | ||
@@ -34,5 +31,5 @@ return _constants.STATUS.error; | ||
} | ||
return status; | ||
} | ||
/** | ||
@@ -44,5 +41,7 @@ * Create a Timer | ||
*/ | ||
function Timer(delay, callback) { | ||
var timerId = void 0; | ||
var start = void 0; | ||
var timerId; | ||
var start; | ||
var remaining = delay; | ||
@@ -54,2 +53,3 @@ | ||
}; | ||
this.resume = function () { | ||
@@ -65,3 +65,2 @@ start = new Date(); | ||
} | ||
/** | ||
@@ -72,2 +71,4 @@ * Treat data of a notification | ||
*/ | ||
function treatNotification(notification) { | ||
@@ -86,3 +87,2 @@ if (notification.dismissAfter) { | ||
} | ||
/** | ||
@@ -94,2 +94,4 @@ * Preload an image | ||
*/ | ||
function preloadImage(url, cb) { | ||
@@ -102,3 +104,2 @@ var image = new Image(); | ||
} | ||
/** | ||
@@ -109,10 +110,10 @@ * Return values of an Object in an Array | ||
*/ | ||
function mapObjectValues(obj) { | ||
var array = []; | ||
Object.keys(obj).forEach(function (key) { | ||
return array.push(obj[key]); | ||
}); | ||
return array; | ||
} |
@@ -1,2 +0,2 @@ | ||
'use strict'; | ||
"use strict"; | ||
@@ -6,29 +6,84 @@ Object.defineProperty(exports, "__esModule", { | ||
}); | ||
exports.removeNotifications = exports.removeNotification = exports.updateNotification = exports.notify = exports.addNotification = exports.reducer = exports.types = exports.actions = exports.POSITIONS = exports.STATUS = exports.DEFAULT_NOTIFICATION = undefined; | ||
Object.defineProperty(exports, "STATUS", { | ||
enumerable: true, | ||
get: function get() { | ||
return _constants.STATUS; | ||
} | ||
}); | ||
Object.defineProperty(exports, "POSITIONS", { | ||
enumerable: true, | ||
get: function get() { | ||
return _constants.POSITIONS; | ||
} | ||
}); | ||
Object.defineProperty(exports, "DEFAULT_NOTIFICATION", { | ||
enumerable: true, | ||
get: function get() { | ||
return _constants.DEFAULT_NOTIFICATION; | ||
} | ||
}); | ||
Object.defineProperty(exports, "reducer", { | ||
enumerable: true, | ||
get: function get() { | ||
return _notifications["default"]; | ||
} | ||
}); | ||
Object.defineProperty(exports, "actions", { | ||
enumerable: true, | ||
get: function get() { | ||
return _notifications.actions; | ||
} | ||
}); | ||
Object.defineProperty(exports, "types", { | ||
enumerable: true, | ||
get: function get() { | ||
return _notifications.types; | ||
} | ||
}); | ||
Object.defineProperty(exports, "addNotification", { | ||
enumerable: true, | ||
get: function get() { | ||
return _notifications.addNotification; | ||
} | ||
}); | ||
Object.defineProperty(exports, "notify", { | ||
enumerable: true, | ||
get: function get() { | ||
return _notifications.notify; | ||
} | ||
}); | ||
Object.defineProperty(exports, "updateNotification", { | ||
enumerable: true, | ||
get: function get() { | ||
return _notifications.updateNotification; | ||
} | ||
}); | ||
Object.defineProperty(exports, "removeNotification", { | ||
enumerable: true, | ||
get: function get() { | ||
return _notifications.removeNotification; | ||
} | ||
}); | ||
Object.defineProperty(exports, "removeNotifications", { | ||
enumerable: true, | ||
get: function get() { | ||
return _notifications.removeNotifications; | ||
} | ||
}); | ||
exports["default"] = void 0; | ||
var _NotificationsSystem = require('./components/NotificationsSystem'); | ||
var _NotificationsSystem = _interopRequireDefault(require("./components/NotificationsSystem")); | ||
var _NotificationsSystem2 = _interopRequireDefault(_NotificationsSystem); | ||
var _constants = require("./constants"); | ||
var _constants = require('./constants'); | ||
var _notifications = _interopRequireWildcard(require("./store/notifications")); | ||
var _notifications = require('./store/notifications'); | ||
function _getRequireWildcardCache() { if (typeof WeakMap !== "function") return null; var cache = new WeakMap(); _getRequireWildcardCache = function _getRequireWildcardCache() { return cache; }; return cache; } | ||
var _notifications2 = _interopRequireDefault(_notifications); | ||
function _interopRequireWildcard(obj) { if (obj && obj.__esModule) { return obj; } var cache = _getRequireWildcardCache(); if (cache && cache.has(obj)) { return cache.get(obj); } var newObj = {}; if (obj != null) { var hasPropertyDescriptor = Object.defineProperty && Object.getOwnPropertyDescriptor; for (var key in obj) { if (Object.prototype.hasOwnProperty.call(obj, key)) { var desc = hasPropertyDescriptor ? Object.getOwnPropertyDescriptor(obj, key) : null; if (desc && (desc.get || desc.set)) { Object.defineProperty(newObj, key, desc); } else { newObj[key] = obj[key]; } } } } newObj["default"] = obj; if (cache) { cache.set(obj, newObj); } return newObj; } | ||
function _interopRequireDefault(obj) { return obj && obj.__esModule ? obj : { default: obj }; } | ||
function _interopRequireDefault(obj) { return obj && obj.__esModule ? obj : { "default": obj }; } | ||
exports.DEFAULT_NOTIFICATION = _constants.DEFAULT_NOTIFICATION; | ||
exports.STATUS = _constants.STATUS; | ||
exports.POSITIONS = _constants.POSITIONS; | ||
exports.actions = _notifications.actions; | ||
exports.types = _notifications.types; | ||
exports.reducer = _notifications2.default; | ||
exports.addNotification = _notifications.addNotification; | ||
exports.notify = _notifications.notify; | ||
exports.updateNotification = _notifications.updateNotification; | ||
exports.removeNotification = _notifications.removeNotification; | ||
exports.removeNotifications = _notifications.removeNotifications; | ||
// NotificationsSystem React component | ||
exports.default = _NotificationsSystem2.default; | ||
var _default = _NotificationsSystem["default"]; | ||
exports["default"] = _default; |
@@ -1,2 +0,2 @@ | ||
'use strict'; | ||
"use strict"; | ||
@@ -6,15 +6,22 @@ Object.defineProperty(exports, "__esModule", { | ||
}); | ||
exports.types = exports.actions = exports.updateNotification = exports.notify = exports.addNotification = undefined; | ||
exports.removeNotification = removeNotification; | ||
exports.removeNotifications = removeNotifications; | ||
exports["default"] = exports.types = exports.actions = exports.updateNotification = exports.notify = exports.addNotification = void 0; | ||
var _helpers = require('../helpers'); | ||
var _helpers = require("../helpers"); | ||
var _constants = require('../constants'); | ||
var _constants = require("../constants"); | ||
function _toConsumableArray(arr) { if (Array.isArray(arr)) { for (var i = 0, arr2 = Array(arr.length); i < arr.length; i++) { arr2[i] = arr[i]; } return arr2; } else { return Array.from(arr); } } | ||
var _this = void 0; | ||
// An array to store notifications object | ||
var INITIAL_STATE = []; | ||
// Action types | ||
function _toConsumableArray(arr) { return _arrayWithoutHoles(arr) || _iterableToArray(arr) || _nonIterableSpread(); } | ||
function _nonIterableSpread() { throw new TypeError("Invalid attempt to spread non-iterable instance"); } | ||
function _iterableToArray(iter) { if (Symbol.iterator in Object(iter) || Object.prototype.toString.call(iter) === "[object Arguments]") return Array.from(iter); } | ||
function _arrayWithoutHoles(arr) { if (Array.isArray(arr)) { for (var i = 0, arr2 = new Array(arr.length); i < arr.length; i++) { arr2[i] = arr[i]; } return arr2; } } | ||
var INITIAL_STATE = []; // Action types | ||
var ADD_NOTIFICATION = 'ADD_NOTIFICATION'; | ||
@@ -24,3 +31,2 @@ var UPDATE_NOTIFICATION = 'UPDATE_NOTIFICATION'; | ||
var REMOVE_NOTIFICATIONS = 'REMOVE_NOTIFICATIONS'; | ||
/** | ||
@@ -34,3 +40,4 @@ * Add a notification (thunk action creator) | ||
*/ | ||
var addNotification = exports.addNotification = function addNotification(notification) { | ||
var addNotification = function addNotification(notification) { | ||
return function (dispatch) { | ||
@@ -40,14 +47,15 @@ if (!notification.id) { | ||
} | ||
notification = (0, _helpers.treatNotification)(notification); | ||
// if there is an image, we preload it | ||
notification = (0, _helpers.treatNotification)(notification); // if there is an image, we preload it | ||
// and add notification when image is loaded | ||
if (notification.image) { | ||
(0, _helpers.preloadImage)(notification.image, dispatch.bind(undefined, _addNotification(notification))); | ||
(0, _helpers.preloadImage)(notification.image, dispatch.bind(_this, _addNotification(notification))); | ||
} else { | ||
dispatch(_addNotification(notification)); | ||
} | ||
return notification; | ||
}; | ||
}; | ||
/** | ||
@@ -60,2 +68,6 @@ * Add a notification (action creator) | ||
*/ | ||
exports.addNotification = addNotification; | ||
function _addNotification(notification) { | ||
@@ -67,3 +79,2 @@ return { | ||
} | ||
/** | ||
@@ -74,3 +85,5 @@ * Update or create a notification | ||
*/ | ||
var notify = exports.notify = function notify(notification) { | ||
var notify = function notify(notification) { | ||
return function (dispatch, getState) { | ||
@@ -89,3 +102,2 @@ var notifications = getState().notifications; | ||
}; | ||
/** | ||
@@ -99,3 +111,7 @@ * Update a notification (thunk action creator) | ||
*/ | ||
var updateNotification = exports.updateNotification = function updateNotification(notification) { | ||
exports.notify = notify; | ||
var updateNotification = function updateNotification(notification) { | ||
return function (dispatch, getState) { | ||
@@ -111,16 +127,14 @@ if (!notification.id) { | ||
var currNotification = notifications[index]; | ||
notification = (0, _helpers.treatNotification)(notification); // if image is different, then we preload it | ||
// and update notification when image is loaded | ||
notification = (0, _helpers.treatNotification)(notification); | ||
// if image is different, then we preload it | ||
// and update notification when image is loaded | ||
if (notification.image && (!currNotification.image || currNotification.image && notification.image !== currNotification.image)) { | ||
(0, _helpers.preloadImage)(notification.image, dispatch.bind(undefined, _updateNotification(notification))); | ||
(0, _helpers.preloadImage)(notification.image, dispatch.bind(_this, _updateNotification(notification))); | ||
} else { | ||
dispatch(_updateNotification(notification)); | ||
} | ||
return notification; | ||
}; | ||
}; | ||
/** | ||
@@ -133,2 +147,6 @@ * Update a notification (action creator) | ||
*/ | ||
exports.updateNotification = updateNotification; | ||
function _updateNotification(notification) { | ||
@@ -140,3 +158,2 @@ return { | ||
} | ||
/** | ||
@@ -148,2 +165,4 @@ * Remove a notification (action creator) | ||
*/ | ||
function removeNotification(notification) { | ||
@@ -155,3 +174,2 @@ return { | ||
} | ||
/** | ||
@@ -162,2 +180,4 @@ * Remove all notifications (action creator) | ||
*/ | ||
function removeNotifications() { | ||
@@ -167,6 +187,6 @@ return { | ||
}; | ||
} | ||
} // Action creators | ||
// Action creators | ||
var actions = exports.actions = { | ||
var actions = { | ||
addNotification: addNotification, | ||
@@ -176,6 +196,6 @@ updateNotification: updateNotification, | ||
removeNotifications: removeNotifications | ||
}; | ||
}; // Actions types | ||
// Actions types | ||
var types = exports.types = { | ||
exports.actions = actions; | ||
var types = { | ||
ADD_NOTIFICATION: ADD_NOTIFICATION, | ||
@@ -185,13 +205,13 @@ UPDATE_NOTIFICATION: UPDATE_NOTIFICATION, | ||
REMOVE_NOTIFICATIONS: REMOVE_NOTIFICATIONS | ||
}; | ||
}; // Reducers | ||
// Reducers | ||
exports.types = types; | ||
exports.default = function () { | ||
var _default = function _default() { | ||
var defaultNotification = arguments.length > 0 && arguments[0] !== undefined ? arguments[0] : _constants.DEFAULT_NOTIFICATION; | ||
return function () { | ||
var state = arguments.length > 0 && arguments[0] !== undefined ? arguments[0] : INITIAL_STATE; | ||
var _ref = arguments[1]; | ||
var type = _ref.type, | ||
var _ref = arguments.length > 1 ? arguments[1] : undefined, | ||
type = _ref.type, | ||
payload = _ref.payload; | ||
@@ -201,4 +221,7 @@ | ||
case ADD_NOTIFICATION: | ||
var notification = Object.assign({}, defaultNotification, payload); | ||
return [].concat(_toConsumableArray(state), [notification]); | ||
{ | ||
var notification = Object.assign({}, defaultNotification, payload); | ||
return [].concat(_toConsumableArray(state), [notification]); | ||
} | ||
case UPDATE_NOTIFICATION: | ||
@@ -209,4 +232,6 @@ return state.map(function (notification) { | ||
} | ||
return notification; | ||
}); | ||
case REMOVE_NOTIFICATION: | ||
@@ -216,4 +241,6 @@ return state.filter(function (notification) { | ||
}); | ||
case REMOVE_NOTIFICATIONS: | ||
return []; | ||
default: | ||
@@ -223,2 +250,4 @@ return state; | ||
}; | ||
}; | ||
}; | ||
exports["default"] = _default; |
130
package.json
{ | ||
"name": "reapop", | ||
"version": "2.0.1", | ||
"version": "2.1.0", | ||
"description": "A React and Redux notifications system", | ||
"main": "lib/index.js", | ||
"scripts": { | ||
"start": "cd demo && node index.js", | ||
"compile": "cd demo && ../node_modules/.bin/webpack --config=./build/webpack.config.js && cp src/index.html dist/ && cp -R src/static dist/", | ||
"lint": "./node_modules/.bin/eslint .", | ||
"lint:fix": "./node_modules/.bin/eslint . --fix", | ||
"react:clean": "npm uninstall react react-dom react-addons-test-utils", | ||
"redux:clean": "npm uninstall redux", | ||
"react-redux:clean": "rm -rf node_modules/react-redux", | ||
"react:14": "npm run react:clean && npm i react@0.14 react-dom@0.14 react-addons-test-utils@0.14", | ||
"react:15": "npm run react:clean && npm i react@15 react-dom@15 react-addons-test-utils@15", | ||
"react:16": "npm run react:clean && npm i react@16.5.2 react-dom@16", | ||
"redux:2": "npm run redux:clean && npm i redux@2", | ||
"redux:3": "npm run redux:clean && npm i redux@3", | ||
"redux:4": "npm run redux:clean && npm i redux@4", | ||
"react-redux:2": "npm run react-redux:clean && npm i react-redux@2", | ||
"react-redux:3.1": "npm run react-redux:clean && npm i react-redux@3.1", | ||
"react-redux:4": "npm run react-redux:clean && npm i react-redux@4", | ||
"react-redux:5": "npm run react-redux:clean && npm i react-redux@5.0.6", | ||
"test": "babel-node ./node_modules/karma/bin/karma start ./build/karma.conf.js --reporters mocha", | ||
"test:all": "npm run react:14 && npm run redux:2 && npm run react-redux:2 && npm t && npm run react:14 && npm run redux:3 && npm run react-redux:3.1 && npm t && npm run react:15 && npm run redux:3 && npm run react-redux:4 && npm t && npm run react:15 && npm run redux:4 && npm run react-redux:5 && npm t && npm run react:16 && npm run redux:3 && npm run react-redux:5 && npm t && npm run react:16 && npm run redux:4 && npm run react-redux:5 && npm t", | ||
"test:coverage": "npm test -- --reporters mocha,coverage", | ||
"test:coveralls": "npm test -- --reporters mocha,coverage,coveralls", | ||
"test:watch": "npm test -- --singleRun=false --reporters mocha", | ||
"prepublish": "node_modules/babel-cli/bin/babel.js src --out-dir lib" | ||
"start": "node demo/index.js", | ||
"compile": "npx webpack --config=./demo/webpack.config.js && cp demo/src/index.html demo/dist/ && cp -R demo/src/static demo/dist/", | ||
"lint": "npx eslint .", | ||
"lint:fix": "npx eslint . --fix", | ||
"test": "npx jest", | ||
"test:all": "./scripts/test-all.sh", | ||
"test:coverage": "npm test -- --coverage", | ||
"test:coveralls": "npm test -- --coverage --coverageReporters=text-lcov | coveralls", | ||
"test:watch": "npm test -- --watch", | ||
"prepublish": "./node_modules/@babel/cli/bin/babel.js src --out-dir lib" | ||
}, | ||
@@ -51,3 +38,3 @@ "repository": { | ||
"redux": "^2.0.0 || ^3.0.0 || ^4.0.0", | ||
"react-redux": "^2.0.0 || ^3.1.0 || ^4.0.0 || ^5.0.6", | ||
"react-redux": "^2.0.0 || ^3.1.0 || ^4.0.0 || ^5.0.6 || ^7.0.1", | ||
"react": "^0.14.0 || ^15.0.0 || ^16.0.0", | ||
@@ -57,57 +44,50 @@ "redux-thunk": "^2.1.0" | ||
"devDependencies": { | ||
"babel-cli": "6.26.0", | ||
"babel-core": "6.26.0", | ||
"babel-eslint": "8.1.2", | ||
"babel-loader": "7.1.1", | ||
"babel-polyfill": "6.26.0", | ||
"babel-preset-es2015": "6.24.1", | ||
"babel-preset-react": "6.24.1", | ||
"babel-preset-stage-0": "6.24.1", | ||
"css-loader": "0.28.7", | ||
"enzyme": "^3.3.0", | ||
"enzyme-adapter-react-14": "1.0.5", | ||
"enzyme-adapter-react-15.4": "1.0.5", | ||
"enzyme-adapter-react-16": "1.6.0", | ||
"eslint": "5.7.0", | ||
"eslint-config-standard": "12.0.0", | ||
"eslint-config-standard-react": "5.0.0", | ||
"eslint-plugin-import": "2.8.0", | ||
"eslint-plugin-node": "5.2.1", | ||
"eslint-plugin-promise": "3.6.0", | ||
"eslint-plugin-react": "7.5.1", | ||
"eslint-plugin-standard": "3.0.1", | ||
"expect": "1.18.0", | ||
"express": "4.16.2", | ||
"@babel/cli": "7.6.2", | ||
"@babel/core": "7.6.2", | ||
"@babel/plugin-proposal-class-properties": "7.5.5", | ||
"@babel/preset-env": "7.6.2", | ||
"@babel/preset-react": "7.0.0", | ||
"babel-eslint": "10.0.3", | ||
"babel-loader": "8.0.6", | ||
"coveralls": "3.0.6", | ||
"css-loader": "2.1.1", | ||
"enzyme": "3.10.0", | ||
"enzyme-adapter-react-14": "1.4.0", | ||
"enzyme-adapter-react-15.4": "1.4.0", | ||
"enzyme-adapter-react-16": "1.14.0", | ||
"eslint": "6.4.0", | ||
"eslint-config-standard": "14.1.0", | ||
"eslint-config-standard-react": "9.2.0", | ||
"eslint-plugin-import": "2.18.2", | ||
"eslint-plugin-node": "10.0.0", | ||
"eslint-plugin-promise": "4.2.1", | ||
"eslint-plugin-react": "7.14.3", | ||
"eslint-plugin-standard": "4.0.0", | ||
"express": "4.17.1", | ||
"faker": "4.1.0", | ||
"file-loader": "1.1.6", | ||
"file-loader": "4.2.0", | ||
"font-awesome": "4.7.0", | ||
"html-webpack-plugin": "2.30.1", | ||
"identity-obj-proxy": "3.0.0", | ||
"import": "0.0.6", | ||
"isparta-loader": "2.0.0", | ||
"json-loader": "0.5.7", | ||
"karma": "2.0.0", | ||
"karma-chrome-launcher": "2.2.0", | ||
"karma-coverage": "1.1.1", | ||
"karma-coveralls": "1.1.2", | ||
"karma-mocha": "1.3.0", | ||
"karma-mocha-reporter": "2.2.5", | ||
"karma-phantomjs-launcher": "1.0.4", | ||
"karma-webpack": "2.0.9", | ||
"mocha": "4.1.0", | ||
"node-sass": "4.7.2", | ||
"istanbul-instrumenter-loader": "3.0.1", | ||
"jest": "24.9.0", | ||
"node-sass": "4.12.0", | ||
"phantomjs-prebuilt": "2.1.16", | ||
"prop-types": "15.6.0", | ||
"prop-types": "15.7.2", | ||
"react": "16.8.6", | ||
"react-addons-update": "15.6.2", | ||
"react-redux": "^5.0.6", | ||
"reapop-theme-bootstrap": "1.0.0", | ||
"reapop-theme-wybo": "1.0.1", | ||
"redux-mock-store": "1.4.0", | ||
"redux-thunk": "2.2.0", | ||
"requirejs": "2.3.5", | ||
"sass-loader": "6.0.6", | ||
"style-loader": "0.19.1", | ||
"url-loader": "0.6.2", | ||
"webpack": "1.13.0", | ||
"webpack-dev-middleware": "2.0.3", | ||
"webpack-hot-middleware": "2.21.0" | ||
"react-dom": "16.8.6", | ||
"react-redux": "7.1.1", | ||
"reapop-theme-bootstrap": "1.0.2", | ||
"reapop-theme-wybo": "1.0.2", | ||
"redux": "4.0.4", | ||
"redux-mock-store": "1.5.3", | ||
"redux-thunk": "2.3.0", | ||
"sass-loader": "8.0.0", | ||
"style-loader": "1.0.0", | ||
"url-loader": "2.1.0", | ||
"webpack": "4.41.0", | ||
"webpack-cli": "3.3.9", | ||
"webpack-dev-middleware": "3.7.2", | ||
"webpack-hot-middleware": "2.25.0" | ||
}, | ||
@@ -114,0 +94,0 @@ "dependencies": { |
@@ -24,3 +24,3 @@ # Reapop | ||
- [react](https://github.com/facebook/react) : **^0.14.0**, **^15.0.0** and **^16.0.0** | ||
- [react-redux](https://github.com/reactjs/react-redux) : **^2.0.0**, **^3.0.0**, **^4.0.0** and **^5.0.6** | ||
- [react-redux](https://github.com/reactjs/react-redux) : **^3.0.0**, **^4.0.0**, **^5.0.0** and **^7.0.0** | ||
- [redux](https://github.com/reactjs/redux) : **^2.0.0**, **^3.0.0** and **^4.0.0** | ||
@@ -30,6 +30,6 @@ | ||
Tested and works with : | ||
| [<img src="https://raw.githubusercontent.com/alrra/browser-logos/master/src/edge/edge_48x48.png" alt="IE / Edge" width="24px" height="24px" />](http://godban.github.io/browsers-support-badges/)</br>IE / Edge | [<img src="https://raw.githubusercontent.com/alrra/browser-logos/master/src/firefox/firefox_48x48.png" alt="Firefox" width="24px" height="24px" />](http://godban.github.io/browsers-support-badges/)</br>Firefox | [<img src="https://raw.githubusercontent.com/alrra/browser-logos/master/src/chrome/chrome_48x48.png" alt="Chrome" width="24px" height="24px" />](http://godban.github.io/browsers-support-badges/)</br>Chrome | [<img src="https://raw.githubusercontent.com/alrra/browser-logos/master/src/safari/safari_48x48.png" alt="Safari" width="24px" height="24px" />](http://godban.github.io/browsers-support-badges/)</br>Safari | [<img src="https://raw.githubusercontent.com/alrra/browser-logos/master/src/opera/opera_48x48.png" alt="Opera" width="24px" height="24px" />](http://godban.github.io/browsers-support-badges/)</br>Opera | | ||
| --------- | --------- | --------- | --------- | --------- | | ||
| IE10, IE11, Edge| last 2 versions| last 2 versions| last 2 versions| last 2 versions | ||
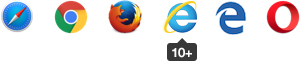 | ||
## Demo | ||
@@ -47,3 +47,3 @@ | ||
Follow this 4 steps to integrate Reapop to your application. | ||
Follow these 3 steps to integrate Reapop to your application. | ||
@@ -115,10 +115,2 @@ ### Integrate `NotificationsSystem` React component | ||
### Install and import `babel-polyfill` package | ||
This package use some ES6 features, to make it compatible in all browsers, you must : | ||
1. Install `babel-polyfill` package with `npm install --save-dev` | ||
2. Import `babel-polyfill` package at the root of your app with `import 'babel-polyfill';` | ||
## Usage | ||
@@ -125,0 +117,0 @@ |
51344
48
904
193