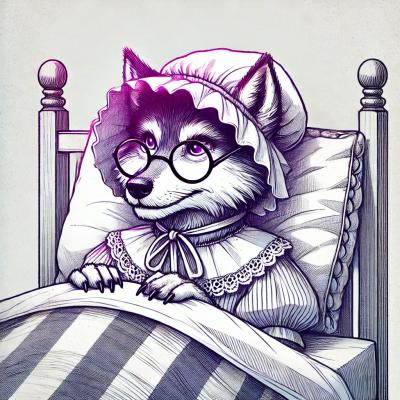
Security News
GitHub Removes Malicious Pull Requests Targeting Open Source Repositories
GitHub removed 27 malicious pull requests attempting to inject harmful code across multiple open source repositories, in another round of low-effort attacks.
redux-subspace
Advanced tools
This is a library to create subspaces for Redux connected React components. It's designed to work with Provider from the react-redux bindings.
The MelbJS presentation that launched this library - Scaling React and Redux at IOOF.
For a Redux connected React component, SubspaceProvider allows you to present a sub-view of the state to the component, allowing it to be ignorant of parent state structure. This means you can reuse these components in multiple parts of your app, or even multiple applications that have different store structures.
Actions dispatched from components can be automatically namespaced to prevent them from being picked up by unrelated reducers that inadvertently use the same action types. Actions dispatched inside thunks executed by Redux-thunk middleware will be automatically namespaced.
Subspace works with global actions by specifying global: true
in the action. Global actions will not be namespaced, allowing them to be picked up by other components' reducers.
npm i --save redux-subspace
Combine component's reducer
import { combineReducers } from 'redux'
import { reducer as subComponent } from 'some-dependency'
...
const reducer = combineReducers({ subComponent })
Wrap sub-component with provider
import { SubspaceProvider } from 'redux-subspace'
import { SubComponent } from 'some-dependency'
...
<SubspaceProvider mapState={state => state.subComponent}>
<SubComponent />
</SubspaceProvider>
Export reducer
const initialState = {
value: "store value"
}
export default function reducer(state = initialState, action) {
...
return state
}
Use in mapStateToProps
const mapStateToProps = state => {
return {
value: state.value,
parentValue: state.root.value
}
}
An additional root
node is added to the state which will reflect the root state.
The subspaced
HOC can be used to wrap components you do not want to directly wrap in jsx
. An example of when this might be useful is when setting Route
components for react-router
.
import { subspaced } from 'redux-subspace'
import { SubComponent } from 'some-dependency'
const SubspacedSubComponent = subspaced(state => state.subComponent)(SubComponent)
Namespacing sub-components allows multiple instances of the component to exist on the same page, without the actions affecting each other's state.
To namespace the sub-component both the provider and the reducer need to be namespaced by the parent component/app. The type
of any dispatched namespaced actions will be in the format givenNamespace/originalType
.
import { SubspaceProvider } from 'redux-subspace'
import { SubComponent } from 'some-dependency'
...
<SubspaceProvider mapState={state => state.subComponent}, namespace='myComponent'>
<SubComponent />
</SubspaceProvider>
import { combineReducers } from 'redux'
import { namespaced } from 'redux-subspace'
import { reducer as subComponent } from 'some-dependency'
...
const reducer = combineReducers({ subComponent: namespaced(subComponent, 'myComponent') })
Occasionally you may have actions that need to go beyond your small view of the world.
If you have control over the action creator, passing your action to the asGlobal
function before it is dispatched will ensure your action does not get namespaced.
import { asGlobal } from 'redux-subspace'
const globalActionCreator = globalValue => {
return asGlobal({ type = "GLOBAL_ACTION", globalValue})
}
This method gives you more control over if and when an action should be treated as global.
To exclude all instances of an action type from namespacing, the GlobalActions.register
function can be used.
import { GlobalActions } from 'redux-subspace'
GlobalActions.register("GLOBAL_ACTION")
const globalActionCreator = globalValue => {
return { type = "GLOBAL_ACTION", globalValue}
}
This is particularly useful when using actionsCreators of dependencies that are unaware that they are being dispatched within a namespaced subspace, such as the navigation actions from react-router-redux (see our example for more details).
The subspaced
HOC also supports namespacing.
import { subspaced } from 'redux-subspace'
import { SubComponent } from 'some-dependency'
const SubspacedSubComponent = subspaced(state => state.subComponent, 'myComponent')(SubComponent)
Any actions dispatched by your thunks are wrapped with the same subspace and namespacing rules as standard actions. If they use the getState
function, they will receive the state provided by the subspace.
When nesting subspaces, the root
node will reflect the top most root state. Namespaced actions and reducers will be prepended with the parent's namespace, if provided.
Examples can be found here.
root
as a field in your state. It will be replaced with the root of the state tree. Sorry.FAQs
Create isolated subspaces of a Redux store
The npm package redux-subspace receives a total of 1,136 weekly downloads. As such, redux-subspace popularity was classified as popular.
We found that redux-subspace demonstrated a not healthy version release cadence and project activity because the last version was released a year ago. It has 4 open source maintainers collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
GitHub removed 27 malicious pull requests attempting to inject harmful code across multiple open source repositories, in another round of low-effort attacks.
Security News
RubyGems.org has added a new "maintainer" role that allows for publishing new versions of gems. This new permission type is aimed at improving security for gem owners and the service overall.
Security News
Node.js will be enforcing stricter semver-major PR policies a month before major releases to enhance stability and ensure reliable release candidates.