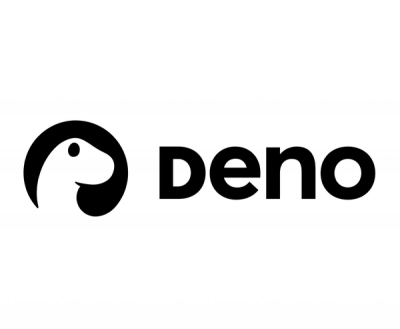
Security News
Deno 2.2 Improves Dependency Management and Expands Node.js Compatibility
Deno 2.2 enhances Node.js compatibility, improves dependency management, adds OpenTelemetry support, and expands linting and task automation for developers.
Takes JavaScript code, along with a config and returns the original code with tokens wrapped as configured.
The redeyed npm package provides functionality for syntax highlighting and code transformation by allowing modifications to JavaScript code through configuration of hooks for tokens and types. It enables parsing JavaScript code, identifying tokens and their types, and then applying transformations or highlighting based on the configuration provided.
Syntax Highlighting
This feature allows for syntax highlighting of JavaScript code. By configuring the `String` token, all string literals in the code can be highlighted. The example demonstrates how to highlight string literals in green using ANSI escape codes.
const redeyed = require('redeyed');
const config = {
String: {
_default: { open: '\x1b[32m', close: '\x1b[39m', _default: true }
}
};
const code = '"Hello, world!"';
const highlighted = redeyed(code, config).code;
console.log(highlighted);
Code Transformation
This feature enables code transformation by applying custom transformations to specific JavaScript tokens. In the example, all instances of the `function` keyword are prefixed with `_function_`, demonstrating a simple transformation.
const redeyed = require('redeyed');
const config = {
Keyword: {
'function': { open: '_function_', close: '' }
}
};
const code = 'function example() {}';
const transformed = redeyed(code, config).code;
console.log(transformed);
Esprima is a popular JavaScript parser that provides a detailed syntax tree of JavaScript code. While it doesn't directly offer syntax highlighting or transformation, it serves as a foundation for building such tools. Compared to redeyed, Esprima offers more in-depth analysis but requires more setup for syntax highlighting or code transformation.
jscodeshift is a toolkit for running codemods over multiple JavaScript or TypeScript files. It uses a different approach than redeyed by focusing on code transformations rather than syntax highlighting. jscodeshift offers a more comprehensive API for complex code modifications, making it more suitable for large-scale refactoring.
highlight.js is a syntax highlighter written in JavaScript. It supports a wide range of programming languages, including JavaScript. Unlike redeyed, which allows for dynamic modification of code through configuration, highlight.js focuses solely on syntax highlighting without the ability to transform code.
Add color to your JavaScript!
Red Eyed Tree Frog (Agalychnis callidryas)
Takes JavaScript code, along with a config and returns the original code with tokens wrapped and/or replaced as configured.
One usecase is adding metadata to your code that can then be used to apply syntax highlighting.
undefined
of each token you want to configure with one of the following'before:after'
: wraps the token inside before/after
{ _before: 'before', _after: 'after' }
: wraps token inside before/after
function (s) { return 'replacement for s'; }
: replaces the token with whatever is returned by the provided function
For the {String}
and {Object}
configurations, 'before' or 'after' may be omitted:
{String}
:
'before:'
(omitting 'after')':after'
(omitting 'before'){Object}
:
{ _before: 'before' }
(omitting '_after'){ _after: 'after' }
(omitting '_before')In these cases the missing half is resolved as follows:
parent._default
(i.e., Keyword._default
) if foundconfig._default
if found''
(empty string)Invoke redeyed with your configuration and a code snippet as in the below example:
var redeyed = require('redeyed')
, config = require('./path/to/config')
, code = 'var a = 3;'
, result;
// redeyed will throw an error (caused by the esprima parser) if the code has invalid javascript
try {
result = redeyed(code, config);
console.log(result);
} catch(err) {
console.error(err);
}
FAQs
Takes JavaScript code, along with a config and returns the original code with tokens wrapped as configured.
The npm package redeyed receives a total of 2,720,027 weekly downloads. As such, redeyed popularity was classified as popular.
We found that redeyed demonstrated a not healthy version release cadence and project activity because the last version was released a year ago. It has 1 open source maintainer collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
Deno 2.2 enhances Node.js compatibility, improves dependency management, adds OpenTelemetry support, and expands linting and task automation for developers.
Security News
React's CRA deprecation announcement sparked community criticism over framework recommendations, leading to quick updates acknowledging build tools like Vite as valid alternatives.
Security News
Ransomware payment rates hit an all-time low in 2024 as law enforcement crackdowns, stronger defenses, and shifting policies make attacks riskier and less profitable.