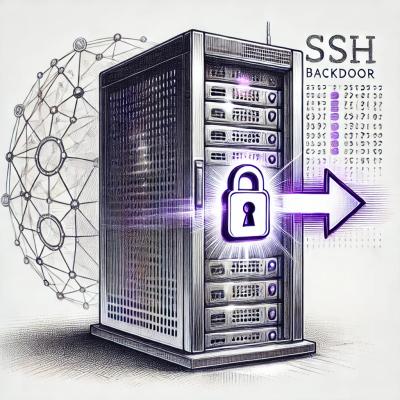
Research
Security News
Malicious npm Packages Inject SSH Backdoors via Typosquatted Libraries
Socket’s threat research team has detected six malicious npm packages typosquatting popular libraries to insert SSH backdoors.
red-black-tree-typed
Advanced tools
This is a standalone Red Black Tree data structure from the data-structure-typed collection. If you wish to access more data structures or advanced features, you can transition to directly installing the complete data-structure-typed package
npm i red-black-tree-typed --save
yarn add red-black-tree-typed
import {RedBlackTree} from 'data-structure-typed';
// /* or if you prefer */ import {RedBlackTree} from 'red-black-tree-typed';
const rbTree = new RedBlackTree<number>();
const idsOrVals = [11, 3, 15, 1, 8, 13, 16, 2, 6, 9, 12, 14, 4, 7, 10, 5];
rbTree.addMany(idsOrVals);
const node6 = rbTree.getNode(6);
node6 && rbTree.getHeight(node6) // 3
node6 && rbTree.getDepth(node6) // 1
const getNodeById = rbTree.getNodeByKey(10);
getNodeById?.id // 10
const getMinNodeByRoot = rbTree.getLeftMost();
getMinNodeByRoot?.id // 1
const node15 = rbTree.getNodeByKey(15);
const getMinNodeBySpecificNode = node15 && rbTree.getLeftMost(node15);
getMinNodeBySpecificNode?.id // 12
const lesserSum = rbTree.lesserSum(10);
lesserSum // 45
const node11 = rbTree.getNodeByKey(11);
node11?.id // 11
const dfs = rbTree.dfs('in');
dfs[0].id // 1
rbTree.perfectlyBalance();
const bfs = rbTree.bfs('node');
rbTree.isPerfectlyBalanced() && bfs[0].id // 8
rbTree.delete(11, true)[0].deleted?.id // 11
rbTree.isAVLBalanced(); // true
node15 && rbTree.getHeight(node15) // 2
rbTree.delete(1, true)[0].deleted?.id // 1
rbTree.isAVLBalanced(); // true
rbTree.getHeight() // 4
rbTree.delete(4, true)[0].deleted?.id // 4
rbTree.isAVLBalanced(); // true
rbTree.getHeight() // 4
rbTree.delete(10, true)[0].deleted?.id // 10
rbTree.isAVLBalanced(); // true
rbTree.getHeight() // 3
rbTree.delete(15, true)[0].deleted?.id // 15
rbTree.isAVLBalanced(); // true
rbTree.getHeight() // 3
rbTree.delete(5, true)[0].deleted?.id // 5
rbTree.isAVLBalanced(); // true
rbTree.getHeight() // 3
rbTree.delete(13, true)[0].deleted?.id // 13
rbTree.isAVLBalanced(); // true
rbTree.getHeight() // 3
rbTree.delete(3, true)[0].deleted?.id // 3
rbTree.isAVLBalanced(); // true
rbTree.getHeight() // 3
rbTree.delete(8, true)[0].deleted?.id // 8
rbTree.isAVLBalanced(); // true
rbTree.getHeight() // 3
rbTree.delete(6, true)[0].deleted?.id // 6
rbTree.delete(6, true).length // 0
rbTree.isAVLBalanced(); // true
rbTree.getHeight() // 2
rbTree.delete(7, true)[0].deleted?.id // 7
rbTree.isAVLBalanced(); // true
rbTree.getHeight() // 2
rbTree.delete(9, true)[0].deleted?.id // 9
rbTree.isAVLBalanced(); // true
rbTree.getHeight() // 2
rbTree.delete(14, true)[0].deleted?.id // 14
rbTree.isAVLBalanced(); // true
rbTree.getHeight() // 1
rbTree.isAVLBalanced(); // true
const lastBFSIds = rbTree.BFS();
lastBFSIds[0] // 12
const lastBFSNodes = rbTree.BFS('node');
lastBFSNodes[0].id // 12
const {RedBlackTree} = require('data-structure-typed');
// /* or if you prefer */ const {RedBlackTree} = require('red-black-tree-typed');
const rbTree = new RedBlackTree();
const idsOrVals = [11, 3, 15, 1, 8, 13, 16, 2, 6, 9, 12, 14, 4, 7, 10, 5];
rbTree.addMany(idsOrVals, idsOrVals);
const node6 = rbTree.getNodeByKey(6);
node6 && rbTree.getHeight(node6) // 3
node6 && rbTree.getDepth(node6) // 1
const getNodeById = rbTree.get(10, 'id');
getNodeById?.id // 10
const getMinNodeByRoot = rbTree.getLeftMost();
getMinNodeByRoot?.id // 1
const node15 = rbTree.getNodeByKey(15);
const getMinNodeBySpecificNode = node15 && rbTree.getLeftMost(node15);
getMinNodeBySpecificNode?.id // 12
const node11 = rbTree.getNodeByKey(11);
node11?.id // 11
const dfs = rbTree.dfs('in');
dfs[0].id // 1
rbTree.perfectlyBalance();
const bfs = rbTree.bfs('node');
rbTree.isPerfectlyBalanced() && bfs[0].id // 8
rbTree.delete(11, true)[0].deleted?.id // 11
rbTree.isAVLBalanced(); // true
node15 && rbTree.getHeight(node15) // 2
rbTree.delete(1, true)[0].deleted?.id // 1
rbTree.isAVLBalanced(); // true
rbTree.getHeight() // 4
rbTree.delete(4, true)[0].deleted?.id // 4
rbTree.isAVLBalanced(); // true
rbTree.getHeight() // 4
rbTree.delete(10, true)[0].deleted?.id // 10
rbTree.isAVLBalanced(); // true
rbTree.getHeight() // 3
rbTree.delete(15, true)[0].deleted?.id // 15
rbTree.isAVLBalanced(); // true
rbTree.getHeight() // 3
rbTree.delete(5, true)[0].deleted?.id // 5
rbTree.isAVLBalanced(); // true
rbTree.getHeight() // 3
rbTree.delete(13, true)[0].deleted?.id // 13
rbTree.isAVLBalanced(); // true
rbTree.getHeight() // 3
rbTree.delete(3, true)[0].deleted?.id // 3
rbTree.isAVLBalanced(); // true
rbTree.getHeight() // 3
rbTree.delete(8, true)[0].deleted?.id // 8
rbTree.isAVLBalanced(); // true
rbTree.getHeight() // 3
rbTree.delete(6, true)[0].deleted?.id // 6
rbTree.delete(6, true).length // 0
rbTree.isAVLBalanced(); // true
rbTree.getHeight() // 2
rbTree.delete(7, true)[0].deleted?.id // 7
rbTree.isAVLBalanced(); // true
rbTree.getHeight() // 2
rbTree.delete(9, true)[0].deleted?.id // 9
rbTree.isAVLBalanced(); // true
rbTree.getHeight() // 2
rbTree.delete(14, true)[0].deleted?.id // 14
rbTree.isAVLBalanced(); // true
rbTree.getHeight() // 1
rbTree.isAVLBalanced(); // true
const lastBFSIds = rbTree.bfs();
lastBFSIds[0] // 12
const lastBFSNodes = rbTree.bfs('node');
lastBFSNodes[0].id // 12
Data Structure | Unit Test | Performance Test | API Docs |
---|---|---|---|
Binary Tree | Binary Tree | ||
Binary Search Tree (BST) | BST | ||
AVL Tree | AVLTree | ||
Red Black Tree | RedBlackTree | ||
Tree Multiset | TreeMultimap | ||
Segment Tree | SegmentTree | ||
Binary Indexed Tree | BinaryIndexedTree | ||
Heap | Heap | ||
Priority Queue | PriorityQueue | ||
Max Priority Queue | MaxPriorityQueue | ||
Min Priority Queue | MinPriorityQueue | ||
Trie | Trie | ||
Graph | AbstractGraph | ||
Directed Graph | DirectedGraph | ||
Undirected Graph | UndirectedGraph | ||
Queue | Queue | ||
Deque | Deque | ||
Linked List | SinglyLinkedList | ||
Singly Linked List | SinglyLinkedList | ||
Doubly Linked List | DoublyLinkedList | ||
Stack | Stack |
Data Structure Typed | C++ STL | java.util | Python collections |
---|---|---|---|
Heap<E> | priority_queue<T> | PriorityQueue<E> | heapq |
Deque<E> | deque<T> | ArrayDeque<E> | deque |
Queue<E> | queue<T> | Queue<E> | - |
HashMap<K, V> | unordered_map<K, V> | HashMap<K, V> | defaultdict |
DoublyLinkedList<E> | list<T> | LinkedList<E> | - |
SinglyLinkedList<E> | - | - | - |
BinaryTree<K, V> | - | - | - |
BST<K, V> | - | - | - |
RedBlackTree<E> | set<T> | TreeSet<E> | - |
RedBlackTree<K, V> | map<K, V> | TreeMap<K, V> | - |
TreeMultimap<K, V> | multimap<K, V> | - | - |
- | multiset<T> | - | - |
Trie | - | - | - |
DirectedGraph<V, E> | - | - | - |
UndirectedGraph<V, E> | - | - | - |
PriorityQueue<E> | priority_queue<T> | PriorityQueue<E> | - |
Array<E> | vector<T> | ArrayList<E> | list |
Stack<E> | stack<T> | Stack<E> | - |
Set<E> | - | HashSet<E> | set |
Map<K, V> | - | HashMap<K, V> | dict |
- | unordered_set<T> | HashSet<E> | - |
Map<K, V> | - | - | OrderedDict |
- | unordered_multiset | - | Counter |
- | - | LinkedHashSet<E> | - |
HashMap<K, V> | - | LinkedHashMap<K, V> | - |
- | unordered_multimap<K, V> | - | - |
- | bitset<N> | - | - |
test name | time taken (ms) | executions per sec | sample deviation |
---|---|---|---|
10,000 add randomly | 31.32 | 31.93 | 3.67e-4 |
10,000 add & delete randomly | 70.90 | 14.10 | 0.00 |
10,000 addMany | 40.58 | 24.64 | 4.87e-4 |
10,000 get | 27.31 | 36.62 | 2.00e-4 |
test name | time taken (ms) | executions per sec | sample deviation |
---|---|---|---|
1,000 add randomly | 12.35 | 80.99 | 7.17e-5 |
1,000 add & delete randomly | 15.98 | 62.58 | 7.98e-4 |
1,000 addMany | 10.96 | 91.27 | 0.00 |
1,000 get | 18.61 | 53.73 | 0.00 |
1,000 dfs | 164.20 | 6.09 | 0.04 |
1,000 bfs | 58.84 | 17.00 | 0.01 |
1,000 morris | 256.66 | 3.90 | 7.70e-4 |
test name | time taken (ms) | executions per sec | sample deviation |
---|---|---|---|
10,000 add randomly | 31.59 | 31.66 | 2.74e-4 |
10,000 add & delete randomly | 74.56 | 13.41 | 8.32e-4 |
10,000 addMany | 29.16 | 34.30 | 0.00 |
10,000 get | 29.24 | 34.21 | 0.00 |
test name | time taken (ms) | executions per sec | sample deviation |
---|---|---|---|
100,000 add | 85.85 | 11.65 | 0.00 |
100,000 add & delete randomly | 211.54 | 4.73 | 0.00 |
100,000 getNode | 37.92 | 26.37 | 1.65e-4 |
test name | time taken (ms) | executions per sec | sample deviation |
---|---|---|---|
SRC PQ 10,000 add | 0.57 | 1748.73 | 4.96e-6 |
CJS PQ 10,000 add | 0.57 | 1746.69 | 4.91e-6 |
MJS PQ 10,000 add | 0.57 | 1749.68 | 4.43e-6 |
SRC PQ 10,000 add & pop | 3.47 | 288.14 | 6.38e-4 |
CJS PQ 10,000 add & pop | 3.39 | 295.36 | 3.90e-5 |
MJS PQ 10,000 add & pop | 3.37 | 297.17 | 3.03e-5 |
test name | time taken (ms) | executions per sec | sample deviation |
---|---|---|---|
1,000 addVertex | 0.10 | 9534.93 | 8.72e-7 |
1,000 addEdge | 6.30 | 158.67 | 0.00 |
1,000 getVertex | 0.05 | 2.16e+4 | 3.03e-7 |
1,000 getEdge | 22.31 | 44.82 | 0.00 |
tarjan | 210.90 | 4.74 | 0.01 |
tarjan all | 214.72 | 4.66 | 0.01 |
topologicalSort | 172.52 | 5.80 | 0.00 |
test name | time taken (ms) | executions per sec | sample deviation |
---|---|---|---|
1,000,000 set | 275.88 | 3.62 | 0.12 |
1,000,000 Map set | 211.66 | 4.72 | 0.01 |
1,000,000 Set add | 177.72 | 5.63 | 0.02 |
1,000,000 set & get | 317.60 | 3.15 | 0.02 |
1,000,000 Map set & get | 274.99 | 3.64 | 0.03 |
1,000,000 Set add & has | 172.23 | 5.81 | 0.02 |
1,000,000 ObjKey set & get | 929.40 | 1.08 | 0.07 |
1,000,000 Map ObjKey set & get | 310.02 | 3.23 | 0.05 |
1,000,000 Set ObjKey add & has | 283.28 | 3.53 | 0.04 |
test name | time taken (ms) | executions per sec | sample deviation |
---|---|---|---|
10,000 add & pop | 5.80 | 172.35 | 8.78e-5 |
10,000 fib add & pop | 357.92 | 2.79 | 0.00 |
test name | time taken (ms) | executions per sec | sample deviation |
---|---|---|---|
1,000,000 push | 221.57 | 4.51 | 0.03 |
1,000,000 unshift | 229.02 | 4.37 | 0.07 |
1,000,000 unshift & shift | 169.21 | 5.91 | 0.02 |
1,000,000 insertBefore | 314.48 | 3.18 | 0.07 |
test name | time taken (ms) | executions per sec | sample deviation |
---|---|---|---|
10,000 push & pop | 212.98 | 4.70 | 0.01 |
10,000 insertBefore | 250.68 | 3.99 | 0.01 |
test name | time taken (ms) | executions per sec | sample deviation |
---|---|---|---|
10,000 refill & poll | 8.91 | 112.29 | 2.26e-4 |
test name | time taken (ms) | executions per sec | sample deviation |
---|---|---|---|
100,000 add & pop | 103.59 | 9.65 | 0.00 |
test name | time taken (ms) | executions per sec | sample deviation |
---|---|---|---|
1,000,000 push | 14.55 | 68.72 | 6.91e-4 |
1,000,000 push & pop | 23.40 | 42.73 | 5.94e-4 |
1,000,000 push & shift | 24.41 | 40.97 | 1.45e-4 |
1,000,000 unshift & shift | 22.56 | 44.32 | 1.30e-4 |
test name | time taken (ms) | executions per sec | sample deviation |
---|---|---|---|
1,000,000 push | 39.90 | 25.07 | 0.01 |
1,000,000 push & shift | 81.79 | 12.23 | 0.00 |
test name | time taken (ms) | executions per sec | sample deviation |
---|---|---|---|
1,000,000 push | 37.60 | 26.60 | 0.00 |
1,000,000 push & pop | 47.01 | 21.27 | 0.00 |
test name | time taken (ms) | executions per sec | sample deviation |
---|---|---|---|
100,000 push | 45.97 | 21.76 | 0.00 |
100,000 getWords | 66.20 | 15.11 | 0.00 |
Algorithm | Function Description | Iteration Type |
---|---|---|
Binary Tree DFS | Traverse a binary tree in a depth-first manner, starting from the root node, first visiting the left subtree, and then the right subtree, using recursion. | Recursion + Iteration |
Binary Tree BFS | Traverse a binary tree in a breadth-first manner, starting from the root node, visiting nodes level by level from left to right. | Iteration |
Graph DFS | Traverse a graph in a depth-first manner, starting from a given node, exploring along one path as deeply as possible, and backtracking to explore other paths. Used for finding connected components, paths, etc. | Recursion + Iteration |
Binary Tree Morris | Morris traversal is an in-order traversal algorithm for binary trees with O(1) space complexity. It allows tree traversal without additional stack or recursion. | Iteration |
Graph BFS | Traverse a graph in a breadth-first manner, starting from a given node, first visiting nodes directly connected to the starting node, and then expanding level by level. Used for finding shortest paths, etc. | Recursion + Iteration |
Graph Tarjan's Algorithm | Find strongly connected components in a graph, typically implemented using depth-first search. | Recursion |
Graph Bellman-Ford Algorithm | Finding the shortest paths from a single source, can handle negative weight edges | Iteration |
Graph Dijkstra's Algorithm | Finding the shortest paths from a single source, cannot handle negative weight edges | Iteration |
Graph Floyd-Warshall Algorithm | Finding the shortest paths between all pairs of nodes | Iteration |
Graph getCycles | Find all cycles in a graph or detect the presence of cycles. | Recursion |
Graph getCutVertexes | Find cut vertices in a graph, which are nodes that, when removed, increase the number of connected components in the graph. | Recursion |
Graph getSCCs | Find strongly connected components in a graph, which are subgraphs where any two nodes can reach each other. | Recursion |
Graph getBridges | Find bridges in a graph, which are edges that, when removed, increase the number of connected components in the graph. | Recursion |
Graph topologicalSort | Perform topological sorting on a directed acyclic graph (DAG) to find a linear order of nodes such that all directed edges go from earlier nodes to later nodes. | Recursion |
Principle | Description |
---|---|
Practicality | Follows ES6 and ESNext standards, offering unified and considerate optional parameters, and simplifies method names. |
Extensibility | Adheres to OOP (Object-Oriented Programming) principles, allowing inheritance for all data structures. |
Modularization | Includes data structure modularization and independent NPM packages. |
Efficiency | All methods provide time and space complexity, comparable to native JS performance. |
Maintainability | Follows open-source community development standards, complete documentation, continuous integration, and adheres to TDD (Test-Driven Development) patterns. |
Testability | Automated and customized unit testing, performance testing, and integration testing. |
Portability | Plans for porting to Java, Python, and C++, currently achieved to 80%. |
Reusability | Fully decoupled, minimized side effects, and adheres to OOP. |
Security | Carefully designed security for member variables and methods. Read-write separation. Data structure software does not need to consider other security aspects. |
Scalability | Data structure software does not involve load issues. |
FAQs
RedBlackTree. Javascript & Typescript Data Structure.
The npm package red-black-tree-typed receives a total of 287 weekly downloads. As such, red-black-tree-typed popularity was classified as not popular.
We found that red-black-tree-typed demonstrated a healthy version release cadence and project activity because the last version was released less than a year ago. It has 0 open source maintainers collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Research
Security News
Socket’s threat research team has detected six malicious npm packages typosquatting popular libraries to insert SSH backdoors.
Security News
MITRE's 2024 CWE Top 25 highlights critical software vulnerabilities like XSS, SQL Injection, and CSRF, reflecting shifts due to a refined ranking methodology.
Security News
In this segment of the Risky Business podcast, Feross Aboukhadijeh and Patrick Gray discuss the challenges of tracking malware discovered in open source softare.