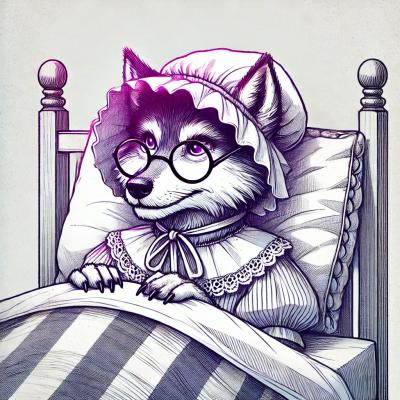
Security News
GitHub Removes Malicious Pull Requests Targeting Open Source Repositories
GitHub removed 27 malicious pull requests attempting to inject harmful code across multiple open source repositories, in another round of low-effort attacks.
regex-cache
Advanced tools
Memoize the results of a call to the RegExp constructor, avoiding repetitious runtime compilation of the same string and options, resulting in dramatic speed improvements.
The regex-cache npm package is designed to cache the results of regular expression operations to improve performance. It is particularly useful when the same regular expressions are used repeatedly, as it avoids the overhead of recompiling the regex each time.
Caching Regular Expressions
This feature allows you to cache a regular expression. The first time the regex is used, it is compiled and cached. Subsequent uses of the same regex will retrieve it from the cache, improving performance.
const regexCache = require('regex-cache');
const re = regexCache(/foo/);
console.log(re.test('foo')); // true
Using Cached Regular Expressions
This feature demonstrates that the same regular expression is retrieved from the cache, ensuring that the same instance is used, which can save memory and processing time.
const regexCache = require('regex-cache');
const re1 = regexCache(/foo/);
const re2 = regexCache(/foo/);
console.log(re1 === re2); // true
The lru-cache package provides a least-recently-used (LRU) cache, which can be used to store any kind of data, including regular expressions. Unlike regex-cache, lru-cache is more general-purpose and can be used for caching various types of data.
The memoizee package is a general-purpose memoization library that can cache the results of function calls. It can be used to cache the results of functions that create regular expressions, providing similar performance benefits to regex-cache but with more flexibility.
The fast-memoize package is another memoization library focused on speed. It can be used to memoize functions that generate regular expressions, offering a high-performance alternative to regex-cache for caching regex results.
Memoize the results of a call to the RegExp constructor, avoiding repetitious runtime compilation of the same string and options, resulting in dramatic speed improvements.
npm i regex-cache --save
Wrap a function like this:
var cache = require('regex-cache');
var someRegex = cache(require('some-regex-lib'));
Caching a regex
If you want to cache a regex after calling new RegExp()
, or you're requiring a module that returns a regex, wrap it with a function first:
var cache = require('regex-cache');
function yourRegex(str, opts) {
// do stuff to str and opts
return new RegExp(str, opts.flags);
}
var regex = cache(yourRegex);
Performance results, with and without regex-cache:
# no args passed (defaults)
with-cache x 8,699,231 ops/sec ±0.86% (93 runs sampled)
without-cache x 2,777,551 ops/sec ±0.63% (95 runs sampled)
# string and six options passed
with-cache x 1,885,934 ops/sec ±0.80% (93 runs sampled)
without-cache x 1,256,893 ops/sec ±0.65% (97 runs sampled)
# string only
with-cache x 7,723,256 ops/sec ±0.87% (92 runs sampled)
without-cache x 2,303,060 ops/sec ±0.47% (99 runs sampled)
# one option passed
with-cache x 4,179,877 ops/sec ±0.53% (100 runs sampled)
without-cache x 2,198,422 ops/sec ±0.47% (95 runs sampled)
# two options passed
with-cache x 3,256,222 ops/sec ±0.51% (99 runs sampled)
without-cache x 2,121,401 ops/sec ±0.79% (97 runs sampled)
# six options passed
with-cache x 1,816,018 ops/sec ±1.08% (96 runs sampled)
without-cache x 1,157,176 ops/sec ±0.53% (100 runs sampled)
#
# diminishing returns happen about here
#
# ten options passed
with-cache x 1,210,598 ops/sec ±0.56% (92 runs sampled)
without-cache x 1,665,588 ops/sec ±1.07% (100 runs sampled)
# twelve options passed
with-cache x 1,042,096 ops/sec ±0.68% (92 runs sampled)
without-cache x 1,389,414 ops/sec ±0.68% (97 runs sampled)
# twenty options passed
with-cache x 661,125 ops/sec ±0.80% (93 runs sampled)
without-cache x 1,208,757 ops/sec ±0.65% (97 runs sampled)
#
# when non-primitive values are compared
#
# single value on the options is an object
with-cache x 1,398,313 ops/sec ±1.05% (95 runs sampled)
without-cache x 2,228,281 ops/sec ±0.56% (99 runs sampled)
Install dev dependencies:
npm i -d && npm run benchmarks
If you're using new RegExp('foo')
instead of a regex literal, it's probably because you need to dyamically generate a regex based on user options or some other potentially changing factors.
When your function creates a string based on user inputs and passes it to the RegExp
constructor, regex-cache caches the results. The next time the function is called if the key of a cached regex matches the user input (or no input was given), the cached regex is returned, avoiding unnecessary runtime compilation.
Using the RegExp constructor offers a lot of flexibility, but the runtime compilation comes at a price - it's slow. Not specifically because of the call to the RegExp constructor, but because you have to build up the string before new RegExp()
is even called.
Install dev dependencies:
npm i -d && npm test
Pull requests and stars are always welcome. For bugs and feature requests, please create an issue
Jon Schlinkert
Copyright (c) 2015 Jon Schlinkert
Released under the MIT license
This file was generated by verb-cli on March 25, 2015.
FAQs
Memoize the results of a call to the RegExp constructor, avoiding repetitious runtime compilation of the same string and options, resulting in surprising performance improvements.
The npm package regex-cache receives a total of 0 weekly downloads. As such, regex-cache popularity was classified as not popular.
We found that regex-cache demonstrated a not healthy version release cadence and project activity because the last version was released a year ago. It has 2 open source maintainers collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
GitHub removed 27 malicious pull requests attempting to inject harmful code across multiple open source repositories, in another round of low-effort attacks.
Security News
RubyGems.org has added a new "maintainer" role that allows for publishing new versions of gems. This new permission type is aimed at improving security for gem owners and the service overall.
Security News
Node.js will be enforcing stricter semver-major PR policies a month before major releases to enhance stability and ensure reliable release candidates.