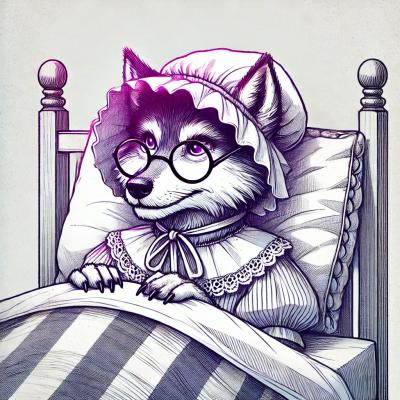
Security News
GitHub Removes Malicious Pull Requests Targeting Open Source Repositories
GitHub removed 27 malicious pull requests attempting to inject harmful code across multiple open source repositories, in another round of low-effort attacks.
Recharts is a composable charting library built on React components. It allows users to create various types of charts such as line, bar, area, pie, and more, using a declarative approach. It is easy to customize and extend, and it integrates well with the React ecosystem.
LineChart
This code sample demonstrates how to create a simple LineChart with two lines, using the Recharts library. The chart includes a grid, axes, tooltips, and a legend.
{"import { LineChart, Line, XAxis, YAxis, CartesianGrid, Tooltip, Legend } from 'recharts';\nconst data = [{name: 'Page A', uv: 400, pv: 2400, amt: 2400}];\n<LineChart width={600} height={300} data={data}><CartesianGrid strokeDasharray=\"3 3\"/><XAxis dataKey=\"name\"/><YAxis/><Tooltip/><Legend /><Line type=\"monotone\" dataKey=\"pv\" stroke=\"#8884d8\" /><Line type=\"monotone\" dataKey=\"uv\" stroke=\"#82ca9d\" /></LineChart>"}
BarChart
This code sample shows how to create a BarChart with two bars per data point. It includes similar features to the LineChart, such as a grid, axes, tooltips, and a legend.
{"import { BarChart, Bar, XAxis, YAxis, CartesianGrid, Tooltip, Legend } from 'recharts';\nconst data = [{name: 'Page A', uv: 400, pv: 2400, amt: 2400}];\n<BarChart width={600} height={300} data={data}><CartesianGrid strokeDasharray=\"3 3\"/><XAxis dataKey=\"name\"/><YAxis/><Tooltip/><Legend /><Bar dataKey=\"pv\" fill=\"#8884d8\" /><Bar dataKey=\"uv\" fill=\"#82ca9d\" /></BarChart>"}
PieChart
This code sample illustrates how to create a PieChart. It includes a Pie component with labels, a Tooltip for additional information on hover, and a Legend.
{"import { PieChart, Pie, Tooltip, Legend } from 'recharts';\nconst data = [{name: 'Group A', value: 400}, {name: 'Group B', value: 300}];\n<PieChart width={800} height={400}><Pie dataKey=\"value\" isAnimationActive={false} data={data} cx={200} cy={200} outerRadius={80} fill=\"#8884d8\" label /><Tooltip/><Legend /></PieChart>"}
Chart.js is a popular canvas-based charting library that provides a wide range of chart types and is highly customizable. It is not React-specific and requires wrappers like 'react-chartjs-2' to integrate with React applications. Compared to Recharts, Chart.js may have better performance for complex animations and large datasets due to its canvas rendering.
Victory is another React chart library that offers a set of composable components for building interactive data visualizations. It is similar to Recharts in its React-centric approach but differs in its API design and customization options. Victory is known for its flexibility and extensive customization capabilities.
Highcharts is a comprehensive charting library that has been around for a long time. The 'highcharts-react-official' package is the official React wrapper for Highcharts. Highcharts offers a vast array of chart types and features, and it is known for its robustness and interactivity. However, it is not as tightly integrated with React as Recharts and may require a commercial license for certain uses.
Recharts is a Redefined chart library built with React and D3.
The main purpose of this library is to help you to write charts in React applications without any pain. Main principles of Recharts are:
Documentation at recharts.org and our storybook (WIP)
Please see the wiki for FAQ.
All development is done on the master
branch. The current latest release and storybook documentation reflects what is on the release
branch.
<LineChart width={400} height={400} data={data} margin={{ top: 5, right: 20, left: 10, bottom: 5 }}>
<XAxis dataKey="name" />
<Tooltip />
<CartesianGrid stroke="#f5f5f5" />
<Line type="monotone" dataKey="uv" stroke="#ff7300" yAxisId={0} />
<Line type="monotone" dataKey="pv" stroke="#387908" yAxisId={1} />
</LineChart>
All the components of Recharts are clearly separated. The lineChart is composed of x axis, tooltip, grid, and line items, and each of them is an independent React Component. The clear separation and composition of components is one of the principle Recharts follows.
NPM is the easiest and fastest way to get started using Recharts. It is also the recommended installation method when building single-page applications (SPAs). It pairs nicely with a CommonJS module bundler such as Webpack.
# latest stable
$ npm install recharts
The UMD build is also available on unpkg.com:
<script src="https://unpkg.com/react/umd/react.production.min.js"></script>
<script src="https://unpkg.com/react-dom/umd/react-dom.production.min.js"></script>
<script src="https://unpkg.com/recharts/umd/Recharts.min.js"></script>
Then you can find the library on window.Recharts
.
$ git clone https://github.com/recharts/recharts.git
$ cd recharts
$ npm install
$ npm run build
To examine the demos in your local build, execute:
$ npm run[-script] demo
and then browse to http://localhost:3000.
We are in the process of unifying documentation and examples in storybook. To run it locally, execute
$ npm run[-script] storybook
and then browse to http://localhost:6006.
Releases are automated via GH Actions - when a new release is created in GH, CI will trigger that:
npm publish
Version increments and tagging are not automated at this time.
Until we can automate more, it should be preferred to test as close to the results of npm publish
as we possibly can. This ensures we don't publish unintended breaking changes. One way to do that is using yalc
- npm i -g yalc
.
yalc publish
in rechartsyalc add recharts
in your test package (ex: in a vite or webpack reach app with recharts installed, imported, and your recent changes used)npm install
Thanks to Chromatic for providing the visual testing platform that helps us review UI changes and catch visual regressions.
Copyright (c) 2015-2023 Recharts Group.
FAQs
React charts
The npm package recharts receives a total of 1,359,932 weekly downloads. As such, recharts popularity was classified as popular.
We found that recharts demonstrated a healthy version release cadence and project activity because the last version was released less than a year ago. It has 3 open source maintainers collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
GitHub removed 27 malicious pull requests attempting to inject harmful code across multiple open source repositories, in another round of low-effort attacks.
Security News
RubyGems.org has added a new "maintainer" role that allows for publishing new versions of gems. This new permission type is aimed at improving security for gem owners and the service overall.
Security News
Node.js will be enforcing stricter semver-major PR policies a month before major releases to enhance stability and ensure reliable release candidates.