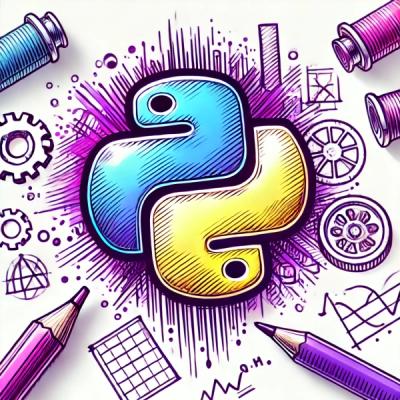
Security News
New Python Packaging Proposal Aims to Solve Phantom Dependency Problem with SBOMs
PEP 770 proposes adding SBOM support to Python packages to improve transparency and catch hidden non-Python dependencies that security tools often miss.
reason-react-context
Advanced tools
It seems to cover the base api. If there is something missing, please file an issue :D
This a an implementation of the new context react context api in pure ReasonML
It seems to cover the base api. If there is something missing, please file an issue :D
npm install --save reason-react-context
An example of using this is at https://github.com/Hehk/example-reason-react-context
Creating a context:
type t =
| Light
| Dark;
module Context =
ReasonReactContext.CreateContext(
{
type state = t;
let name = "Theme";
let defaultValue = Light;
}
);
Using the Provider module:
type action =
| ChangeTheme(Theme.t);
type state = {theme: Theme.t};
let component = ReasonReact.reducerComponent("App");
let make = _children => {
...component,
initialState: () => {theme: Light},
reducer: (action, _state) =>
switch action {
| ChangeTheme(newTheme) => ReasonReact.Update({theme: newTheme})
},
render: ({send, state}) =>
<div className="App">
<Theme.Context.Provider value=state.theme>
<Background>
<button onClick=(_e => send(ChangeTheme(state.theme === Dark ? Light : Dark)))>
(ReasonReact.stringToElement("Toggle Theme"))
</button>
<Title message="Reason Context" />
</Background>
</Theme.Context.Provider>
</div>
};
Using the Consumer module:
let component = ReasonReact.statelessComponent("background");
let make = children => {
...component,
render: _self =>
<Theme.Context.Consumer>
...(
theme =>
ReasonReact.createDomElement(
"div",
~props={"className": theme === Light ? "background-light" : "background-dark"},
children
)
)
</Theme.Context.Consumer>
};
FAQs
It seems to cover the base api. If there is something missing, please file an issue :D
We found that reason-react-context demonstrated a not healthy version release cadence and project activity because the last version was released a year ago. It has 1 open source maintainer collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
PEP 770 proposes adding SBOM support to Python packages to improve transparency and catch hidden non-Python dependencies that security tools often miss.
Security News
Socket CEO Feross Aboukhadijeh discusses open source security challenges, including zero-day attacks and supply chain risks, on the Cyber Security Council podcast.
Security News
Research
Socket researchers uncover how threat actors weaponize Out-of-Band Application Security Testing (OAST) techniques across the npm, PyPI, and RubyGems ecosystems to exfiltrate sensitive data.