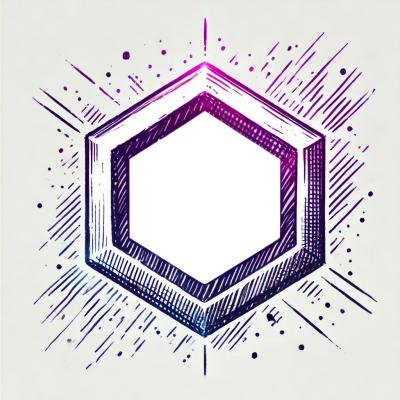
Security News
Maven Central Adds Sigstore Signature Validation
Maven Central now validates Sigstore signatures, making it easier for developers to verify the provenance of Java packages.
reduce-reducers
Advanced tools
The reduce-reducers npm package allows you to combine multiple reducer functions into a single reducer function. This is particularly useful in state management scenarios, such as with Redux, where you may want to split the logic for handling different parts of the state into separate reducers and then combine them.
Combining Multiple Reducers
This feature allows you to combine multiple reducers into a single reducer function. The code sample demonstrates how to combine a countReducer and a textReducer into a rootReducer using reduce-reducers.
const { combineReducers } = require('redux');
const reduceReducers = require('reduce-reducers');
const initialState = { count: 0, text: '' };
const countReducer = (state = initialState.count, action) => {
switch (action.type) {
case 'INCREMENT':
return state + 1;
case 'DECREMENT':
return state - 1;
default:
return state;
}
};
const textReducer = (state = initialState.text, action) => {
switch (action.type) {
case 'SET_TEXT':
return action.text;
default:
return state;
}
};
const rootReducer = reduceReducers(
(state = initialState, action) => state,
combineReducers({ count: countReducer, text: textReducer })
);
console.log(rootReducer(undefined, { type: 'INCREMENT' })); // { count: 1, text: '' }
console.log(rootReducer(undefined, { type: 'SET_TEXT', text: 'Hello' })); // { count: 0, text: 'Hello' }
Redux is a popular state management library for JavaScript applications. It provides a combineReducers function that allows you to combine multiple reducers into a single reducer. While reduce-reducers focuses on combining reducers in a more flexible way, Redux's combineReducers is more straightforward and is a core part of the Redux library.
Redux Loop is an alternative to redux-thunk and redux-saga. It allows you to handle side effects in your reducers. While it doesn't directly compete with reduce-reducers, it offers a different approach to managing complex state logic and side effects in Redux applications.
Reducer Composer is another library that allows you to combine multiple reducers into one. It provides a similar functionality to reduce-reducers but with a different API. It focuses on composing reducers in a more functional programming style.
Reduce multiple reducers into a single reducer from left to right
npm install reduce-reducers
import reduceReducers from 'reduce-reducers';
const initialState = { A: 0, B: 0 };
const addReducer = (state, payload) => ({ ...state, A: state.A + payload });
const multReducer = (state, payload) => ({ ...state, B: state.B * payload });
const reducer = reduceReducers(initialState, addReducer, multReducer);
const state = { A: 1, B: 2 };
const payload = 3;
reducer(state, payload); // { A: 4, B: 6 }
Originally created to combine multiple Redux reducers that correspond to different actions (e.g. like this). Technically works with any reducer, not just with Redux, though I don't know of any other use cases.
reduceReducers
and combineReducers
?This StackOverflow post explains it very well: https://stackoverflow.com/a/44371190/5741172
FAQs
Reduce multiple reducers into a single reducer
We found that reduce-reducers demonstrated a not healthy version release cadence and project activity because the last version was released a year ago. It has 2 open source maintainers collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
Maven Central now validates Sigstore signatures, making it easier for developers to verify the provenance of Java packages.
Security News
CISOs are racing to adopt AI for cybersecurity, but hurdles in budgets and governance may leave some falling behind in the fight against cyber threats.
Research
Security News
Socket researchers uncovered a backdoored typosquat of BoltDB in the Go ecosystem, exploiting Go Module Proxy caching to persist undetected for years.