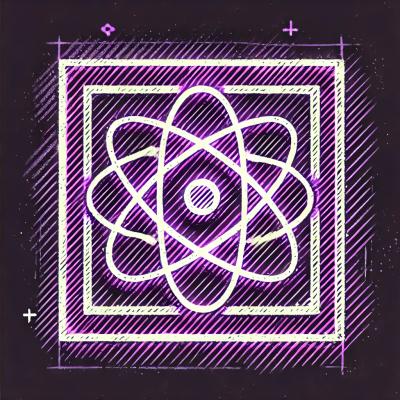
Security News
Create React App Officially Deprecated Amid React 19 Compatibility Issues
Create React App is officially deprecated due to React 19 issues and lack of maintenance—developers should switch to Vite or other modern alternatives.
recoil-custom-persist
Advanced tools
Package for recoil to persist and rehydrate store with custom serialize/deserialize function
Tiny module for recoil to store and sync state to
Storage
. It is only 354 bytes (minified and gzipped). No dependencies.
Size Limit controls the size.
If you are using recoil-persist with version 1.x.x please check migration guide to version 2.x.x.
import React from 'react'
import ReactDOM from 'react-dom'
import App from './App'
import { atom, RecoilRoot, useRecoilState } from 'recoil'
import { recoilPersist } from 'recoil-custom-persist'
const { persistAtom } = recoilPersist()()
const counterState = atom({
key: 'count',
default: 0,
effects_UNSTABLE: [persistAtom],
})
function App() {
const [count, setCount] = useRecoilState(counterState)
return (
<div>
<h3>Counter: {count}</h3>
<button onClick={() => setCount(count + 1)}>Increase</button>
<button onClick={() => setCount(count - 1)}>Decrease</button>
</div>
)
}
ReactDOM.render(
<React.StrictMode>
<RecoilRoot>
<App />
</RecoilRoot>
</React.StrictMode>,
document.getElementById('root'),
)
npm install recoil-custom-persist
or
yarn add recoil-custom-persist
Now you could add persisting a state to your app:
import React from 'react';
import ReactDOM from 'react-dom';
import App from './App';
import { RecoilRoot } from "recoil";
+import { recoilPersist } from 'recoil-persist'
+const { persistAtom } = recoilPersist()()
const counterState = atom({
key: 'count',
default: 0,
+ effects_UNSTABLE: [persistAtom],
})
function App() {
const [count, setCount] = useRecoilState(counterState)
return (
<div>
<h3>Counter: {count}</h3>
<button onClick={() => setCount(count + 1)}>Increase</button>
<button onClick={() => setCount(count - 1)}>Decrease</button>
</div>
)
}
ReactDOM.render(
<React.StrictMode>
<RecoilRoot>
<App />
</RecoilRoot>
</React.StrictMode>,
document.getElementById('root'),
)
After this each changes in atom will be store and sync to localStorage
.
import { recoilPersist } from 'recoil-persist'
const { persistAtom } = recoilPersist({
key: 'recoil-persist', // this key is using to store data in local storage
storage: localStorage, // configurate which stroage will be used to store the data
})({
serialize: (input) => JSON.stringify(input), // Custom serialize function
deserialize: (input) => JSON.parse(input) // Custom deserialize function
})
type config.key = String
Default value of config.key
is recoil-persist
. This key is using to store
data in storage.
type config.storage = Storage
Set config.storage
with sessionStorage
or other Storage
implementation to
change storage target. Otherwise localStorage
is used (default).
The API changed from version 1.x.x.
To update your code just use this migration guide:
import React from 'react';
import ReactDOM from 'react-dom';
import App from './App';
import { RecoilRoot } from "recoil";
import { recoilPersist } from 'recoil-persist' // import stay the same
const {
- RecoilPersist,
- updateState
+ persistAtom
} = recoilPersist(
- ['count'], // no need for specifying atoms keys
{
key: 'recoil-persist', // configuration stay the same too
storage: localStorage
}
)
const counterState = atom({
key: 'count',
default: 0,
- persistence_UNSTABLE: { // Please remove persistence_UNSTABLE from atom definition
- type: 'log',
- },
+ effects_UNSTABLE: [persistAtom], // Please add effects_UNSTABLE key to atom definition
})
function App() {
const [count, setCount] = useRecoilState(counterState)
return (
<div>
<h3>Counter: {count}</h3>
<button onClick={() => setCount(count + 1)}>Increase</button>
<button onClick={() => setCount(count - 1)}>Decrease</button>
</div>
)
}
ReactDOM.render(
<React.StrictMode>
- <RecoilRoot initializeState={({set}) => updateState({set})>
+ <RecoilRoot> // Please remove updateState function from initiallizeState
- <RecoilPersist /> // and also remove RecoilPersist component
<App />
</RecoilRoot>
</React.StrictMode>,
document.getElementById('root')
);
$ git clone git@github.com:thanhlmm/recoil-persist.git
$ cd recoil-persist
$ npm install
$ npm run start
Please open localhost:1234.
FAQs
Package for recoil to persist and rehydrate store with custom serialize/deserialize function
The npm package recoil-custom-persist receives a total of 2 weekly downloads. As such, recoil-custom-persist popularity was classified as not popular.
We found that recoil-custom-persist demonstrated a not healthy version release cadence and project activity because the last version was released a year ago. It has 1 open source maintainer collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
Create React App is officially deprecated due to React 19 issues and lack of maintenance—developers should switch to Vite or other modern alternatives.
Security News
Oracle seeks to dismiss fraud claims in the JavaScript trademark dispute, delaying the case and avoiding questions about its right to the name.
Security News
The Linux Foundation is warning open source developers that compliance with global sanctions is mandatory, highlighting legal risks and restrictions on contributions.