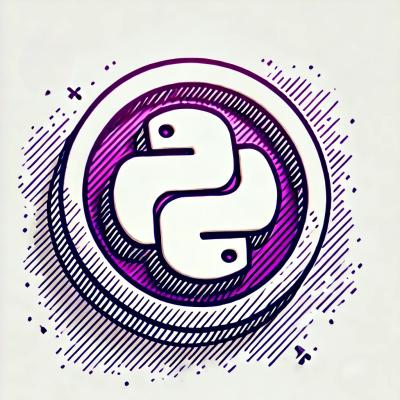
Security News
PyPI Now Supports iOS and Android Wheels for Mobile Python Development
PyPI now supports iOS and Android wheels, making it easier for Python developers to distribute mobile packages.
redux-act-array-async
Advanced tools
Reducing the boilerplate of redux asynchronous applications
Create async multi actions and reducers based on redux-act
npm install redux-act-array-async --save
import thunk from 'redux-thunk'
import {createStore, applyMiddleware} from 'redux';
import {createActionAsync, createReducerAsync} from 'redux-act-array-async';
// The async api to call, must be a function that returns a promise
let user = {id: 8};
function apiOk(){
return Promise.resolve(user);
}
// createActionAsync will create 4 synchronous action creators:
// login.request, login.ok, login.error and login.reset
const login = createActionAsync('LOGIN', apiOk);
/*
createReducerAsync takes an async action created by createActionAsync.
It reduces the following state given the four actions: request, ok, error and reset.
const defaultsState = {
loading: false,
request: null,
data: null,
error: null
};
if you need to overwrite the defaultsState just insert your initialState as a second paramenter in the createReducerAsync function. Just like that:
const initialState = {
loading: false,
request: null,
data: {custom: "intitial data"},
error: null
};
const reducer = createReducerAsync(login, initialState)
*/
const reducer = createReducerAsync(login)
const store = createStore(reducer, applyMiddleware(thunk));
await store.dispatch(login({username:'lolo', password: 'password'}));
In a nutshell, the following code:
const options = {noRethrow: false};
const loginAction = createActionAsync('LOGIN', api, options);
const loginReducer = createReducerAsync(loginAction)
is equivalent to:
const LOGIN_REQUEST = 'LOGIN_REQUEST'
const LOGIN_OK = 'LOGIN_OK'
const LOGIN_ERROR = 'LOGIN_ERROR'
const LOGIN_RESET = 'LOGIN_RESET'
const loginRequest = (value) => ({
type: LOGIN_REQUEST,
payload: value
})
const loginOk = (value) => ({
type: LOGIN_OK,
payload: value
})
const loginError = (value) => ({
type: LOGIN_ERROR,
payload: value
})
const loginReset = (value) => ({
type: LOGIN_RESET,
payload: value
})
const options = {noRethrow: true};
export const login = (...args) => {
return (dispatch, getState) => {
dispatch(loginRequest(...args));
return api(...args, dispatch, getState)
.then(response => {
const out = {
request: args,
response: response
}
dispatch(loginOk(out))
return out;
})
.catch(error => {
const errorOut = {
actionAsync,
request: args,
error: error
}
dispatch(loginError(errorOut))
if(!options.noRethrow) throw errorOut;
})
}
}
const defaultsState = {
loading: false,
request: null,
data: null,
error: null
};
const reducer = createReducer({
[actionAsync.request]: (state, payload) => ({
...state,
request: payload,
loading: true,
data: null,
error: null
}),
[actionAsync.ok]: (state, payload) => ({
...state,
loading: false,
data: payload.response
}),
[actionAsync.error]: (state, payload) => ({
...state,
loading: false,
error: payload.error
}),
[actionAsync.reset]: () => (defaultsState)
} , defaultsState);
That's 3 lines against 78 lines, a good way to reduce boilerplate code.
Here are all the options to configure an asynchronous action:
const actionOptions = {
noRethrow: false,
request:{
callback: (dispatch, getState, ...args) => {
},
payloadReducer: (payload) => {
return payload
},
metaReducer: (meta) => {
return ASYNC_META.REQUEST
}
},
ok:{
callback: (dispatch, getState, ...args) => {
},
payloadReducer: (payload) => {
return payload
},
metaReducer: () => {
return ASYNC_META.OK
}
},
error:{
callback: (dispatch, getState, ...args) => {
},
payloadReducer: (payload) => {
return payload
},
metaReducer: () => {
return ASYNC_META.ERROR
}
}
}
const loginAction = createActionAsync('LOGIN', api, actionOptions);
This library has been extracted originally from starhack.it, a React/Node Full Stack Starter Kit.
FAQs
Reducing the boilerplate of redux asynchronous applications
The npm package redux-act-array-async receives a total of 1 weekly downloads. As such, redux-act-array-async popularity was classified as not popular.
We found that redux-act-array-async demonstrated a not healthy version release cadence and project activity because the last version was released a year ago. It has 1 open source maintainer collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
PyPI now supports iOS and Android wheels, making it easier for Python developers to distribute mobile packages.
Security News
Create React App is officially deprecated due to React 19 issues and lack of maintenance—developers should switch to Vite or other modern alternatives.
Security News
Oracle seeks to dismiss fraud claims in the JavaScript trademark dispute, delaying the case and avoiding questions about its right to the name.