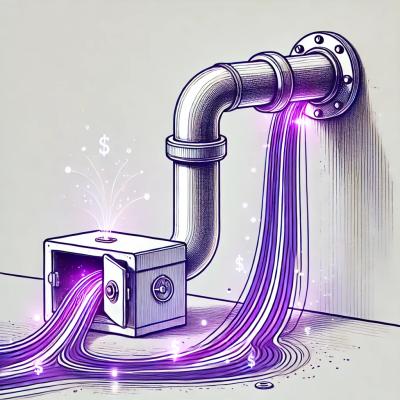
Research
Security News
Malicious npm Package Targets Solana Developers and Hijacks Funds
A malicious npm package targets Solana developers, rerouting funds in 2% of transactions to a hardcoded address.
redis-expiry
Advanced tools
Use redis to expire your keys and handling the value
$ npm install redis-expiry
const Redis = require("redis");
const redisExpiry = require("redis-expiry");
const redisSetter = Redis.createClient(process.env.REDIS_URL);
const redisGetter = Redis.createClient(process.env.REDIS_URL);
const rexp = redisExpiry(redisSetter, redisGetter);
rexp.set("myKeyByTimeout", "myValue").timeout(60000); // key will be expire in 60sec
const expireDate = new Date();
expireDate.setSeconds(expireDate.getSeconds() + 60);
rexp.set("myKeyByDate", "myValue").at(expireDate); // key will be expire in 60sec
rexp.set("myKeyByCron", "myValue").cron("*/30 * * * * *"); // key will be expire every 30sec
rexp.on(/myKeyBy(.)/, (value, key) => { // event will always be scheduled if the application restart
console.log("Value returned", value, "From key", key);
});
Create a new instance :
const Redis = require("redis");
const redisExpiry = require("redis-expiry");
const redisSetter = Redis.createClient(process.env.REDIS_URL);
const redisGetter = Redis.createClient(process.env.REDIS_URL);
const rexp = redisExpiry(redisSetter, redisGetter);
The code bellow is deprecated since v1.0.4
const rexp = redisExpiry(redisSetter, process.env.REDIS_URL);
⚠ If your application is shutdown and one of your keys expire, redis-expiry
will detect them ⚠
⚠ Then when your application will be operationnal, the event rexp.on("myKey", callback)
will be called⚠
Before choosing the type of expiration, you have to set the key/value:
rexp.set("myKey", "myValue")...
rexp.set(...).infinit();
A simple redis set
:
await rexp.set("myKeyByInfinit", "myValue").infinit();
myKeyByInfinit
will never expired.
rexp.set(...).at(date);
Schedule from a date:
const currentDate = new Date();
currentDate.setSeconds(currentDate.getSeconds() + 30);
await rexp.set("myKeyByAt", "myValue").at(currentDate);
myKeyByAt
will expire in 30 seconds.
rexp.set(...).timeout(integer);
Schedule from a timeout:
await rexp.set("myKeyByTimeout", "myValue").timeout(60000);
myKeyByTimeout
will expire in 60 seconds.
rexp.set(...).now();
Schedule from now:
await rexp.set("myKeyByNow", "myValue").now();
myKeyByNow
will expire in few milliseconds.
rexp.set(...).cron();
Schedule from cron:
await rexp.set("myKeyByCron", "myValue").cron("*/4 * * * * *", cronOption);
myKeyByCron
will expire in the next multiple of 4 seconds.
⚠ after expiration the event is rescheduled. To stop the cron, check "Adding event handler" part bellow⚠
cronOption
is optional, check the link bellow to know more.
More information: https://www.npmjs.com/package/cron-parser
The handler will be call every time a specified key expires:
rexp.on("myKeyByTimeout", (value, key) => {
// value === "myValue"
}, {
maxConcurrent: 1 // Synchro event
});
To stop the cron task execute stop
parameter:
rexp.on("myKeyByTimeout", (value, key, stop) => {
stop(); // stop cron task
});
Using regexp :
rexp.on(/myKeyBy(.)/, (value, key, stop) => {
// value === "myValue"
});
Every myKeyBy*
key will be returned
If no value is specified then every keys will be removed:
await rexp.del("myKeyByTimeout");
Remove specific contents by value:
await rexp.del("myKeyByTimeout", "myValue");
By regexp:
await rexp.del(/(.)Timeout/);
By regexp with a value:
await rexp.del(/(.)Timeout/, "myValue");
By guuid:
await rexp.delByGuuid("Dzokijo");
If no value is specified then every keys will be returned:
const result = await rexp.get("myKeyByTimeout");
Return specific contents by value:
const result = await rexp.get("myKeyByTimeout", "myValue");
By regexp:
await rexp.get(/(.)Timeout/);
By regexp with a value:
await rexp.get(/(.)Timeout/, "myValue");
By guuid:
await rexp.getByGuuid("Dzokijo");
If no value is specified then every keys will be updated:
const result = await rexp.update("myKeyByTimeout")("myNewValue");
Update specific contents by value:
const result = await rexp.update("myKeyByTimeout", "myValue")("myNewValue");
By regexp:
await rexp.update(/(.)Timeout/)("myNewValue");
By regexp with a value:
await rexp.update(/(.)Timeout/, "myValue")("myNewValue");
By guuid:
await rexp.updateByGuuid("Dzokijo")("myNewValue");
Reschedule date:
const currentDate = new Date();
currentDate.setSeconds(currentDate.getSeconds() + 30);
await rexp.reschedule("myKeyByAt", "myValue").at(currentDate);
Reschedule timeout:
await rexp.reschedule("myKeyByTimeout", "myValue").timeout(60000);
Reschedule now:
await rexp.reschedule("myKeyByNow", "myValue").now();
Reschedule cron:
await rexp.reschedule("myKeyByCron", "myValue").cron("*/4 * * * * *");
Reschedule all contents :
await rexp.reschedule("myKeyByTimeout").timeout(30000);
By regexp:
await rexp.reschedule(/(.)Timeout/).timeout(30000);
By regexp with a value:
await rexp.reschedule(/(.)Timeout/, "myValue").timeout(30000);
Every contents is rescheduled with a timeout at 30 secs
By guuid:
await rexp.rescheduleByGuuid("Dzokijo").timeout(30000);
Chainable API
Update value with andUpdateValue
function :
await rexp.rescheduleByGuuid("Dzokijo").andUpdateValue("myNewValue").timeout(30000);
Clone the repo and run from the project root:
$ npm install
$ npm test
FAQs
Use redis to expire your keys and handling the value
We found that redis-expiry demonstrated a not healthy version release cadence and project activity because the last version was released a year ago. It has 1 open source maintainer collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Research
Security News
A malicious npm package targets Solana developers, rerouting funds in 2% of transactions to a hardcoded address.
Security News
Research
Socket researchers have discovered malicious npm packages targeting crypto developers, stealing credentials and wallet data using spyware delivered through typosquats of popular cryptographic libraries.
Security News
Socket's package search now displays weekly downloads for npm packages, helping developers quickly assess popularity and make more informed decisions.