What is redux-observable?
redux-observable is a middleware for Redux that allows you to handle asynchronous actions using RxJS observables. It enables complex async flows in your Redux applications by leveraging the power of reactive programming.
What are redux-observable's main functionalities?
Handling Asynchronous Actions
This feature allows you to handle asynchronous actions such as API calls. The example demonstrates an epic that listens for 'FETCH_USER' actions, makes an AJAX request to fetch user data, and dispatches either a 'FETCH_USER_FULFILLED' or 'FETCH_USER_FAILED' action based on the result.
const fetchUserEpic = action$ => action$.pipe(
ofType('FETCH_USER'),
mergeMap(action =>
ajax.getJSON(`/api/users/${action.payload}`).pipe(
map(response => ({ type: 'FETCH_USER_FULFILLED', payload: response })),
catchError(error => of({ type: 'FETCH_USER_FAILED', payload: error }))
)
)
);
Combining Multiple Epics
redux-observable allows you to combine multiple epics into a single root epic. This is useful for organizing your code and managing complex async flows. The example shows how to combine `fetchUserEpic` with another epic.
const rootEpic = combineEpics(
fetchUserEpic,
anotherEpic
);
Cancellation of Actions
redux-observable supports the cancellation of ongoing actions. The example demonstrates using `switchMap` to cancel any ongoing AJAX request if a new 'FETCH_USER' action is dispatched, ensuring only the latest request is processed.
const fetchUserEpic = action$ => action$.pipe(
ofType('FETCH_USER'),
switchMap(action =>
ajax.getJSON(`/api/users/${action.payload}`).pipe(
map(response => ({ type: 'FETCH_USER_FULFILLED', payload: response })),
catchError(error => of({ type: 'FETCH_USER_FAILED', payload: error }))
)
)
);
Other packages similar to redux-observable
redux-saga
redux-saga is a middleware for Redux that uses generator functions to handle side effects. It is similar to redux-observable in that it helps manage complex async flows, but it uses a different approach based on ES6 generators rather than RxJS observables.
redux-thunk
redux-thunk is a middleware that allows you to write action creators that return a function instead of an action. It is simpler than redux-observable and redux-saga, making it a good choice for handling basic async actions without the need for complex flow control.
redux-promise-middleware
redux-promise-middleware is a middleware that allows you to dispatch promises as actions. It automatically dispatches pending, fulfilled, and rejected actions based on the promise's state. It is less powerful than redux-observable but can be useful for straightforward async operations.

RxJS 6-based middleware for
Redux. Compose and cancel async actions to create side effects and more.
https://redux-observable.js.org
https://redux-observable-cn.js.org 中文版(非官方)
Supporting redux-observable
redux-observable is an independent project with ongoing development and support made possible thanks to donations made by these awesome backers and sponsorship by This Dot. If you'd like to join them, please consider:
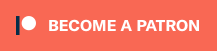

Get support, training, and project consulting by the people who created Redux Observable and RxJS!
No Platinum or Gold sponsors yet. Become one and your name or logo will be here! Help keep Open Source sustainable.
Install
This has peer dependencies of rxjs@6.x.x
and redux@4.x.x
, which will have to be installed as well.
npm install --save redux-observable
UMD
We publish a UMD build inside our npm package. You can use it via the unpkg CDN:
https://unpkg.com/redux-observable@latest/dist/redux-observable.min.js
Watch an introduction
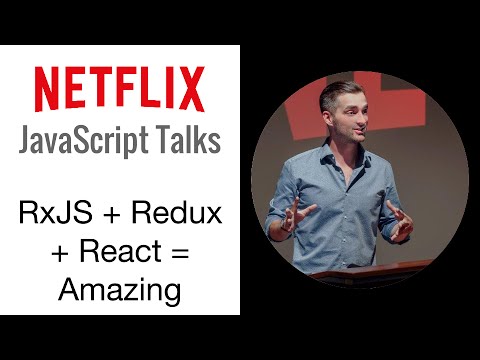
Online Live Examples
To see redux-observable in action, here's a very simple example to play around with:
Documentation
Discuss

Everyone is welcome on our Gitter channel!
Custom Emoji
Save this:
Add the redux-observable spinning logo to your Slack channel! Slack Instructions
*redux-observable is a community-driven, entirely volunteer project and is not officially affiliated with or sponsored by any company.
:shipit: